A Beginner’s Guide to the Solana Ecosystem for Python Developers
As the blockchain industry matures, developers from various programming backgrounds are exploring opportunities to build decentralized applications (dApps). While Rust is the primary language for Solana development, Python, with its simplicity and versatility, has carved a niche for itself within the Solana ecosystem. In this article, we will explore how Python developers can effectively enter the Solana ecosystem in 2025, leveraging their existing skills to contribute to this high-performance blockchain. Why Python? Python has long been a favorite among developers due to its readability, extensive libraries, and vibrant community. Despite being traditionally associated with data science, machine learning, and web development, Python has found a significant presence in the blockchain world. Here’s why: Ease of Learning and Use: Python’s simple syntax makes it an excellent choice for developers transitioning to blockchain development. Rich Ecosystem: Python offers libraries like solana-py and tools to interact with the Solana blockchain seamlessly. Versatility: Python can be used for tasks ranging from scripting and data analysis to building backend services for dApps. Community Support: Python’s extensive community ensures that resources, tutorials, and forums are readily available for developers. Getting Started with Solana Using Python 1. Understanding Solana Basics Before diving into Python tools, it’s crucial to understand Solana’s key features: Proof of History (PoH): A consensus mechanism that enables high throughput and low latency. Parallel Processing: Solana’s architecture allows transactions to be processed concurrently. Native Programs: Pre-built programs, like token management, are available to simplify development. As a Python developer, you don’t need to master Solana’s inner workings right away. Focus on learning how to interact with the blockchain through Python libraries. 2. Installing the Necessary Tools To start building with Python on Solana, you’ll need to install a few essential tools: Python: Ensure Python 3.8 or higher is installed on your system. solana-py: The official Python SDK for interacting with Solana. AnchorPy: A Python library for working with Anchor, Solana’s popular framework for smart contract development. Installation Example pip install solana-py anchorpy These libraries provide everything you need to interact with Solana’s JSON RPC API, send transactions, and query on-chain data. 3. Connecting to the Solana Cluster Solana operates on different clusters: Mainnet Beta: The production network. Devnet: A testing environment for developers. Testnet: A network for more rigorous testing. You can connect to these clusters using the solana-py library. For example: from solana.rpc.api import Client # Connect to Devnet client = Client("https://api.devnet.solana.com") # Fetch cluster status status = client.get_health() print("Cluster status:", status) 4. Interacting with Accounts and Wallets Accounts and wallets are fundamental concepts in Solana. You can create and manage wallets using Python: from solana.keypair import Keypair from solana.rpc.async_api import AsyncClient from solana.rpc.types import TxOpts # Create a new wallet keypair = Keypair.generate() print("Public Key:", keypair.public_key) # Connect to Devnet async_client = AsyncClient("https://api.devnet.solana.com") # Airdrop SOL to the wallet await async_client.request_airdrop(keypair.public_key, 1_000_000_000) # 1 SOL in lamports 5. Building a Simple Transaction Sending transactions is a common task. Here’s how to send SOL from one account to another: from solana.transaction import Transaction, AccountMeta, TransactionInstruction from solana.rpc.async_api import