How To Handle Form Data In React JS
Introduction. Handling form data in React JS is a topic that touches every developer who builds interactive web applications. I’ve spent a lot of time working with forms and managing user input, and I know first-hand that forms are the bridge between your users and your app’s logic. In this post, I’ll share my insights, tips, and best practices to help you confidently handle form data in React JS. Why Form Data Matters Forms are everywhere—registration pages, search bars, comment sections, and even complex data entry applications. The way you handle form data affects not only the performance of your app but also the overall user experience. With React JS, handling forms is straightforward once you understand the core concepts. This post is meant to be a friendly guide that walks you through the basic to advanced ideas while keeping things light and accessible. React gives me the flexibility to build powerful interactive applications with clean code, and the way it manages state makes handling form inputs both enjoyable and efficient. The process might seem tricky at first, but with a few simple steps, you’ll be able to build forms that feel responsive and intuitive. Understanding Controlled vs. Uncontrolled Components One of the first things I learned when working with forms in React JS was the concept of controlled and uncontrolled components. Controlled Components: These are the go-to for many developers because they tie the form’s data directly to the component state. Every time a user types into a form input, an event handler updates the state, and the input displays the updated state. This approach gives you complete control over the data and makes it easier to validate user input on the fly. For example, if you want to display a custom error message when a user enters an invalid email, a controlled component lets you do that easily. Uncontrolled Components: In this approach, the form data is handled by the DOM itself. It can be useful in certain cases where you need a simpler setup or when dealing with third-party libraries. However, I often prefer controlled components because they allow for a tighter integration with the rest of the application’s logic. When you build forms using controlled components, you can react to user input immediately. This helps in creating dynamic user experiences, such as enabling or disabling buttons based on the validity of the input or providing real-time feedback on password strength. Getting Started With a Simple Form To get you started, I’ll walk through a basic example of a form in React JS. Consider a simple login form with an email and password field. In a controlled component, the input fields would look something like this: import React, { useState } from 'react'; function LoginForm() { const [email, setEmail] = useState(''); const [password, setPassword] = useState(''); const handleSubmit = (event) => { event.preventDefault(); // You can process the login data here console.log('Email:', email); console.log('Password:', password); }; return ( Email: setEmail(e.target.value)} /> Password: setPassword(e.target.value)} /> Login ); } export default LoginForm; In this snippet, I use the useState hook to keep track of the input values. The handleSubmit function prevents the default behavior of the form and then logs the user’s input to the console. This is the basis for handling more advanced operations like form validation, submission to a server, or even integration with a state management library. Best Practices for Handling Form Data Based on my experience, here are a few best practices that I’ve found useful: Keep It Simple: Don’t overcomplicate your form logic. Start with a small, manageable component and build up complexity as needed. Use Meaningful Names: When naming your state variables and input fields, choose names that indicate what they represent. This makes your code easier to read and maintain. Validate Early: I always try to validate form data as soon as the user enters it. This not only improves the user experience by catching errors early but also saves time when it comes to debugging. Handle Errors Gracefully: If something goes wrong, provide feedback to the user in a friendly manner. This might mean showing an error message near the input or using visual cues like a red border around a problematic field. Consider Accessibility: Make sure your forms are accessible. Use proper labels and ARIA attributes when needed. Accessible forms benefit everyone, including users with disabilities. Leverage Libraries: Sometimes, the complexity of forms might require more advanced solutions. Libraries like Formik and React Hook Form provide additional tools and patterns that can simplify the process. I have used t
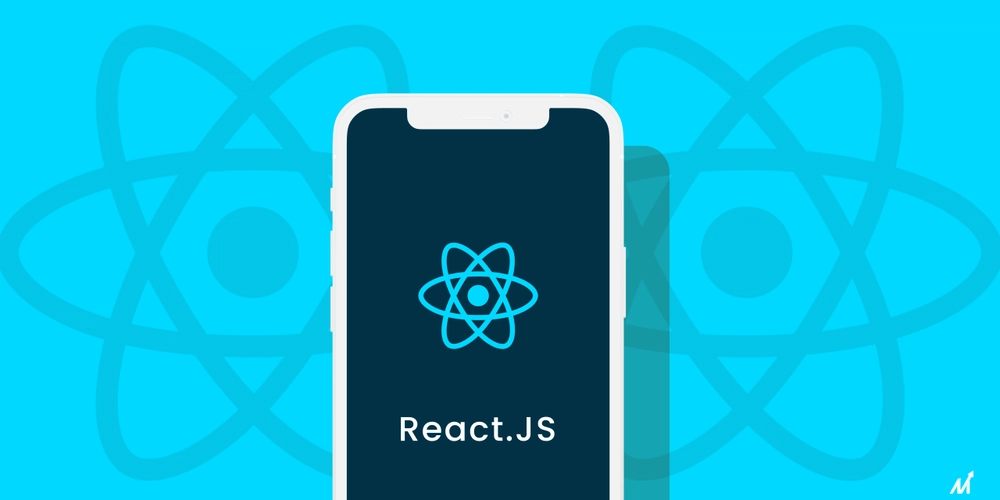
Introduction.
