Go - (7) Maps
Maps A collection of key-value pairs You can initialize maps like below. students := make(map[string]int) students["SE"] = 100 students["BA"] = 73 students["DA"] = 49 ages := map[string]int { "Bella": 17, "Edward": 18, } To get the number of key-value pairs in the map, use the len () function. Searching for a value in a map using its key is much faster than searching a slice. You should search the index by index in a slice until you find the value. Syntaxes of Maps insert a value: mapName[key] = value get a value: value = mapName[key] delete a value: delete(mapName, key) check if a key exists: value =, ok := mapName[key] Any type can be used as map values, but not as map keys. Keys should be comparable types (numeric, string, bool, channel, pointer, and interface). So, types like slice or array cannot be used as map keys. If you try to access a nil map, the code panics. If you try to access a value where the key doesn't exist, the code returns the zero value. You can't have duplicate keys. A key can have a maximum of one value assigned to it. If you pass a map to a function and update its elements inside the function, the content of the original map is changed.
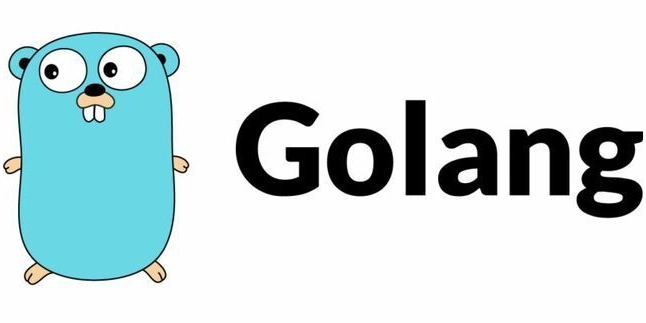
Maps
- A collection of key-value pairs
You can initialize maps like below.
students := make(map[string]int)
students["SE"] = 100
students["BA"] = 73
students["DA"] = 49
ages := map[string]int {
"Bella": 17,
"Edward": 18,
}
To get the number of key-value pairs in the map, use the len ()
function.
Searching for a value in a map using its key is much faster than searching a slice. You should search the index by index in a slice until you find the value.
Syntaxes of Maps
- insert a value:
mapName[key] = value
- get a value:
value = mapName[key]
- delete a value:
delete(mapName, key)
- check if a key exists:
value =, ok := mapName[key]
Any type can be used as map values, but not as map keys. Keys should be comparable types (numeric, string, bool, channel, pointer, and interface). So, types like slice or array cannot be used as map keys.
- If you try to access a nil map, the code panics.
- If you try to access a
value
where the key doesn't exist, the code returns the zero value. - You can't have duplicate keys. A key can have a maximum of one value assigned to it.
- If you pass a map to a function and update its elements inside the function, the content of the original map is changed.