Mastering the Odoo Python API as an Odoo Python Developer
Why Mastering the Odoo Python API Matters As an Odoo Python developer you pull sales data from an Odoo server or build a custom feature for a client using the Odoo Model API as your magic wand. This API lets you connect Python to Odoo, fetch data, automate processes and create tailored solutions all without touching the core code. Whether you automate reports or integrate Odoo with another application, mastering the API will make you more efficient and more valuable as a developer. In this guide I’ll show you how to do it step by step, and unlock endless possibilities for custom Odoo solutions. Skills, Setup, and Practical Use of the API Build the Essential Skills for an Odoo Python Developer Start by mastering the basics. Get good at Python, focus on loops, functions and dictionaries as Odoo is built on top of Python. Learn Odoo’s Object Relational Mapping (ORM) to manage data flow between Python and Odoo’s database. Learn XML, which shapes Odoo views and API interactions. Get to know PostgreSQL, the database engine for Odoo. By combining these skills you can handle the API and create custom. Configure Your Environment for the Odoo RPC API Let's dive into the practical setup. Begin by installing Odoo locally or accessing a server such as Odoo.sh or a client’s instance. Next, set up Python version 3.7 or higher is recommended. Use pip to install the xmlrpc.client library, which facilitates connection to Odoo’s API. Here’s a quick script to authenticate: python import xmlrpc.client url = "http://your-odoo-server.com" db = "your_database" username = "your_username" password = "your_password" common = xmlrpc.client.ServerProxy(f"{url}/xmlrpc/2/common") uid = common.authenticate(db, username, password, {}) Execute this script after replacing the placeholders with your server details to connect to Odoo’s data and features. Decide Between XMLRPC and JSON RPC for Your Project Odoo provides two API options: XMLRPC and JSON RPC. XMLRPC is the older, reliable choice, compatible with all Odoo versions, making it ideal for legacy systems. JSON RPC is newer and faster, perfect for lightweight tasks like mobile apps. Choose XMLRPC for broad compatibility or JSON RPC for speed in modern projects. Both are effective; your selection should depend on your specific needs. Create Tailored Solutions with the Odoo External API Now, let's build something practical. If you want to retrieve all sales orders, after authenticating (as described in the setup), add this: python models = xmlrpc.client.ServerProxy(f"{url}/xmlrpc/2/object") sales = models.execute_kw(db, uid, password, "sale.order", "search_read", [[]], {"fields": ["name", "amount_total"]}) print(sales) This script fetches order names and totals. You can modify it to retrieve customers, products, or any data stored in Odoo. Save the data to a CSV or integrate it with another application this is where customization begins. Adopt Best Practices for Python API Development Ensure your code remains clean and secure. Always handle errors by wrapping API calls in a try-except block to catch connection issues. Avoid hardcoding passwords by using environment variables: python import os password = os.environ.get("ODOO_PASSWORD") Limit API calls by fetching only the necessary fields (as in the example with name and amount_total) to enhance speed. These practices ensure your solutions are both reliable and efficient. Address Common Challenges in Odoo API Customization You may encounter obstacles. If the server is distant (e.g., hosted in Europe while you’re in the U.S.), latency can