Handling form data in React JS is a topic that touches every developer who builds interactive web applications.
I’ve spent a lot of time working with forms and managing user input, and I know first-hand that forms are the bridge between your users and your app’s logic.
In this post, I’ll share my insights, tips, and best practices to help you confidently handle form data in React JS.
Why Form Data Matters
Forms are everywhere—registration pages, search bars, comment sections, and even complex data entry applications. The way you handle form data affects not only the performance of your app but also the overall user experience.
With React JS, handling forms is straightforward once you understand the core concepts.
This post is meant to be a friendly guide that walks you through the basic to advanced ideas while keeping things light and accessible.
React gives me the flexibility to build powerful interactive applications with clean code, and the way it manages state makes handling form inputs both enjoyable and efficient.
The process might seem tricky at first, but with a few simple steps, you’ll be able to build forms that feel responsive and intuitive.
Understanding Controlled vs. Uncontrolled Components
One of the first things I learned when working with forms in React JS was the concept of controlled and uncontrolled components.
- Controlled Components: These are the go-to for many developers because they tie the form’s data directly to the component state.
Every time a user types into a form input, an event handler updates the state, and the input displays the updated state.
This approach gives you complete control over the data and makes it easier to validate user input on the fly.
For example, if you want to display a custom error message when a user enters an invalid email, a controlled component lets you do that easily.
- Uncontrolled Components: In this approach, the form data is handled by the DOM itself. It can be useful in certain cases where you need a simpler setup or when dealing with third-party libraries.
However, I often prefer controlled components because they allow for a tighter integration with the rest of the application’s logic.
When you build forms using controlled components, you can react to user input immediately.
This helps in creating dynamic user experiences, such as enabling or disabling buttons based on the validity of the input or providing real-time feedback on password strength.
Getting Started With a Simple Form
To get you started, I’ll walk through a basic example of a form in React JS.
Consider a simple login form with an email and password field. In a controlled component, the input fields would look something like this:
import React, { useState } from 'react';
function LoginForm() {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
// You can process the login data here
console.log('Email:', email);
console.log('Password:', password);
};
return (
<form onSubmit={handleSubmit}>
<label>
Email:
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
label>
<br />
<label>
Password:
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
label>
<br />
<button type="submit">Loginbutton>
form>
);
}
export default LoginForm;
In this snippet, I use the useState
hook to keep track of the input values.
The handleSubmit
function prevents the default behavior of the form and then logs the user’s input to the console.
This is the basis for handling more advanced operations like form validation, submission to a server, or even integration with a state management library.
Best Practices for Handling Form Data
Based on my experience, here are a few best practices that I’ve found useful:
Keep It Simple: Don’t overcomplicate your form logic. Start with a small, manageable component and build up complexity as needed.
Use Meaningful Names: When naming your state variables and input fields, choose names that indicate what they represent. This makes your code easier to read and maintain.
