Implementing End-to-End Data Encryption and Data Masking in MongoDB On-Premises with Node.js
MongoDB Enterprise Advanced offers comprehensive security features to protect sensitive data throughout its lifecycle—in transit, at rest, and in use. This guide demonstrates how to implement robust encryption and data masking mechanisms using Client-Side Field-Level Encryption (CSFLE) and Queryable Encryption, specifically for MongoDB on-premises setups with Node.js. MongoDB Encryption Overview 1. Encryption in Transit MongoDB supports TLS/SSL for encrypting communication between clients, servers, and nodes. Requires configuration of TLS certificates on the server and client. 2. Encryption at Rest MongoDB Enterprise Advanced enables encryption at rest with the WiredTiger storage engine, using AES-256 encryption. Supports integration with Key Management Systems (KMS) such as AWS KMS, Azure Key Vault, or a local KMIP-compliant key manager. 3. In-Use Encryption Client-Side Field-Level Encryption (CSFLE): Encrypts sensitive fields on the client side before storage, ensuring the server never has access to plaintext data. Queryable Encryption: Allows performing equality and range queries directly on encrypted data, protecting sensitive information even during query execution. Prerequisites for On-Premises Setup MongoDB Enterprise Advanced installed on your server. Securely configured TLS/SSL certificates for encrypted communication. Integration with a KMIP-compliant key manager for encryption key storage. Node.js 18+ and the MongoDB Node.js driver installed. Install Required Dependencies npm install mongodb mongodb-client-encryption Step 1: Configure Encryption Keys for On-Premises Generate a Local Master Key (Development Only) For production, use a KMIP-compliant key manager. const crypto = require('crypto'); const localMasterKey = crypto.randomBytes(96); Set Up Key Vault and Data Encryption Key (DEK) const { MongoClient } = require('mongodb'); const { ClientEncryption } = require('mongodb-client-encryption'); const uri = "mongodb://localhost:27017"; const keyVaultNamespace = "encryption.__keyVault"; const kmsProviders = { local: { key: localMasterKey } }; const client = new MongoClient(uri); await client.connect(); const encryption = new ClientEncryption(client, { keyVaultNamespace, kmsProviders }); const dekId = await encryption.createDataKey('local'); console.log('Data Encryption Key ID:', dekId.toString('base64')); Step 2: Define Field-Level Encryption Schema Create a JSON schema to specify which fields should be encrypted: const schemaMap = { "mydb.users": { bsonType: "object", encryptMetadata: { keyId: [dekId] }, properties: { ssn: { encrypt: { bsonType: "string", algorithm: "AEAD_AES_256_CBC_HMAC_SHA_512-Deterministic" } }, salary: { encrypt: { bsonType: "double", algorithm: "AEAD_AES_256_CBC_HMAC_SHA_512-Random" } } } } }; Step 3: Enable Auto-Encryption in MongoDB Configure mongocryptd For Queryable Encryption and CSFLE, you need to enable mongocryptd, a lightweight service for encryption operations. Ensure mongocryptd is installed and running: mongocryptd --logpath /var/log/mongocryptd.log --fork Connect