Hidden Classes and Inline Caches in V8
Hidden Classes and Inline Caches in V8: A Definitive Guide Introduction JavaScript has evolved significantly since its inception in 1995, transitioning from a simple scripting language to a cornerstone of modern web development. One of the key engines enabling this transformation is Google's V8, an open-source JavaScript engine designed for high performance. Central to V8's efficiency are two advanced concepts: Hidden Classes and Inline Caches. Understanding these mechanisms is crucial for optimizing JavaScript applications, especially those that require intricate data interactions or performance-sensitive operations. In this comprehensive guide, we will delve into the historical context, technical details, and practical implications of hidden classes and inline caches, providing the depth and nuance required for advanced JavaScript developers. Historical Context The Need for Optimization As JavaScript gained popularity, performance became a primary concern, especially with the dawn of rich web applications that relied extensively on JavaScript. Traditional interpreted languages perform poorly in computational-heavy environments. JavaScript engines like V8 adopted Just-In-Time (JIT) compilation to enhance execution speed. However, the dynamic nature of JavaScript—such as variable types being swapped or properties being added during runtime—poses challenges to optimization. The Genesis of Hidden Classes V8 addresses some of these challenges through the use of Hidden Classes. When an object is created, V8 maps it to a hidden class that defines its structure. This allows the engine to perform optimizations by changing how it accesses and manages properties based on the structure rather than the object's identity. The hidden class mechanism can be thought of as a shape descriptor for the object, enabling rapid property access. This was introduced as part of "Optimizing Compilation" to maximize the JIT efficiency while maintaining JavaScript’s dynamic capabilities. The Emergence of Inline Caches Inline Caches (ICs) are another optimization applied by V8 to reduce the overhead of property access. When an object property is accessed, V8 utilizes ICs to remember the last known type of the receiver object and the hidden class associated with that property. This optimization mitigates the need to traverse the prototype chain or rebuild hidden classes dynamically, enabling faster access to properties. Both hidden classes and inline caches are foundational to V8’s ability to achieve significant speed gains, taking JavaScript performance to levels that were previously unreachable. Technical Overview Understanding Hidden Classes When V8 creates an object, it assigns it a hidden class based on its structure. If the object is modified—either by adding or removing properties—V8 may create a new hidden class to reflect these changes. Consider the following example: function createPoint(x, y) { return { x: x, y: y }; } let p1 = createPoint(1, 2); let p2 = createPoint(3, 4); In the above function, each invocation of createPoint creates distinct objects p1 and p2 that share the same hidden class if no dynamic modifications are made. Transitioning Hidden Classes Hidden classes can transition. Suppose you add a z property to p1: p1.z = 5; Here, V8 transitions p1 from its initial hidden class to a new hidden class that accounts for the added z property. The graph below illustrates the transition: Initial Hidden Class --> Adding Property 'z' --> New Hidden Class Exploring Inline Caches Inline caches aim to optimize property access by storing the result of the last property lookup. Let’s examine a simple function that utilizes inline caching: function getX(point) { return point.x; } When getX is invoked multiple times with the same object structure, V8 records the hidden class of point associated with the property x. Subsequent calls use the cached descriptor, significantly reducing lookup overhead. Example with Inline Caches: let p = createPoint(1, 2); console.log(getX(p)); // First call, cache miss console.log(getX(p)); // Second call, cache hit with IC In this scenario, the first call updates the inline cache with p’s hidden class, improving access speed on the second invocation. Advanced Implementation Scenarios 1. Property Deletion: Interestingly, deleting properties can lead to performance pitfalls. For example: delete p1.z; // Causes the hidden class to transition to a more generic one This transition can lead to a decrease in efficiency since every subsequent access to p1 may need to revert to a less optimized path due to the change in property shape. 2. Frequent Object Modifications: Consider a case where you frequently update an object dynamically: function updatePoint(p, newX, newY) { p.x = newX; p.y = newY; } Ev
