How to Efficiently Randomly Select from Rust BitSet
Introduction If you're working with a simulation program in Rust that leverages a BitSet for efficient storage of integers, you might find yourself needing to select random values quickly. Your current implementation uses an iterator combined with a random number generator, but in high-performance applications, every millisecond counts. Let's explore an optimized approach to selecting a random element from a BitSet with minimal overhead. Why Performance Matters In your case, the random_element function is called around one million times per simulation step. This translates to significant time spent in this function during critical parts of your simulation. Each call to the function involves iterating through the BitSet and making a random choice, which can be inefficient, particularly when the BitSet might be represented compactly in memory. If the typical size of your BitSet is small (e.g., around 30 integers), there is potential for optimization by accessing elements directly instead of iterating. By taking full advantage of how BitSet stores data in memory, we can streamline the random selection process. Optimized Random Selection Strategy Instead of using set.iter().choose(rng), we can directly work with the BitSet representation. Here’s how you can do it efficiently: Step 1: Count Set Bits First, we need to count how many bits are set in the BitSet. This requires a simple traversal through the BitSet, which should be relatively inexpensive. Step 2: Generate a Random Index Next, we can generate a random index from 0 to the count of set bits. Then, we iterate over the bits again, this time keeping track of the index until we find our target. Step 3: Implement the Function Here’s the revamped implementation: use bit_set::BitSet; use rand::Rng; /// Returns a random element from the given set efficiently. fn random_element(set: &BitSet, rng: &mut impl Rng) -> Option { // Count the number of set bits let count = set.len(); // count of set bits if count == 0 { return None; // Prevents selection from an empty set } // Generate a random index let target_index = rng.gen_range(0..count); // Exclude the unset bits by iterating through the BitSet let mut current_index = 0; for i in 0..set.capacity() { if set.contains(i) { if current_index == target_index { return Some(i); } current_index += 1; } } None // Safety return } Explanation of the Code Counting Set Bits: The len method returns the number of set bits, allowing us to know how many valid selections are possible. Random Index Generation: Using rng.gen_range(0..count) generates a random number that directly indexes into the range of available set bits. Efficient Iteration: Instead of checking every element with an iterator, we utilize a for-loop that checks each index directly, stopping when we reach our target. This effectively reduces the number of iterations needed, especially when the BitSet is densely populated. Conclusion The above implementation should yield significant performance improvements for your specific use case, especially under the tight constraints of simulation timing. This approach eliminates unnecessary iterations and streamlines access patterns, giving you a much faster way to select random elements from your BitSet. Frequently Asked Questions How does this method improve performance compared to the previous one? This method reduces the overhead of using an iterator by directly accessing the bits in the set based on their indices, which is more optimal for small fixed-sized ranges, allowing quicker selections. What happens if the BitSet is empty? The function gracefully handles an empty BitSet by returning None, ensuring that you avoid potential runtime errors in the case of accessing non-existent elements. Can this method handle large BitSet sizes? While this method is optimized for smaller sizes (like your typical usage around 30 integers), it can still handle larger sizes up to the limits imposed by memory. For very large sizes, you may need to consider other data structures or methods suited for large direct access.