be an issue. Use Python’s pandas library to cache data locally as a solution. If you lack source code access, stick to the API or mimic browser requests by examining the network tab in your browser. To debug errors, print API responses for quick troubleshooting. Overcoming these challenges keeps your projects on track. Leverage Tools and Libraries to Enhance Your Workflow To work more efficiently, consider libraries like odooly or OdooRPC. They simplify API calls, requiring less code for more results. For instance, OdooRPC connects with a single line: python import odoorpc odoo = odoorpc.ODOO("your-odoo-server.com", port=8069) odoo.login("your_database", "your_username", "your_password") Be cautious, as these tools may lag with new Odoo versions. If issues arise, revert to raw XMLRPC for dependable results. Advance Your Career and Access Resources Progress from API Basics to Odoo Python Expert Mastering the Odoo External API is more than just coding it’s a career enhancer. Start with simple data extractions, then move on to custom Odoo modules or integrations. Businesses need developers who can tailor Odoo for specific industries like retail or manufacturing. The more you create, the more you distinguish yourself. Eventually, you could lead projects or consult, earning mo
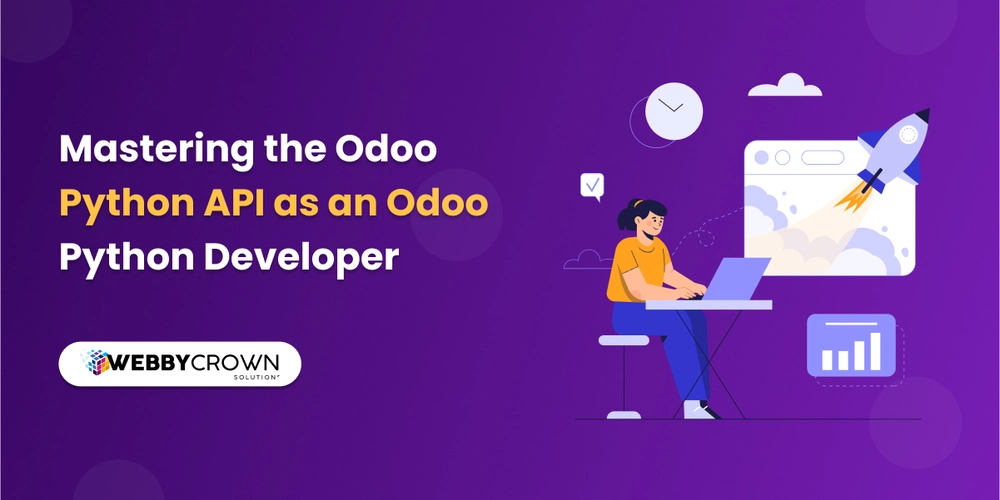
Why Mastering the Odoo Python API Matters
As an Odoo Python developer you pull sales data from an Odoo server or build a custom feature for a client using the Odoo Model API as your magic wand. This API lets you connect Python to Odoo, fetch data, automate processes and create tailored solutions all without touching the core code. Whether you automate reports or integrate Odoo with another application, mastering the API will make you more efficient and more valuable as a developer. In this guide I’ll show you how to do it step by step, and unlock endless possibilities for custom Odoo solutions.
Skills, Setup, and Practical Use of the API
Build the Essential Skills for an Odoo Python Developer
Start by mastering the basics. Get good at Python, focus on loops, functions and dictionaries as Odoo is built on top of Python. Learn Odoo’s Object Relational Mapping (ORM) to manage data flow between Python and Odoo’s database. Learn XML, which shapes Odoo views and API interactions. Get to know PostgreSQL, the database engine for Odoo. By combining these skills you can handle the API and create custom.
Configure Your Environment for the Odoo RPC API
Let's dive into the practical setup. Begin by installing Odoo locally or accessing a server such as Odoo.sh or a client’s instance. Next, set up Python version 3.7 or higher is recommended. Use pip to install the xmlrpc.client library, which facilitates connection to Odoo’s API. Here’s a quick script to authenticate:
python
import xmlrpc.client
url = "http://your-odoo-server.com"
db = "your_database"
username = "your_username"
password = "your_password"
common = xmlrpc.client.ServerProxy(f"{url}/xmlrpc/2/common")
uid = common.authenticate(db, username, password, {})
Execute this script after replacing the placeholders with your server details to connect to Odoo’s data and features.
Decide Between XMLRPC and JSON RPC for Your Project
Odoo provides two API options: XMLRPC and JSON RPC. XMLRPC is the older, reliable choice, compatible with all Odoo versions, making it ideal for legacy systems. JSON RPC is newer and faster, perfect for lightweight tasks like mobile apps. Choose XMLRPC for broad compatibility or JSON RPC for speed in modern projects. Both are effective; your selection should depend on your specific needs.