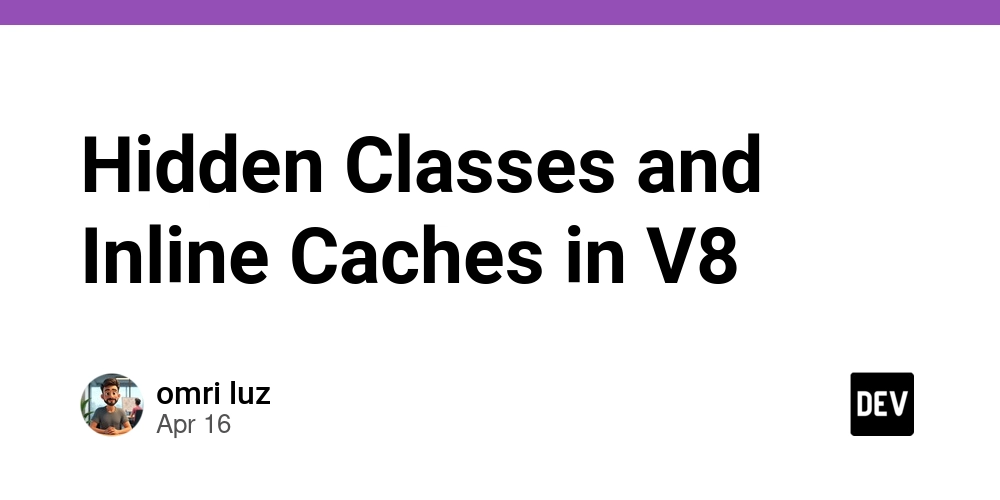
Hidden Classes and Inline Caches in V8: A Definitive Guide
Introduction
JavaScript has evolved significantly since its inception in 1995, transitioning from a simple scripting language to a cornerstone of modern web development. One of the key engines enabling this transformation is Google's V8, an open-source JavaScript engine designed for high performance. Central to V8's efficiency are two advanced concepts: Hidden Classes and Inline Caches. Understanding these mechanisms is crucial for optimizing JavaScript applications, especially those that require intricate data interactions or performance-sensitive operations. In this comprehensive guide, we will delve into the historical context, technical details, and practical implications of hidden classes and inline caches, providing the depth and nuance required for advanced JavaScript developers.
Historical Context
The Need for Optimization
As JavaScript gained popularity, performance became a primary concern, especially with the dawn of rich web applications that relied extensively on JavaScript. Traditional interpreted languages perform poorly in computational-heavy environments. JavaScript engines like V8 adopted Just-In-Time (JIT) compilation to enhance execution speed. However, the dynamic nature of JavaScript—such as variable types being swapped or properties being added during runtime—poses challenges to optimization.
The Genesis of Hidden Classes
V8 addresses some of these challenges through the use of Hidden Classes. When an object is created, V8 maps it to a hidden class that defines its structure. This allows the engine to perform optimizations by changing how it accesses and manages properties based on the structure rather than the object's identity.
The hidden class mechanism can be thought of as a shape descriptor for the object, enabling rapid property access. This was introduced as part of "Optimizing Compilation" to maximize the JIT efficiency while maintaining JavaScript’s dynamic capabilities.
The Emergence of Inline Caches
Inline Caches (ICs) are another optimization applied by V8 to reduce the overhead of property access. When an object property is accessed, V8 utilizes ICs to remember the last known type of the receiver object and the hidden class associated with that property. This optimization mitigates the need to traverse the prototype chain or rebuild hidden classes dynamically, enabling faster access to properties.
Both hidden classes and inline caches are foundational to V8’s ability to achieve significant speed gains, taking JavaScript performance to levels that were previously unreachable.
Technical Overview
Understanding Hidden Classes
When V8 creates an object, it assigns it a hidden class based on its structure. If the object is modified—either by adding or removing properties—V8 may create a new hidden class to reflect these changes.
Consider the following example:
function createPoint(x, y) {
return { x: x, y: y };
}
let p1 = createPoint(1, 2);
let p2 = createPoint(3, 4);
In the above function, each invocation of createPoint
creates distinct objects p1
and p2
that share the same hidden class if no dynamic modifications are made.
Transitioning Hidden Classes
Hidden classes can transition. Suppose you add a z
property to p1
:
p1.z = 5;
Here, V8 transitions p1
from its initial hidden class to a new hidden class that accounts for the added z
property. The graph below illustrates the transition:
Initial Hidden Class --> Adding Property 'z' --> New Hidden Class
Exploring Inline Caches
Inline caches aim to optimize property access by storing the result of the last property lookup. Let’s examine a simple function that utilizes inline caching:
function getX(point) {
return point.x;
}
When getX
is invoked multiple times with the same object structure, V8 records the hidden class of point
associated with the property x
. Subsequent calls use the cached descriptor, significantly reducing lookup overhead.
