Laravel PWA Master Guide: Build App-Like Experiences with Laravel in 5 Minutes
Introduction In today's mobile-first world, Progressive Web Applications (PWAs) have revolutionized how users interact with websites. For Laravel developers looking to enhance user experience, PWAs offer the perfect solution by combining the best features of web and mobile applications. This guide will walk you through integrating PWA functionality into your Laravel projects using the powerful erag/laravel-pwa package. What Are Progressive Web Applications? Progressive Web Applications are websites that function like native mobile applications. They offer: Offline Access: Users can access your application even without an internet connection Fast Loading: PWAs load quickly, even on slow networks Home Screen Installation: Users can add your app to their home screen Native-Like Experience: Smooth transitions and responsive design Why Add PWA to Your Laravel Project? Enhanced User Experience: Provide a seamless, app-like experience Increased Engagement: Users spend 40% more time on PWAs than on regular websites Better Conversion Rates: PWAs have shown to increase conversions by up to 36% Reduced Development Costs: Build once for all platforms instead of separate native apps Improved SEO: Google ranks mobile-friendly websites higher Getting Started with Laravel PWA Integration Let's transform your Laravel application into a PWA with the erag/laravel-pwa package. Step 1: Install the Package Begin by installing the package via Composer: composer require erag/laravel-pwa Step 2: Run the Installation Command Once installed, publish the PWA configuration files using: php artisan erag:install-pwa This command creates the necessary configuration files and sets up the PWA foundation for your application. Step 3: Configure Your PWA The installation creates a config/pwa.php file where you can customize all aspects of your PWA: return [ 'install-button' => true, // Control visibility of the install button 'manifest' => [ 'name' => 'Your App Name', 'short_name' => 'App', 'background_color' => '#6777ef', 'display' => 'fullscreen', 'description' => 'Your app description here', 'theme_color' => '#6777ef', 'icons' => [ [ 'src' => 'logo.png', 'sizes' => '512x512', 'type' => 'image/png', ], ], ], 'debug' => env('APP_DEBUG', false), ]; Step 4: Update Your Layout Files To integrate PWA functionality, add the following Blade directives to your layout file: In the section: @PwaHead Before the closing tag: @RegisterServiceWorkerScript Step 5: Update the Manifest After customizing your configuration, update the manifest file: php artisan erag:update-manifest This generates a manifest.json file in your public directory. Step 6: Add a Custom Logo For the best user experience, add a custom logo: Create a form with a file input field named "logo" Process the upload with the provided utility method: namespace App\Http\Controllers; use EragLaravelPwa\Core\PWA; use Illuminate\Http\Request; use Illuminate\Routing\Controller; class SettingsController extends Controller { public function uploadLogo(Request $request) { $response = PWA::processLogo($request); if ($response['status']) { return redirect()->back()->with('success', $response['message']); } return redirect()->back()->withErrors($response['errors']); } } Advanced Configuration Options Customizing the Install Button You can control the visibility of the install button globally in the config file or conditionally in your views: @if(auth()->user()->isPremium()) @PwaInstallButton @endif Offline Page Customization The package automatically generates an offline page. You can customize this by creating your own offline template in resources/views/vendor/pwa/offline.blade.php. Service Worker Strategies For advanced users, you can modify the caching strategies in the service worker file: Publish the service worker template Adjust caching rules for different file types Implement custom fetch handlers Testing Your PWA Before deployment, test your PWA thoroughly: Lighthouse Audit: Use Chrome's Lighthouse to audit your PWA Offline Testing: Test functionality without an internet connection Installation Flow: Verify the app installs correctly on various devices Performance: Check loading times and responsiveness Common Issues and Solutions HTTPS Requirement Issue: PWAs require HTTPS to function correctly. Solution: Set up SSL certificates for your production environment. For local development, use Laravel Valet or Laravel Homestead which provide HTTPS support. Service Worker Not Regi
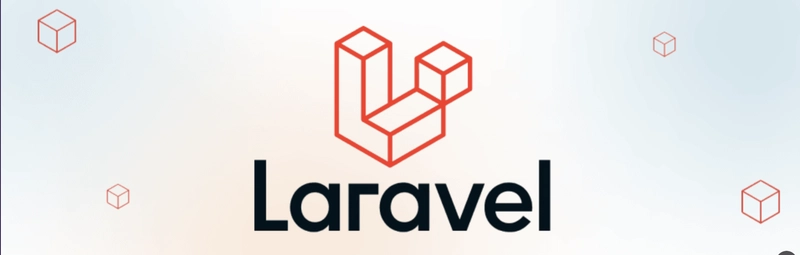
Introduction
In today's mobile-first world, Progressive Web Applications (PWAs) have revolutionized how users interact with websites. For Laravel developers looking to enhance user experience, PWAs offer the perfect solution by combining the best features of web and mobile applications. This guide will walk you through integrating PWA functionality into your Laravel projects using the powerful erag/laravel-pwa
package.
