Mastering React Forms with a State Object: My Personal Experience
As a frontend developer constantly learning and building, one of my recent tasks was to create a form in React where multiple input fields were all tied to a single state object. Simple in theory, but I discovered a few powerful patterns that made the process clean, scalable, and easy to manage — and I’d love to share my experience here. The Challenge I needed to build a “Create New Post” form with fields like title, date, time, content, and even an image. Rather than manage each input with its own state, I decided to keep things tidy by using one useState hook with an object like this: const [newPost, setNewPost] = useState({ title: "", date: "", time: "", content: "", image: null }); But then came the question: How do I update individual fields in this object when users type into the form? The Pattern That Changed Everything Instead of writing separate functions for each input, I used an inline onChange handler that looks like this: onChange={(e) => setNewPost({ ...newPost, [e.target.name]: e.target.value }) } At first glance, it felt a little complex — but once I understood it, it became second nature. Here’s the breakdown: e.target.name is the name of the input (like "title"). e.target.value is the value typed in. [e.target.name]: e.target.value dynamically updates the correct field in the newPost object. The spread operator ...newPost ensures all other values in the object stay unchanged. This pattern made the form feel way more dynamic and scalable. I could add more fields without needing to touch the onChange logic again. And that’s exactly the kind of flexibility I love as a developer. Bonus: File Uploads The only difference was with the image input. File inputs use e.target.files[0] instead of e.target.value, so I updated that one like this: onChange={(e) => setNewPost({ ...newPost, image: e.target.files[0] }) } My Key Takeaway Learning this pattern helped me write cleaner React forms and gave me a better grasp of how state management really works under the hood. It’s one of those small wins that makes a big difference in bigger projects. If you're just getting started with React forms, I highly recommend this method. It keeps things neat, avoids repetition, and scales beautifully as your form grows.
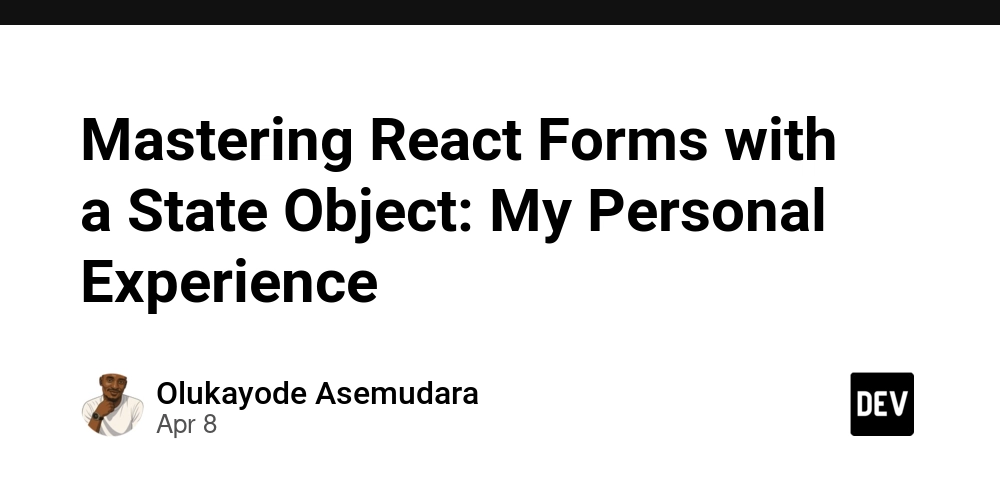
As a frontend developer constantly learning and building, one of my recent tasks was to create a form in React where multiple input fields were all tied to a single state object. Simple in theory, but I discovered a few powerful patterns that made the process clean, scalable, and easy to manage — and I’d love to share my experience here.
The Challenge
I needed to build a “Create New Post” form with fields like title, date, time, content, and even an image. Rather than manage each input with its own state, I decided to keep things tidy by using one useState
hook with an object like this:
const [newPost, setNewPost] = useState({
title: "",
date: "",
time: "",
content: "",
image: null
});
But then came the question: How do I update individual fields in this object when users type into the form?
The Pattern That Changed Everything
Instead of writing separate functions for each input, I used an inline onChange
handler that looks like this:
onChange={(e) =>
setNewPost({ ...newPost, [e.target.name]: e.target.value })
}
At first glance, it felt a little complex — but once I understood it, it became second nature.
Here’s the breakdown:
-
e.target.name
is the name of the input (like "title"). -
e.target.value
is the value typed in. -
[e.target.name]: e.target.value
dynamically updates the correct field in thenewPost
object. - The spread operator
...newPost
ensures all other values in the object stay unchanged.
This pattern made the form feel way more dynamic and scalable. I could add more fields without needing to touch the onChange
logic again. And that’s exactly the kind of flexibility I love as a developer.
Bonus: File Uploads
The only difference was with the image input. File inputs use e.target.files[0]
instead of e.target.value
, so I updated that one like this:
onChange={(e) =>
setNewPost({ ...newPost, image: e.target.files[0] })
}
My Key Takeaway
Learning this pattern helped me write cleaner React forms and gave me a better grasp of how state management really works under the hood. It’s one of those small wins that makes a big difference in bigger projects.
If you're just getting started with React forms, I highly recommend this method. It keeps things neat, avoids repetition, and scales beautifully as your form grows.