How to Add a Login Page with SQL for Username and Password?
Creating a login page for your project is essential for user authentication and security. In this article, we'll walk you through the process of adding a SQL-based login page to your existing project. You can access the project at this GitHub link, where the fundamental files are stored. By the end of this tutorial, you'll understand how to implement a basic username and password entry system. Understanding the Importance of User Authentication User authentication is critical for any web application that requires user privacy and data protection. By allowing users to enter their username and password, you can ensure that sensitive information and resources remain secure. Database Setup for Username and Password Storage To add user authentication, we need a database to store the username and password. We will be using SQL for this purpose. Here’s a step-by-step guide to set up your database. Step 1: Create a Database First, you need to create a database where we will store user information. Run the following SQL command: CREATE DATABASE user_auth; Step 2: Create a User Table Next, create a table to store the username and hashed password information: USE user_auth; CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(50) NOT NULL UNIQUE, password VARCHAR(255) NOT NULL ); Step 3: Inserting Sample User Data For testing purposes, we can insert a sample user into the database. Here's how you can do it: INSERT INTO users (username, password) VALUES ('testuser', SHA2('mypassword', 256)); Note: We are using the SHA-256 hashing algorithm to encrypt passwords before storing them in the database, promoting better security. Integrating the Login Page Now that we have our database and user table set up, let’s integrate a login page into your project. This will involve creating a simple HTML form that allows users to enter their credentials. Step 4: Creating the HTML Login Form Create a new HTML file named login.html with the following code: Login Page Login Username: Password: Login Step 5: Back-end Authentication Logic Next, create a PHP file named authenticate.php to handle authentication:
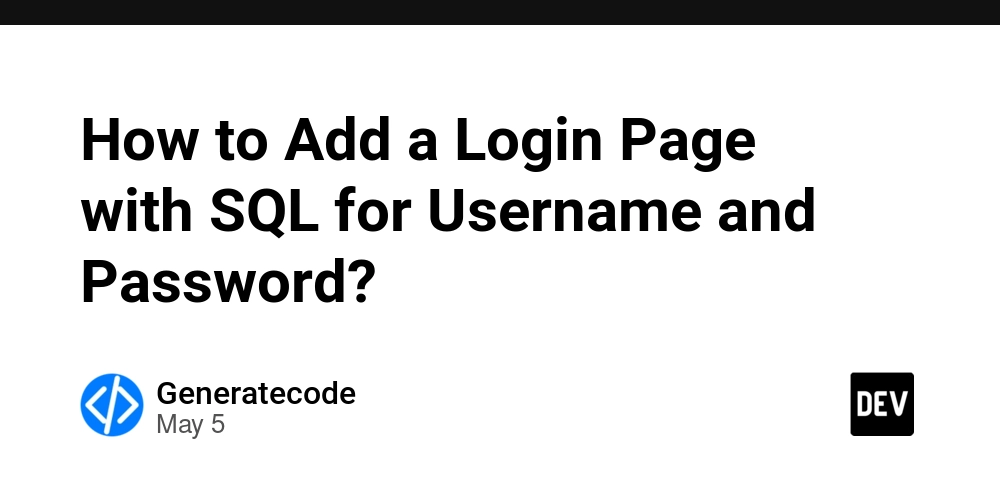
Creating a login page for your project is essential for user authentication and security. In this article, we'll walk you through the process of adding a SQL-based login page to your existing project. You can access the project at this GitHub link, where the fundamental files are stored. By the end of this tutorial, you'll understand how to implement a basic username and password entry system.
Understanding the Importance of User Authentication
User authentication is critical for any web application that requires user privacy and data protection. By allowing users to enter their username and password, you can ensure that sensitive information and resources remain secure.
Database Setup for Username and Password Storage
To add user authentication, we need a database to store the username and password. We will be using SQL for this purpose. Here’s a step-by-step guide to set up your database.
Step 1: Create a Database
First, you need to create a database where we will store user information. Run the following SQL command:
CREATE DATABASE user_auth;
Step 2: Create a User Table
Next, create a table to store the username and hashed password information:
USE user_auth;
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL UNIQUE,
password VARCHAR(255) NOT NULL
);
Step 3: Inserting Sample User Data
For testing purposes, we can insert a sample user into the database. Here's how you can do it:
INSERT INTO users (username, password) VALUES ('testuser', SHA2('mypassword', 256));
Note: We are using the SHA-256 hashing algorithm to encrypt passwords before storing them in the database, promoting better security.
Integrating the Login Page
Now that we have our database and user table set up, let’s integrate a login page into your project. This will involve creating a simple HTML form that allows users to enter their credentials.
Step 4: Creating the HTML Login Form
Create a new HTML file named login.html
with the following code:
Login Page
Login
Step 5: Back-end Authentication Logic
Next, create a PHP file named authenticate.php
to handle authentication:
connect_error) {
die("Connection failed: " . $conn->connect_error);
}
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$user = $_POST['username'];
$pass = hash('sha256', $_POST['password']); // Securely hash password for comparison
$sql = "SELECT * FROM users WHERE username='$user' AND password='$pass';";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
echo "Login successful!";
} else {
echo "Invalid username or password.";
}
}
$conn->close();
?>
Explanation of the PHP Code
In this back-end script, we're connecting to the database and checking if the submitted username and password match those stored in the users table. If they do, a success message is displayed; otherwise, an error message appears.
Frequently Asked Questions
How do I secure the password storage further?
To further secure the password, consider using a password hashing library, such as password_hash()
in PHP, which implements stronger encryption mechanisms.
Can I add more fields to the user table?
Yes, you can extend the table by adding more fields, such as email, full name, or even user roles for further authentication features.
What if I forget my registered username or password?
Implementing a password recovery feature would allow users to reset their passwords through email verification or security questions.
Conclusion
In this tutorial, we walked through how to add a functional login page with SQL to manage usernames and passwords securely. By following these steps, you can integrate authentication into your project and ensure users have a secure experience. Make sure to further enhance security measures where necessary for better data protection.