Mocking API Requests in Unit Tests
In many applications, it's common to send requests to external services to acquire various types of data. To ensure our code handles these responses properly, testing is essential. However, in a test environment, making actual calls to these external services is something we'd typically want to avoid. PHPUnit offers several ways to prevent this. In this blog post, I'll demonstrate how you can mock HTTP requests in your unit tests when you're using the GuzzleHttp client. Scenario Overview Let's consider a straightforward "geocoder" service that provides the coordinates of a location based on a given address. This geocoder leverages the GuzzleHttp client to communicate with an external service, Google Maps in this case, to retrieve the desired coordinates. Here's how the code is structured:
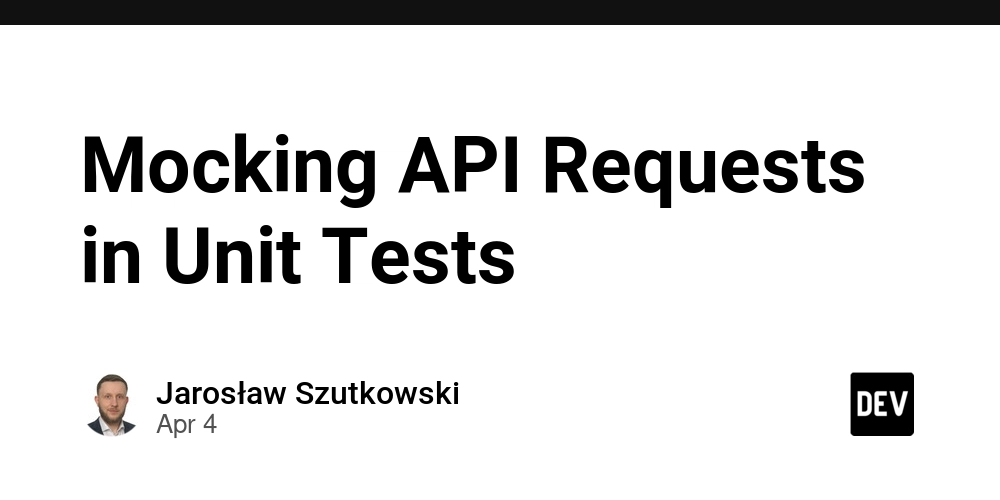
In many applications, it's common to send requests to external services to acquire various types of data. To ensure our code handles these responses properly, testing is essential. However, in a test environment, making actual calls to these external services is something we'd typically want to avoid. PHPUnit offers several ways to prevent this. In this blog post, I'll demonstrate how you can mock HTTP requests in your unit tests when you're using the GuzzleHttp client.
Scenario Overview
Let's consider a straightforward "geocoder" service that provides the coordinates of a location based on a given address. This geocoder leverages the GuzzleHttp client to communicate with an external service, Google Maps in this case, to retrieve the desired coordinates. Here's how the code is structured:
declare(strict_types=1);
namespace App\Infrastructure\Geocoder;
use App\Domain\GeocoderInterface;
use App\Domain\ValueObject\Address;
use App\Domain\ValueObject\Coordinates;
use GuzzleHttp\Client;
final readonly class GoogleMapsGeocoder implements GeocoderInterface
{
private const string ENDPOINT = 'https://maps.googleapis.com/maps/api/geocode/json';
public function __construct(
private Client $client,
private string $apiKey
) {}
public function geocode(Address $address): ?Coordinates
{
$params = [
'query' => [
'address' => $address->street,
'components' => \sprintf(
'country:%s|locality:%s|postal_code:%s',
$address->countryCode,
$address->city,
$address->postcode
),
'key' => $this->apiKey,
],
];
$response = $this->client->get(self::ENDPOINT, $params);
$data = \json_decode($response->getBody()->getContents(), true, 512, JSON_THROW_ON_ERROR);
if ($data['results'] === []) {
return null;
}
$firstResult = $data['results'][0];
if ($firstResult['geometry']['location_type'] !== 'ROOFTOP') {
return null;
}
return new Coordinates(
$firstResult['geometry']['location']['lat'],
$firstResult['geometry']['location']['lng']
);
}
}
Testing
To test different scenarios, such as whether the response is correctly converted to a Coordinates object or if the response is empty, we need to mock the HTTP request. The simplest way to do this is by using the MockHandler
class from GuzzleHttp
. It lets us create a mock client that returns a predefined response. Here's how you can do it:
use GuzzleHttp\Client;
use GuzzleHttp\Handler\MockHandler;
$this->handler = new MockHandler();
new Client(['handler' => new MockHandler()]);
Then we can add a response to the handler:
$this->handler->append(
new Response(200, [], \json_encode([
'results' => [
[
'geometry' => [
'location' => [
'lat' => '1.0',
'lng' => '2.0',
],
'location_type' => 'ROOFTOP',
],
],
],
]))
);
After we call external service, we can also check whether the request was made with the correct parameters by using getLastRequest()
method:
$this->handler->getLastRequest();
Finally, the whole unit test can look like this:
declare(strict_types=1);
namespace App\Tests\Infrastructure\Geocoder;
use App\Domain\ValueObject\Address;
use App\Infrastructure\Geocoder\GoogleMapsGeocoder;
use GuzzleHttp\Client;
use GuzzleHttp\Handler\MockHandler;
use GuzzleHttp\Psr7\Response;
use PHPUnit\Framework\Attributes\Test;
use PHPUnit\Framework\TestCase;
use Psr\Http\Message\RequestInterface;
final class GoogleMapsGeocoderUnitTest extends TestCase
{
private const string API_KEY = 'api-key';
private readonly MockHandler $handler;
private readonly GoogleMapsGeocoder $fixture;
protected function setUp(): void
{
parent::setUp();
$this->handler = new MockHandler();
$client = new Client(['handler' => $this->handler]);
$this->fixture = new GoogleMapsGeocoder(
$client,
self::API_KEY,
);
}
#[Test]
public function it_geocodes_address(): void
{
$address = new Address(
countryCode: 'UK',
city: 'London',
street: 'Buckingham Palace',
postcode: 'SW1A 1AA',
);
$expectedLat = '51.5073509';
$expectedLng = '-0.1277583';
$responseData = [
'results' => [
[
'geometry' => [
'location' => [
'lat' => $expectedLat,
'lng' => $expectedLng,
],
'location_type' => 'ROOFTOP',
],
],
],
];
$this->handler->append(new Response(200, [], (string) \json_encode($responseData, JSON_THROW_ON_ERROR)));
$coordinates = $this->fixture->geocode($address);
$this->assertNotNull($coordinates);
$this->assertSame($expectedLat, $coordinates->lat);
$this->assertSame($expectedLng, $coordinates->lng);
$lastRequest = $this->handler->getLastRequest();
$this->assertNotNull($lastRequest);
$this->assertRequest($lastRequest, $address);
}
private function assertRequest(RequestInterface $request, Address $address): void
{
$queryArray = [];
\parse_str(
$request->getUri()->getQuery(),
$queryArray
);
$this->assertSame(self::API_KEY, $queryArray['key']);
$this->assertSame($address->street, $queryArray['address']);
$this->assertSame(
\sprintf(
'country:%s|locality:%s|postal_code:%s',
$address->countryCode,
$address->city,
$address->postcode,
),
$queryArray['components']
);
}
}
Conclusion
In this blog post, I demonstrated how to mock HTTP requests in unit tests using the GuzzleHttp client. By using the MockHandler
class, we can create a mock client that returns predefined responses, allowing us to test different scenarios without making actual calls to external services. This approach is essential for ensuring our code handles responses correctly and efficiently.