Create Tailored Solutions with the Odoo External API
Now, let's build something practical. If you want to retrieve all sales orders, after authenticating (as described in the setup), add this:
python
models = xmlrpc.client.ServerProxy(f"{url}/xmlrpc/2/object")
sales = models.execute_kw(db, uid, password, "sale.order", "search_read", [[]], {"fields": ["name", "amount_total"]})
print(sales)
This script fetches order names and totals. You can modify it to retrieve customers, products, or any data stored in Odoo. Save the data to a CSV or integrate it with another application this is where customization begins.
Adopt Best Practices for Python API Development
Ensure your code remains clean and secure. Always handle errors by wrapping API calls in a try-except block to catch connection issues. Avoid hardcoding passwords by using environment variables:
python
import os
password = os.environ.get("ODOO_PASSWORD")
Limit API calls by fetching only the necessary fields (as in the example with name and amount_total) to enhance speed. These practices ensure your solutions are both reliable and efficient.
Address Common Challenges in Odoo API Customization
You may encounter obstacles. If the server is distant (e.g., hosted in Europe while you’re in the U.S.), latency can be an issue. Use Python’s pandas library to cache data locally as a solution. If you lack source code access, stick to the API or mimic browser requests by examining the network tab in your browser. To debug errors, print API responses for quick troubleshooting. Overcoming these challenges keeps your projects on track.
Leverage Tools and Libraries to Enhance Your Workflow
To work more efficiently, consider libraries like odooly or OdooRPC. They simplify API calls, requiring less code for more results. For instance, OdooRPC connects with a single line:
python
import odoorpc
odoo = odoorpc.ODOO("your-odoo-server.com", port=8069)
odoo.login("your_database", "your_username", "your_password")
Be cautious, as these tools may lag with new Odoo versions. If issues arise, revert to raw XMLRPC for dependable results.
Advance Your Career and Access Resources
Progress from API Basics to Odoo Python Expert
Mastering the Odoo External API is more than just coding it’s a career enhancer. Start with simple data extractions, then move on to custom Odoo modules or integrations. Businesses need developers who can tailor Odoo for specific industries like retail or manufacturing. The more you create, the more you distinguish yourself. Eventually, you could lead projects or consult, earning more and tackling bigger challenges.
ow to Use Python to Extract Data from Odoo via the External API?
You can use Python to pull data from Odoo by connecting to its External API, specifically the RPC (Remote Procedure Call) interface. First, install Python on your system and import the xmlrpc.client library. Then, write a script to connect to your Odoo server using a URL, database name, username, and password. After logging in, call methods like execute_kw to fetch data from Odoo models, such as customers or sales orders. For example, you can grab a list of product names with a few lines of code. Test your script with small queries first, and you’ll see how easy it is to pull data directly into Python!
Top Python Libraries for Efficiently Using Odoo’s API
Several Python libraries make working with Odoo’s API simple and efficient. The xmlrpc. client library, built into Python, tops the list because it handles XML RPC calls to Odoo effortlessly. If you prefer JSON RPC, the requests library works great for sending HTTP requests to Odoo’s JSON endpoints. For managing data, pandas helps you organize and analyze the information you pull. Lastly, odoo client (a third party library) simplifies API calls with pre built functions. Pick xmlrpc.client for basic tasks or explore API requests and pandas for more advanced projects management!
Should You Use XML RPC or JSON RPC for Odoo API Integration?
Choosing between XML RPC and JSON RPC for Odoo API integration depends on your business needs. XML RPC works well because Python includes xmlrpc. client by default, making it quick to set up and reliable for most tasks, like fetching records or updating data. JSON RPC, on the other hand, shines if you need faster, lightweight communication or plan to integrate with web apps, since it uses JSON, a modern data format. Go with XML RPC for simplicity and stability, but pick JSON RPC if speed and flexibility matter more to you!
