Javascript: Optional Chaining
As a self-taught developer I came across this that I've never heard of don’t judge me though. Optional chaining a method so simple as it is yet powerful tool to safely access deeply nested object properties, without worrying about errors like "Cannot read property of undefined." The Problem Before Optional Chaining During my study or should i say discovery, accessing nested properties in objects required a lot of checks to prevent the infamous type error. For instance in this scenario: const user = {profile: {name: "John",},}; console.log(user.profile.name); // "John" console.log(user.profile.age); // undefined This works fine, but what if ‘profile’ itself was undefined? const user = {}; console.log(user.profile.name); // Uncaught TypeError: Cannot read property name of undefined You would typically need to check each level like this: const user = {}; console.log(user && user.profile && user.profile.name); // undefined** ` This to me was verbose and hard to maintain as the object structure became more complex Enter Optional Chaining (?.) Optional chaining simplifies this by allowing you to safely access properties at any depth. If any part of the chain is null or undefined, it will short-circuit and return undefined instead of throwing an error. const user = {}; console.log(user.profile?.name); // undefined, no error With optional chaining, you can now write cleaner, more readable code that is much safer to work with when accessing nested properties. How It Works The ?. operator works by checking if the value before it is null or undefined. If it is, the entire expression evaluates to undefined. If not, it continues to access the property. Here’s a breakdown: object?.property: Accesses object.property if object exists. object?.[key]: Accesses object[key] if object exists. object?.method(): Calls object.method() if object and method exist. A Few More Examples Accessing Nested Properties: const user = {profile: {name: "Alice", contact: {email: alice@example.com",},},}; console.log(user.profile?.contact?.email); // alice@example.com console.log(user.profile?.contact?.phone); // undefined Without optional chaining, you’d have to check every property manually to avoid runtime errors. Calling Methods: ` const user = { profile: { greet() { return "Hello!"; }, }, }; console.log(user.profile?.greet()); // "Hello!" console.log(user.profile?.farewell?.()); // undefined, no error ` You can even safely call methods without worrying whether they exist. When to Use Optional Chaining? Optional chaining is best used when you’re dealing with objects that might not always have all the properties you expect. This is especially common when working with data from APIs or user-generated content. It helps avoid unnecessary checks and makes your code more concise. Fallback Values with Nullish Coalescing (??) A good companion to optional chaining is the nullish coalescing operator (??). It allows you to provide a default value when optional chaining returns undefined or null. const user = {}; const name = user.profile?.name ?? "Guest"; // "Guest" In this example, if user.profile?.name is undefined, the fallback value "Guest" will be used. Conclusion Optional chaining is a simple yet powerful tool that helps eliminate common pitfalls when accessing nested properties in JavaScript. It makes your code cleaner, more readable, and easier to maintain. If you haven’t started using it yet, now is the time to incorporate this feature into your projects!
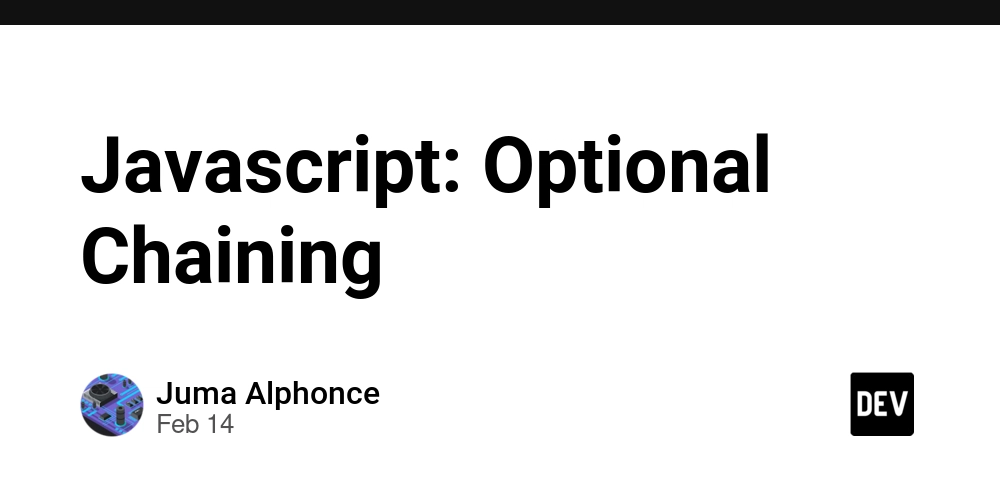
As a self-taught developer I came across this that I've never heard of don’t judge me though. Optional chaining a method so simple as it is yet powerful tool to safely access deeply nested object properties, without worrying about errors like "Cannot read property of undefined."
The Problem Before Optional Chaining
During my study or should i say discovery, accessing nested properties in objects required a lot of checks to prevent the infamous type error. For instance in this scenario:
const user = {profile: {name: "John",},}; console.log(user.profile.name); // "John" console.log(user.profile.age); // undefined
This works fine, but what if ‘profile’ itself was undefined?
const user = {};
console.log(user.profile.name); // Uncaught TypeError: Cannot read property name of undefined
You would typically need to check each level like this:
const user = {};
console.log(user && user.profile && user.profile.name); // undefined**
`
This to me was verbose and hard to maintain as the object structure became more complex
Enter Optional Chaining (?.)
Optional chaining simplifies this by allowing you to safely access properties at any depth. If any part of the chain is null or undefined, it will short-circuit and return undefined instead of throwing an error.
const user = {};
console.log(user.profile?.name); // undefined, no error
With optional chaining, you can now write cleaner, more readable code that is much safer to work with when accessing nested properties.
How It Works
The ?. operator works by checking if the value before it is null or undefined. If it is, the entire expression evaluates to undefined. If not, it continues to access the property.
Here’s a breakdown:
object?.property: Accesses object.property if object exists.
object?.[key]: Accesses object[key] if object exists.
object?.method(): Calls object.method() if object and method exist.
A Few More Examples
Accessing Nested Properties:
const user = {profile: {name: "Alice", contact: {email: alice@example.com",},},};
console.log(user.profile?.contact?.email); // alice@example.com
console.log(user.profile?.contact?.phone); // undefined
Without optional chaining, you’d have to check every property manually to avoid runtime errors.
Calling Methods:
`
const user = {
profile: {
greet() {
return "Hello!";
},
},
};
console.log(user.profile?.greet()); // "Hello!"
console.log(user.profile?.farewell?.()); // undefined, no error
`
You can even safely call methods without worrying whether they exist.
When to Use Optional Chaining?
Optional chaining is best used when you’re dealing with objects that might not always have all the properties you expect. This is especially common when working with data from APIs or user-generated content. It helps avoid unnecessary checks and makes your code more concise.
Fallback Values with Nullish Coalescing (??)
A good companion to optional chaining is the nullish coalescing operator (??). It allows you to provide a default value when optional chaining returns undefined or null.
const user = {};
const name = user.profile?.name ?? "Guest"; // "Guest"
In this example, if user.profile?.name is undefined, the fallback value "Guest" will be used.
Conclusion
Optional chaining is a simple yet powerful tool that helps eliminate common pitfalls when accessing nested properties in JavaScript. It makes your code cleaner, more readable, and easier to maintain. If you haven’t started using it yet, now is the time to incorporate this feature into your projects!