Building Robust Python Data Models with Pydantic Validators
What is Pydantic? Pydantic is a powerful data validation library for Python that leverages Python’s type hints. It is particularly useful for ensuring data integrity and consistency in complex applications, helping to catch errors in development. What are Validators? Validators in Pydantic ensure that data conforms to specific rules beyond extend beyond basic type checking, enabling the enforcement of sophisticated validation logic. Working Example Let's take the example of a % discount on a product. With pydantic, we can define the model of a Product and ensure that the discount value will be a float from pydantic import BaseModel class Product(BaseModel): name: str discount: float Unfortunatly, this description of the product does not give us full control over the data structure. The discount is enforced to be a floating point number, but it could be 0.1, 999.1 or -3.14, which does not make sense in the context of a percentage. Where Validators come in Using Pydantic Validators, we can enforce custom type checking! In this case lets set the bounds of discount to be between 0 and 1. There are 2 ways of defining a custom validator: Using the @field_validator Decorator we define a decorated function used to validate the discount field, where we return a ValueError if the validation criteria is not met. from pydantic import BaseModel, field_validator class Product(BaseModel): name: str discount: float @field_validator('discount') def bounded_0_1(value: float) -> float: if 0.
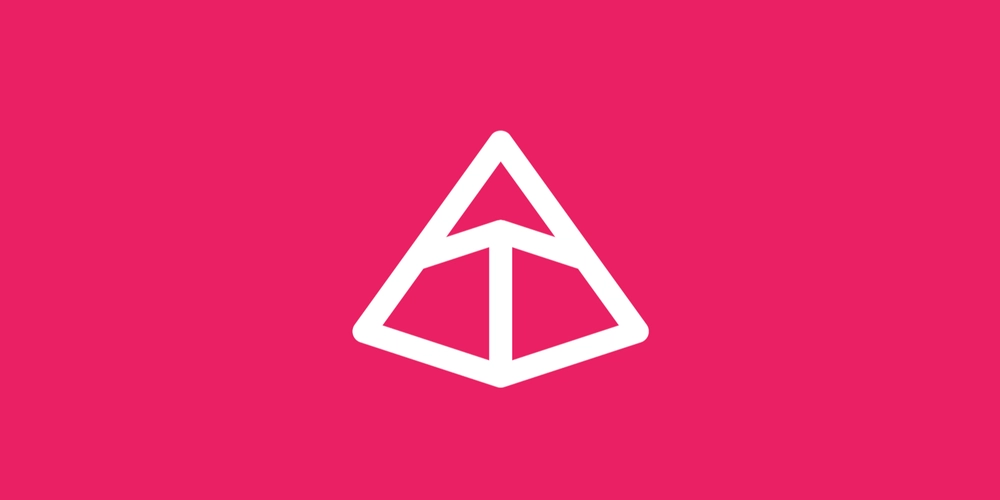
What is Pydantic?
Pydantic is a powerful data validation library for Python that leverages Python’s type hints. It is particularly useful for ensuring data integrity and consistency in complex applications, helping to catch errors in development.
What are Validators?
Validators in Pydantic ensure that data conforms to specific rules beyond extend beyond basic type checking, enabling the enforcement of sophisticated validation logic.
Working Example
Let's take the example of a % discount on a product. With pydantic, we can define the model of a Product
and ensure that the discount value will be a float
from pydantic import BaseModel
class Product(BaseModel):
name: str
discount: float
Unfortunatly, this description of the product does not give us full control over the data structure. The discount is enforced to be a floating point number, but it could be 0.1, 999.1 or -3.14, which does not make sense in the context of a percentage.
Where Validators come in
Using Pydantic Validators, we can enforce custom type checking! In this case lets set the bounds of discount
to be between 0 and 1.
There are 2 ways of defining a custom validator:
Using the @field_validator
Decorator
we define a decorated function used to validate the discount
field, where we return a ValueError
if the validation criteria is not met.
from pydantic import BaseModel, field_validator
class Product(BaseModel):
name: str
discount: float
@field_validator('discount')
def bounded_0_1(value: float) -> float:
if 0. <= value and value <= 1.:
return value
raise ValueError(f'{value} is not between 0 and 1')
Using Annotated
from the typing
module
You can also define a validator by providing a metadata function to the standard library's Annotated
. This is especialy useful if you have a commonly used validator that resides somewhere else in the code base, allowing you to import it when needed:
from pydantic import BaseModel, AfterValidator
from typing import Annotated # Avaliable from typing_extensions in python versions older than 3.9
from validators import bounded_0_1
class Product(BaseModel):
name: str
discount: Annotated[float, AfterValidator(bounded_0_1)]
AfterValidator runs after Pydantic's internal validation. I would almost always advocate for using this over other types of validators, as they are generally more type safe.
Builtin Validators
Pydantic also provides a number of built in validation via the Field
class, which allows us to acheive the same result using a shorter inline notation:
from pydantic import BaseModel, Field
class Product(BaseModel):
name: str
discount: Annotated[float, Field(strict=True, ge=0, le=1)
Conclusion
Pydantic validators provide a flexible and powerful way to enforce custom validation logic in your data models. By leveraging these validators, you can ensure that your data is accurate and consistent, reducing the likelihood of errors and improving the overall robustness of your applications.
I urge you to check out Pydantic further, as Validators are just one of many powerful features that transform python into a more reliable programming language for large scale projects.