Is Redux Necessary or is it Just About Following 'Best Practices' Without Questioning?
If you've been around React devs long enough, you've probably heard these sacred commandments: Thou shalt not use Context for state management. Thou shalt install Redux for all global state needs. Okay, maybe they aren't official, but it sure feels that way. But here’s the thing, Redux was built to solve a problem React no longer has at the same scale. So, do you actually need it? Or is it just about following 'best practices' without questioning? Let's talk about it. About Context API Context has always been the underdog. It was originally built to solve prop drilling, not global state management. And if you tried using it for state updates back in the pre-Hooks era, you probably had flashbacks of: You just triggered a full app re-render, lol! No wonder people ran to Redux. But then came Hooks. Suddenly, with useContextand useReducer, Context could actually manage state, update it predictably, and optimize performance with useMemo and React.memo. So, does that mean Context + Hooks can replace Redux? When Context + Hooks Is Enough For most apps, Context + Hooks gets the job done. It works great for: Themes (light/dark mode) Authentication (user session data) App settings (language, preferences) And with useReducer, it can even handle more structured updates. For example, let’s say you need authentication state: const AuthContext = createContext(); const authReducer = (state, action) => { switch (action.type) { case "LOGIN": return { ...state, user: action.payload, isAuthenticated: true }; case "LOGOUT": return { ...state, user: null, isAuthenticated: false }; default: return state; } }; export const AuthProvider = ({ children }) => { const [state, dispatch] = useReducer(authReducer, { user: null, isAuthenticated: false }); return {children}; }; export const useAuth = () => useContext(AuthContext); That’s state management without Redux’s boilerplate. But What About Redux’s Superpowers ? Look, I get it,Redux does have some cool tricks: Time-travel debugging (because who doesn’t want to play god with their app’s history?) Middleware(like redux-thunk for async operations) Global predictability (because structuring state matters in big teams) But here’s the question, Does your app need all of that? If your app is handling: High-frequency state updates (real-time dashboards, websockets) Complex derived state (data that depends on multiple sources) Massive developer teams with strict state management rules Then Redux might be worth it. But if your app doesn’t check those boxes, you might just be over-engineering things. Still Not Sure? Let’s Play "Should I Use Redux?" Do you need to debug state like it’s a Netflix time-travel show? Yes? Go Redux. No? Context + Hooks is fine. Does your app need middleware like redux-thunk or redux-saga? Yes? Redux. No? Context + Hooks. Do you want to write a reducer, action, dispatch, and store just to toggle a sidebar? Yes? Seek help. No? Context + Hooks. Small to medium apps? Context + Hooks" is your friend. Huge, complex apps with heavy async workflows? Redux might be worth the extra setup. Still confused? Start with Context. If things get messy, migrate to Redux later. So,do you really need Redux? Before you type npm install redux, ask yourself: "Am I solving a real problem, or am I just doing what everyone else does?" Still unsure? Check out my explanatory article about the specific use cases of each. Happy coding !
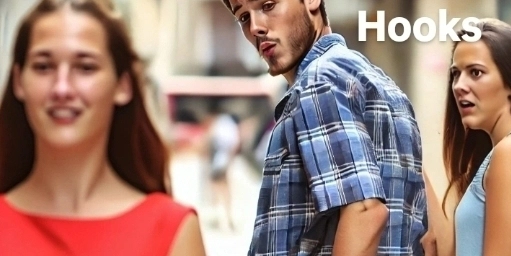
If you've been around React devs long enough, you've probably heard these sacred commandments:
Thou shalt not use Context for state management.
Thou shalt install Redux for all global state needs.
Okay, maybe they aren't official, but it sure feels that way.
But here’s the thing, Redux was built to solve a problem React no longer has at the same scale. So, do you actually need it? Or is it just about following 'best practices' without questioning?
Let's talk about it.
About Context API
Context has always been the underdog. It was originally built to solve prop drilling, not global state management. And if you tried using it for state updates back in the pre-Hooks era, you probably had flashbacks of:
You just triggered a full app re-render, lol!
No wonder people ran to Redux.
But then came Hooks.
Suddenly, with useContext
and useReducer
, Context could actually manage state, update it predictably, and optimize performance with useMemo
and React.memo
.
So, does that mean Context + Hooks can replace Redux?
When Context + Hooks Is Enough
For most apps, Context + Hooks gets the job done. It works great for:
- Themes (light/dark mode)
- Authentication (user session data)
- App settings (language, preferences)
And with useReducer
, it can even handle more structured updates.
For example, let’s say you need authentication state:
const AuthContext = createContext();
const authReducer = (state, action) => {
switch (action.type) {
case "LOGIN":
return { ...state, user: action.payload, isAuthenticated: true };
case "LOGOUT":
return { ...state, user: null, isAuthenticated: false };
default:
return state;
}
};
export const AuthProvider = ({ children }) => {
const [state, dispatch] = useReducer(authReducer, { user: null, isAuthenticated: false });
return {children} ;
};
export const useAuth = () => useContext(AuthContext);
That’s state management without Redux’s boilerplate.
But What About Redux’s Superpowers ?
Look, I get it,Redux does have some cool tricks:
- Time-travel debugging (because who doesn’t want to play god with their app’s history?)
- Middleware(like redux-thunk for async operations)
- Global predictability (because structuring state matters in big teams)
But here’s the question, Does your app need all of that?
If your app is handling:
- High-frequency state updates (real-time dashboards, websockets)
- Complex derived state (data that depends on multiple sources)
- Massive developer teams with strict state management rules
Then Redux might be worth it.
But if your app doesn’t check those boxes, you might just be over-engineering things.
Still Not Sure? Let’s Play "Should I Use Redux?"
Do you need to debug state like it’s a Netflix time-travel show?
Yes? Go Redux.
No? Context + Hooks is fine.Does your app need middleware like redux-thunk or redux-saga?
Yes? Redux.
No? Context + Hooks.
Do you want to write a reducer
, action
, dispatch
, and store
just to toggle a sidebar?
Yes? Seek help.
No? Context + Hooks.
- Small to medium apps? Context + Hooks" is your friend.
- Huge, complex apps with heavy async workflows? Redux might be worth the extra setup.
Still confused? Start with Context. If things get messy, migrate to Redux later.
So,do you really need Redux?
Before you type npm install redux
, ask yourself:
"Am I solving a real problem, or am I just doing what everyone else does?"
Still unsure? Check out my explanatory article about the specific use cases of each.
Happy coding !