How to Use Laravel's API Resource Collections for Efficient Data Handling
How to Use Laravel's API Resource Collections for Efficient Data Handling Laravel's API Resource Collections are a powerful feature that allows developers to transform and structure large sets of data efficiently for API responses. Resource Collections are designed to work seamlessly with Eloquent models, making it easier to format data for JSON responses. In this guide, we will walk through the process of using API Resource Collections for efficient data handling in Laravel. What Are API Resources and Collections? Before diving into collections, let's understand what Laravel API Resources are. API Resources in Laravel are a way to format your data when sending it as JSON. They allow you to control what data is returned and how it is structured. Resource Collections are used when dealing with multiple records, such as a list of users, products, or posts. They help you apply consistent formatting to large sets of data efficiently. Laravel provides these out-of-the-box, allowing you to customize the output of your API in a clean and reusable way. Why Use API Resource Collections? Using Resource Collections in Laravel offers several advantages: Consistency: Ensures consistent data structures across your API responses. Separation of Concerns: Keeps data transformation logic out of your controllers. Efficient Handling: Optimizes data handling, especially for large datasets, by allowing lazy loading and pagination. Customizable: Enables developers to add custom metadata, links, or relationships to the data without mixing this logic into the controller. Creating a Resource Collection To demonstrate how to use Resource Collections, let's assume you have a Post model with related comments and author information. Step 1: Create an API Resource First, let's create an API resource for individual posts. Run the following Artisan command: php artisan make:resource PostResource This command will generate a PostResource class under app/Http/Resources/PostResource.php. Here is what the basic PostResource class might look like:
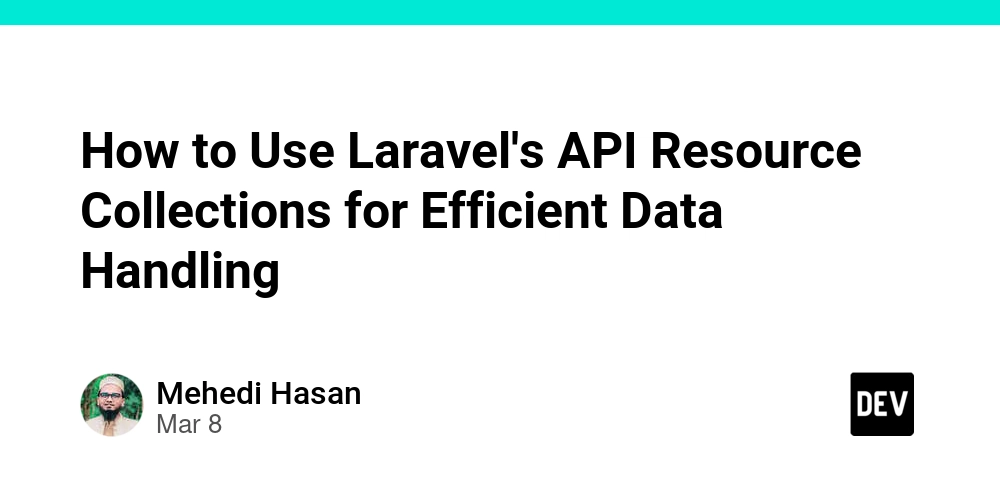
How to Use Laravel's API Resource Collections for Efficient Data Handling
Laravel's API Resource Collections are a powerful feature that allows developers to transform and structure large sets of data efficiently for API responses. Resource Collections are designed to work seamlessly with Eloquent models, making it easier to format data for JSON responses. In this guide, we will walk through the process of using API Resource Collections for efficient data handling in Laravel.
What Are API Resources and Collections?
Before diving into collections, let's understand what Laravel API Resources are.
- API Resources in Laravel are a way to format your data when sending it as JSON. They allow you to control what data is returned and how it is structured.
- Resource Collections are used when dealing with multiple records, such as a list of users, products, or posts. They help you apply consistent formatting to large sets of data efficiently.
Laravel provides these out-of-the-box, allowing you to customize the output of your API in a clean and reusable way.
Why Use API Resource Collections?
Using Resource Collections in Laravel offers several advantages:
- Consistency: Ensures consistent data structures across your API responses.
- Separation of Concerns: Keeps data transformation logic out of your controllers.
- Efficient Handling: Optimizes data handling, especially for large datasets, by allowing lazy loading and pagination.
- Customizable: Enables developers to add custom metadata, links, or relationships to the data without mixing this logic into the controller.
Creating a Resource Collection
To demonstrate how to use Resource Collections, let's assume you have a Post
model with related comments
and author
information.
