Separation of concerns between business layer, data layer and presentation layer without losing information
I'm developing an api in Fast API using sqlalchemy to manage my ORM classes and operations with the database. I'm dealing with some design decisions to have my classes as little coupled as possible without losing information. For example, this is one of my entities called Topic this class defined in models.py and is supposed to be use by the TopicRepository class Topic(Base): __tablename__ = "topics" id = Column(Integer, primary_key=True, autoincrement=True) name = Column(String, unique=True) category_id = Column( Integer, ForeignKey("categories.id", ondelete="CASCADE"), nullable=False ) category = relationship("Category", back_populates="topic", lazy="subquery") I have some "core" classes where this are supposed to live in the business layer and is used by the TopicService, for example class Topic: def __init__(self, id: int, title: str, cost: int = 0, capacity=0, category=None): self._id = id self._title = title self._category = category self._capacity = capacity # properties # methods for Topic The presentation layer which is the TopicRouter uses these classes for making the json responses for different kinds of topic request. schemas.py class SimpleTopic(BaseModel): """Represents a simple topic with just a name""" name: str model_config = ConfigDict(from_attributes=True) class TopicRequest(SimpleTopic): category: str class TopicResponse(SimpleTopic): id: int category: SimpleCategory = Field(validation_alias="category") class TopicList(RootModel): root: List[TopicResponse] def __iter__(self): return iter(self.root) So, my doubts here are: Is this kind of separation, ok? Should the TopicService know the classes from schemas.py, models.py and topic.py in order to mapped from one to the other? ORM objects should not be used as domain classes so in that case, do I need to duplicate each class? Thanks! :)
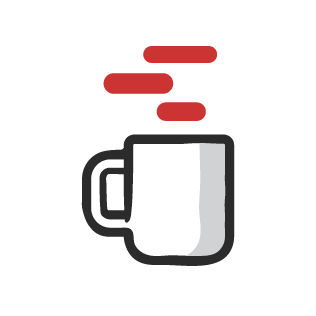
I'm developing an api in Fast API using sqlalchemy to manage my ORM classes and operations with the database.
I'm dealing with some design decisions to have my classes as little coupled as possible without losing information.
For example, this is one of my entities called Topic
this class defined in models.py and is supposed to be use by the TopicRepository
class Topic(Base):
__tablename__ = "topics"
id = Column(Integer, primary_key=True, autoincrement=True)
name = Column(String, unique=True)
category_id = Column(
Integer, ForeignKey("categories.id", ondelete="CASCADE"), nullable=False
)
category = relationship("Category", back_populates="topic", lazy="subquery")
I have some "core" classes where this are supposed to live in the business layer and is used by the TopicService, for example
class Topic:
def __init__(self, id: int, title: str, cost: int = 0, capacity=0, category=None):
self._id = id
self._title = title
self._category = category
self._capacity = capacity
# properties
# methods for Topic
The presentation layer which is the TopicRouter uses these classes for making the json responses for different kinds of topic request. schemas.py
class SimpleTopic(BaseModel):
"""Represents a simple topic with just a name"""
name: str
model_config = ConfigDict(from_attributes=True)
class TopicRequest(SimpleTopic):
category: str
class TopicResponse(SimpleTopic):
id: int
category: SimpleCategory = Field(validation_alias="category")
class TopicList(RootModel):
root: List[TopicResponse]
def __iter__(self):
return iter(self.root)
So, my doubts here are:
- Is this kind of separation, ok?
- Should the TopicService know the classes from schemas.py, models.py and topic.py in order to mapped from one to the other?
- ORM objects should not be used as domain classes so in that case, do I need to duplicate each class?
Thanks! :)