Why Doesn't Anyone Want to Write Code comments Anymore?
Comments, second only to "Hello, world!" in programming, are usually the next thing you learn after greeting the world. They typically come in two forms: single-line comments and multi-line comments, such as // and /* */. Their purpose is to explain the current code logic and provide annotations. What Are Comments? Before diving into the question "Why Doesn't Anyone Want to Write Code comments Anymore?", let's first look at how people actually write comments. For example, some projects or coding standards require declaring copyright information at the beginning of a file. This could include ASCII art or licensing details: /* * Copyright 2024-2025 the original author or authors. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * https://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ Some even include ASCII art: /* * _oo0oo_ * o8888888o * 88" . "88 * (| -_- |) * 0\ = /0 * ___/`---'\___ * .' \\| |// '. * / \\||| : |||// \ * / _||||| -:- |||||- \ * | | \\\ - /// | | * | \_| ''\---/'' |_/ | * \ .-\__ '-' ___/-. / * ___'. .' /--.--\ `. .'___ * ."" '< `.___\__/___.' >' "". * | | : `- \`.;`\ _ /`;.`/ - ` : | | * \ \ `_. \_ __\ /__ _/ .-` / / * =====`-.____`.___ \_____/___.-`___.-'===== * `=---=' * * ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * * Buddha bless, never crashes, never bugs */ When working at a company, it's common to declare the author of a function. If the function has issues, it's easier to find the person responsible (to take the blame): /** * Title base agent. * Description base agent. * * @author yuanci.ytb * @since 1.0.0-M2 */ Some IDEs even generate comment formats for you automatically, such as Laravel (or Java’s Spring framework): /** * Create a new repository builder instance. * * @param \Dotenv\Repository\Adapter\ReaderInterface[] $readers * @param \Dotenv\Repository\Adapter\WriterInterface[] $writers * @param bool $immutable * @param string[]|null $allowList * * @return void */ private function __construct(array $readers = [], array $writers = [], bool $immutable = false, ?array $allowList = null) { $this->readers = $readers; $this->writers = $writers; $this->immutable = $immutable; $this->allowList = $allowList; } Sometimes, comments are used to disable old functionality: { // image } { /* {isValidImageIcon ? : (icon && icon !== '') ? : } */ } So Why Doesn't Anyone Want to Write Code comments Anymore? Comments generally fall into two categories: one explains information, and the other annotates functionality. Consider the following code without comments—it takes time to understand what it does: function lengthOfLongestSubstring(s) { let charIndexMap = new Map(), longest = 0, left = 0; for (let right = 0; right = left) { left = charIndexMap.get(s[right]) + 1; } charIndexMap.set(s[right], right); longest = Math.max(longest, right - left + 1); } return longest; } Adding comments makes it easier to understand: // Computes the length of the longest substring without repeating characters function lengthOfLongestSubstring(s) { let charIndexMap = new Map(), longest = 0, left = 0; for (let right = 0; right = left pointer, move left pointer if (charIndexMap.has(s[right]) && charIndexMap.get(s[right]) >= left) { left = charIndexMap.get(s[right]) + 1; } // Update the character's latest index in the map charIndexMap.set(s[right], right); // Update the longest substring length longest = Math.max(longest, right - left + 1); } return longest; } People would rather spend five minutes sorting out the logic later than take the time to write comments when inspiration strikes. I think it's largely because of the fear of the following situations: Afraid someone might say: "Isn't it just a calculation logic for finding the length of the longest substring without repeating characters? I could do that in elementary school. You wrote a comment for this? You're so weak." "This person writes so many comments, th
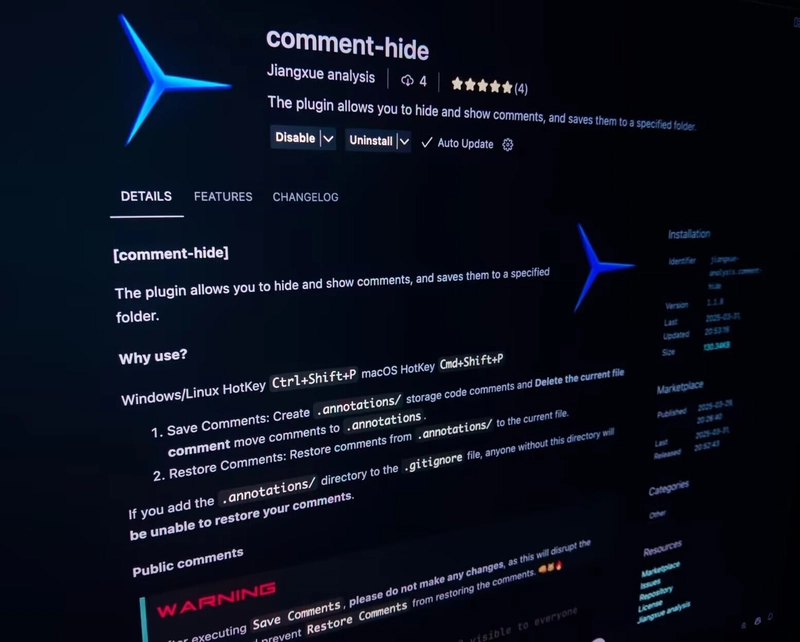
Comments, second only to "Hello, world!" in programming, are usually the next thing you learn after greeting the world. They typically come in two forms: single-line comments and multi-line comments, such as //
and /* */
. Their purpose is to explain the current code logic and provide annotations.
What Are Comments?
Before diving into the question "Why Doesn't Anyone Want to Write Code comments Anymore?", let's first look at how people actually write comments.
For example, some projects or coding standards require declaring copyright information at the beginning of a file. This could include ASCII art or licensing details:
/*
* Copyright 2024-2025 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
Some even include ASCII art:
/*
* _oo0oo_
* o8888888o
* 88" . "88
* (| -_- |)
* 0\ = /0
* ___/`---'\___
* .' \\| |// '.
* / \\||| : |||// \
* / _||||| -:- |||||- \
* | | \\\ - /// | |
* | \_| ''\---/'' |_/ |
* \ .-\__ '-' ___/-. /
* ___'. .' /--.--\ `. .'___
* ."" '< `.___\_<|>_/___.' >' "".
* | | : `- \`.;`\ _ /`;.`/ - ` : | |
* \ \ `_. \_ __\ /__ _/ .-` / /
* =====`-.____`.___ \_____/___.-`___.-'=====
* `=---='
*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*
* Buddha bless, never crashes, never bugs
*/
When working at a company, it's common to declare the author of a function. If the function has issues, it's easier to find the person responsible (to take the blame):
/**
* Title base agent.
* Description base agent.
*
* @author yuanci.ytb
* @since 1.0.0-M2
*/
Some IDEs even generate comment formats for you automatically, such as Laravel (or Java’s Spring framework):
/**
* Create a new repository builder instance.
*
* @param \Dotenv\Repository\Adapter\ReaderInterface[] $readers
* @param \Dotenv\Repository\Adapter\WriterInterface[] $writers
* @param bool $immutable
* @param string[]|null $allowList
*
* @return void
*/
private function __construct(array $readers = [], array $writers = [], bool $immutable = false, ?array $allowList = null)
{
$this->readers = $readers;
$this->writers = $writers;
$this->immutable = $immutable;
$this->allowList = $allowList;
}
Sometimes, comments are used to disable old functionality:
{
// image
}
{
/* {isValidImageIcon
?
: (icon && icon !== '') ? :