Ready to become a real developer study -day01
Java History It was developed by SUN(Standford Univeristy Network) company by James Gosling. Sun company was bought by Oracle in 2009. What is Java? Java is a programming language understood by human being to write scripts for computer to execute. However, computer does not understand the scripts written by java or by human. Therefore, the scripts written by human needs to be compiled to machine language. ![[Pasted image 20250416122219.png]] Computer is composed by different types of CPU, GPU,chips, etc. To accomplish same goals/tasks, different hardware needs different commands to execute tasks. How about using same scripts running on different computers to execute the same tasks? we need a third-party agent to help it. **JVM(java virtual machine) java scripts can run on JVM and JVM can execute the compiled .class codes on different computers/platforms. It is platform-independent — meaning you can write Java code once and run it anywhere. Java code isn’t run directly by your computer — the JVM runs it, acting as a middle layer between your code and the hardware. What Does the JVM Do? ==Loads== Java bytecode (compiled .class files) -> ==not compile== code to .class file. Verifies the bytecode to ensure it’s safe Executes the bytecode (either by interpreting it or compiling it just-in-time to native code) Manages memory, including garbage collection Provides a runtime environment for Java programs JVM Internal Structure (Visual Style) ![[Pasted image 20250416141051.png]] How to view threads running ? `public class Main { public static void main(String[] args) { System.out.println("主线程开始运行"); new Thread(() -> { try { Thread.sleep(5000); // 睡 5 秒 } catch (InterruptedException e) { e.printStackTrace(); } }, "My-Custom-Thread").start(); Thread.getAllStackTraces().keySet().forEach(thread -> { System.out.println("线程名: " + thread.getName() + ",是否守护线程: " + thread.isDaemon() + ",优先级: " + thread.getPriority()); }); System.out.println("主线程结束"); } }` ![[Pasted image 20250416142047.png]] Other relative concepts JDK=java development kit is the most popular SDK(software develpoment kit). Commonly used JDK version: JAVA SE 8, JAVA SE 11, JAVA SE 17. JRE (Java Runtime Environment): The JRE is a software package that provides the environment required to run Java applications. It contains everything you need to run Java programs, including the JVM, libraries(Java class libraries like java.util, java.io, etc.), and other components(configuration files and other resources needed to run Java programs) required for running Java programs. ![[Pasted image 20250416142535.png]] Intellij IDEA shotcuts: ctrl + D : copy code to next line ctrl + Y: delete code line
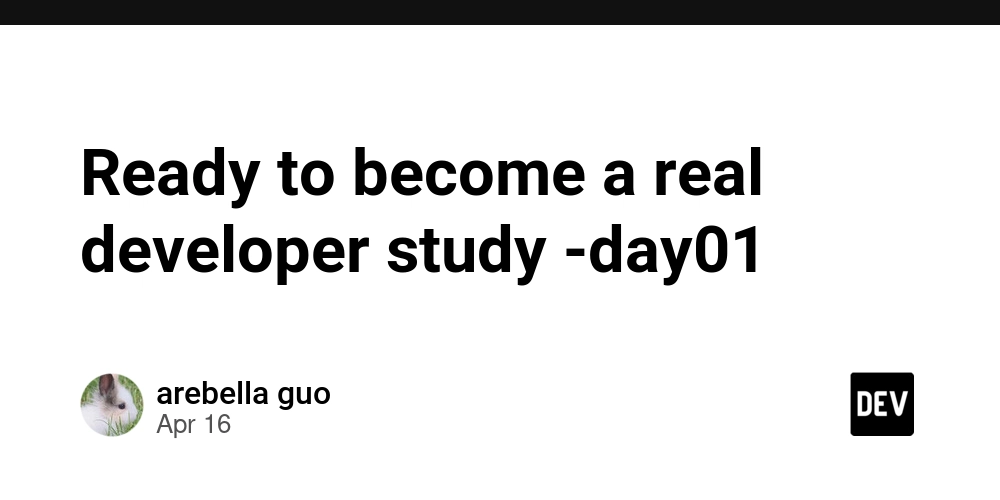
Java History
It was developed by SUN(Standford Univeristy Network) company by James Gosling. Sun company was bought by Oracle in 2009.
What is Java?
Java is a programming language understood by human being to write scripts for computer to execute. However, computer does not understand the scripts written by java or by human. Therefore, the scripts written by human needs to be compiled to machine language.
![[Pasted image 20250416122219.png]]
Computer is composed by different types of CPU, GPU,chips, etc. To accomplish same goals/tasks, different hardware needs different commands to execute tasks.
How about using same scripts running on different computers to execute the same tasks? we need a third-party agent to help it.
**JVM(java virtual machine)
java scripts can run on JVM and JVM can execute the compiled .class codes on different computers/platforms. It is platform-independent — meaning you can write Java code once and run it anywhere. Java code isn’t run directly by your computer — the JVM runs it, acting as a middle layer between your code and the hardware.
What Does the JVM Do?
==Loads== Java bytecode (compiled .class files) -> ==not compile== code to .class file.
Verifies the bytecode to ensure it’s safe
Executes the bytecode (either by interpreting it or compiling it just-in-time to native code)
Manages memory, including garbage collection
Provides a runtime environment for Java programs
JVM Internal Structure (Visual Style)
![[Pasted image 20250416141051.png]]
How to view threads running ?
`public class Main {
public static void main(String[] args) {
System.out.println("主线程开始运行");
new Thread(() -> {
try {
Thread.sleep(5000); // 睡 5 秒
} catch (InterruptedException e) {
e.printStackTrace();
}
}, "My-Custom-Thread").start();
Thread.getAllStackTraces().keySet().forEach(thread -> {
System.out.println("线程名: " + thread.getName() +
",是否守护线程: " + thread.isDaemon() +
",优先级: " + thread.getPriority());
});
System.out.println("主线程结束");
}
}`
![[Pasted image 20250416142047.png]]
Other relative concepts
JDK=java development kit is the most popular SDK(software develpoment kit). Commonly used JDK version: JAVA SE 8, JAVA SE 11, JAVA SE 17.
JRE (Java Runtime Environment): The JRE is a software package that provides the environment required to run Java applications.
It contains everything you need to run Java programs, including the JVM, libraries(Java class libraries like
java.util
,java.io
, etc.), and other components(configuration files and other resources needed to run Java programs) required for running Java programs.
![[Pasted image 20250416142535.png]]
Intellij IDEA shotcuts:ctrl + D : copy code to next line
ctrl + Y: delete code line