High-Level Language vs. Low-Level Language: A Detailed Comparison
Introduction Programming languages are broadly classified into high-level languages and low-level languages based on their abstraction from machine code. This classification impacts performance, readability, portability, and control over hardware. This blog provides a detailed comparison between high-level and low-level languages, covering their definitions, types, advantages, disadvantages, and real-world use cases. 1. What is a High-Level Language? A high-level programming language (HLL) provides an abstraction from machine details, allowing developers to write human-readable code. These languages use English-like syntax, making them easier to write, read, and maintain. Examples of High-Level Languages: General-purpose: C, C++, Java, Python, JavaScript Web development: HTML, CSS, PHP Scripting: Python, Perl, Ruby Data Science & AI: R, Julia Features of High-Level Languages: ✅ Human-readable: Uses English-like syntax (e.g., print("Hello, World!")) ✅ Portable: Can run on multiple operating systems without modification ✅ Memory management: Automatic memory allocation and garbage collection ✅ Built-in functions & libraries: Provides predefined functions for faster development ✅ Error handling: Provides exception handling mechanisms 2. What is a Low-Level Language? A low-level programming language interacts directly with hardware and provides little to no abstraction from a computer’s architecture. It is machine-dependent and optimized for performance and efficiency. Types of Low-Level Languages: Machine Language (1st Generation) Direct binary code (0s and 1s) Fastest execution but difficult to write Assembly Language (2nd Generation) Uses mnemonics (MOV, ADD, SUB) instead of binary Requires an assembler to convert into machine code Examples of Low-Level Languages: Machine Language (Binary Code) → 10110101 00001101 Assembly Language (Mnemonics) → MOV AX, 1 Features of Low-Level Languages: ✅ Fast execution: Runs directly on hardware ✅ Efficient memory usage: Provides full control over memory allocation ✅ Precise hardware control: Used in device drivers, operating systems, and embedded systems 3. Key Differences Between High-Level and Low-Level Languages Feature High-Level Language Low-Level Language Abstraction High (closer to human language) Low (closer to hardware) Ease of Use Easy to learn and use Harder to learn Performance Slower than low-level languages Extremely fast execution Portability Platform-independent Machine-dependent Memory Management Automatic (Garbage Collection) Manual (developer-controlled) Error Handling Strong exception handling Minimal or no error handling Compilation/Interpretation Uses compilers or interpreters Uses assemblers 4. Advantages & Disadvantages High-Level Language ✅ Advantages: Easy to learn and write Portable across different platforms Built-in debugging and error handling ❌ Disadvantages: Slower than low-level languages Less control over hardware Requires a compiler or interpreter Low-Level Language ✅ Advantages: Extremely fast execution Full control over hardware Efficient use of memory ❌ Disadvantages: Hard to learn and debug Not portable (machine-dependent) Requires deep hardware knowledge 5. When to Use Which? Scenario Best Choice Web & Mobile Apps High-Level Language (JavaScript, Python, Java, Swift) System Programming (OS, Drivers) Low-Level Language (C, Assembly) Game Development C++ (mix of both high and low level) Embedded Systems (IoT, Microcontrollers) Low-Level Language (Assembly, C) Machine Learning & Data Science High-Level Language (Python, R) Database Management High-Level Language (SQL, Python) 6. Real-World Examples Example of High-Level Language (Python) # Python program to print "Hello, World!" print("Hello, World!") ✅ Easy to read and understand ✅ Portable across Windows, Linux, Mac Example of Low-Level Language (Assembly) section .data msg db "Hello, World!", 0 section .text global _start _start: mov eax, 4 ; sys_write mov ebx, 1 ; file descriptor (stdout) mov ecx, msg ; message to print mov edx, 13 ; message length int 0x80 ; call kernel mov eax, 1 ; sys_exit xor ebx, ebx ; return 0 int 0x80 ✅ Fast execution but harder to understand 7. Conclusion If you want... Choose... Easy development & maintenance High-Level Language Best performance & efficiency Low-Level Language Hardware-level control Low-Level Language Cross-platform compatibility High-Level Language Most modern applications use high-level languages for faster development, while low-level languages are used for performance-cr
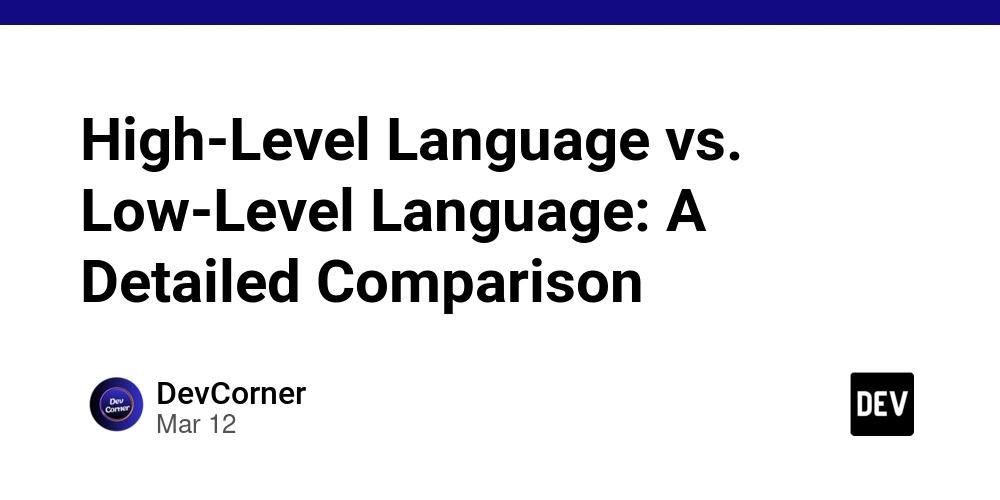
Introduction
Programming languages are broadly classified into high-level languages and low-level languages based on their abstraction from machine code. This classification impacts performance, readability, portability, and control over hardware.
