Performance optimization using React-Compiler
So, let me share a real story from my own project. I was working on my subscription tracking app something I built to keep tack of all the services I use (like Spotify and Netflix). Everything was going fine until I added a search bar to filter subscriptions. At first, I thought, “This is going to be a simple input with some filtering logic no big deal.” But as soon as I started typing into the search field, something weird happened… My entire page was re-rendering. Every time I typed a single letter, boom all the subscription cards (Spotify, Netflix, etc.) were re-rendering. It didn’t matter if the card was related to the search or not. Even if I typed something that didn’t match any subscription at all, everything still updated. Here’s what I saw: FPS dropped slightly when typing fast. Even the components that didn’t change were updating. And yeah, it felt like overkill for just a simple search bar. I used useState to store the search input and updated it on every keystroke which is pretty standard. But I quickly realized how that single state change was triggering a domino effect of re-renders. That’s when I started thinking: There has to be a smarter way to handle this. And that makes sense because that's how react works it renders from Top to bottom whenever the props change and re-renders every UI component even the components that didn’t change were updating. But that this will slow down my application performance in the long run. The Traditional way for handling this was these issue was wrapping my Buttons and Cards into useMemo and React.memo And then I tried the React Compiler for the first time, just out of curiosity. I wasn’t expecting anything crazy just thought I’d check out what the hype was about. But honestly? I was blown away. So, What Is the React Compiler? The React Compiler is a new tool created by the React team that helps your app perform better by automatically optimizing how your components update. Think of it like a smart assistant that watches your code and says, "Hey, this part doesn’t need to run again—let me handle that for you." It removes the need to manually memorize functions or cache values using hooks like useMemo or useCallback. You write plain, clean React code—and it handles performance in the background. What React Compiler Does Differently Instead of you worrying about memoization, React Compiler does it for you—automatically. Here’s what it does: It analyzes your code during the build process. It understands what actually changes in your component. It adds memoization behind the scenes, so your component only re-renders when it really needs to. This means: No more useCallback to keep function references stable. No more useMemo for caching values. No need to wrap everything in React.memo. You just write code, and React Compiler takes care of the optimization. Final Thoughts React Compiler feels like one of those game-changing features that silently upgrades your development experience. You write regular, simple React code—and it takes care of performance like a pro. It now doesn't re-render all the cards again and again. If you haven’t tried it yet, give it a spin. I’m telling you—once you see it in action, you won’t want to go back to manually optimizing everything again.
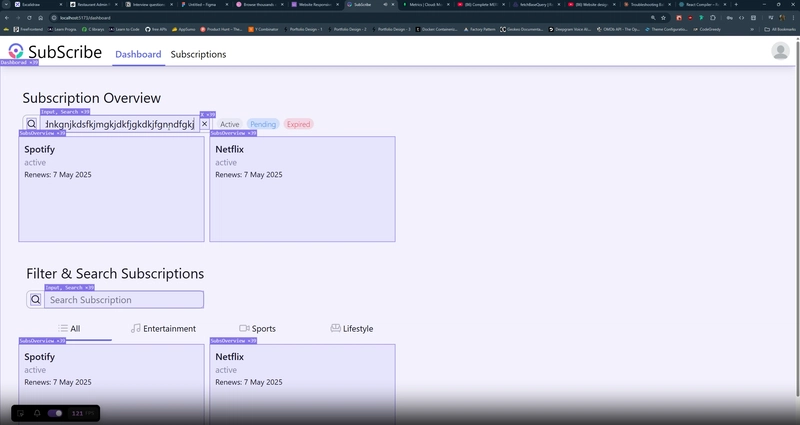
So, let me share a real story from my own project.
I was working on my subscription tracking app something I built to keep tack of all the services I use (like Spotify and Netflix). Everything was going fine until I added a search bar to filter subscriptions.
At first, I thought, “This is going to be a simple input with some filtering logic no big deal.” But as soon as I started typing into the search field, something weird happened…
My entire page was re-rendering.
Every time I typed a single letter, boom all the subscription cards (Spotify, Netflix, etc.) were re-rendering. It didn’t matter if the card was related to the search or not. Even if I typed something that didn’t match any subscription at all, everything still updated.
Here’s what I saw:
FPS dropped slightly when typing fast.
Even the components that didn’t change were updating.
And yeah, it felt like overkill for just a simple search bar.
I used useState to store the search input and updated it on every keystroke which is pretty standard. But I quickly realized how that single state change was triggering a domino effect of re-renders.
That’s when I started thinking:
There has to be a smarter way to handle this.
And that makes sense because that's how react works it renders from Top to bottom whenever the props change and re-renders every UI component even the components that didn’t change were updating. But that this will slow down my application performance in the long run.
The Traditional way for handling this was these issue was wrapping my Buttons and Cards into useMemo and React.memo
And then I tried the React Compiler for the first time, just out of curiosity. I wasn’t expecting anything crazy just thought I’d check out what the hype was about.
But honestly? I was blown away.
So, What Is the React Compiler?
The React Compiler is a new tool created by the React team that helps your app perform better by automatically optimizing how your components update.
Think of it like a smart assistant that watches your code and says,
"Hey, this part doesn’t need to run again—let me handle that for you."
It removes the need to manually memorize functions or cache values using hooks like useMemo or useCallback. You write plain, clean React code—and it handles performance in the background.
What React Compiler Does Differently
Instead of you worrying about memoization, React Compiler does it for you—automatically.
Here’s what it does:
It analyzes your code during the build process.
It understands what actually changes in your component.
It adds memoization behind the scenes, so your component only re-renders when it really needs to.
This means:
No more useCallback to keep function references stable.
No more useMemo for caching values.
No need to wrap everything in React.memo.
You just write code, and React Compiler takes care of the optimization.
Final Thoughts
React Compiler feels like one of those game-changing features that silently upgrades your development experience. You write regular, simple React code—and it takes care of performance like a pro.
It now doesn't re-render all the cards again and again.
If you haven’t tried it yet, give it a spin. I’m telling you—once you see it in action, you won’t want to go back to manually optimizing everything again.