What Are Progressive Web Applications?
Progressive Web Applications are websites that function like native mobile applications. They offer:
- Offline Access: Users can access your application even without an internet connection
- Fast Loading: PWAs load quickly, even on slow networks
- Home Screen Installation: Users can add your app to their home screen
- Native-Like Experience: Smooth transitions and responsive design
Why Add PWA to Your Laravel Project?
- Enhanced User Experience: Provide a seamless, app-like experience
- Increased Engagement: Users spend 40% more time on PWAs than on regular websites
- Better Conversion Rates: PWAs have shown to increase conversions by up to 36%
- Reduced Development Costs: Build once for all platforms instead of separate native apps
- Improved SEO: Google ranks mobile-friendly websites higher
Getting Started with Laravel PWA Integration
Let's transform your Laravel application into a PWA with the erag/laravel-pwa
package.
Step 1: Install the Package
Begin by installing the package via Composer:
composer require erag/laravel-pwa
Step 2: Run the Installation Command
Once installed, publish the PWA configuration files using:
php artisan erag:install-pwa
This command creates the necessary configuration files and sets up the PWA foundation for your application.
Step 3: Configure Your PWA
The installation creates a config/pwa.php
file where you can customize all aspects of your PWA:
return [
'install-button' => true, // Control visibility of the install button
'manifest' => [
'name' => 'Your App Name',
'short_name' => 'App',
'background_color' => '#6777ef',
'display' => 'fullscreen',
'description' => 'Your app description here',
'theme_color' => '#6777ef',
'icons' => [
[
'src' => 'logo.png',
'sizes' => '512x512',
'type' => 'image/png',
],
],
],
'debug' => env('APP_DEBUG', false),
];
Step 4: Update Your Layout Files
To integrate PWA functionality, add the following Blade directives to your layout file:
In the section:
@PwaHead
Before the closing tag:
@RegisterServiceWorkerScript
Step 5: Update the Manifest
After customizing your configuration, update the manifest file:
php artisan erag:update-manifest
This generates a manifest.json
file in your public directory.
Step 6: Add a Custom Logo
For the best user experience, add a custom logo:
- Create a form with a file input field named "logo"
- Process the upload with the provided utility method:
namespace App\Http\Controllers;
use EragLaravelPwa\Core\PWA;
use Illuminate\Http\Request;
use Illuminate\Routing\Controller;
class SettingsController extends Controller
{
public function uploadLogo(Request $request)
{
$response = PWA::processLogo($request);
if ($response['status']) {
return redirect()->back()->with('success', $response['message']);
}
return redirect()->back()->withErrors($response['errors']);
}
}
Advanced Configuration Options
Customizing the Install Button
You can control the visibility of the install button globally in the config file or conditionally in your views:
@if(auth()->user()->isPremium())
@PwaInstallButton
@endif
Offline Page Customization
The package automatically generates an offline page. You can customize this by creating your own offline template in resources/views/vendor/pwa/offline.blade.php
.
Service Worker Strategies
For advanced users, you can modify the caching strategies in the service worker file:
- Publish the service worker template
- Adjust caching rules for different file types
- Implement custom fetch handlers
Testing Your PWA
Before deployment, test your PWA thoroughly:
- Lighthouse Audit: Use Chrome's Lighthouse to audit your PWA
- Offline Testing: Test functionality without an internet connection
- Installation Flow: Verify the app installs correctly on various devices
- Performance: Check loading times and responsiveness
Common Issues and Solutions
HTTPS Requirement
Issue: PWAs require HTTPS to function correctly.
Solution: Set up SSL certificates for your production environment. For local development, use Laravel Valet or Laravel Homestead which provide HTTPS support.
Service Worker Not Registering
Issue: The service worker fails to register.
Solution: Ensure the service worker path is correct and accessible. Check browser console for errors.
Manifest Not Found
Issue: Browser cannot find the manifest file.
Solution: Verify the manifest.json is in the public directory and properly referenced in your HTML.
Compatibility
The erag/laravel-pwa
package supports:
- Laravel 8, 9, 10, 11, and 12
- All major browsers (Chrome, Firefox, Safari, Edge)
- Mobile and desktop devices
Conclusion
Implementing PWA functionality in your Laravel application enhances user experience dramatically while potentially boosting engagement and conversion rates. With the erag/laravel-pwa
package, this integration becomes straightforward, allowing you to focus on building great features rather than wrestling with PWA complexities.
Take your Laravel application to the next level by transforming it into a Progressive Web App today. Your users will thank you with increased engagement and loyalty.
Updated: April 2025 | Laravel PWA Implementation Guide