Maintain Code Quality Like a Big Tech Team — Without Paying for Expensive Automation
When I started my career, I was focused on meeting deadlines rather than worrying about code quality. But as I transitioned to a tech lead role, I realized how crucial it was to architect the codebase in a way that was easy for everyone to understand and maintain. By using tools like Husky and Lint-Staged, I was able to automate code checks and enforce clean code practices, which helped keep the codebase scalable and maintainable as the team grew. In this blog, I’ll share how small teams can maintain code quality without expensive solutions. Husky & Lint-Staged: The Perfect Duo Think of Husky and Lint-Staged like Leonardo DiCaprio and Martin Scorsese — when they team up, you get the best out of both. Similarly, Husky and Lint-Staged work together to bring the best discipline to your codebase. They help catch small mistakes early, enforce clean code, and automate formatting right when a developer tries to commit code. This setup ensures that even in fast-moving teams, code quality doesn’t slip through the cracks. Simply install following package in your any Javascript ot Typescript project. npm i lint-staged@12.3.2 Lint-Staged helps run linting (and other scripts) only on the files that are staged for commit. Instead of linting the entire project — which can be slow and unnecessary — it targets only the files you actually changed. This ensures faster linting and that only clean code gets committed, without slowing down your workflow. Next, install Husky: npm i husky Husky allows you to manage Git hooks easily. You can hook into Git events like pre-commit, pre-push, and commit-msg to run commands automatically. In this setup, Husky will trigger Lint-Staged right before a commit, ensuring that only well-formatted, linted files enter the repository. In short: Lint-Staged → Focus on the changed files. Husky → Automate the checks before a commit. npx husky init this will generate husky related configuration side the code base { "name": "my-app", "version": "0.1.0", "private": true, "scripts": { "dev": "next dev --turbopack", "build": "next build", "start": "next start", "lint": "next lint", "lint-staged": "lint-staged", "prepare": "husky" }, "dependencies": { "husky": "^9.1.7", "lint-staged": "^15.2.10", "next": "15.3.1", "react": "^19.0.0", "react-dom": "^19.0.0" }, "devDependencies": { "@eslint/eslintrc": "^3.1.0", "@types/node": "^20.16.11", "@types/react": "^19.0.0-rc.1", "@types/react-dom": "^19.0.0-rc.1", "eslint": "^9.13.0", "eslint-config-next": "15.3.1", "typescript": "^5.6.3" }, "lint-staged": { "*.{js,jsx,ts,tsx}": [ "eslint --fix", "prettier --write" ], "*.{css,md,json}": [ "prettier --write" ] } } to automate the lint stage add npm run lint-staged inside the pre-commit file. then commit your changes Once the isssues were fixed the lnit stage will allow to commit. your code By adding ESLint rules and Test Rules in the lint-staged configuration, we can easily enforce good coding practices and auto-formatting will help escape from unnecessary merge conflicts too.
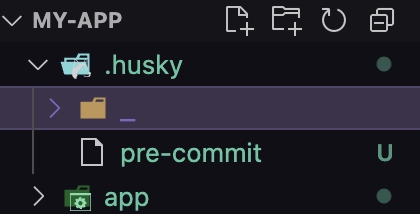
When I started my career, I was focused on meeting deadlines rather than worrying about code quality. But as I transitioned to a tech lead role, I realized how crucial it was to architect the codebase in a way that was easy for everyone to understand and maintain. By using tools like Husky and Lint-Staged, I was able to automate code checks and enforce clean code practices, which helped keep the codebase scalable and maintainable as the team grew.
In this blog, I’ll share how small teams can maintain code quality without expensive solutions.
Husky & Lint-Staged: The Perfect Duo
Think of Husky and Lint-Staged like Leonardo DiCaprio and Martin Scorsese — when they team up, you get the best out of both. Similarly, Husky and Lint-Staged work together to bring the best discipline to your codebase. They help catch small mistakes early, enforce clean code, and automate formatting right when a developer tries to commit code.
This setup ensures that even in fast-moving teams, code quality doesn’t slip through the cracks. Simply install following package in your any Javascript ot Typescript project.
npm i lint-staged@12.3.2
Lint-Staged helps run linting (and other scripts) only on the files that are staged for commit.
Instead of linting the entire project — which can be slow and unnecessary — it targets only the files you actually changed.
This ensures faster linting and that only clean code gets committed, without slowing down your workflow.
Next, install Husky:
npm i husky
Husky allows you to manage Git hooks easily.
You can hook into Git events like pre-commit, pre-push, and commit-msg to run commands automatically.
In this setup, Husky will trigger Lint-Staged right before a commit, ensuring that only well-formatted, linted files enter the repository.
In short:
Lint-Staged → Focus on the changed files.
Husky → Automate the checks before a commit.
npx husky init
this will generate husky related configuration side the code base
{
"name": "my-app",
"version": "0.1.0",
"private": true,
"scripts": {
"dev": "next dev --turbopack",
"build": "next build",
"start": "next start",
"lint": "next lint",
"lint-staged": "lint-staged",
"prepare": "husky"
},
"dependencies": {
"husky": "^9.1.7",
"lint-staged": "^15.2.10",
"next": "15.3.1",
"react": "^19.0.0",
"react-dom": "^19.0.0"
},
"devDependencies": {
"@eslint/eslintrc": "^3.1.0",
"@types/node": "^20.16.11",
"@types/react": "^19.0.0-rc.1",
"@types/react-dom": "^19.0.0-rc.1",
"eslint": "^9.13.0",
"eslint-config-next": "15.3.1",
"typescript": "^5.6.3"
},
"lint-staged": {
"*.{js,jsx,ts,tsx}": [
"eslint --fix",
"prettier --write"
],
"*.{css,md,json}": [
"prettier --write"
]
}
}
to automate the lint stage add npm run lint-staged
inside the pre-commit file. then commit your changes
Once the isssues were fixed the lnit stage will allow to commit. your code
By adding ESLint rules and Test Rules in the lint-staged configuration,
we can easily enforce good coding practices and auto-formatting will help escape from unnecessary merge conflicts too.