Mastering Goroutines, Concurrency, and Mutexes in Go
Introduction Concurrency is one of the most powerful features of the Go programming language. Go provides first-class support for concurrent programming through goroutines and channels, making it an ideal choice for building scalable applications. However, with great power comes great responsibility, and handling shared resources safely requires mechanisms like mutexes. This article will provide a deep dive into goroutines, concurrency, and mutexes in Go, complete with examples and best practices. What Are Goroutines? A goroutine is a lightweight thread of execution. Unlike traditional threads, goroutines have minimal overhead and can be spawned in large numbers without consuming significant resources. Syntax of a Goroutine A goroutine is created by prefixing a function call with the go keyword: Key Characteristics of Goroutines: Goroutines are asynchronous and non-blocking. They use cooperative scheduling instead of preemptive scheduling. They share the same address space, leading to possible race conditions. Understanding Concurrency in Go Concurrency is the ability of a program to execute multiple tasks simultaneously. Go achieves concurrency efficiently using goroutines and channels. Using Channels for Safe Communication A channel is a typed conduit that allows goroutines to communicate safely without explicit locking. Buffered Channels Buffered channels allow multiple values to be stored before they are read: If a buffer is full, a send operation will block until space is available. Select Statement for Multiple Channels The select statement allows a goroutine to wait on multiple communication operations: Handling Race Conditions with Mutexes Since goroutines share memory, simultaneous read and write operations can lead to race conditions. Example of a Race Condition Solving Race Conditions with Mutex A mutex (short for mutual exclusion) ensures that only one goroutine accesses a shared resource at a tim RWMutex: Read-Write Locks If multiple goroutines only read from a shared resource, using a read-write mutex (sync.RWMutex) can improve performance. Best Practices for Concurrency in Go Use Goroutines Wisely: Avoid spawning too many goroutines that consume unnecessary resources. Prefer Channels Over Shared Memory: Channels provide safe communication between goroutines. Use Mutexes for Shared Data: When necessary, sync.Mutex helps prevent race conditions. Utilize sync.WaitGroup: Ensures goroutines complete execution before the main function exits. Use the race Detector: Detects race conditions during testing (go run -race main.go). Conclusion Go’s concurrency model, powered by goroutines and channels, makes writing efficient concurrent programs easier than in many other languages. However, improper handling of shared resources can lead to race conditions, which can be mitigated using sync.Mutex and sync.RWMutex. Mastering these concepts will help you write efficient, safe, and scalable Go applications. Happy coding!
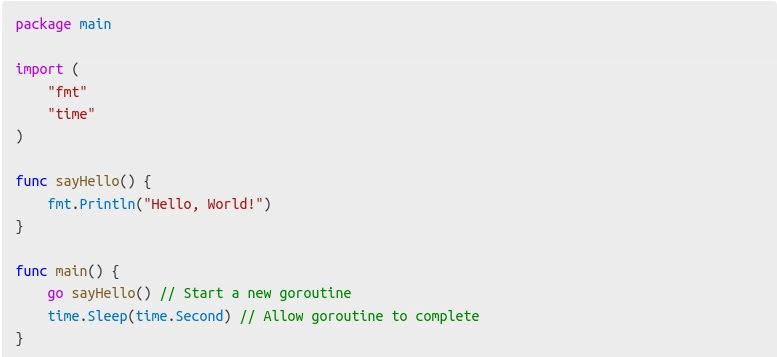
Introduction
Concurrency is one of the most powerful features of the Go programming language. Go provides first-class support for concurrent programming through goroutines and channels, making it an ideal choice for building scalable applications. However, with great power comes great responsibility, and handling shared resources safely requires mechanisms like mutexes.
This article will provide a deep dive into goroutines, concurrency, and mutexes in Go, complete with examples and best practices.
What Are Goroutines?
A goroutine is a lightweight thread of execution. Unlike traditional threads, goroutines have minimal overhead and can be spawned in large numbers without consuming significant resources.
Syntax of a Goroutine
A goroutine is created by prefixing a function call with the go keyword:
Key Characteristics of Goroutines:
- Goroutines are asynchronous and non-blocking.
- They use cooperative scheduling instead of preemptive scheduling.
- They share the same address space, leading to possible race conditions.
Understanding Concurrency in Go
Concurrency is the ability of a program to execute multiple tasks simultaneously. Go achieves concurrency efficiently using goroutines and channels.
Using Channels for Safe Communication
A channel is a typed conduit that allows goroutines to communicate safely without explicit locking.
Buffered Channels
Buffered channels allow multiple values to be stored before they are read:
If a buffer is full, a send operation will block until space is available.
Select Statement for Multiple Channels
The select statement allows a goroutine to wait on multiple communication operations:
Handling Race Conditions with Mutexes
Since goroutines share memory, simultaneous read and write operations can lead to race conditions.
Example of a Race Condition
Solving Race Conditions with Mutex
A mutex (short for mutual exclusion) ensures that only one goroutine accesses a shared resource at a tim
RWMutex: Read-Write Locks
If multiple goroutines only read from a shared resource, using a read-write mutex (sync.RWMutex) can improve performance.
Best Practices for Concurrency in Go
- Use Goroutines Wisely: Avoid spawning too many goroutines that consume unnecessary resources.
- Prefer Channels Over Shared Memory: Channels provide safe communication between goroutines.
- Use Mutexes for Shared Data: When necessary, sync.Mutex helps prevent race conditions.
- Utilize sync.WaitGroup: Ensures goroutines complete execution before the main function exits.
- Use the race Detector: Detects race conditions during testing (go run -race main.go).
Conclusion
Go’s concurrency model, powered by goroutines and channels, makes writing efficient concurrent programs easier than in many other languages. However, improper handling of shared resources can lead to race conditions, which can be mitigated using sync.Mutex and sync.RWMutex.
Mastering these concepts will help you write efficient, safe, and scalable Go applications. Happy coding!