Inlining and Deoptimization in JavaScript Engines
Inlining and Deoptimization in JavaScript Engines: A Comprehensive Guide Table of Contents Introduction Historical Context The Role of JavaScript Engines What is Inlining? 4.1 Technical Fundamentals 4.2 Inline Caches Deoptimization: The Necessary Evil 5.1 Deoptimization vs. Inlining 5.2 Scenarios for Deoptimization Advanced Implementation Techniques 6.1 Example Scenarios 6.2 Edge Cases Performance Considerations 7.1 Benchmarking Techniques 7.2 Trade-offs in Inlining Real-World Use Cases 8.1 Industry Standard Applications Pitfalls and Debugging Techniques Comparison with Alternative Approaches Conclusion References and Further Reading 1. Introduction In the realm of JavaScript, optimization is paramount, particularly when considering performance-critical applications. Among these optimizations are inlining and deoptimization, mechanisms by which JavaScript engines enhance or retract optimizations for better performance. This article delves deep into what inlining and deoptimization are, how they work, their historical context and performance implications, advanced techniques, real-world applications, and potential pitfalls. 2. Historical Context The evolution of JavaScript engines, particularly Google’s V8, Mozilla’s SpiderMonkey, and Microsoft’s Chakra, marks a shift from interpretation to just-in-time (JIT) compilation. When JavaScript first emerged, engines interpreted code line by line, yielding slow execution speeds. As demand for dynamic applications increased, the need for speed drove engine developers to implement sophisticated strategies such as JIT compilation, inlining, and deoptimization. Key Milestones: 1995: JavaScript introduced in Netscape, interpreted execution. 2008: V8 engine introduced with JIT compilation. 2011: SpiderMonkey uses TraceMonkey, focusing on optimizing hot code paths. These developments laid the groundwork for sophisticated systems that could analyze JavaScript execution patterns to optimize performance dynamically. 3. The Role of JavaScript Engines JavaScript engines are responsible for executing JavaScript code. They utilize a multi-stage approach involving parsing, interpreting, and compiling: Parsing: The engine converts the source code into an Abstract Syntax Tree (AST). Interpreting: JavaScript is initially interpreted, allowing immediate execution while collecting runtime information. JIT Compilation: Code that is executed frequently (hot code) is compiled into machine code for faster execution. JIT compilers employ optimization techniques like inlining and inline caching to enhance performance dynamically. 4. What is Inlining? Inlining is an optimization technique where the actual code of a function is inserted directly at the call site rather than being called via the typical function call mechanism. This minimizes the overhead of function calls and allows the JIT compiler to perform additional optimizations based on the code structure. 4.1 Technical Fundamentals When a function is inlined, its parameters and local variables are available, allowing further optimizations such as constant folding and partial evaluation. Basic Example of Inlining function add(a, b) { return a + b; } function compute(num) { return add(num, 10); } // With inlining optimization // function compute(num) { // return num + 10; // direct function body inserted here // } Inline optimization significantly reduces the overhead associated with function calls. 4.2 Inline Caches Inline caches (ICs) serve as a mechanism to speed up property access and method calls. Instead of re-evaluating the structure of objects every time a property is accessed, inline caches store the last known type and shape of objects, allowing quick access. function getProperty(obj) { return obj.prop; // could be optimized with inline caching } If obj is observed frequently in particular shapes, the JIT compiler can create an inline cache for those shapes. 5. Deoptimization: The Necessary Evil Deoptimization is the process of reverting optimized code back to an interpreted state, due mostly to changing runtime conditions like variable types. 