Important Design Patterns Every Developer Should Know
Design patterns are reusable solutions to common programming challenges that developers face regularly. Understanding these patterns can significantly improve your code organization, flexibility, and maintainability. Let's dive into some of the most crucial design patterns highlighted in the image. Creational Patterns Singleton The Singleton pattern ensures that a class has only one instance while providing global access to it. This pattern is useful when exactly one object is needed to coordinate actions across the system, such as a configuration manager or connection pool. Learn more about Singleton Pattern Factory The Factory pattern provides an interface for creating objects without specifying their concrete classes. This pattern delegates object creation to subclasses, making code more flexible by decoupling the client code from specific class implementations. Learn more about Factory Pattern Abstract Factory Building on the Factory pattern, the Abstract Factory provides an interface for creating families of related objects without specifying their concrete classes. This pattern is particularly useful when your system needs to work with multiple families of products. Learn more about Abstract Factory Pattern Builder The Builder pattern separates the construction of complex objects from their representation. This pattern allows you to create different configurations of an object step by step, making the construction process more readable and manageable. Learn more about Builder Pattern Structural Patterns Adapter The Adapter pattern allows objects with incompatible interfaces to collaborate. It acts as a bridge between two incompatible interfaces, converting the interface of one class into another that clients expect. Learn more about Adapter Pattern Decorator The Decorator pattern lets you attach new behaviors to objects by placing them inside wrapper objects. This pattern provides a flexible alternative to subclassing for extending functionality. Learn more about Decorator Pattern Facade The Facade pattern provides a simplified interface to a complex subsystem. It doesn't hide the subsystem but rather provides a higher-level interface that makes the subsystem easier to use. Learn more about Facade Pattern Composite The Composite pattern lets you compose objects into tree structures to represent part-whole hierarchies. This pattern allows clients to treat individual objects and compositions of objects uniformly. Learn more about Composite Pattern Behavioral Patterns Observer The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This pattern is commonly used in implementing event handling systems. Learn more about Observer Pattern Command The Command pattern turns a request into a stand-alone object containing all information about the request. This transformation allows you to parameterize methods with different requests, delay or queue a request's execution, and support undoable operations. Learn more about Command Pattern Strategy The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern lets the algorithm vary independently from the clients that use it. Learn more about Strategy Pattern State The State pattern allows an object to alter its behavior when its internal state changes. The object will appear to change its class, enabling different behaviors for different states. Learn more about State Pattern Template Method The Template Method pattern defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure. This pattern is particularly useful when you want to standardize an algorithm while allowing for variations in certain steps. Learn more about Template Method Pattern Additional Resources Design Patterns: Elements of Reusable Object-Oriented Software - The original "Gang of Four" book Head First Design Patterns - A more accessible introduction to design patterns SourceMaking Design Patterns - Comprehensive guide with examples Dofactory JavaScript Design Patterns - For JavaScript developers Python Patterns - Design patterns implemented in Python Conclusion These design patterns are essential tools in any developer's toolkit. By understanding and implementing these patterns appropriately, you can create more flexible, reusable, and maintainable code. Remember that design patterns are not one-size-fits-all solutions—they should be applied thoughtfully based on your specific requirements and constraints. Which of these patterns have you used in your projects? Are there any particular patterns you find especially useful or ch
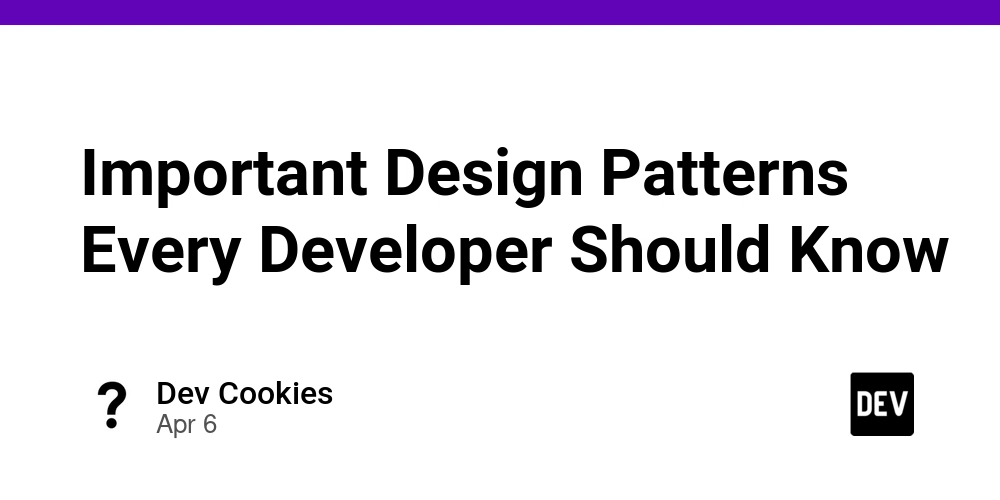
Design patterns are reusable solutions to common programming challenges that developers face regularly. Understanding these patterns can significantly improve your code organization, flexibility, and maintainability. Let's dive into some of the most crucial design patterns highlighted in the image.