This blog provides a detailed comparison between high-level and low-level languages, covering their definitions, types, advantages, disadvantages, and real-world use cases.
1. What is a High-Level Language?
A high-level programming language (HLL) provides an abstraction from machine details, allowing developers to write human-readable code. These languages use English-like syntax, making them easier to write, read, and maintain.
Examples of High-Level Languages:
- General-purpose: C, C++, Java, Python, JavaScript
- Web development: HTML, CSS, PHP
- Scripting: Python, Perl, Ruby
- Data Science & AI: R, Julia
Features of High-Level Languages:
✅ Human-readable: Uses English-like syntax (e.g., print("Hello, World!")
)
✅ Portable: Can run on multiple operating systems without modification
✅ Memory management: Automatic memory allocation and garbage collection
✅ Built-in functions & libraries: Provides predefined functions for faster development
✅ Error handling: Provides exception handling mechanisms
2. What is a Low-Level Language?
A low-level programming language interacts directly with hardware and provides little to no abstraction from a computer’s architecture. It is machine-dependent and optimized for performance and efficiency.
Types of Low-Level Languages:
-
Machine Language (1st Generation)
- Direct binary code (0s and 1s)
- Fastest execution but difficult to write
-
Assembly Language (2nd Generation)
- Uses mnemonics (
MOV
,ADD
,SUB
) instead of binary - Requires an assembler to convert into machine code
- Uses mnemonics (
Examples of Low-Level Languages:
-
Machine Language (Binary Code) →
10110101 00001101
-
Assembly Language (Mnemonics) →
MOV AX, 1
Features of Low-Level Languages:
✅ Fast execution: Runs directly on hardware
✅ Efficient memory usage: Provides full control over memory allocation
✅ Precise hardware control: Used in device drivers, operating systems, and embedded systems
3. Key Differences Between High-Level and Low-Level Languages
Feature | High-Level Language | Low-Level Language |
---|---|---|
Abstraction | High (closer to human language) | Low (closer to hardware) |
Ease of Use | Easy to learn and use | Harder to learn |
Performance | Slower than low-level languages | Extremely fast execution |
Portability | Platform-independent | Machine-dependent |
Memory Management | Automatic (Garbage Collection) | Manual (developer-controlled) |
Error Handling | Strong exception handling | Minimal or no error handling |
Compilation/Interpretation | Uses compilers or interpreters | Uses assemblers |
4. Advantages & Disadvantages
High-Level Language
✅ Advantages:
- Easy to learn and write
- Portable across different platforms
- Built-in debugging and error handling
❌ Disadvantages:
- Slower than low-level languages
- Less control over hardware
- Requires a compiler or interpreter
Low-Level Language
✅ Advantages:
- Extremely fast execution
- Full control over hardware
- Efficient use of memory
❌ Disadvantages:
- Hard to learn and debug
- Not portable (machine-dependent)
- Requires deep hardware knowledge
5. When to Use Which?
Scenario | Best Choice |
---|---|
Web & Mobile Apps | High-Level Language (JavaScript, Python, Java, Swift) |
System Programming (OS, Drivers) | Low-Level Language (C, Assembly) |
Game Development | C++ (mix of both high and low level) |
Embedded Systems (IoT, Microcontrollers) | Low-Level Language (Assembly, C) |
Machine Learning & Data Science | High-Level Language (Python, R) |
Database Management | High-Level Language (SQL, Python) |
6. Real-World Examples
Example of High-Level Language (Python)
# Python program to print "Hello, World!"
print("Hello, World!")
✅ Easy to read and understand
✅ Portable across Windows, Linux, Mac
Example of Low-Level Language (Assembly)
section .data
msg db "Hello, World!", 0
section .text
global _start
_start:
mov eax, 4 ; sys_write
mov ebx, 1 ; file descriptor (stdout)
mov ecx, msg ; message to print
mov edx, 13 ; message length
int 0x80 ; call kernel
mov eax, 1 ; sys_exit
xor ebx, ebx ; return 0
int 0x80
✅ Fast execution but harder to understand
7. Conclusion
If you want... | Choose... |
---|---|
Easy development & maintenance | High-Level Language |
Best performance & efficiency | Low-Level Language |
Hardware-level control | Low-Level Language |
Cross-platform compatibility | High-Level Language |
Most modern applications use high-level languages for faster development, while low-level languages are used for performance-critical applications like operating systems, embedded systems, and drivers.
Both types are essential in different scenarios, and as a developer, understanding them will help you choose the right tool for the right job.
8. Related Topics