Handling Large Datasets in PHP Without Running Out of Memory
One major problem developers have while working with PHP is managing big databases effectively without experiencing memory decline. Whether you're searching a large database or importing millions of entries from a CSV file, memory management becomes essential. This article examines reliable methods for managing big datasets in PHP in an efficient and sustainable manner, along with thorough explanations, useful code samples, and expert advice. Why PHP Struggles with Large Datasets PHP scripts are not by default designed to handle workloads requiring a lot of memory. Scripts are frequently run with memory and execution time limits specified in php.ini, and PHP operates in a sandbox. If you're not careful with data handling, you may run into problems when working with large datasets. Typical symptoms include: Allowed memory size of x bytes exhausted Noticeable slowdown in execution Web or CLI script timeouts The first step towards effectively managing big datasets is to comprehend PHP's memory mechanism and steer clear of needless memory usage. Proven Techniques for Handling Large Datasets 1. Temporarily Increase PHP Memory Limit Sometimes, increasing the memory limit is a quick way to avoid immediate failure. However, this is not a scalable or recommended long-term solution. ini_set('memory_limit', '512M'); Use this approach sparingly and only if you’re certain that your script’s architecture warrants additional memory. 2. Leverage Generators for Memory-Efficient Iteration PHP generators let you loop through data without putting the complete dataset into memory. They provide values one at a time rather than an array. function readLargeCSV($filePath) { $handle = fopen($filePath, 'r'); while (($data = fgetcsv($handle)) !== false) { yield $data; } fclose($handle); } foreach (readLargeCSV('large_file.csv') as $row) { // Process each row as needed } Why it works: Generators use a lazy-loading pattern, dramatically reducing memory usage. 3. Stream Files Instead of Loading Entirely If you’re working with large files, always use streaming functions like fgets(), fread(), or stream_get_line(). $handle = fopen("huge_text_file.txt", "r"); if ($handle) { while (($line = fgets($handle)) !== false) { // Process the current line } fclose($handle); } This method ensures that only a small portion of the file is loaded at any given time. 4. Execute Chunked Database Queries Avoid pulling millions of rows in a single query. Use chunking with LIMIT and OFFSET or better yet, indexed pagination. $limit = 1000; $offset = 0; while (true) { $stmt = $pdo->prepare("SELECT * FROM large_table LIMIT :limit OFFSET :offset"); $stmt->bindValue(':limit', $limit, PDO::PARAM_INT); $stmt->bindValue(':offset', $offset, PDO::PARAM_INT); $stmt->execute(); $rows = $stmt->fetchAll(PDO::FETCH_ASSOC); if (count($rows) === 0) break; foreach ($rows as $row) { // Process row } $offset += $limit; } Pro Tip: Avoid using OFFSET with huge datasets; instead use indexed id based pagination for better performance. 5. Use Cursor-Based Iteration (Indexed Pagination) Large datasets are traversed consistently and efficiently with cursor-based iteration. $lastId = 0; $limit = 1000; while (true) { $stmt = $pdo->prepare("SELECT * FROM large_table WHERE id > :lastId ORDER BY id ASC LIMIT :limit"); $stmt->bindValue(':lastId', $lastId, PDO::PARAM_INT); $stmt->bindValue(':limit', $limit, PDO::PARAM_INT); $stmt->execute(); $rows = $stmt->fetchAll(PDO::FETCH_ASSOC); if (empty($rows)) break; foreach ($rows as $row) { $lastId = $row['id']; // Handle each record efficiently } } 6. Run Long-Processing Tasks via CLI Scripts Avoid using web requests for demanding tasks. Incorporate scripts for the command-line interface (CLI): php process_large_dataset.php Better resource management is ensured, and browser-related execution time constraints are eliminated. 7. Offload Heavy Tasks to Specialized Tools Some operations are best handled outside PHP: Use awk, sed, or grep for pre-processing text files. Use Python or Go for computation-heavy logic. Use stored procedures for processing within the database. Integrating such tools can significantly improve performance. 8. Profile and Monitor Your Scripts Understanding your script’s memory profile is essential: echo "Memory usage: " . memory_get_usage(true) . " bytes\n"; echo "Peak usage: " . memory_get_peak_usage(true) . " bytes\n"; Also consider: Xdebug for advanced profiling Blackfire.io for performance tuning Best Practices Recap ✅ Stream data incrementally — avoid full file loads ✅ Use generators and cursors where possible ✅ Always profile and monitor ✅ Use CLI over HTTP for intensive jo
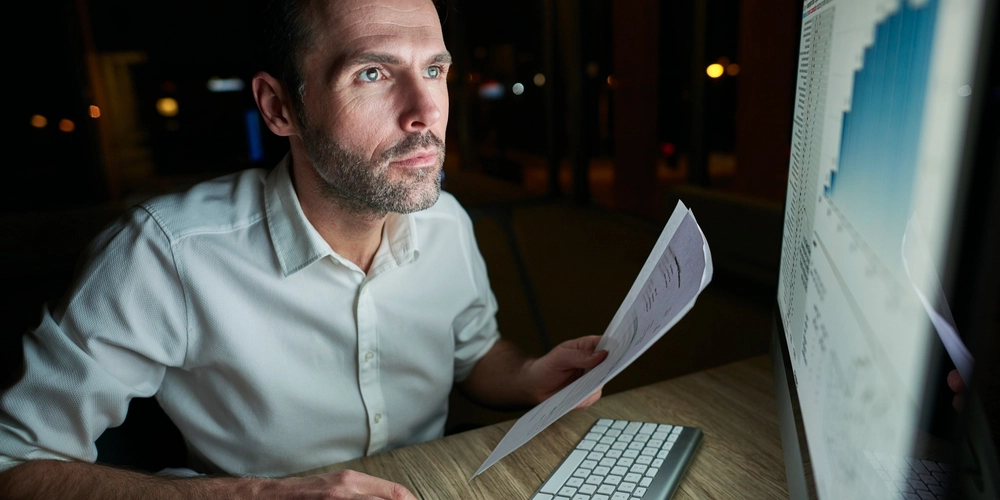
One major problem developers have while working with PHP is managing big databases effectively without experiencing memory decline. Whether you're searching a large database or importing millions of entries from a CSV file, memory management becomes essential.