How to Schedule Automated Data Pulls from Odoo Using Python?
You can schedule automated data pulls from Odoo by combining Python with a scheduling tool. Write a Python script using xmlrpc.client to connect to Odoo and fetch data, like sales or inventory updates. Save the data to a file or database. Then, use a tool like cron (on Linux/Mac) or Task Scheduler (on Windows) to run your script at set times say, every hour or daily. Test your script manually first, then set the schedule. This way, you automate data pulls without lifting a finger!
How to Use Odoo’s API for Real Time Data Analysis with Python?
You can use Odoo’s API for real time data analysis by connecting Python to Odoo and processing data on the fly. Start with a Python script that uses xmlrpc. client to pull live data, like current sales or stock levels, from Odoo models. Next, bring in pandas to clean and structure the data, and use matplotlib or seaborn to create charts for quick insights. Run the script whenever you need fresh numbers, or loop it to update continuously. This setup gives you real time analysis with minimal effort!
Should You Hire an Odoo Developer or Train an In House Python Programmer?
Deciding between hiring an Odoo developer or training a Python programmer depends on your primary goals. Hire an Odoo developer if you need fast results, like custom Odoo modules or complex integrations, since they already know Odoo’s quirks and tools. Train an in house Python programmer if you want long term flexibility and have time to invest someone with Python skills can learn Odoo in a few months with practice. Go for the developer for speed, but train your team if you value control and cost savings!
How Does Odoo’s Framework Differ from Standard Python Development?
Odoo’s framework differs from standard Python development in a few key ways. In regular Python, you build apps from scratch, controlling everything. Odoo, however, gives you a ready made structure with models, views, and controllers for business apps, like ERP systems. You write Python code to customize Odoo’s objects, using its ORM (Object Relational Mapping) to handle databases instead of raw SQL. Plus, Odoo mixes in XML and JavaScript for interfaces, unlike pure Python projects. Think of Odoo as a Lego set you build within its rules, not from the ground up!
Is General Python Programming Sufficient, or Do I Need Odoo Specific Experience?
You don’t need Odoo specific Programming experience to start, but general Python programming concepts alone isn’t quite enough. Python skills let you write scripts and understand Odoo’s code, like loops and functions. However, Odoo adds its own layers think ORM, RPC APIs, and QWeb templates that you’ll need to learn. If you know Python, you can pick up Odoo faster, but expect to spend time figuring out its unique tools. Start with Python, then dive into Odoo basics to bridge the gap!
How Quickly Can You Learn Odoo Development with a Python Background?
With a Python strong background, you can learn Odoo development in about 3 to 6 months, depending on your pace. Spend a few weeks mastering Odoo’s basics like models, views, and APIs since you already know Python syntax. Practice building small modules (like a custom report) in a month or two. Tackle advanced topics, like security or performance, in the next couple of months. Study Odoo docs, watch tutorials, and code daily, and you’ll be comfortable in half a year or less!
Key Skills Required for Odoo Development
You need a mix of skills to excel in Odoo Custom development. First, master Python programming for writing logic and scripts. Learn Odoo’s ORM to manage data models easily. Understand XML and QWeb to design views and reports. Get comfortable with RPC APIs (XML RPC or JSON RPC) for integrations. Pick up basic PostgreSQL knowledge to tweak databases. Add some JavaScript skills for front end tweaks. Finally, practice problem solving and debugging to handle real world projects. Start with Python and build these skills step by step!
Share Your Thoughts and Try It Out
Ready to dive in? Copy the sales order script above, swap in your server details, and run it. See what data you pull! Got a challenge like slow connections or tricky data needs? Drop a comment below. I’d love to hear how you’re using the Odoo Python API and help with any roadblocks. Let’s build custom solutions together!