day-27: Java Abstraction and Inheritance: Key Concepts, Code Examples, and Interview Insights
*Note: * Always we try to approach the problem logically. Abstract Classes Can have at least one abstract method? True Abstract classes SHOULD have at least one abstract method? False. package educationloanpackage; public abstract class Bank { } Abstract Methods are decleared to have no body, leaving their implementation to sub classes. *Abstraction: * Inheritance, Polymorphism we need to know. package educationloanpackage; public abstract class Parents { public abstract void study(); public void distribute_choco(){ } public void grow() { System.out.println("Take Care"); } public static void main(String[] args) { // TODO Auto-generated method stub } } package educationloanpackage; public class Children extends Parents { @Override // annotation - metadata - data about data public void study() { System.out.println("LLB Laws..."); } // can't decrease the access... private void distribute_choco(){ } public static void main(){ Children ch = new Children(); // Inheritance ch.study(); // abstract and run time polymorphism ch.distribute_choco(); ch.grow(); } } public abstract class Child2 { // child class also abstract public static void main(String[] args) { // TODO Auto-generated method stub } } Super --> Superior (or) Parent - Super - base Subordinate --> Child - Sub - Derived We can't change the parent class method's access modifier. But can increase the access modifier Like private to public... Abstract methods -->> Access Maintain --> Increase What is Inheritance? Child Object Behaves as Parent Object. Parent child Loosely coupled Relationship Interview Questions: 1). Abstract class should have at least one abstract method - False 2). Abstract Classes can be instantiated --> False 3). Abstract Classes can be have normal --> True 4). Final Classes can't be inherited --> True 5). Final Classes can't be used in Inheritance - True (It will be child class). Final classes can't have sub-Classes. 6). Abstract classes should sub classes --> True 7). Can we have abstract final class --> False 8). We cannot create objects for abstract classes - True 9). Object is very closely related with constructors --> True 10). Can we have constructor is abstract class? - True 11). When constructors will be called? When object is created. Abstract class won't have object we can call the parent's class constructor using child class object. Child Object = Parent Object public abstract class Parents{ Public Parents{ System.out.println("this is parent constract"); } } public class Child extends Parents{ public static void main(){ Child ch = new Child(); // 11th question.. } } *Interface: * Abstract Class = 0 to 100% Abstraction Interface is 100% Abstraction. As you've already learned, objects define their interaction with the outside world through the methods that they expose. Methods form the object's interface with the outside world; the buttons on the front of your television set, for example, are the interface between you and the electrical wiring on the other side of its plastic casing. You press the "power" button to turn the television on and off. Button, Plateform... In its most common form, an interface is a group of related methods with empty bodies. Ref for Interface: https://docs.oracle.com/javase/tutorial/java/concepts/interface.html
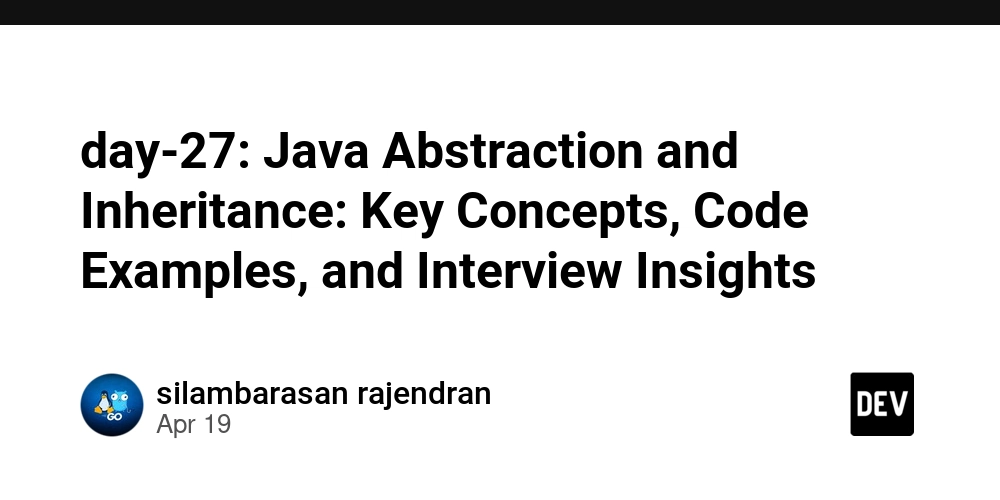
*Note: *
Always we try to approach the problem logically.
Abstract Classes Can have at least one abstract method? True
Abstract classes SHOULD have at least one abstract method? False.
package educationloanpackage;
public abstract class Bank {
}
Abstract Methods are decleared to have no body, leaving their implementation to sub classes.
*Abstraction: *
Inheritance, Polymorphism we need to know.
package educationloanpackage;
public abstract class Parents {
public abstract void study();
public void distribute_choco(){
}
public void grow() {
System.out.println("Take Care");
}
public static void main(String[] args) {
// TODO Auto-generated method stub
}
}
package educationloanpackage;
public class Children extends Parents {
@Override // annotation - metadata - data about data
public void study() {
System.out.println("LLB Laws...");
}
// can't decrease the access...
private void distribute_choco(){
}
public static void main(){
Children ch = new Children(); // Inheritance
ch.study(); // abstract and run time polymorphism
ch.distribute_choco();
ch.grow();
}
}
public abstract class Child2 {
// child class also abstract
public static void main(String[] args) {
// TODO Auto-generated method stub
}
}
Super --> Superior (or) Parent - Super - base
Subordinate --> Child - Sub - Derived
We can't change the parent class method's access modifier. But can increase the access modifier
Like private to public...
Abstract methods -->> Access Maintain --> Increase
What is Inheritance?
Child Object Behaves as Parent Object.
Parent child Loosely coupled Relationship
Interview Questions:
1). Abstract class should have at least one abstract method - False
2). Abstract Classes can be instantiated --> False
3). Abstract Classes can be have normal --> True
4). Final Classes can't be inherited --> True
5). Final Classes can't be used in Inheritance - True (It will be child class).
Final classes can't have sub-Classes.
6). Abstract classes should sub classes --> True
7). Can we have abstract final class --> False
8). We cannot create objects for abstract classes - True
9). Object is very closely related with constructors --> True
10). Can we have constructor is abstract class? - True
11). When constructors will be called?
When object is created.
Abstract class won't have object we can call the parent's class constructor using child class object.
Child Object = Parent Object
public abstract class Parents{
Public Parents{
System.out.println("this is parent constract");
}
}
public class Child extends Parents{
public static void main(){
Child ch = new Child(); // 11th question..
}
}
*Interface: *
Abstract Class = 0 to 100% Abstraction
Interface is 100% Abstraction.
As you've already learned, objects define their interaction with the outside world through the methods that they expose. Methods form the object's interface with the outside world; the buttons on the front of your television set, for example, are the interface between you and the electrical wiring on the other side of its plastic casing. You press the "power" button to turn the television on and off.
Button, Plateform...
In its most common form, an interface is a group of related methods with empty bodies.
Ref for Interface: https://docs.oracle.com/javase/tutorial/java/concepts/interface.html