Learning Operating System Development from Scratch
Developing an operating system (OS) from scratch is one of the most challenging yet rewarding projects for aspiring software developers and computer scientists. It provides deep insights into how computers work, the role of software in hardware management, and the intricacies of system programming. In this post, we’ll explore the fundamental concepts of OS development and provide a roadmap to get started. What is an Operating System? An operating system is software that acts as an intermediary between computer hardware and the user applications. It manages hardware resources, provides a user interface, and enables multitasking and process management. Core Functions of an OS Process Management: Handling the execution of processes and multitasking. Memory Management: Allocating and managing memory for processes. File System Management: Organizing and managing data storage. Device Management: Controlling hardware devices and managing input/output operations. User Interface: Providing user interactions through command-line or graphical interfaces. Prerequisites for OS Development Programming Skills: Strong knowledge of C/C++ and assembly language. Computer Architecture: Understanding how hardware components interact with software. Data Structures & Algorithms: Familiarity with common data structures and algorithms used in OS design. System Programming: Knowledge of system calls and low-level programming. Development Environment Setup Choose a Programming Language: Primarily C or C++ for the OS kernel. Set Up a Cross-Compiler: Use a cross-compiler to generate binaries for your target architecture. Create a Bootable Image: Learn to create bootable images to run on emulators or real hardware. Use Emulators: QEMU or Bochs for testing your OS without needing physical hardware. Basic Structure of an Operating System #include include void kernel_main() { // This is where your kernel code will begin executing. const char *str = "Hello, Operating System!"; // Function to output to the screen (implement your own). print_string(str); } void print_string(const char *str) { while (*str) { // Output each character to the screen // Implementation dependent on the hardware. } } Learning Resources Books: "Operating Systems: Three Easy Pieces" by Remzi H. Arpaci-Dusseau, "Modern Operating Systems" by Andrew S. Tanenbaum. Online Courses: Look for OS development courses on platforms like Coursera or edX. Open Source Projects: Study existing open-source OS projects like Linux, MINIX, or FreeRTOS. Communities: Engage in forums like Stack Overflow, Reddit, or specialized OS development communities. Challenges in OS Development Debugging low-level code can be complex and time-consuming. Resource management and concurrency can introduce unexpected behavior. Compatibility with various hardware can be a significant hurdle. Performance optimization is crucial for a functional OS. Conclusion Building an operating system from scratch is a daunting task that requires patience, dedication, and a thirst for knowledge. However, the skills you develop and the understanding you gain will be invaluable in your programming career. Start with small projects, gradually expand your scope, and enjoy the journey into the world of OS development!
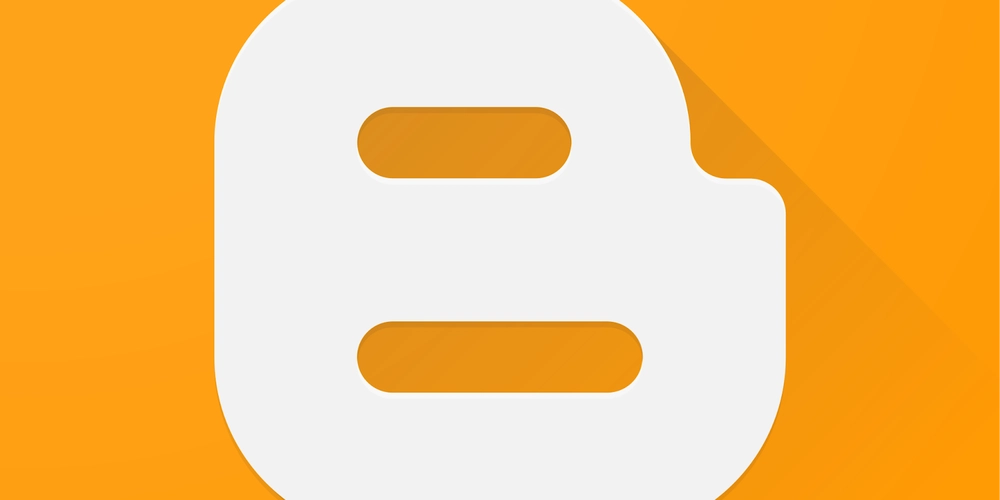
Developing an operating system (OS) from scratch is one of the most challenging yet rewarding projects for aspiring software developers and computer scientists. It provides deep insights into how computers work, the role of software in hardware management, and the intricacies of system programming. In this post, we’ll explore the fundamental concepts of OS development and provide a roadmap to get started.
What is an Operating System?
An operating system is software that acts as an intermediary between computer hardware and the user applications. It manages hardware resources, provides a user interface, and enables multitasking and process management.
Core Functions of an OS
- Process Management: Handling the execution of processes and multitasking.
- Memory Management: Allocating and managing memory for processes.
- File System Management: Organizing and managing data storage.
- Device Management: Controlling hardware devices and managing input/output operations.
- User Interface: Providing user interactions through command-line or graphical interfaces.
Prerequisites for OS Development
- Programming Skills: Strong knowledge of C/C++ and assembly language.
- Computer Architecture: Understanding how hardware components interact with software.
- Data Structures & Algorithms: Familiarity with common data structures and algorithms used in OS design.
- System Programming: Knowledge of system calls and low-level programming.
Development Environment Setup
- Choose a Programming Language: Primarily C or C++ for the OS kernel.
- Set Up a Cross-Compiler: Use a cross-compiler to generate binaries for your target architecture.
- Create a Bootable Image: Learn to create bootable images to run on emulators or real hardware.
- Use Emulators: QEMU or Bochs for testing your OS without needing physical hardware.
Basic Structure of an Operating System
#include
include
void kernel_main() {
// This is where your kernel code will begin executing.
const char *str = "Hello, Operating System!";
// Function to output to the screen (implement your own).
print_string(str);
}
void print_string(const char *str) {
while (*str) {
// Output each character to the screen
// Implementation dependent on the hardware.
}
}
Learning Resources
- Books: "Operating Systems: Three Easy Pieces" by Remzi H. Arpaci-Dusseau, "Modern Operating Systems" by Andrew S. Tanenbaum.
- Online Courses: Look for OS development courses on platforms like Coursera or edX.
- Open Source Projects: Study existing open-source OS projects like Linux, MINIX, or FreeRTOS.
- Communities: Engage in forums like Stack Overflow, Reddit, or specialized OS development communities.
Challenges in OS Development
- Debugging low-level code can be complex and time-consuming.
- Resource management and concurrency can introduce unexpected behavior.
- Compatibility with various hardware can be a significant hurdle.
- Performance optimization is crucial for a functional OS.
Conclusion
Building an operating system from scratch is a daunting task that requires patience, dedication, and a thirst for knowledge. However, the skills you develop and the understanding you gain will be invaluable in your programming career. Start with small projects, gradually expand your scope, and enjoy the journey into the world of OS development!