5.1 Deoptimization vs. Inlining Inlining: A performance enhancement, whereby functions are expanded inline. Deoptimization: A necessary fallback when code assumptions change, leading to discrepancies in execution and potentially erroneous behavior if optimizations remain. 5.2 Scenarios for Deoptimization Deoptimization can occur under various circumstances, such as: Type Changes: A variable originally typed as a number may receive a string. Shape Changes: Objects may have their structure modified, affecting inline caches. Example of Deoptimization Trigger let obj = { value: 42 }; function getValue(o) { return o.value; // inline
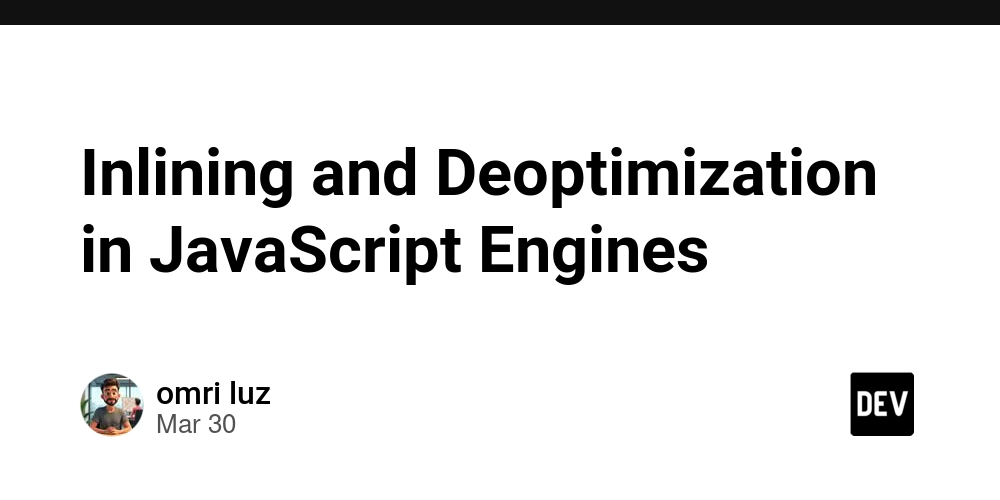
Inlining and Deoptimization in JavaScript Engines: A Comprehensive Guide
Table of Contents
- Introduction
- Historical Context
- The Role of JavaScript Engines
-
What is Inlining?
- 4.1 Technical Fundamentals
- 4.2 Inline Caches
-
Deoptimization: The Necessary Evil
- 5.1 Deoptimization vs. Inlining
- 5.2 Scenarios for Deoptimization
-
Advanced Implementation Techniques
- 6.1 Example Scenarios
- 6.2 Edge Cases
-
Performance Considerations
- 7.1 Benchmarking Techniques
- 7.2 Trade-offs in Inlining
-
Real-World Use Cases
- 8.1 Industry Standard Applications
- Pitfalls and Debugging Techniques
- Comparison with Alternative Approaches
- Conclusion
- References and Further Reading
1. Introduction
In the realm of JavaScript, optimization is paramount, particularly when considering performance-critical applications. Among these optimizations are inlining and deoptimization, mechanisms by which JavaScript engines enhance or retract optimizations for better performance. This article delves deep into what inlining and deoptimization are, how they work, their historical context and performance implications, advanced techniques, real-world applications, and potential pitfalls.
2. Historical Context
The evolution of JavaScript engines, particularly Google’s V8, Mozilla’s SpiderMonkey, and Microsoft’s Chakra, marks a shift from interpretation to just-in-time (JIT) compilation. When JavaScript first emerged, engines interpreted code line by line, yielding slow execution speeds. As demand for dynamic applications increased, the need for speed drove engine developers to implement sophisticated strategies such as JIT compilation, inlining, and deoptimization.
Key Milestones:
- 1995: JavaScript introduced in Netscape, interpreted execution.
- 2008: V8 engine introduced with JIT compilation.
- 2011: SpiderMonkey uses TraceMonkey, focusing on optimizing hot code paths.
These developments laid the groundwork for sophisticated systems that could analyze JavaScript execution patterns to optimize performance dynamically.
3. The Role of JavaScript Engines
JavaScript engines are responsible for executing JavaScript code. They utilize a multi-stage approach involving parsing, interpreting, and compiling:
- Parsing: The engine converts the source code into an Abstract Syntax Tree (AST).
- Interpreting: JavaScript is initially interpreted, allowing immediate execution while collecting runtime information.
- JIT Compilation: Code that is executed frequently (hot code) is compiled into machine code for faster execution.
JIT compilers employ optimization techniques like inlining and inline caching to enhance performance dynamically.
4. What is Inlining?
Inlining is an optimization technique where the actual code of a function is inserted directly at the call site rather than being called via the typical function call mechanism. This minimizes the overhead of function calls and allows the JIT compiler to perform additional optimizations based on the code structure.
4.1 Technical Fundamentals
When a function is inlined, its parameters and local variables are available, allowing further optimizations such as constant folding and partial evaluation.
Basic Example of Inlining
function add(a, b) {
return a + b;
}
function compute(num) {
return add(num, 10);
}
// With inlining optimization
// function compute(num) {
// return num + 10; // direct function body inserted here
// }
Inline optimization significantly reduces the overhead associated with function calls.