Step 1: Create an API Resource
First, let's create an API resource for individual posts.
Run the following Artisan command:
php artisan make:resource PostResource
This command will generate a PostResource
class under app/Http/Resources/PostResource.php
.
Here is what the basic PostResource
class might look like:
namespace App\Http\Resources;
use Illuminate\Http\Request;
use Illuminate\Http\Resources\Json\JsonResource;
class PostResource extends JsonResource
{
/**
* Transform the resource into an array.
*
* @param \Illuminate\Http\Request $request
* @return array
*/
public function toArray($request)
{
return [
'id' => $this->id,
'title' => $this->title,
'body' => $this->body,
'author' => $this->author->name, // assuming there is an author relationship
'created_at' => $this->created_at->toDateString(),
'updated_at' => $this->updated_at->toDateString(),
];
}
}
In this example, we format the Post
model by extracting only the necessary fields (id
, title
, body
, etc.), which can also include related data like the author
.
Step 2: Create a Resource Collection
Next, we’ll create a Resource Collection that can handle multiple Post
resources. Use the following Artisan command:
php artisan make:resource PostCollection
This will create a PostCollection
class in the same directory as PostResource
.
Here’s an example of how you can structure it:
namespace App\Http\Resources;
use Illuminate\Http\Request;
use Illuminate\Http\Resources\Json\ResourceCollection;
class PostCollection extends ResourceCollection
{
/**
* Transform the resource collection into an array.
*
* @param \Illuminate\Http\Request $request
* @return array
*/
public function toArray($request)
{
return [
'data' => $this->collection->map(function ($post) {
return new PostResource($post); // Use PostResource for individual transformation
}),
'meta' => [
'total_posts' => $this->collection->count(),
'custom_info' => 'This is a custom message',
],
];
}
}
In this collection, the toArray
method applies the PostResource
to each item in the collection and adds a meta
section for custom data like the total number of posts or other useful information.
Step 3: Using the Resource Collection in the Controller
Now, let’s modify the controller to use this Resource Collection.
For example, in the PostController
:
namespace App\Http\Controllers;
use App\Http\Resources\PostCollection;
use App\Models\Post;
class PostController extends Controller
{
public function index()
{
$posts = Post::with('author')->paginate(10); // Assuming you're paginating the posts
return new PostCollection($posts);
}
}
In this example, the index
method retrieves a paginated list of posts and passes it to the PostCollection
, which formats the response using the PostResource
.
Step 4: Customizing Resource Collection Responses
You can also include additional information like pagination links and metadata directly in your Resource Collection. Laravel makes this simple.
Let’s modify our PostCollection
to include pagination:
public function toArray($request)
{
return [
'data' => $this->collection->map(function ($post) {
return new PostResource($post);
}),
'meta' => [
'total_posts' => $this->total(),
'per_page' => $this->perPage(),
'current_page' => $this->currentPage(),
'last_page' => $this->lastPage(),
],
'links' => [
'first' => $this->url(1),
'last' => $this->url($this->lastPage()),
'prev' => $this->previousPageUrl(),
'next' => $this->nextPageUrl(),
],
];
}
This structure provides detailed pagination metadata and links, which are especially useful for frontend applications that consume the API.
Step 5: Returning Clean and Efficient API Responses
Now that you’ve set up your Resource Collection and integrated it into your controller, your API will return clean and consistent responses, even when handling large datasets.
Here’s an example of how the JSON response might look:
{
"data": [
{
"id": 1,
"title": "First Post",
"body": "This is the body of the first post.",
"author": "John Doe",
"created_at": "2023-03-01",
"updated_at": "2023-03-02"
},
{
"id": 2,
"title": "Second Post",
"body": "This is the body of the second post.",
"author": "Jane Smith",
"created_at": "2023-03-01",
"updated_at": "2023-03-02"
}
],
"meta": {
"total_posts": 2,
"per_page": 10,
"current_page": 1,
"last_page": 1
},
"links": {
"first": "http://example.com?page=1",
"last": "http://example.com?page=1",
"prev": null,
"next": null
}
}
This provides an organized and informative response for clients, making it easier to work with the data.
Conclusion
Using Laravel's API Resource Collections simplifies data handling, ensuring that your API responses are efficient, consistent, and easy to manage. By leveraging resource collections and resources, you can cleanly separate data transformation logic from your controllers while adding flexibility to handle metadata, pagination, and more.
Resource Collections are essential for scaling Laravel APIs and delivering structured responses, which are crucial when building large applications or exposing endpoints for third-party developers.