with Auto-Encryption Configured const secureClient = new MongoClient(uri, { autoEncryption: { keyVaultNamespace, kmsProviders, schemaMap } }); await secureClient.connect(); Step 4: Insert and Query Encrypted Data Insert Encrypted Document const users = secureClient.db('mydb').collection('users'); await users.insertOne({ name: "John Doe", ssn: "123-45-6789", salary: 85000 }); Query Encrypted Fields Deterministic encryption allows equality searches: const user = await users.findOne({ ssn: "123-45-6789" }); console.log(user); Step 5: Configure and Use Queryable Encryption Queryable Encryption enables encrypted range queries in MongoDB Enterprise Advanced. Create Collection with Queryable Encryption const encryptedFields = { fields: [ { path: "salary", keyId: dekId, bsonType: "double", queries: { queryType: "range", contention: 0 } } ] }; await secureClient.db('mydb').createCollection("employees", { encryptedFields }); Insert and Query Encrypted Data const employees = secureClient.db('mydb').collection('employees'); await employees.insertMany([ { name: "Alice Johnson", salary: 95000 }, { name: "Bob Smith", salary: 120000 }, { name: "Carol White", salary: 78000 } ]); const highEarners = await employees.find({ salary: { $gt: 90000 } }).toArray(); console.log("Employees with salary > 90,000:", highEarners); Step 6: Implement Data Masking Data masking ensures sensitive fields a
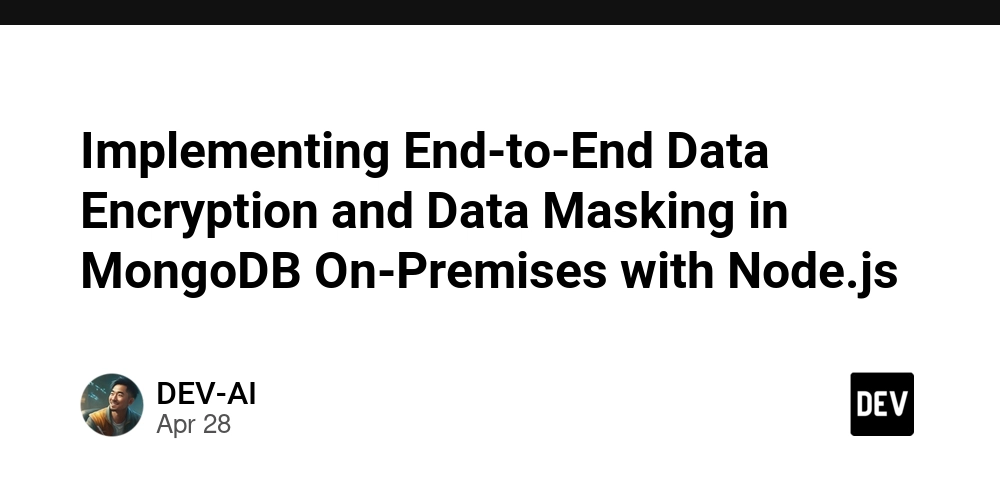
MongoDB Enterprise Advanced offers comprehensive security features to protect sensitive data throughout its lifecycle—in transit, at rest, and in use. This guide demonstrates how to implement robust encryption and data masking mechanisms using Client-Side Field-Level Encryption (CSFLE) and Queryable Encryption, specifically for MongoDB on-premises setups with Node.js.
MongoDB Encryption Overview
1. Encryption in Transit
- MongoDB supports TLS/SSL for encrypting communication between clients, servers, and nodes.
- Requires configuration of TLS certificates on the server and client.
2. Encryption at Rest
- MongoDB Enterprise Advanced enables encryption at rest with the WiredTiger storage engine, using AES-256 encryption.
- Supports integration with Key Management Systems (KMS) such as AWS KMS, Azure Key Vault, or a local KMIP-compliant key manager.
3. In-Use Encryption
- Client-Side Field-Level Encryption (CSFLE): Encrypts sensitive fields on the client side before storage, ensuring the server never has access to plaintext data.
- Queryable Encryption: Allows performing equality and range queries directly on encrypted data, protecting sensitive information even during query execution.
Prerequisites for On-Premises Setup
- MongoDB Enterprise Advanced installed on your server.
- Securely configured TLS/SSL certificates for encrypted communication.
- Integration with a KMIP-compliant key manager for encryption key storage.
- Node.js 18+ and the MongoDB Node.js driver installed.
Install Required Dependencies
npm install mongodb mongodb-client-encryption
Step 1: Configure Encryption Keys for On-Premises
Generate a Local Master Key (Development Only)
For production, use a KMIP-compliant key manager.
