My Type of Library: Axios TypeScript Conversion
This is the first post in an ad-hoc series where I convert libraries to TypeScript, and analyze the results. In the same vein as my re-conversion of the Turbo Framework to TypeScript, I decided to take a popular JavaScript library, this time one that had always been in JavaScript, and see what converting the codebase to TypeScript revealed. I chose Axios, because it’s: Popular, well used, extensively developed Written in JavaScript, with a manually generated TypeScript definitions file Small enough to be easily convertible, and large enough to be interesting The functional issues revealed during the conversion show the flexibility of JavaScript and the clever “tricks” we often perform with it. In highlighting the issues, I utilized AI agents through the Windsurf editor, and when it offered suggestions for fixes, I realized that even with the extensive context provided by the codebase, its solutions were still sub-par and illustrative of the limits of how AI can help with TypeScript problems. To that end, I’ll highlight some of the issues found in the conversion that necessitate functional changes, referencing both my converted code and the Axios main branch as of this writing. I’ll also compare the solutions to these issues that were provided by Claude 3.7, and contrast them with my preferred solutions to show how Claude conceives of the code and solutions, and how this differs from the way a skilled developer (if I do say so myself) views them. To be clear, there are many errors in my conversion. Where I left things there are 300 odd errors, and the majority of these are probably not hinting at actual issues in the library. My goal in all this is to gain insight into how people write JavaScript utilizing TypeScript as a lens, not port the entire library and tests. Instead of doing that conversion writing a book, I'll write these posts about files where the Axios rubber meets the road. The first is the "fetch adapter", which integrates the modern fetch browser API into Axios. Although it's not Axios's default adapter, it still provides some elucidating errors it when converted. It was also created mostly in one PR by a core maintainer, so it highlights what TypeScript can do on the single developer, small scale. In the next post I'll take a look at another file, one that can tell us more about TypeScript in an active historical codebase, and when looked at in concert with the git blame and the associated pull requests, can give us an idea of how issues organically come about. TS2322: Type is not assignable Error: Type 'string' is not assignable to type 'ResponseType | undefined'. JS File lib/adapters/fetch.js:114 TS File: lib/adapters/fetch.ts:131 responseType = responseType ? (responseType + '').toLowerCase() : 'text'; To begin with this error, let's start with the type in question: ResponseType, which represents the different kinds of response formats that might be received from a fetch request. This comes from the index.d.ts file manually generated by the project itself: export type ResponseType = | 'arraybuffer' | 'blob' | 'document' | 'json' | 'text' | 'stream' | 'formdata'; This error comes from the fact that we take a response type, and convert it from the more specific union type to a general string by concatenating it with an empty string converting it to lowercase. The "obvious" solution, and the one recommended by Claude, is to simply say "This was a possibly incorrectly cased ResponseType, it's now a ResponseType" using as: responseType = (responseType ? (responseType + '').toLowerCase() : 'text') as ResponseType; Let's think about this for a second though, why was it incorrectly cased in the first place? And if it was incorrectly cased, is it definitely a ResponseType like value in the first place? The answer is no, it might not be. This value comes directly from the user, and tracing the path from input to this point I was unable to find any validation that ensures this value is a valid response type. Later on in the file we see that the responseType is used to access a resolver, which in turn accesses a Response method to deserialize the responseData: let responseData = await resolvers[utils.findKey(resolvers, responseType) || 'text'](response, config); If the type of response provided was incorrect, it wouldn't be found in the map of possible resolvers, and default to 'text'. What if I just typed bloob instead of blob though, does this lead to a logical error and a good user experience, as the library tries to parse my binary file as text? Probably not, and there is a better way (besides using types). A friendlier solution that is hinted at by this error is to create some validation, and implement it much earlier on when we're processing the configuration from the user. There is already some generic code for merging configuration values with defaults, it seems that a similar validateConfig.js file could b
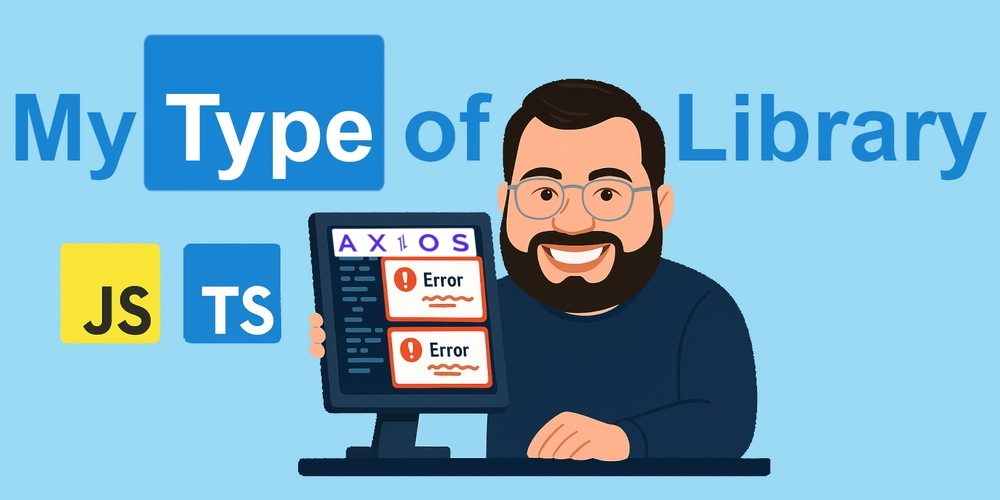