AsyncClient # Set up sender and receiver keypairs sender = Keypair.generate() receiver = Keypair.generate() # Create a transaction transaction = Transaction() instruction = TransactionInstruction( keys=[ AccountMeta(pubkey=sender.public_key, is_signer=True, is_writable=True), AccountMeta(pubkey=receiver.public_key, is_signer=False, is_writable=True), ], program_id=SystemProgram.id(), data=b"", # Add instruction-specific data here ) transaction.add(instruction) # Send the transaction response = await async_client.send_transaction(transaction, sender) print("Transaction Response:", response) 6. Interacting with Tokens Solana’s SPL Token Program enables the creation and management of fungible and non-fungible tokens. Python makes it easy to interact with these tokens: Create a Token Account from solana.rpc.api import Client from spl.token.client import Token from spl.token.constants import TOKEN_PROGRAM_ID # Connect to Devnet client = Client("https://api.devnet.solana.com") # Create a token token = Token.create_mint( client, Keypair.gener
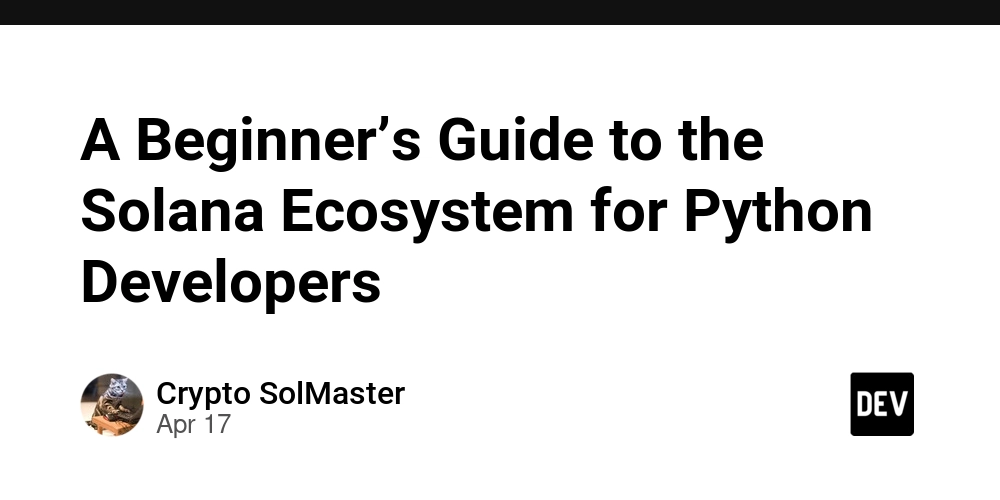
As the blockchain industry matures, developers from various programming backgrounds are exploring opportunities to build decentralized applications (dApps). While Rust is the primary language for Solana development, Python, with its simplicity and versatility, has carved a niche for itself within the Solana ecosystem. In this article, we will explore how Python developers can effectively enter the Solana ecosystem in 2025, leveraging their existing skills to contribute to this high-performance blockchain.
Why Python?
Python has long been a favorite among developers due to its readability, extensive libraries, and vibrant community. Despite being traditionally associated with data science, machine learning, and web development, Python has found a significant presence in the blockchain world. Here’s why:
- Ease of Learning and Use: Python’s simple syntax makes it an excellent choice for developers transitioning to blockchain development.
- Rich Ecosystem: Python offers libraries like solana-py and tools to interact with the Solana blockchain seamlessly.
- Versatility: Python can be used for tasks ranging from scripting and data analysis to building backend services for dApps.
- Community Support: Python’s extensive community ensures that resources, tutorials, and forums are readily available for developers.
Getting Started with Solana Using Python
1. Understanding Solana Basics
Before diving into Python tools, it’s crucial to understand Solana’s key features:
- Proof of History (PoH): A consensus mechanism that enables high throughput and low latency.
- Parallel Processing: Solana’s architecture allows transactions to be processed concurrently.
- Native Programs: Pre-built programs, like token management, are available to simplify development.
As a Python developer, you don’t need to master Solana’s inner workings right away. Focus on learning how to interact with the blockchain through Python libraries.
2. Installing the Necessary Tools
To start building with Python on Solana, you’ll need to install a few essential tools:
- Python: Ensure Python 3.8 or higher is installed on your system.
- solana-py: The official Python SDK for interacting with Solana.
- AnchorPy: A Python library for working with Anchor, Solana’s popular framework for smart contract development.
Installation Example
pip install solana-py anchorpy
These libraries provide everything you need to interact with Solana’s JSON RPC API, send transactions, and query on-chain data.
3. Connecting to the Solana Cluster
Solana operates on different clusters:
- Mainnet Beta: The production network.
- Devnet: A testing environment for developers.
- Testnet: A network for more rigorous testing.