const crypto = require('crypto');
const localMasterKey = crypto.randomBytes(96);
Set Up Key Vault and Data Encryption Key (DEK)
const { MongoClient } = require('mongodb');
const { ClientEncryption } = require('mongodb-client-encryption');
const uri = "mongodb://localhost:27017";
const keyVaultNamespace = "encryption.__keyVault";
const kmsProviders = {
local: { key: localMasterKey }
};
const client = new MongoClient(uri);
await client.connect();
const encryption = new ClientEncryption(client, { keyVaultNamespace, kmsProviders });
const dekId = await encryption.createDataKey('local');
console.log('Data Encryption Key ID:', dekId.toString('base64'));
Step 2: Define Field-Level Encryption Schema
Create a JSON schema to specify which fields should be encrypted:
const schemaMap = {
"mydb.users": {
bsonType: "object",
encryptMetadata: { keyId: [dekId] },
properties: {
ssn: {
encrypt: {
bsonType: "string",
algorithm: "AEAD_AES_256_CBC_HMAC_SHA_512-Deterministic"
}
},
salary: {
encrypt: {
bsonType: "double",
algorithm: "AEAD_AES_256_CBC_HMAC_SHA_512-Random"
}
}
}
}
};
Step 3: Enable Auto-Encryption in MongoDB
Configure mongocryptd
For Queryable Encryption and CSFLE, you need to enable mongocryptd
, a lightweight service for encryption operations. Ensure mongocryptd
is installed and running:
mongocryptd --logpath /var/log/mongocryptd.log --fork
Connect with Auto-Encryption Configured
const secureClient = new MongoClient(uri, {
autoEncryption: {
keyVaultNamespace,
kmsProviders,
schemaMap
}
});
await secureClient.connect();
Step 4: Insert and Query Encrypted Data
Insert Encrypted Document
const users = secureClient.db('mydb').collection('users');
await users.insertOne({
name: "John Doe",
ssn: "123-45-6789",
salary: 85000
});
Query Encrypted Fields
Deterministic encryption allows equality searches:
const user = await users.findOne({ ssn: "123-45-6789" });
console.log(user);
Step 5: Configure and Use Queryable Encryption
Queryable Encryption enables encrypted range queries in MongoDB Enterprise Advanced.
Create Collection with Queryable Encryption
const encryptedFields = {
fields: [
{
path: "salary",
keyId: dekId,
bsonType: "double",
queries: { queryType: "range", contention: 0 }
}
]
};
await secureClient.db('mydb').createCollection("employees", { encryptedFields });
Insert and Query Encrypted Data
const employees = secureClient.db('mydb').collection('employees');
await employees.insertMany([
{ name: "Alice Johnson", salary: 95000 },
{ name: "Bob Smith", salary: 120000 },
{ name: "Carol White", salary: 78000 }
]);
const highEarners = await employees.find({ salary: { $gt: 90000 } }).toArray();
console.log("Employees with salary > 90,000:", highEarners);
Step 6: Implement Data Masking
Data masking ensures sensitive fields are displayed partially or obfuscated in application logs or interfaces.
Example: Mask SSN Numbers
function maskSSN(ssn) {
return ssn.replace(/^(\d{3})-\d{2}-(\d{4})$/, "$1-XX-$2");
}
// Usage example:
const maskedSSN = maskSSN("123-45-6789");
console.log(maskedSSN); // Outputs "123-XX-6789"
Integrate Masking into Query Results
const fetchedUser = await users.findOne({ name: "John Doe" });
fetchedUser.ssn = maskSSN(fetchedUser.ssn);
console.log(fetchedUser);
// { name: 'John Doe', ssn: '123-XX-6789', salary: 85000 }
Step 7: Best Practices for On-Premises Security
- Key Management: Use a KMIP-compliant key manager instead of local keys.
- TLS/SSL Configuration: Set up mutual TLS for secure connections between clients and servers.
- Regular Auditing: Enable MongoDB's auditing feature to monitor database access and modifications.
- Encrypt Sensitive Fields Only: Optimize performance by encrypting only necessary fields (e.g., PII, PHI).
- Queryable Encryption: Use for advanced queries on encrypted fields without exposing data.
Further Resources and Documentation
- MongoDB Security Capabilities
- Queryable Encryption Documentation
- MongoDB Enterprise Advanced Security
- MongoDB Cryptography Research Group
Conclusion
By leveraging TLS encryption, AES-256 encryption at rest, and Client-Side Field-Level Encryption (CSFLE), MongoDB Enterprise Advanced ensures robust data protection for on-premises deployments. Queryable Encryption further enhances security by enabling expressive queries on encrypted data. Following these steps and best practices will help you build secure, compliant, and performance-focused applications with MongoDB and Node.js.