Mastering LinkedIn API: Step-by-Step Guide for Seamless Integration
LinkedIn offers a powerful API that allows developers to automate posting and retrieve user information. In this guide, we will walk you through the process of integrating with LinkedIn’s API, covering GET requests, posting text, uploading images, and posting videos. Step 1: Setting Up LinkedIn API Access To begin, follow these steps to set up your LinkedIn API access: Ensure you have a LinkedIn account – If you don’t have one, sign up at LinkedIn. Create a Company Page – You need a company page to post on behalf of an organization. Register an App – Go to LinkedIn Developer Portal and create an app. Use Your Page Name – When creating an app, use the name of your LinkedIn Page. Enable Products – Under the Products tab, add: Share on LinkedIn Sign In with LinkedIn using OpenID Connect Verify LinkedIn Page – In the Settings tab, verify your LinkedIn page to allow posting access. Generate Access Token – Navigate to the Auth tab and use the OAuth 2.0 tools on the right side to generate an access token. Get User Asset ID using Postman – Once you obtain the access token, use Postman to make API requests and retrieve the asset ID. Step 2: Making a GET Request to Retrieve User Info To fetch user details, use the following Python script: Loads environment variables to securely store your LinkedIn Access Token. Makes a GET request to LinkedIn’s API to fetch user details. If successful, prints user information; otherwise, displays an error. import requests import os from dotenv import load_dotenv # Load environment variables load_dotenv() ACCESS_TOKEN = os.getenv("LINKEDIN_ACCESS_TOKEN") headers = { "Authorization": f"Bearer {ACCESS_TOKEN}" } response = requests.get("https://api.linkedin.com/v2/userinfo", headers=headers) if response.status_code == 200: print("✅ User Info:", response.json()) else: print(f"❌ Error: {response.status_code}, {response.text}") Step 3: Posting Text to LinkedIn Once you have your User URN, you can post a text update: Defines the API endpoint for posting text. Constructs the payload including the text message and visibility settings. Sends a POST request with the payload to LinkedIn. Handles success or failure responses. post_url = "https://api.linkedin.com/v2/ugcPosts" data = { "author": "urn:li:person:YOUR_USER_URN", "lifecycleState": "PUBLISHED", "specificContent": { "com.linkedin.ugc.ShareContent": { "shareCommentary": { "text": "
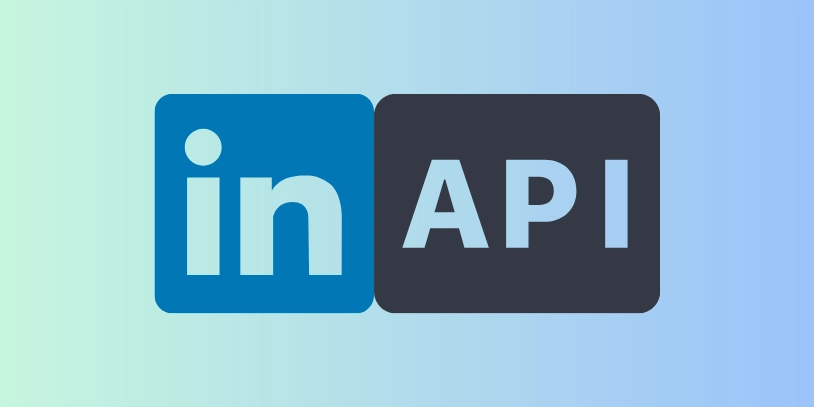
LinkedIn offers a powerful API that allows developers to automate posting and retrieve user information. In this guide, we will walk you through the process of integrating with LinkedIn’s API, covering GET requests, posting text, uploading images, and posting videos.
Step 1: Setting Up LinkedIn API Access
To begin, follow these steps to set up your LinkedIn API access:
- Ensure you have a LinkedIn account – If you don’t have one, sign up at LinkedIn.
- Create a Company Page – You need a company page to post on behalf of an organization.
- Register an App – Go to LinkedIn Developer Portal and create an app.
- Use Your Page Name – When creating an app, use the name of your LinkedIn Page.
-
Enable Products – Under the Products tab, add:
- Share on LinkedIn
- Sign In with LinkedIn using OpenID Connect
- Verify LinkedIn Page – In the Settings tab, verify your LinkedIn page to allow posting access.
- Generate Access Token – Navigate to the Auth tab and use the OAuth 2.0 tools on the right side to generate an access token.
- Get User Asset ID using Postman – Once you obtain the access token, use Postman to make API requests and retrieve the asset ID.
Step 2: Making a GET Request to Retrieve User Info
To fetch user details, use the following Python script:
- Loads environment variables to securely store your LinkedIn Access Token.
- Makes a GET request to LinkedIn’s API to fetch user details.
- If successful, prints user information; otherwise, displays an error.
import requests
import os
from dotenv import load_dotenv
# Load environment variables
load_dotenv()
ACCESS_TOKEN = os.getenv("LINKEDIN_ACCESS_TOKEN")
headers = {
"Authorization": f"Bearer {ACCESS_TOKEN}"
}
response = requests.get("https://api.linkedin.com/v2/userinfo", headers=headers)
if response.status_code == 200:
print("✅ User Info:", response.json())
else:
print(f"❌ Error: {response.status_code}, {response.text}")
Step 3: Posting Text to LinkedIn
Once you have your User URN, you can post a text update:
- Defines the API endpoint for posting text.
- Constructs the payload including the text message and visibility settings.
- Sends a POST request with the payload to LinkedIn.
- Handles success or failure responses.
post_url = "https://api.linkedin.com/v2/ugcPosts"
data = {
"author": "urn:li:person:YOUR_USER_URN",
"lifecycleState": "PUBLISHED",
"specificContent": {
"com.linkedin.ugc.ShareContent": {
"shareCommentary": {
"text": "