LangGraph: Where AI Workflows Meet Finger Painting (for Grown-Up Devs)
Welcome to the Playground, Nerds! Hey there, code wranglers and AI enthusiasts! Pull up a chair, grab your favorite caffeinated beverage, and let's chat about something that's got me more excited than finding an unexpected semicolon in JavaScript that actually belongs there. Today, we're diving into the colorful world of LangGraph – it's like finger painting for AI workflows, but instead of ending up with a mess on your parents' fridge, you might just revolutionize your development process. What in the Name of Turing is LangGraph? LangGraph is the new kid on the block in the AI development playground. It's a visual tool that lets you build AI workflows without getting lost in a labyrinth of code. Think of it as the lovechild of a flowchart and an AI model – beautiful, intuitive, and surprisingly powerful. But why should you care? Well, my fellow keyboard warriors, LangGraph is here to make our lives easier. It's like having a really smart friend who can translate your napkin scribbles into a working AI system. And who doesn't want a friend like that? Getting Started: Your First LangGraph Masterpiece Alright, let's roll up our sleeves and get our hands dirty (metaphorically, of course – we're not actually finger painting here). To start your LangGraph journey, you'll need: A computer (duh) Python installed (preferably a version that doesn't predate the invention of the wheel) A burning desire to make AI bend to your will First things first, let's install LangGraph: pip install langgraph If your terminal spits out a bunch of text and doesn't catch fire, congratulations! You're halfway there. Your First LangGraph Flow: The "Hello, AI" of Workflows Let's create a simple workflow that takes a user's input, processes it through a language model, and returns a witty response. Because if we're going to play with AI, we might as well make it sass us back, right? Here's a basic example: from langgraph import Graph from langgraph.components import LLMComponent, InputComponent, OutputComponent # Create our graph graph = Graph() # Add components input_component = InputComponent() llm_component = LLMComponent(model="gpt-3.5-turbo") output_component = OutputComponent() # Connect the components graph.add_node(input_component, "input") graph.add_node(llm_component, "process") graph.add_node(output_component, "output") graph.add_edge("input", "process") graph.add_edge("process", "output") # Run the graph result = graph.run("Tell me a joke about programming") print(result) And voila! You've just created an AI workflow that can tell jokes. It's like having a stand-up comedian in your code, minus the awkward silence when the jokes fall flat. LangGraph: The Swiss Army Knife of AI Workflows Now, I know what you're thinking: "This is cool and all, but can it help me take over the world?" Well, not exactly (and please don't), but LangGraph is incredibly versatile. Here are just a few things you can do with it: Create chatbots that are actually helpful (a rare breed indeed) Build content generation pipelines that don't sound like they were written by a caffeinated squirrel Develop question-answering systems that don't just reply "42" to everything The beauty of LangGraph is that it lets you focus on the logic and flow of your AI system, rather than getting bogged down in implementation details. It's like being the director of an AI movie – you get to call the shots without having to personally operate every camera. Tips and Tricks for LangGraph Mastery As you embark on your LangGraph adventure, here are some pearls of wisdom to keep in mind: Start simple: Don't try to build Skynet on your first go. Start with basic workflows and build up. Visualize your graph: LangGraph lets you export your workflow as a visual graph. Use this to debug and explain your work (or to impress your crush at the next hackathon). Experiment with different components: LangGraph comes with a variety of pre-built components. Mix and match to find the perfect combination for your needs. Don't forget error handling: Even AI can have bad days. Make sure your workflow can handle unexpected inputs gracefully. Document your workflows: Future you will thank present you for leaving breadcrumbs explaining what each node does. The Future is Graphical (and Slightly Sarcastic) As we wrap up our journey into the land of LangGraph, remember that this is just the beginning. The field of AI is evolving faster than a chameleon on a disco floor, and tools like LangGraph are making it more accessible than ever. So go forth, my fellow code slingers! Build workflows, create AI wonders, and maybe, just maybe, you'll create something that makes even the most stoic senior developer crack a smile. And hey, if you enjoyed this rollercoaster ride through the world of LangGraph, why not follow me for more dev adventures? I promise more terrible puns, questionable anal
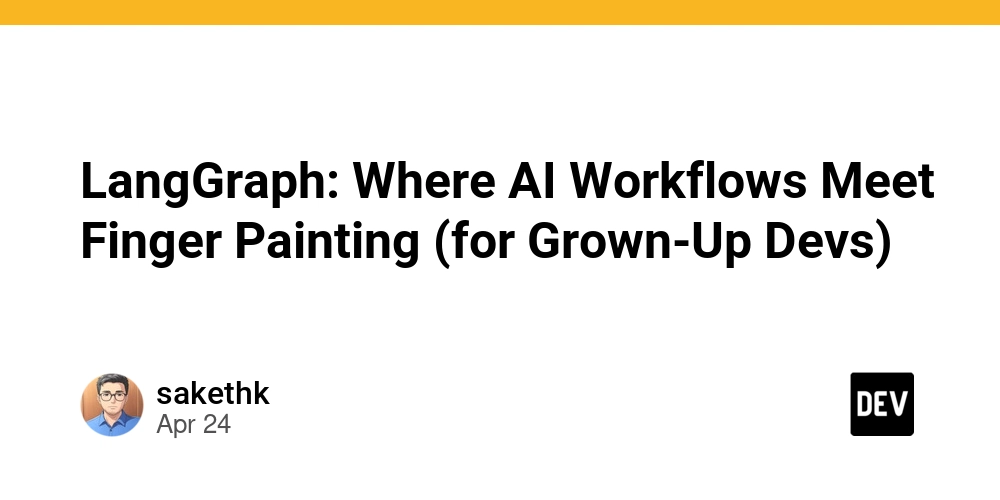
Welcome to the Playground, Nerds!