This is the first post in an ad-hoc series where I convert libraries to TypeScript, and analyze the results. In the same vein as my re-conversion of the Turbo Framework to TypeScript, I decided to take a popular JavaScript library, this time one that had always been in JavaScript, and see what converting the codebase to TypeScript revealed. I chose Axios, because it’s:
- Popular, well used, extensively developed
- Written in JavaScript, with a manually generated TypeScript definitions file
- Small enough to be easily convertible, and large enough to be interesting
The functional issues revealed during the conversion show the flexibility of JavaScript and the clever “tricks” we often perform with it. In highlighting the issues, I utilized AI agents through the Windsurf editor, and when it offered suggestions for fixes, I realized that even with the extensive context provided by the codebase, its solutions were still sub-par and illustrative of the limits of how AI can help with TypeScript problems.
To that end, I’ll highlight some of the issues found in the conversion that necessitate functional changes, referencing both my converted code and the Axios main branch as of this writing. I’ll also compare the solutions to these issues that were provided by Claude 3.7, and contrast them with my preferred solutions to show how Claude conceives of the code and solutions, and how this differs from the way a skilled developer (if I do say so myself) views them.
To be clear, there are many errors in my conversion. Where I left things there are 300 odd errors, and the majority of these are probably not hinting at actual issues in the library. My goal in all this is to gain insight into how people write JavaScript utilizing TypeScript as a lens, not port the entire library and tests.
Instead of doing that conversion writing a book, I'll write these posts about files where the Axios rubber meets the road. The first is the "fetch adapter", which integrates the modern fetch
browser API into Axios. Although it's not Axios's default adapter, it still provides some elucidating errors it when converted. It was also created mostly in one PR by a core maintainer, so it highlights what TypeScript can do on the single developer, small scale. In the next post I'll take a look at another file, one that can tell us more about TypeScript in an active historical codebase, and when looked at in concert with the git blame
and the associated pull requests, can give us an idea of how issues organically come about.
TS2322: Type is not assignable
Error: Type 'string' is not assignable to type 'ResponseType | undefined'
.
JS File lib/adapters/fetch.js:114
TS File: lib/adapters/fetch.ts:131
responseType = responseType ? (responseType + '').toLowerCase() : 'text';
To begin with this error, let's start with the type in question: ResponseType
, which represents the different kinds of response formats that might be received from a fetch request. This comes from the index.d.ts file manually generated by the project itself:
export type ResponseType =
| 'arraybuffer'
| 'blob'
| 'document'
| 'json'
| 'text'
| 'stream'
| 'formdata';
This error comes from the fact that we take a response type, and convert it from the more specific union type to a general string by concatenating it with an empty string converting it to lowercase. The "obvious" solution, and the one recommended by Claude, is to simply say "This was a possibly incorrectly cased ResponseType
, it's now a ResponseType
" using as
:
responseType = (responseType ? (responseType + '').toLowerCase() : 'text') as ResponseType;
Let's think about this for a second though, why was it incorrectly cased in the first place? And if it was incorrectly cased, is it definitely a ResponseType
like value in the first place? The answer is no, it might not be. This value comes directly from the user, and tracing the path from input to this point I was unable to find any validation that ensures this value is a valid response type.
Later on in the file we see that the responseType
is used to access a resolver
, which in turn accesses a Response
method to deserialize the responseData
:
let responseData = await resolvers[utils.findKey(resolvers, responseType) || 'text'](response, config);
If the type of response provided was incorrect, it wouldn't be found in the map of possible resolvers, and default to 'text'. What if I just typed bloob
instead of blob
though, does this lead to a logical error and a good user experience, as the library tries to parse my binary file as text? Probably not, and there is a better way (besides using types).
A friendlier solution that is hinted at by this error is to create some validation, and implement it much earlier on when we're processing the configuration from the user. There is already some generic code for merging configuration values with defaults, it seems that a similar validateConfig.js
file could be created that follows a similar pattern, and provides functions for validating each value in the passed configuration. This could throw errors at the point where the configuration is passed, improving the developer experience in my example case and others involving similarly incorrect configuration values.
