How to Protect Your Web3 Project from Smart Contract Hacks
Introduction Smart contract vulnerabilities have led to billions of dollars in losses due to hacks, exploits, and scams. Reentrancy attacks, flash loan exploits, and private key leaks are some of the most common threats in Web3. If you’re building a decentralized application (dApp), securing your smart contracts is critical. What You’ll Learn ✔ Common smart contract vulnerabilities ✔ Best security practices for Ethereum and EVM-based blockchains ✔ How to audit and test smart contracts ✔ Tools to secure Web3 projects Common Smart Contract Vulnerabilities 1.1 Reentrancy Attacks What happens? A malicious contract repeatedly calls a function before the first execution is completed. Example: The DAO Hack (2016) resulted in a $60M loss. Prevention: Use ReentrancyGuard from OpenZeppelin. import "@openzeppelin/contracts/security/ReentrancyGuard.sol"; contract SecureContract is ReentrancyGuard { mapping(address => uint256) balances; function withdraw(uint256 _amount) external nonReentrant { require(balances[msg.sender] >= _amount, "Insufficient balance"); balances[msg.sender] -= _amount; payable(msg.sender).transfer(_amount); } } 1.2 Integer Overflow & Underflow What happens? Values exceed the data type limit, causing unintended behavior. Example: Many older Solidity versions had this issue. Prevention: Use SafeMath from OpenZeppelin or Solidity 0.8+ (which has built-in overflow checks). uint256 public totalSupply = 1000; function burn(uint256 amount) public { require(amount uint256) lastWithdrawal; modifier rateLimit() { require(block.timestamp >= lastWithdrawal[msg.sender] + 1 days, "Wait before withdrawing again"); _; } 2.4 Store Data Off-Chain When Possible Prevents sensitive data leaks. Use IPFS, Arweave, or Filecoin instead of storing everything on-chain. 2.5 Follow Gas Optimization Techniques Gas-intensive contracts attract DoS (denial-of-service) attacks. Use Solidity best practices to optimize contract performance. Example: ✅ Use fixed-size arrays instead of dynamic arrays ✅ Replace for loops with mapping structures mapping(address => uint256) balances; Auditing and Testing Smart Contracts 3.1 Use Static Analysis Tools
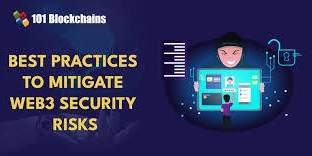
Introduction
Smart contract vulnerabilities have led to billions of dollars in losses due to hacks, exploits, and scams. Reentrancy attacks, flash loan exploits, and private key leaks are some of the most common threats in Web3. If you’re building a decentralized application (dApp), securing your smart contracts is critical.
What You’ll Learn
✔ Common smart contract vulnerabilities
✔ Best security practices for Ethereum and EVM-based blockchains
✔ How to audit and test smart contracts
✔ Tools to secure Web3 projects
- Common Smart Contract Vulnerabilities
1.1 Reentrancy Attacks
What happens? A malicious contract repeatedly calls a function before the first execution is completed.
Example: The DAO Hack (2016) resulted in a $60M loss.
Prevention: Use ReentrancyGuard from OpenZeppelin.
import "@openzeppelin/contracts/security/ReentrancyGuard.sol";
contract SecureContract is ReentrancyGuard {
mapping(address => uint256) balances;
function withdraw(uint256 _amount) external nonReentrant {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
payable(msg.sender).transfer(_amount);
}
}
1.2 Integer Overflow & Underflow
What happens? Values exceed the data type limit, causing unintended behavior.
Example: Many older Solidity versions had this issue.
Prevention: Use SafeMath from OpenZeppelin or Solidity 0.8+ (which has built-in overflow checks).
uint256 public totalSupply = 1000;
function burn(uint256 amount) public {
require(amount <= totalSupply, "Overflow risk");
totalSupply -= amount;
}
1.3 Flash Loan Attacks
What happens? Attackers borrow large amounts of crypto without collateral and manipulate prices.
Example: The bZx hack led to a $55M exploit.
Prevention: Use price oracles like Chainlink instead of manipulating internal price feeds.
uint256 price = uint256(ChainlinkOracle.latestAnswer());
1.4 Private Key Leaks
What happens? Developers hardcode private keys in smart contracts or repositories.
Example: Multiple hacks occur due to GitHub key leaks.
Prevention: Use environment variables and never expose private keys.
PRIVATE_KEY=your_private_key
1.5 Lack of Access Control
What happens? Poorly designed admin functions allow unauthorized users to take control.
Example: The Parity Wallet hack ($150M frozen) was caused by an unprotected admin function.
Prevention: Use modifier-based access control and OpenZeppelin’s Ownable.
import "@openzeppelin/contracts/access/Ownable.sol";
contract SecureContract is Ownable {
function adminOnlyFunction() public onlyOwner {
// Secure logic
}
}
- Best Security Practices for Smart Contracts
2.1 Use Time Locks for Critical Functions
Protects against rug pulls and admin abuse.
Requires a delay before executing high-value transactions.
import "@openzeppelin/contracts/governance/TimelockController.sol";
2.2 Implement Multi-Signature Wallets (Gnosis Safe)
Requires multiple approvals before executing transactions.
Reduces the risk of a single point of failure.
Gnosis Safe is the most trusted multi-signature wallet.
2.3 Use Rate Limits and Circuit Breakers
Limits the amount of withdrawals per transaction.
Prevents flash loan attacks.
mapping(address => uint256) lastWithdrawal;
modifier rateLimit() {
require(block.timestamp >= lastWithdrawal[msg.sender] + 1 days, "Wait before withdrawing again");
_;
}
2.4 Store Data Off-Chain When Possible
Prevents sensitive data leaks.
Use IPFS, Arweave, or Filecoin instead of storing everything on-chain.
2.5 Follow Gas Optimization Techniques
Gas-intensive contracts attract DoS (denial-of-service) attacks.
Use Solidity best practices to optimize contract performance.
Example:
✅ Use fixed-size arrays instead of dynamic arrays
✅ Replace for loops with mapping structures
mapping(address => uint256) balances;
- Auditing and Testing Smart Contracts
3.1 Use Static Analysis Tools