This article examines reliable methods for managing big datasets in PHP in an efficient and sustainable manner, along with thorough explanations, useful code samples, and expert advice.
Why PHP Struggles with Large Datasets
PHP scripts are not by default designed to handle workloads requiring a lot of memory. Scripts are frequently run with memory and execution time limits specified in php.ini, and PHP operates in a sandbox. If you're not careful with data handling, you may run into problems when working with large datasets.
Typical symptoms include:
- Allowed memory size of x bytes exhausted
- Noticeable slowdown in execution
- Web or CLI script timeouts
The first step towards effectively managing big datasets is to comprehend PHP's memory mechanism and steer clear of needless memory usage.
Proven Techniques for Handling Large Datasets
1. Temporarily Increase PHP Memory Limit
Sometimes, increasing the memory limit is a quick way to avoid immediate failure. However, this is not a scalable or recommended long-term solution.
ini_set('memory_limit', '512M');
Use this approach sparingly and only if you’re certain that your script’s architecture warrants additional memory.
2. Leverage Generators for Memory-Efficient Iteration
PHP generators let you loop through data without putting the complete dataset into memory. They provide values one at a time rather than an array.
function readLargeCSV($filePath) {
$handle = fopen($filePath, 'r');
while (($data = fgetcsv($handle)) !== false) {
yield $data;
}
fclose($handle);
}
foreach (readLargeCSV('large_file.csv') as $row) {
// Process each row as needed
}
Why it works: Generators use a lazy-loading pattern, dramatically reducing memory usage.
3. Stream Files Instead of Loading Entirely
If you’re working with large files, always use streaming functions like fgets()
, fread()
, or stream_get_line()
.
$handle = fopen("huge_text_file.txt", "r");
if ($handle) {
while (($line = fgets($handle)) !== false) {
// Process the current line
}
fclose($handle);
}
This method ensures that only a small portion of the file is loaded at any given time.
4. Execute Chunked Database Queries
Avoid pulling millions of rows in a single query. Use chunking with LIMIT
and OFFSET
or better yet, indexed pagination.
$limit = 1000;
$offset = 0;
while (true) {
$stmt = $pdo->prepare("SELECT * FROM large_table LIMIT :limit OFFSET :offset");
$stmt->bindValue(':limit', $limit, PDO::PARAM_INT);
$stmt->bindValue(':offset', $offset, PDO::PARAM_INT);
$stmt->execute();
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC);
if (count($rows) === 0) break;
foreach ($rows as $row) {
// Process row
}
$offset += $limit;
}
Pro Tip: Avoid using OFFSET
with huge datasets; instead use indexed id
based pagination for better performance.
5. Use Cursor-Based Iteration (Indexed Pagination)
Large datasets are traversed consistently and efficiently with cursor-based iteration.
$lastId = 0;
$limit = 1000;
while (true) {
$stmt = $pdo->prepare("SELECT * FROM large_table WHERE id > :lastId ORDER BY id ASC LIMIT :limit");
$stmt->bindValue(':lastId', $lastId, PDO::PARAM_INT);
$stmt->bindValue(':limit', $limit, PDO::PARAM_INT);
$stmt->execute();
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC);
if (empty($rows)) break;
foreach ($rows as $row) {
$lastId = $row['id'];
// Handle each record efficiently
}
}
6. Run Long-Processing Tasks via CLI Scripts
Avoid using web requests for demanding tasks. Incorporate scripts for the command-line interface (CLI):
php process_large_dataset.php
Better resource management is ensured, and browser-related execution time constraints are eliminated.
7. Offload Heavy Tasks to Specialized Tools
Some operations are best handled outside PHP:
- Use
awk
,sed
, orgrep
for pre-processing text files. - Use Python or Go for computation-heavy logic.
- Use stored procedures for processing within the database.
Integrating such tools can significantly improve performance.
8. Profile and Monitor Your Scripts
Understanding your script’s memory profile is essential:
echo "Memory usage: " . memory_get_usage(true) . " bytes\n";
echo "Peak usage: " . memory_get_peak_usage(true) . " bytes\n";
Also consider:
- Xdebug for advanced profiling
- Blackfire.io for performance tuning
Best Practices Recap
- ✅ Stream data incrementally — avoid full file loads
- ✅ Use generators and cursors where possible
- ✅ Always profile and monitor
- ✅ Use CLI over HTTP for intensive jobs
- ✅ Clean up with
unset()
andgc_collect_cycles()
Final Thoughts
Using PHP to handle big datasets is not only feasible, but it's also effective and scalable when done correctly. PHP is capable of handling large data operations with confidence by fusing memory-conscious patterns like streaming, chunking, and generators with top-notch tools and external processing where necessary.
Think PHP is old school? Think again. In 2025, PHP isn’t just alive — it’s evolving, thriving, and innovating with frameworks that make modern web development faster, cleaner, and more scalable than ever. Detailed Version of PHP & its trending frameworks.
Continue to test, optimize, and always consider scale when designing.