Hey there, code wranglers and AI enthusiasts! Pull up a chair, grab your favorite caffeinated beverage, and let's chat about something that's got me more excited than finding an unexpected semicolon in JavaScript that actually belongs there. Today, we're diving into the colorful world of LangGraph – it's like finger painting for AI workflows, but instead of ending up with a mess on your parents' fridge, you might just revolutionize your development process.
What in the Name of Turing is LangGraph?
LangGraph is the new kid on the block in the AI development playground. It's a visual tool that lets you build AI workflows without getting lost in a labyrinth of code. Think of it as the lovechild of a flowchart and an AI model – beautiful, intuitive, and surprisingly powerful.
But why should you care? Well, my fellow keyboard warriors, LangGraph is here to make our lives easier. It's like having a really smart friend who can translate your napkin scribbles into a working AI system. And who doesn't want a friend like that?
Getting Started: Your First LangGraph Masterpiece
Alright, let's roll up our sleeves and get our hands dirty (metaphorically, of course – we're not actually finger painting here). To start your LangGraph journey, you'll need:
- A computer (duh)
- Python installed (preferably a version that doesn't predate the invention of the wheel)
- A burning desire to make AI bend to your will
First things first, let's install LangGraph:
pip install langgraph
If your terminal spits out a bunch of text and doesn't catch fire, congratulations! You're halfway there.
Your First LangGraph Flow: The "Hello, AI" of Workflows
Let's create a simple workflow that takes a user's input, processes it through a language model, and returns a witty response. Because if we're going to play with AI, we might as well make it sass us back, right?
Here's a basic example:
from langgraph import Graph
from langgraph.components import LLMComponent, InputComponent, OutputComponent
# Create our graph
graph = Graph()
# Add components
input_component = InputComponent()
llm_component = LLMComponent(model="gpt-3.5-turbo")
output_component = OutputComponent()
# Connect the components
graph.add_node(input_component, "input")
graph.add_node(llm_component, "process")
graph.add_node(output_component, "output")
graph.add_edge("input", "process")
graph.add_edge("process", "output")
# Run the graph
result = graph.run("Tell me a joke about programming")
print(result)
And voila! You've just created an AI workflow that can tell jokes. It's like having a stand-up comedian in your code, minus the awkward silence when the jokes fall flat.
LangGraph: The Swiss Army Knife of AI Workflows
Now, I know what you're thinking: "This is cool and all, but can it help me take over the world?" Well, not exactly (and please don't), but LangGraph is incredibly versatile. Here are just a few things you can do with it:
- Create chatbots that are actually helpful (a rare breed indeed)
- Build content generation pipelines that don't sound like they were written by a caffeinated squirrel
- Develop question-answering systems that don't just reply "42" to everything
The beauty of LangGraph is that it lets you focus on the logic and flow of your AI system, rather than getting bogged down in implementation details. It's like being the director of an AI movie – you get to call the shots without having to personally operate every camera.
Tips and Tricks for LangGraph Mastery
As you embark on your LangGraph adventure, here are some pearls of wisdom to keep in mind:
Start simple: Don't try to build Skynet on your first go. Start with basic workflows and build up.
Visualize your graph: LangGraph lets you export your workflow as a visual graph. Use this to debug and explain your work (or to impress your crush at the next hackathon).
Experiment with different components: LangGraph comes with a variety of pre-built components. Mix and match to find the perfect combination for your needs.
Don't forget error handling: Even AI can have bad days. Make sure your workflow can handle unexpected inputs gracefully.
Document your workflows: Future you will thank present you for leaving breadcrumbs explaining what each node does.
The Future is Graphical (and Slightly Sarcastic)
As we wrap up our journey into the land of LangGraph, remember that this is just the beginning. The field of AI is evolving faster than a chameleon on a disco floor, and tools like LangGraph are making it more accessible than ever.
So go forth, my fellow code slingers! Build workflows, create AI wonders, and maybe, just maybe, you'll create something that makes even the most stoic senior developer crack a smile.
And hey, if you enjoyed this rollercoaster ride through the world of LangGraph, why not follow me for more dev adventures? I promise more terrible puns, questionable analogies, and the occasional nugget of actual wisdom. It's like subscribing to a comedy newsletter, but with more brackets and fewer restraining orders.
Keep coding, stay curious, and remember: in the world of AI, we're all just trying to teach machines to be a little more human... and sometimes a little less like that one coworker who always microwaves fish in the office kitchen.