TS2345: Argument is not assignable to parameter
Error: Argument of type '{ body: ReadableStream; method: string; readonly duplex: string; }'
is not assignable to parameter of type 'RequestInit'
.
JS File: lib/adapters/fetch.js:31
TS File: lib/adapters/fetch.ts:39
const supportsRequestStream = isReadableStreamSupported && test(() => {
let duplexAccessed = false;
const hasContentType = new Request(platform.origin, {
body: new ReadableStream(),
method: 'POST',
get duplex() {
duplexAccessed = true;
return 'half';
},
}).headers.has('Content-Type');
return duplexAccessed && !hasContentType;
});
What's wrong with this picture? Trick question, there's nothing fundamentally wrong in my mind with this JavaScript. It's an example of feature detection, where stream request capability (as of this writing supported by Chrome, Edge, and Opera, and Node LTS and on) is supported by the current runtime. The method is actually quite creative, and twofold. First, pass an instance of ReadableStream
as the request body, and check that the generated request does not have a content-type, which tells us we're not in FireFox for example which will return a content-type of "text/plain"
.
There is a problem with this in node environments however, in that older versions of Node will also return an object without a content type header, even though they don't implement streaming requests. So the final bit is to add a getter for the duplex property, and see if its actually read by the runtime's Request
implementation. Brilliant! JavaScript allows us to pass objects with unused properties, so it won't tell us if anyone cares about them or not. This does, very cool!
While JavaScript allows us to pass these unused properties, TypeScript does not. Since the duplex property is not completely supported across major browsers, its not included in the RequestInit
type that's expected as the second argument to the Request
constructor. So now that we've established its all good, how do we tell TypeScript not to worry. Claude AI comes up with what I think is a wrong answer given the context. Basically, let's create our own type that isn't in the standard library, and tell TypeScript its that instead. Something like this:
interface ExtendedRequestInit extends RequestInit {
duplex?: string;
}
// Then use it:
new Request(platform.origin, {
// ...
} as ExtendedRequestInit);
Why is this a bad idea? Well someday that type will no longer be needed, as the duplex property and functionality becomes more widely supported. If that happens, and the standard library is updated, this band-aid will still be there, and become unneeded. A better solution to this problem is to use the @ts-expect-error
directive, which tells TypeScript "I know this is wrong, trust me". And if one day it becomes correct, TypeScript will return the favor and say "Actually, you're wrong, it's right." When it becomes right we remove the directive, and all is well!
const supportsRequestStream = isReadableStreamSupported && test(() => {
let duplexAccessed = false;
const hasContentType = new Request(platform.origin, {
body: new ReadableStream(),
method: 'POST',
// @ts-expect-error duplex is an experimental property
get duplex() {
duplexAccessed = true;
return 'half';
},
}).headers.has('Content-Type');
return duplexAccessed && !hasContentType;
});
Directives are a feature and they're useful, @ts-ignore
over any
as the former is documenting, @ts-expect-error
is even better as it will tell you if its fixed. My personal opinion is that any
is not a type its a type-hole, its not a feature of TypeScript its a compiler error, don't use it.
TS2367: This comparison appears to be unintentional
Error: This comparison appears to be unintentional because the types have no overlap.
JS File: lib/adapters/fetch.js:172
TS File: lib/adapters/fetch.ts:189
const isStreamResponse = supportsResponseStream && (responseType === 'stream' || responseType === 'response');
Take a look at this one, and thinking back to what we know about ResponseType
, what might be the issue? If you said 'response'
isn't a valid ResponseType
, you'd be right! We know this because it's in the type definitions that exist already in Axios. I also know this because a search of the codebase reveals that the string doesn't appear in any other context in relation to a ResponseType
.
This line existed in the original PR, and was actually modified in a second pr to fix a bug, but in both cases this comparison appears. Maybe it was an addition for an idea that was never completed or wasn't needed by the end of the PR, but either way TypeScript tells us its probably a mistake. Tell us Claude, how should we fix this? What's that, create a new CustomResponseType
to include 'response'?
type CustomResponseType = ResponseType | 'response';
This is a solution, but it completely misses the reality of this kind of error. Its pointing to code that should be removed, not simply a compiler error to be silenced. We sometimes get into this habit ourselves as developers, especially when we're the second developer to touch a piece of code. It can be scary to delete from the codebase. Isn't it "safer" to put a bandaid on the error in TypeLand®️, rather than risk it all in the real world?
This is where TypeScript gives us an edge, because a) it would have prevented the problem in the first place. That's it actually, there is no b. And, when things do come up, TypeScript and properly typed code gives us the confidence to refactor without fear of breaking the contracts that the types define.
Up Next!
Stay tuned for my next post in this series, where I'll break down a second file in the Axios codebase!