Creational Patterns
Singleton
The Singleton pattern ensures that a class has only one instance while providing global access to it. This pattern is useful when exactly one object is needed to coordinate actions across the system, such as a configuration manager or connection pool.
Learn more about Singleton Pattern
Factory
The Factory pattern provides an interface for creating objects without specifying their concrete classes. This pattern delegates object creation to subclasses, making code more flexible by decoupling the client code from specific class implementations.
Learn more about Factory Pattern
Abstract Factory
Building on the Factory pattern, the Abstract Factory provides an interface for creating families of related objects without specifying their concrete classes. This pattern is particularly useful when your system needs to work with multiple families of products.
Learn more about Abstract Factory Pattern
Builder
The Builder pattern separates the construction of complex objects from their representation. This pattern allows you to create different configurations of an object step by step, making the construction process more readable and manageable.
Learn more about Builder Pattern
Structural Patterns
Adapter
The Adapter pattern allows objects with incompatible interfaces to collaborate. It acts as a bridge between two incompatible interfaces, converting the interface of one class into another that clients expect.
Learn more about Adapter Pattern
Decorator
The Decorator pattern lets you attach new behaviors to objects by placing them inside wrapper objects. This pattern provides a flexible alternative to subclassing for extending functionality.
Learn more about Decorator Pattern
Facade
The Facade pattern provides a simplified interface to a complex subsystem. It doesn't hide the subsystem but rather provides a higher-level interface that makes the subsystem easier to use.
Learn more about Facade Pattern
Composite
The Composite pattern lets you compose objects into tree structures to represent part-whole hierarchies. This pattern allows clients to treat individual objects and compositions of objects uniformly.
Learn more about Composite Pattern
Behavioral Patterns
Observer
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This pattern is commonly used in implementing event handling systems.
Learn more about Observer Pattern
Command
The Command pattern turns a request into a stand-alone object containing all information about the request. This transformation allows you to parameterize methods with different requests, delay or queue a request's execution, and support undoable operations.
Learn more about Command Pattern
Strategy
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern lets the algorithm vary independently from the clients that use it.
Learn more about Strategy Pattern
State
The State pattern allows an object to alter its behavior when its internal state changes. The object will appear to change its class, enabling different behaviors for different states.
Learn more about State Pattern
Template Method
The Template Method pattern defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure. This pattern is particularly useful when you want to standardize an algorithm while allowing for variations in certain steps.
Learn more about Template Method Pattern
Additional Resources
- Design Patterns: Elements of Reusable Object-Oriented Software - The original "Gang of Four" book
- Head First Design Patterns - A more accessible introduction to design patterns
- SourceMaking Design Patterns - Comprehensive guide with examples
- Dofactory JavaScript Design Patterns - For JavaScript developers
- Python Patterns - Design patterns implemented in Python
Conclusion
These design patterns are essential tools in any developer's toolkit. By understanding and implementing these patterns appropriately, you can create more flexible, reusable, and maintainable code. Remember that design patterns are not one-size-fits-all solutions—they should be applied thoughtfully based on your specific requirements and constraints.
Which of these patterns have you used in your projects? Are there any particular patterns you find especially useful or challenging to implement?