Resolving CORS Errors in a NestJS App Deployed on Vercel
When deploying a NestJS application on Vercel, you may encounter CORS (Cross-Origin Resource Sharing) issues, particularly when your API is accessed from different domains. To resolve these issues and allow your application to accept cross-origin requests, you can configure the necessary CORS headers in your Vercel deployment settings. Why CORS is Important CORS is a security mechanism that allows servers to control which domains are permitted to access their resources. This is important to prevent malicious websites from interacting with your server on behalf of the user without their consent. When you deploy a NestJS application and try to access its API from another domain, the browser sends a preflight request to check if the server allows it. If the server doesn't respond with the correct headers, you’ll encounter CORS errors. The Solution In Vercel, you can manage CORS headers using a combination of rewrites and headers in your vercel.json configuration file. This configuration will allow your API to accept requests from any domain, which is particularly useful during development or when you want to expose your API to the public. Here’s a step-by-step guide on how to configure CORS headers in your Vercel deployment: 1. Update Your vercel.json Configuration You can set up rewrites and headers in the vercel.json file. This will ensure that all incoming requests to your Vercel server will correctly include the necessary CORS headers. Here’s an example configuration: { "rewrites": [ { "source": "/(.*)", "destination": "/api" } ], "headers": [ { "source": "/(.*)", "headers": [ { "key": "Access-Control-Allow-Credentials", "value": "true" }, { "key": "Access-Control-Allow-Origin", "value": "*" }, { "key": "Access-Control-Allow-Methods", "value": "GET,OPTIONS,PATCH,DELETE,POST,PUT" }, { "key": "Access-Control-Allow-Headers", "value": "X-CSRF-Token, X-Requested-With, Accept, Accept-Version, Content-Length, Content-MD5, Content-Type, Date, X-Api-Version" } ] } ] } Explanation of the Configuration: Rewrites: This part of the configuration ensures that all incoming requests to any path (/(.*)) are rewritten to the /api route. You can adjust the destination based on your application structure. Headers: The headers section defines the CORS headers that will be sent with every request: Access-Control-Allow-Credentials: Allows cookies and credentials to be included in the request. Access-Control-Allow-Origin: Specifies which origins are allowed to access the resources. Setting it to * allows requests from any domain. Be cautious with this in production environments, as it might open up your API to abuse. Access-Control-Allow-Methods: Specifies which HTTP methods are allowed when making requests. In this case, it allows all common HTTP methods. Access-Control-Allow-Headers: Specifies which headers can be used in the actual request. 2. Deploy Your Changes to Vercel Once you've updated the vercel.json file, deploy your changes to Vercel. This will automatically apply the CORS configuration, allowing your application to accept cross-origin requests from any domain. Regards, N I Rimon
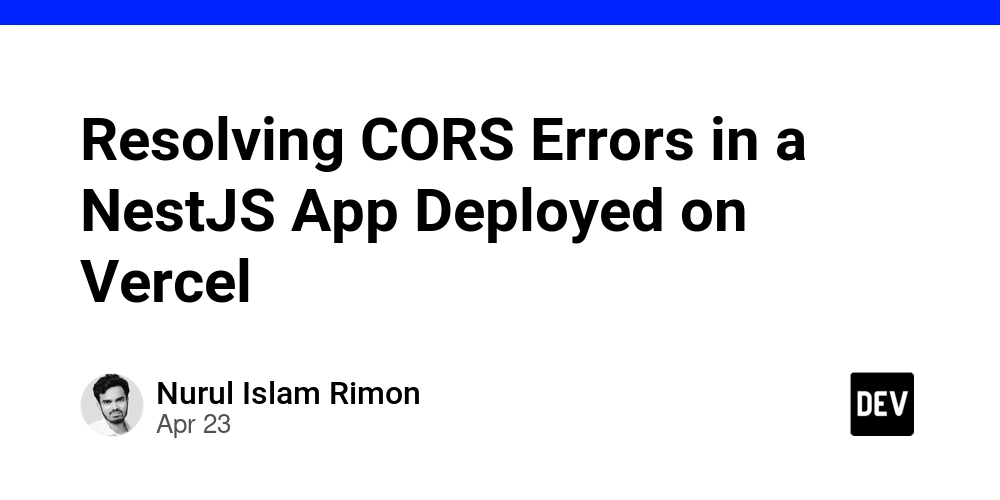
When deploying a NestJS application on Vercel, you may encounter CORS (Cross-Origin Resource Sharing) issues, particularly when your API is accessed from different domains. To resolve these issues and allow your application to accept cross-origin requests, you can configure the necessary CORS headers in your Vercel deployment settings.
Why CORS is Important
CORS is a security mechanism that allows servers to control which domains are permitted to access their resources. This is important to prevent malicious websites from interacting with your server on behalf of the user without their consent. When you deploy a NestJS application and try to access its API from another domain, the browser sends a preflight request to check if the server allows it. If the server doesn't respond with the correct headers, you’ll encounter CORS errors.
The Solution
In Vercel, you can manage CORS headers using a combination of rewrites and headers in your vercel.json configuration file. This configuration will allow your API to accept requests from any domain, which is particularly useful during development or when you want to expose your API to the public.
Here’s a step-by-step guide on how to configure CORS headers in your Vercel deployment:
1. Update Your vercel.json Configuration
You can set up rewrites and headers in the vercel.json file. This will ensure that all incoming requests to your Vercel server will correctly include the necessary CORS headers.
Here’s an example configuration:
{
"rewrites": [
{
"source": "/(.*)",
"destination": "/api"
}
],
"headers": [
{
"source": "/(.*)",
"headers": [
{ "key": "Access-Control-Allow-Credentials", "value": "true" },
{ "key": "Access-Control-Allow-Origin", "value": "*" },
{
"key": "Access-Control-Allow-Methods",
"value": "GET,OPTIONS,PATCH,DELETE,POST,PUT"
},
{
"key": "Access-Control-Allow-Headers",
"value": "X-CSRF-Token, X-Requested-With, Accept, Accept-Version, Content-Length, Content-MD5, Content-Type, Date, X-Api-Version"
}
]
}
]
}
Explanation of the Configuration:
- Rewrites:
This part of the configuration ensures that all incoming requests to any path (/(.*)) are rewritten to the /api route. You can adjust the destination based on your application structure.
Headers:
The headers section defines the CORS headers that will be sent with every request:
Access-Control-Allow-Credentials: Allows cookies and credentials to be included in the request.
Access-Control-Allow-Origin: Specifies which origins are allowed to access the resources. Setting it to * allows requests from any domain. Be cautious with this in production environments, as it might open up your API to abuse.
Access-Control-Allow-Methods: Specifies which HTTP methods are allowed when making requests. In this case, it allows all common HTTP methods.
Access-Control-Allow-Headers: Specifies which headers can be used in the actual request.
2. Deploy Your Changes to Vercel
Once you've updated the vercel.json file, deploy your changes to Vercel. This will automatically apply the CORS configuration, allowing your application to accept cross-origin requests from any domain.
Regards,
N I Rimon