Example with Inline Caches:
let p = createPoint(1, 2);
console.log(getX(p)); // First call, cache miss
console.log(getX(p)); // Second call, cache hit with IC
In this scenario, the first call updates the inline cache with p
’s hidden class, improving access speed on the second invocation.
Advanced Implementation Scenarios
1. Property Deletion: Interestingly, deleting properties can lead to performance pitfalls. For example:
delete p1.z; // Causes the hidden class to transition to a more generic one
This transition can lead to a decrease in efficiency since every subsequent access to p1
may need to revert to a less optimized path due to the change in property shape.
2. Frequent Object Modifications: Consider a case where you frequently update an object dynamically:
function updatePoint(p, newX, newY) {
p.x = newX;
p.y = newY;
}
Every call to updatePoint
not only modifies properties but also can transition hidden classes, leading to performance degradation, as V8 may have to create new hidden classes on the fly.
let point = createPoint(1, 2);
for (let i = 0; i < 100000; i++) {
updatePoint(point, i, i + 1);
}
In such cases, it's advisable to create dedicated structures upfront, mitigating the overhead of continual hidden class transitions.
Performance Considerations
Benchmarking Hidden Classes and Inline Caches
To evaluate the performance implications, you can run benchmarks using Node.js and the built-in console.time
:
console.time('createPoints');
for (let i = 0; i < 1000000; i++) {
createPoint(i, i + 1);
}
console.timeEnd('createPoints'); // Analyze creation time
Optimization Strategies
- Avoid Dynamic Property Modifications: As elucidated, dynamic property addition or deletion can hamper optimizations. Define the object's structure up front.
- Use Class Syntax for Consistency: Leverage class syntax to create a more static blueprints for objects which V8 can optimize more effectively.
class Point {
constructor(x, y) {
this.x = x;
this.y = y;
}
}
- Profile Regularly: Employ tooling such as Chrome DevTools or Node's built-in profiling tools to understand how hidden classes and inline caches affect your code's performance.
Potential Pitfalls
Overhead of Hidden Classes
Creating hidden classes incurs overhead when the object's shape changes frequently. As applications grow and evolve, the custom handling of object structures based on usage patterns becomes critical to maintain performance.
Debugging Hidden Class Transitions
Detecting performance regressions due to hidden class transitions can be challenging. One effective strategy is to utilize Node.js's V8 inspector.
- Start your application with the inspector enabled:
node --inspect-brk app.js
Open Chrome and navigate to
chrome://inspect
.You can observe memory structures and performance metrics related to hidden classes.
Real-World Use Cases
Framework Optimization: Large frameworks like React or Vue.js maintain performance by leveraging predictable object structures, minimizing unnecessary transitions and cache misses.
Game Engines: Complex game applications often rely on high-performance rendering through consistent object shapes, enhancing runtime efficiency through V8's optimizations.
Comparison with Alternative Approaches
Performance Trade-offs
Other JavaScript engines, like Firefox's SpiderMonkey or Apple's JavaScriptCore, adopt varying optimization techniques. For instance, both engines use similar optimization strategies but differ in implementation details.
Inline Caches vs. Method JIT Compilation
- Inline Caches are largely focused on property access performance, while more comprehensive strategies may involve JIT compilation, which optimizes entire methods based on execution context and usage patterns.
- In comparison to V8's hidden classes, SpiderMonkey's system might utilize "shape" structures more dynamically, potentially offering different trade-offs in terms of flexibility versus performance.
Advanced References
- V8 GitHub Repository
- Google V8 Blog
- JavaScript Engine Design - In-depth discussion on optimization strategies and engine internals.
Conclusion
In conclusion, hidden classes and inline caches are pivotal in making V8 a leading JavaScript engine in terms of performance. By gaining a deep understanding of these concepts, developers can write more efficient JavaScript code that scales well. Performance considerations, trade-offs, and optimization techniques outlined provide ample guidance for building performant applications that leverage the full power of V8's capabilities. Mastery of hidden classes and inline caches is an excellent investment for any serious JavaScript developer aiming to harness maximum performance from their applications.