4.2 Inline Caches
Inline caches (ICs) serve as a mechanism to speed up property access and method calls. Instead of re-evaluating the structure of objects every time a property is accessed, inline caches store the last known type and shape of objects, allowing quick access.
function getProperty(obj) {
return obj.prop; // could be optimized with inline caching
}
If obj
is observed frequently in particular shapes, the JIT compiler can create an inline cache for those shapes.
5. Deoptimization: The Necessary Evil
Deoptimization is the process of reverting optimized code back to an interpreted state, due mostly to changing runtime conditions like variable types.
5.1 Deoptimization vs. Inlining
- Inlining: A performance enhancement, whereby functions are expanded inline.
- Deoptimization: A necessary fallback when code assumptions change, leading to discrepancies in execution and potentially erroneous behavior if optimizations remain.
5.2 Scenarios for Deoptimization
Deoptimization can occur under various circumstances, such as:
- Type Changes: A variable originally typed as a number may receive a string.
- Shape Changes: Objects may have their structure modified, affecting inline caches.
Example of Deoptimization Trigger
let obj = { value: 42 };
function getValue(o) {
return o.value; // inline cached initially
}
obj.value = 'hello'; // This change will trigger a deoptimization.
6. Advanced Implementation Techniques
6.1 Example Scenarios
Let’s explore an advanced example to illustrate pitfalls and benefits:
Initial Setup with Possible Inlining:
function processEntries(entries) {
let total = 0;
for (let entry of entries) {
total += calculate(entry);
}
return total;
}
function calculate(entry) {
return entry.value * 2; // potential inlining opportunity
}
After Profiling:
// If 'calculate' is heavily used:
function processEntries(entries) {
let total = 0;
for (let entry of entries) {
// Inlining `calculate` here would enhance performance
total += entry.value * 2;
}
return total;
}
6.2 Edge Cases
- Recursion: Inlining can complicate recursive functions, leading to stack overflow if not managed correctly.
function factorial(n) {
if (n === 0) return 1;
return n * factorial(n - 1); // recursive calls may inhibit inlining.
}
- Non-simple Types: Inlining may not occur reliably with functions returning non-simple types (like objects), leading to performance issues.
7. Performance Considerations
7.1 Benchmarking Techniques
- Micro-optimizations: Small function calls often benefit from inlining; benchmark cache hit and miss rates.
- Amazon’s RAIL Model: Focus on response times (16ms per frame) to optimize hot code paths.
7.2 Trade-offs in Inlining
- Memory Footprint: Excessive inlining can blow up memory utilization due to code bloat.
- Increased Compilation Time: While inlining improves runtime, it can slow down the JIT compilation phase.
8. Real-World Use Cases
1. Google Search: Utilizes optimized inlining via V8 engine for rapid query response.
2. Facebook: Uses React, benefiting from function inlining to manage state updates efficiently.
9. Pitfalls and Debugging Techniques
Potential Pitfalls
- Incorrect Type Assumptions: This can cause silent failures since JavaScript is weakly typed.
- Deoptimizations: Can result in unexpected performance regression.
Advanced Debugging Techniques
- Chrome DevTools: Analyze performance where you can view inlined and optimized code.
-
Node.js Inspector: Use the
--inspect
flag to monitor live execution and deoptimization logs.
node --inspect app.js
10. Comparison with Alternative Approaches
Alternative: Precompilation
Some environments use Ahead-Of-Time (AOT) compilation, where the code is compiled before execution, negating inlining complexities. However, it sacrifices the ability to optimize based on runtime type information.
- Pros: Predictable performance, reduced runtime overhead.
- Cons: May be less efficient in environments where the shape and types of code change frequently.
11. Conclusion
Inlining and deoptimization are critical concepts within JavaScript performance optimization. Understanding how JavaScript engines leverage these techniques not only allows developers to write more efficient code but also enables them to anticipate potential pitfalls and performance regressions. By combining insights from historical context, real-world applications, and performance metrics, developers can craft well-optimized JavaScript capable of powering complex web applications in a dynamic environment.
12. References and Further Reading
- V8 JavaScript Engine Documentation: V8 Blog
- MDN Web Docs on JIT compilation: MDN JIT
- "Optimizing JavaScript" - Blog series by Google Developers: Google Developers
By taking a comprehensive approach to inlining and deoptimization, this guide aims to empower senior developers with the knowledge to optimize JavaScript performance considerably.