How to Resolve Firebase Initialization Issues in Dart for Web and Android?
In a multi-platform environment, you may encounter issues when initializing Firebase in Dart, particularly when targeting both web and Android. A common concern is ensuring that your Firebase configuration seamlessly transitions between these platforms. In this article, we will explore the nuances of Firebase initialization in Dart and why certain configurations work for one platform but not the other. Understanding Firebase Initialization Issues When developing applications for both Android and web using Flutter, it's crucial to initialize Firebase correctly. The initialization process requires platform-specific configurations. When you use FirebaseOptions() for web, it requires specific parameters: apiKey, appId, messagingSenderId, and projectId. However, when you comment out this configuration for Android, an error may arise indicating that a Firebase app named "[default]" already exists. Common Errors Encountered Error on Android: "A Firebase app named '[default]' already exists" This error typically indicates that the Firebase app is being initialized more than once. In Android, Firebase automatically initializes using the configuration found in google-services.json, which means you don't need to provide FirebaseOptions(). Error on Web: "FirebaseOptions cannot be null when creating the default app" For web applications, not providing the necessary FirebaseOptions will lead to failures, as the web version requires explicit configuration to set up the Firebase app correctly. Solutions for Firebase Initialization in Dart To resolve these initialization issues, you can use separate configurations based on the platform. Below are steps detailing how to implement this. Step 1: Check Platform Use Flutter's built-in support for checking the platform to decide which initialization method to use. import 'dart:io' show Platform; import 'package:firebase_core/firebase_core.dart'; class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'My App', home: HomePage(), ); } } void main() async { WidgetsFlutterBinding.ensureInitialized(); if (kIsWeb) { await Firebase.initializeApp( options: FirebaseOptions( apiKey: "---", appId: "---", messagingSenderId: "---", projectId: "---", ), ); } else { await Firebase.initializeApp(); } runApp(const MyApp()); } Explanation of the Solution In the code snippet above, we first check if the application is running on the web using kIsWeb. If it is indeed a web application, we pass FirebaseOptions() with the required parameters to the initializeApp method. For Android, we simply call Firebase.initializeApp() without any parameters, relying on the configuration defined in the native google-services.json file. Step 2: Testing the Implementation Make sure to test this implementation thoroughly: Run the app in a web browser to ensure it initializes Firebase correctly. Run the app on an Android emulator or device to confirm there are no initialization issues. Frequently Asked Questions Q1: Why does Firebase require different options for web and Android? A1: Firebase uses different initialization mechanisms for web and mobile platforms. Web requires explicit configuration through FirebaseOptions, while Android uses a configuration file. Q2: What if I still encounter issues after implementing this code? A2: Ensure that you're using the latest versions of the Firebase packages and that your google-services.json and web app configurations are correct. Q3: Can I use this approach for other platforms like iOS? A3: Yes, you can extend this approach by adding additional platform checks for iOS, using similar logic for initialization based on expected behavior on that platform. In conclusion, initializing Firebase in a Flutter application for both web and Android requires understanding the specific requirements of each platform. By following the outlined steps, you can seamlessly manage Firebase initialization across different environments, preventing common issues and ensuring a smooth development experience.
