Integrating ChatGPT with Node.js: Build Your Own AI Chatbot
Introduction AI chatbots are revolutionizing how businesses and users interact with technology. By integrating ChatGPT with Node.js, you can build intelligent, conversational bots capable of answering queries, handling tasks, and improving customer experiences. In this blog, we’ll explore how to integrate OpenAI’s ChatGPT with a Node.js backend. Prerequisites Node.js installed OpenAI API key (get it from OpenAI) Basic knowledge of Express.js Step 1: Set Up Your Node.js Project mkdir chatgpt-chatbot cd chatgpt-chatbot npm init -y npm install express axios dotenv Create .env File OPENAI_API_KEY=your_openai_api_key Step 2: Create Express Server index.js const express = require('express'); const axios = require('axios'); require('dotenv').config(); const app = express(); app.use(express.json()); const OPENAI_API_KEY = process.env.OPENAI_API_KEY; app.post('/chat', async (req, res) => { const { message } = req.body; try { const response = await axios.post( 'https://api.openai.com/v1/chat/completions', { model: 'gpt-3.5-turbo', messages: [{ role: 'user', content: message }] }, { headers: { 'Authorization': `Bearer ${OPENAI_API_KEY}`, 'Content-Type': 'application/json' } } ); const reply = response.data.choices[0].message.content; res.json({ reply }); } catch (error) { res.status(500).json({ error: 'Something went wrong' }); } }); app.listen(3000, () => console.log('Server running on http://localhost:3000')); Step 3: Test Your Chatbot Use Postman or a frontend interface to make a POST request: Endpoint: http://localhost:3000/chat Method: POST Body (JSON): { "message": "What is the capital of France?" } Response: { "reply": "The capital of France is Paris." } Conclusion You’ve just built a simple AI chatbot using Node.js and OpenAI’s ChatGPT API! This is a foundational setup that can be expanded with user sessions, database integration, or even a real-time UI with WebSockets. AI is the future—build something amazing!
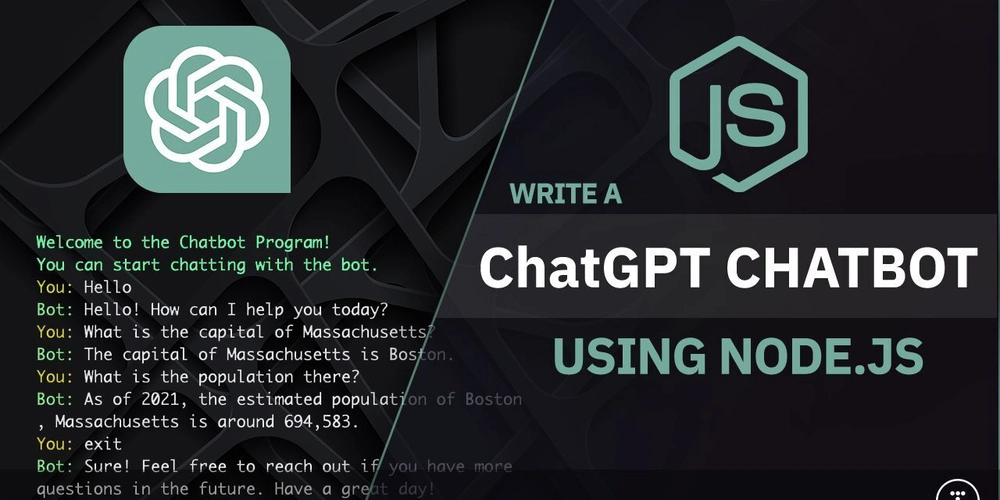
Introduction
AI chatbots are revolutionizing how businesses and users interact with technology. By integrating ChatGPT with Node.js, you can build intelligent, conversational bots capable of answering queries, handling tasks, and improving customer experiences. In this blog, we’ll explore how to integrate OpenAI’s ChatGPT with a Node.js backend.
Prerequisites
- Node.js installed
- OpenAI API key (get it from OpenAI)
- Basic knowledge of Express.js
Step 1: Set Up Your Node.js Project
mkdir chatgpt-chatbot
cd chatgpt-chatbot
npm init -y
npm install express axios dotenv
Create .env
File
OPENAI_API_KEY=your_openai_api_key
Step 2: Create Express Server
index.js
const express = require('express');
const axios = require('axios');
require('dotenv').config();
const app = express();
app.use(express.json());
const OPENAI_API_KEY = process.env.OPENAI_API_KEY;
app.post('/chat', async (req, res) => {
const { message } = req.body;
try {
const response = await axios.post(
'https://api.openai.com/v1/chat/completions',
{
model: 'gpt-3.5-turbo',
messages: [{ role: 'user', content: message }]
},
{
headers: {
'Authorization': `Bearer ${OPENAI_API_KEY}`,
'Content-Type': 'application/json'
}
}
);
const reply = response.data.choices[0].message.content;
res.json({ reply });
} catch (error) {
res.status(500).json({ error: 'Something went wrong' });
}
});
app.listen(3000, () => console.log('Server running on http://localhost:3000'));
Step 3: Test Your Chatbot
Use Postman or a frontend interface to make a POST request:
-
Endpoint:
http://localhost:3000/chat
- Method: POST
- Body (JSON):
{
"message": "What is the capital of France?"
}
Response:
{
"reply": "The capital of France is Paris."
}
Conclusion
You’ve just built a simple AI chatbot using Node.js and OpenAI’s ChatGPT API! This is a foundational setup that can be expanded with user sessions, database integration, or even a real-time UI with WebSockets. AI is the future—build something amazing!