How to Fix 'Cannot regenerate session id - headers already sent' in Yii
When migrating a Yii application to a new shared host, you may encounter the warning: session_regenerate_id(): Cannot regenerate session id - headers already sent. This issue typically arises when output is sent to the browser before PHP attempts to modify the session. Let's dive into the causes and solutions for this common problem, ensuring your Yii application runs smoothly on the new server. Understanding the Warning The warning signifies that some output was sent to the browser before your PHP script tried to refresh the session. This can often happen if there are unexpected characters or whitespace before the opening tag in your PHP files. Other causes can include echo or print statements executed before session manipulation. The use of the function headers_sent($filename, $linenum) in your actionLogin method is a good approach to identify where the issue is occurring. However, if you find no output errors with that function, further investigation into file encoding and whitespace issues is necessary. Steps to Troubleshoot and Fix the Issue 1. Check for Extra Whitespace One of the most common causes of this warning is extraneous whitespace. Ensure your PHP files do not contain any whitespace or blank lines outside the tags: Pay special attention to files that are included or required. It’s a good practice not to include closing PHP tags in files that contain only PHP code to avoid this issue:
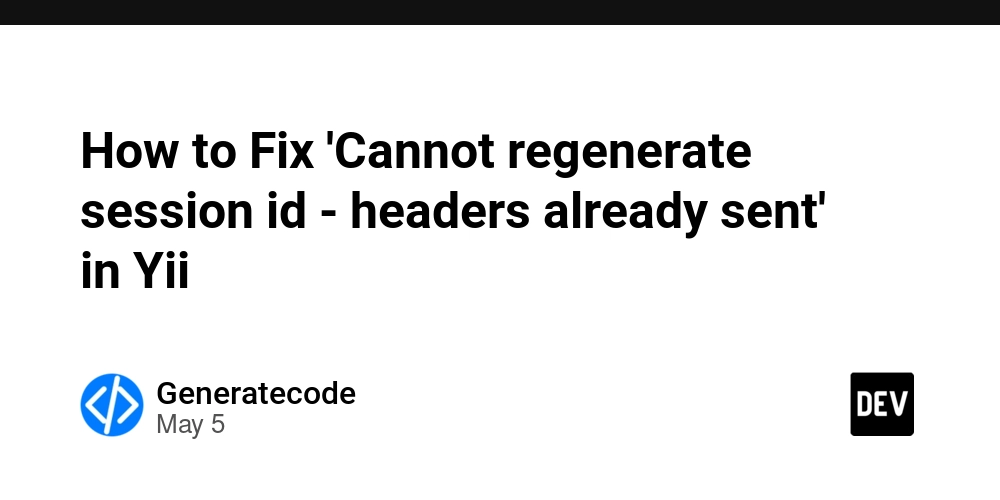
When migrating a Yii application to a new shared host, you may encounter the warning: session_regenerate_id(): Cannot regenerate session id - headers already sent
. This issue typically arises when output is sent to the browser before PHP attempts to modify the session. Let's dive into the causes and solutions for this common problem, ensuring your Yii application runs smoothly on the new server.
Understanding the Warning
The warning signifies that some output was sent to the browser before your PHP script tried to refresh the session. This can often happen if there are unexpected characters or whitespace before the opening The use of the function One of the most common causes of this warning is extraneous whitespace. Ensure your PHP files do not contain any whitespace or blank lines outside the Pay special attention to files that are included or required. It’s a good practice not to include closing PHP tags in files that contain only PHP code to avoid this issue:
It's great that you've confirmed your files are saved as 'UTF-8 without BOM'. However, sometimes you might want to ensure they are compiled correctly without any BOM. You can do this using Notepad++ by going to Encoding > Encode in UTF-8 without BOM. Then, re-save the files.
If your main file runs well, it’s critical to check any included files for the same issues. Open all files that are included in your Yii application and repeat the checks for whitespace and BOM.
Ensure you do not have any statements like Sometimes, server settings may cause headers to be sent earlier than anticipated. Check your server configuration and ensure there are no output buffering issues or other settings limiting session handling.
By following the steps outlined above, you should be able to resolve the warning and ensure your Yii app runs properly after migration. If you still face issues, consider enabling output buffering at the start of your script using:
This will buffer all output until your script terminates, preventing premature header calls. Remember, careful monitoring and consistent practices will help prevent these issues in the future.
What is output buffering in PHP? What does headers already sent mean? Can I ignore this warning? tag or after the closing
?>
tag in your PHP files. Other causes can include echo or print statements executed before session manipulation.
headers_sent($filename, $linenum)
in your actionLogin
method is a good approach to identify where the issue is occurring. However, if you find no output errors with that function, further investigation into file encoding and whitespace issues is necessary.
Steps to Troubleshoot and Fix the Issue
1. Check for Extra Whitespace
and
?>
tags:
2. Confirm File Encoding
3. Inspect Included Files
4. Review Output Commands
echo
, print
, or HTML code before the session manipulation. In your actionLogin
, isolate the header call and session functions from any output generating code. Here’s a clean code structure:
public function actionLogin($name = null) {
$model = new LoginForm();
if ($name) {
$model->username = $name;
}
if (isset($_POST['ajax']) && $_POST['ajax'] === 'login-form') {
echo CActiveForm::validate($model);
Yii::app()->end();
}
// Validate only if POST request is from the login form
if (isset($_POST['LoginForm'])) {
$model->attributes = $_POST['LoginForm'];
// Check if headers have already been sent
if (headers_sent($filename, $linenum)) {
echo "Headers have been sent in {$filename} line number is {$linenum}";
exit;
}
// Validate user input and redirect if valid
if ($model->validate() && $model->login()) {
$this->redirect(Yii::app()->user->returnUrl);
}
}
// Display the login form
$this->render('login', array('model' => $model));
}
5. Review Server Configuration
Conclusion
ob_start();
Frequently Asked Questions (FAQ)
Output buffering allows you to manage the output sent to the browser, preventing accidental header outputs.
It means that PHP has already started to send response headers to the client, hence it cannot change session-related headers anymore.
No, it is essential to resolve it as it indicates that sessions may not work correctly, affecting authentication and state management in your application.