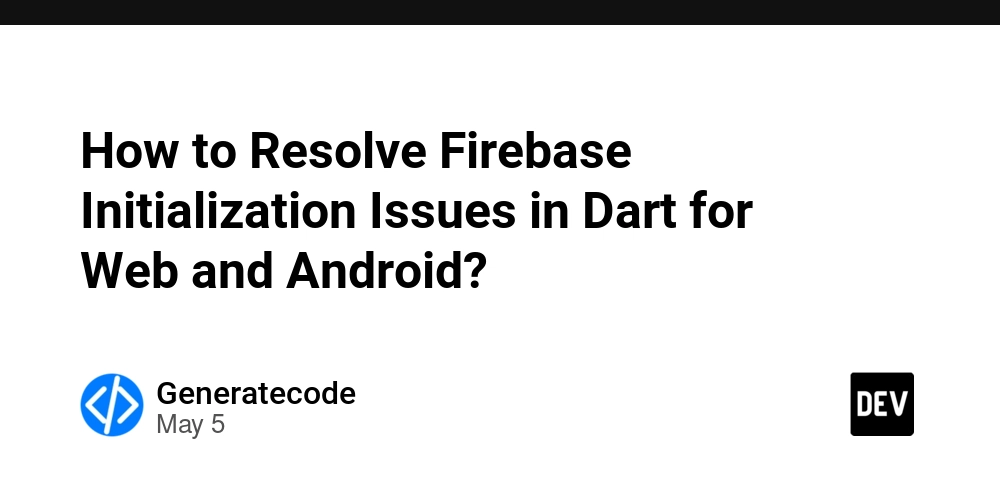
In a multi-platform environment, you may encounter issues when initializing Firebase in Dart, particularly when targeting both web and Android. A common concern is ensuring that your Firebase configuration seamlessly transitions between these platforms. In this article, we will explore the nuances of Firebase initialization in Dart and why certain configurations work for one platform but not the other.
Understanding Firebase Initialization Issues
When developing applications for both Android and web using Flutter, it's crucial to initialize Firebase correctly. The initialization process requires platform-specific configurations. When you use FirebaseOptions()
for web, it requires specific parameters: apiKey, appId, messagingSenderId, and projectId. However, when you comment out this configuration for Android, an error may arise indicating that a Firebase app named "[default]" already exists.
Common Errors Encountered
Error on Android:
-
"A Firebase app named '[default]' already exists"
This error typically indicates that the Firebase app is being initialized more than once. In Android, Firebase automatically initializes using the configuration found in
google-services.json
, which means you don't need to provideFirebaseOptions()
.
Error on Web:
-
"FirebaseOptions cannot be null when creating the default app"
For web applications, not providing the necessary
FirebaseOptions
will lead to failures, as the web version requires explicit configuration to set up the Firebase app correctly.
Solutions for Firebase Initialization in Dart
To resolve these initialization issues, you can use separate configurations based on the platform. Below are steps detailing how to implement this.
Step 1: Check Platform
Use Flutter's built-in support for checking the platform to decide which initialization method to use.
import 'dart:io' show Platform;
import 'package:firebase_core/firebase_core.dart';
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'My App',
home: HomePage(),
);
}
}
void main() async {
WidgetsFlutterBinding.ensureInitialized();
if (kIsWeb) {
await Firebase.initializeApp(
options: FirebaseOptions(
apiKey: "---",
appId: "---",
messagingSenderId: "---",
projectId: "---",
),
);
} else {
await Firebase.initializeApp();
}
runApp(const MyApp());
}
Explanation of the Solution
In the code snippet above, we first check if the application is running on the web using kIsWeb
. If it is indeed a web application, we pass FirebaseOptions()
with the required parameters to the initializeApp
method. For Android, we simply call Firebase.initializeApp()
without any parameters, relying on the configuration defined in the native google-services.json
file.
Step 2: Testing the Implementation
Make sure to test this implementation thoroughly:
- Run the app in a web browser to ensure it initializes Firebase correctly.
- Run the app on an Android emulator or device to confirm there are no initialization issues.
Frequently Asked Questions
Q1: Why does Firebase require different options for web and Android?
A1: Firebase uses different initialization mechanisms for web and mobile platforms. Web requires explicit configuration through FirebaseOptions
, while Android uses a configuration file.
Q2: What if I still encounter issues after implementing this code?
A2: Ensure that you're using the latest versions of the Firebase packages and that your google-services.json
and web app configurations are correct.
Q3: Can I use this approach for other platforms like iOS?
A3: Yes, you can extend this approach by adding additional platform checks for iOS, using similar logic for initialization based on expected behavior on that platform.
In conclusion, initializing Firebase in a Flutter application for both web and Android requires understanding the specific requirements of each platform. By following the outlined steps, you can seamlessly manage Firebase initialization across different environments, preventing common issues and ensuring a smooth development experience.