You can connect to these clusters using the solana-py library. For example:
from solana.rpc.api import Client
# Connect to Devnet
client = Client("https://api.devnet.solana.com")
# Fetch cluster status
status = client.get_health()
print("Cluster status:", status)
4. Interacting with Accounts and Wallets
Accounts and wallets are fundamental concepts in Solana. You can create and manage wallets using Python:
from solana.keypair import Keypair
from solana.rpc.async_api import AsyncClient
from solana.rpc.types import TxOpts
# Create a new wallet
keypair = Keypair.generate()
print("Public Key:", keypair.public_key)
# Connect to Devnet
async_client = AsyncClient("https://api.devnet.solana.com")
# Airdrop SOL to the wallet
await async_client.request_airdrop(keypair.public_key, 1_000_000_000) # 1 SOL in lamports
5. Building a Simple Transaction
Sending transactions is a common task. Here’s how to send SOL from one account to another:
from solana.transaction import Transaction, AccountMeta, TransactionInstruction
from solana.rpc.async_api import AsyncClient
# Set up sender and receiver keypairs
sender = Keypair.generate()
receiver = Keypair.generate()
# Create a transaction
transaction = Transaction()
instruction = TransactionInstruction(
keys=[
AccountMeta(pubkey=sender.public_key, is_signer=True, is_writable=True),
AccountMeta(pubkey=receiver.public_key, is_signer=False, is_writable=True),
],
program_id=SystemProgram.id(),
data=b"", # Add instruction-specific data here
)
transaction.add(instruction)
# Send the transaction
response = await async_client.send_transaction(transaction, sender)
print("Transaction Response:", response)
6. Interacting with Tokens
Solana’s SPL Token Program enables the creation and management of fungible and non-fungible tokens. Python makes it easy to interact with these tokens:
Create a Token Account
from solana.rpc.api import Client
from spl.token.client import Token
from spl.token.constants import TOKEN_PROGRAM_ID
# Connect to Devnet
client = Client("https://api.devnet.solana.com")
# Create a token
token = Token.create_mint(
client, Keypair.generate(), Keypair.generate().public_key, 9, TOKEN_PROGRAM_ID
)
print("Token Address:", token.pubkey)
7. Using Python for Data Analysis on Solana
Python’s data science capabilities can be leveraged to analyze blockchain data. For example, you can use pandas to analyze token transfer histories:
import pandas as pd
from solana.rpc.api import Client
client = Client("https://api.mainnet-beta.solana.com")
# Fetch token transfer history
transfers = client.get_token_transfer_history("TOKEN_ADDRESS")
df = pd.DataFrame(transfers['result'])
print(df.head())
8. Deploying Smart Contracts with AnchorPy
While Python cannot be used to write Solana smart contracts directly, you can interact with and deploy contracts written in Rust using AnchorPy:
from anchorpy import Program, Provider, Wallet
from solana.rpc.async_api import AsyncClient
# Connect to Devnet
async_client = AsyncClient("https://api.devnet.solana.com")
provider = Provider(async_client, Wallet.local())
# Load an Anchor program
program = Program.load("example.json", provider)
# Interact with the program
response = await program.rpc["initialize"]({"arg": "value"})
print("Program Response:", response)
Challenges and Tips for Python Developers
Challenges:
- Performance Limitations: Python is slower than Rust, making it unsuitable for certain on-chain tasks.
- Learning Curve: Understanding blockchain concepts may require additional effort.
Tips:
- Focus on leveraging Python for off-chain development and prototyping.
- Use Python’s rich ecosystem for data analysis and backend integration.
- Collaborate with Rust developers to create end-to-end solutions.
Conclusion
Python developers have a significant role to play in the Solana ecosystem. By leveraging tools like solana-py and AnchorPy, you can interact with Solana, build backend services, and analyze blockchain data effectively. While Rust remains the primary language for on-chain development, Python’s versatility ensures that it will continue to be a valuable tool for developers entering the blockchain space.