Leetcode - 226. Invert Binary Tree
One of the most elegant problems in tree manipulation is inverting a binary tree — essentially flipping it along its vertical axis. It's a simple yet beautiful example of recursion and tree traversal. Let’s dive into how we can solve this in JavaScript, and explore alternate approaches and their complexities. ✅ Recursive Solution (Elegant and Classic) /** * Definition for a binary tree node. * function TreeNode(val, left, right) { * this.val = (val===undefined ? 0 : val) * this.left = (left===undefined ? null : left) * this.right = (right===undefined ? null : right) * } */ /** * @param {TreeNode} root * @return {TreeNode} */ var invertTree = function(root) { if (root === null) return null; // Recursively invert subtrees first const left = invertTree(root.left); const right = invertTree(root.right); // Swap using array destructuring (clean and readable) [root.left, root.right] = [right, left]; return root; };
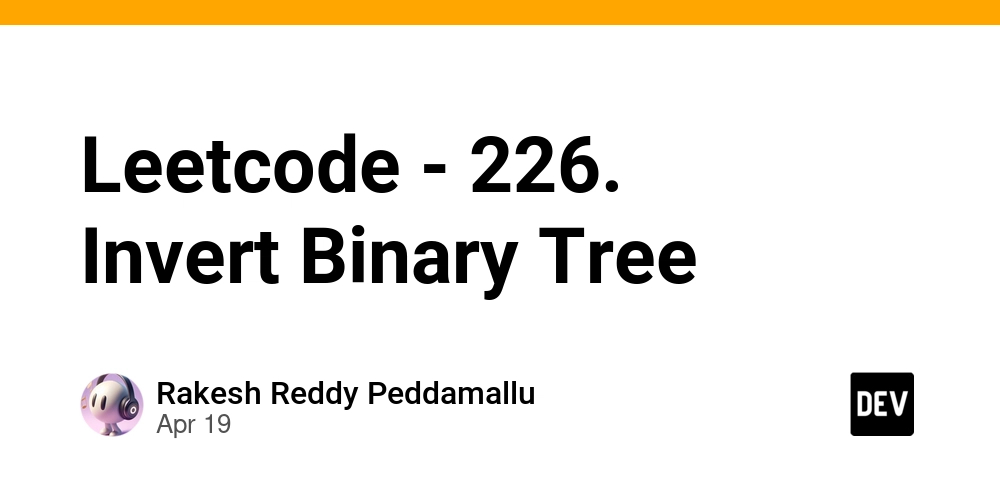
One of the most elegant problems in tree manipulation is inverting a binary tree — essentially flipping it along its vertical axis. It's a simple yet beautiful example of recursion and tree traversal.
Let’s dive into how we can solve this in JavaScript, and explore alternate approaches and their complexities.
✅ Recursive Solution (Elegant and Classic)
/**
* Definition for a binary tree node.
* function TreeNode(val, left, right) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
*/
/**
* @param {TreeNode} root
* @return {TreeNode}
*/
var invertTree = function(root) {
if (root === null) return null;
// Recursively invert subtrees first
const left = invertTree(root.left);
const right = invertTree(root.right);
// Swap using array destructuring (clean and readable)
[root.left, root.right] = [right, left];
return root;
};