Validate Early: I always try to validate form data as soon as the user enters it. This not only improves the user experience by catching errors early but also saves time when it comes to debugging.
Handle Errors Gracefully: If something goes wrong, provide feedback to the user in a friendly manner.
This might mean showing an error message near the input or using visual cues like a red border around a problematic field.
Consider Accessibility: Make sure your forms are accessible. Use proper labels and ARIA attributes when needed. Accessible forms benefit everyone, including users with disabilities.
Leverage Libraries: Sometimes, the complexity of forms might require more advanced solutions. Libraries like Formik and React Hook Form provide additional tools and patterns that can simplify the process.
I have used these libraries on several projects to reduce boilerplate code and improve performance.
Advanced Techniques for Complex Forms
For those times when your form grows in complexity, handling data can become a bit trickier. Here are a few techniques I’ve found invaluable:
Dynamic Forms
Sometimes, the form fields are not static and may change based on user input or external data. In these cases, I recommend building your form components dynamically.
For example, if you have a list of questions that change based on the user’s previous answers, you can map over an array of question objects and render the appropriate input fields.
This technique keeps your code modular and makes it easier to handle conditional logic.
Custom Hooks
Creating custom hooks for form handling can help encapsulate repetitive logic.
By creating a hook that handles the common tasks—such as input change events, validations, and error handling—you can reuse this logic across multiple forms. This approach not only saves time but also makes your code cleaner.
State Management
As forms become more complex, managing state can be challenging. In these cases, I often rely on state management libraries like Redux or Context API.
This allows me to manage the state of my form data in a more scalable way, especially when multiple components need to interact with the form data.
Handling File Uploads
When your forms include file uploads, the complexity increases. React doesn’t handle file uploads directly; instead, you need to work with the browser’s FormData API.
I typically create a separate function that processes the file input and sends the data to the server using AJAX.
This separation of concerns helps keep the main form component uncluttered.
Testing Your Forms
No form handling discussion would be complete without mentioning testing. I make it a point to test my forms to ensure that they behave as expected.
Tools like Jest and React Testing Library come in handy for simulating user interactions and checking that the form state updates correctly.
Testing not only helps catch bugs early but also gives you confidence that your form will work in production.
Frequently Asked Questions
What is the difference between controlled and uncontrolled components in React JS?
Controlled components have their form data managed by React state, while uncontrolled components rely on the DOM to handle form data.
I tend to prefer controlled components because they allow for better validation and real-time feedback.
How can I validate form data in React JS?
I usually validate form data on every change by adding functions that check the value of the input fields.
For example, you can check if an email input includes an "@" symbol. There are also libraries available that provide more advanced validation options.
Are there libraries that can help manage forms in React?
Yes, libraries like Formik and React Hook Form are popular choices.
They simplify the process by handling state, validation, and error messages, allowing you to focus on building your app’s logic.
How do I handle file uploads in React forms?
For file uploads, I use the browser’s FormData API. This allows you to gather the file from an input field and send it to the server via AJAX, keeping the main form logic separate.
Further Resources
- React Documentation: The official React docs are a great place to start for understanding forms in React.
- Formik Official Site: This library can help simplify your form handling tasks.
- React Hook Form: An alternative library for handling forms with an emphasis on performance.
- MDN Web Docs: For a deeper dive into the FormData API and file uploads.
I’ve found that diving into these resources can provide new ideas and techniques that might be just the right solution for your next project.
Conclusion
Form data is a critical part of creating interactive, user-friendly applications in React JS.
From the basics of controlled components to more advanced topics like dynamic forms and file uploads, there’s a lot to consider when building forms.
I hope this post has provided you with a clear path forward and has demystified some of the more challenging aspects of handling form data.
Every project comes with its own set of challenges, but with a good grasp of these techniques and a bit of practice, you’ll be well on your way to building robust forms that improve the user experience.
Now, I’d love to hear from you. How do you handle form data in React JS?