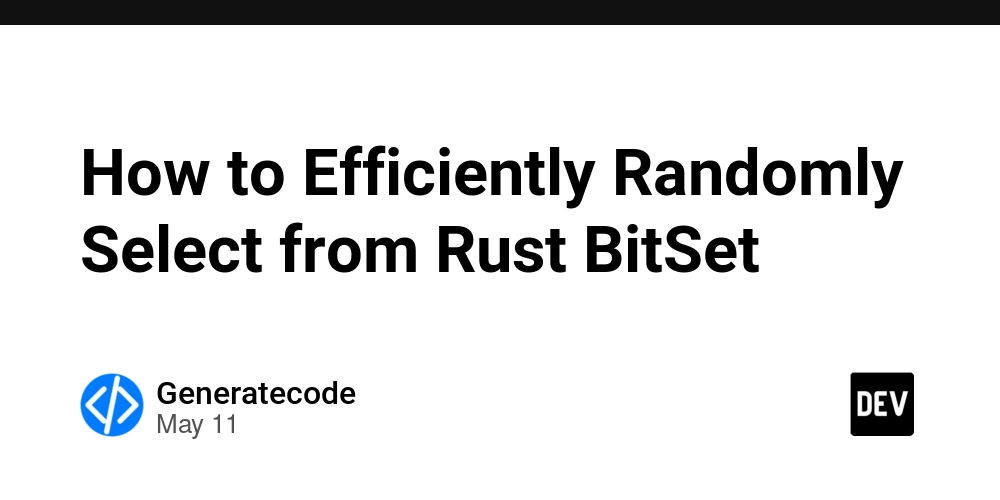
Introduction
If you're working with a simulation program in Rust that leverages a BitSet
for efficient storage of integers, you might find yourself needing to select random values quickly. Your current implementation uses an iterator combined with a random number generator, but in high-performance applications, every millisecond counts. Let's explore an optimized approach to selecting a random element from a BitSet
with minimal overhead.
Why Performance Matters
In your case, the random_element
function is called around one million times per simulation step. This translates to significant time spent in this function during critical parts of your simulation. Each call to the function involves iterating through the BitSet
and making a random choice, which can be inefficient, particularly when the BitSet
might be represented compactly in memory.
If the typical size of your BitSet
is small (e.g., around 30 integers), there is potential for optimization by accessing elements directly instead of iterating. By taking full advantage of how BitSet
stores data in memory, we can streamline the random selection process.
Optimized Random Selection Strategy
Instead of using set.iter().choose(rng)
, we can directly work with the BitSet
representation. Here’s how you can do it efficiently:
Step 1: Count Set Bits
First, we need to count how many bits are set in the BitSet
. This requires a simple traversal through the BitSet
, which should be relatively inexpensive.
Step 2: Generate a Random Index
Next, we can generate a random index from 0
to the count of set bits. Then, we iterate over the bits again, this time keeping track of the index until we find our target.
Step 3: Implement the Function
Here’s the revamped implementation:
use bit_set::BitSet;
use rand::Rng;
/// Returns a random element from the given set efficiently.
fn random_element(set: &BitSet, rng: &mut impl Rng) -> Option {
// Count the number of set bits
let count = set.len(); // count of set bits
if count == 0 {
return None; // Prevents selection from an empty set
}
// Generate a random index
let target_index = rng.gen_range(0..count);
// Exclude the unset bits by iterating through the BitSet
let mut current_index = 0;
for i in 0..set.capacity() {
if set.contains(i) {
if current_index == target_index {
return Some(i);
}
current_index += 1;
}
}
None // Safety return
}
Explanation of the Code
-
Counting Set Bits: The
len
method returns the number of set bits, allowing us to know how many valid selections are possible. -
Random Index Generation: Using
rng.gen_range(0..count)
generates a random number that directly indexes into the range of available set bits. -
Efficient Iteration: Instead of checking every element with an iterator, we utilize a for-loop that checks each index directly, stopping when we reach our target. This effectively reduces the number of iterations needed, especially when the
BitSet
is densely populated.
Conclusion
The above implementation should yield significant performance improvements for your specific use case, especially under the tight constraints of simulation timing. This approach eliminates unnecessary iterations and streamlines access patterns, giving you a much faster way to select random elements from your BitSet
.
Frequently Asked Questions
How does this method improve performance compared to the previous one?
This method reduces the overhead of using an iterator by directly accessing the bits in the set based on their indices, which is more optimal for small fixed-sized ranges, allowing quicker selections.
What happens if the BitSet is empty?
The function gracefully handles an empty BitSet
by returning None
, ensuring that you avoid potential runtime errors in the case of accessing non-existent elements.
Can this method handle large BitSet sizes?
While this method is optimized for smaller sizes (like your typical usage around 30 integers), it can still handle larger sizes up to the limits imposed by memory. For very large sizes, you may need to consider other data structures or methods suited for large direct access.