Getting Started with Slugs in React: My Experience
Hey everyone! I recently started working with React and wanted to share how I implemented slugs in my project. It was easier than I expected and made a big difference in my app's URLs. What is a slug? A slug is just the part of a URL that identifies a page in human-readable keywords. For example, in mysite.com/blog/learning-react, the slug is learning-react. Why I needed slugs I was building a small portfolio site with a blog section. At first, my URLs looked like this: mysite.com/posts/1 mysite.com/posts/2 These numbers didn't tell users or search engines anything about the content. I wanted something more descriptive. How I implemented slugs 1. Installing slugify I used the slugify package: npm install slugify 2. Creating the slugs Here's the simple code I used: import slugify from 'slugify'; // When creating a new post function createPost(title, content) { const slug = slugify(title, { lower: true }); // Save post with the slug savePost({ title, content, slug }); return slug; } 3. Setting up routes I used React Router to handle the slug-based URLs: import { Routes, Route } from 'react-router-dom'; function App() { return ( ); } 4. Displaying posts In my BlogPost component: import { useParams } from 'react-router-dom'; function BlogPost() { const { slug } = useParams(); const [post, setPost] = useState(null); useEffect(() => { // Find post by slug const foundPost = posts.find(p => p.slug === slug); setPost(foundPost); }, [slug]); if (!post) return Post not found; return ( {post.title} {post.content} ); } Problems I ran into The only issue I had was duplicate slugs. If two posts had the same title, they'd get the same slug. I fixed this by adding a simple counter: function createUniqueSlug(title, existingSlugs) { let slug = slugify(title, { lower: true }); let counter = 1; while(existingSlugs.includes(slug)) { slug = `${slugify(title, { lower: true })}-${counter}`; counter++; } return slug; } What I learned Using slugs was a simple change that made my React app more professional. The URLs are now readable and SEO-friendly. The slugify package handled all the complicated stuff like removing special characters and spaces. If you're new to React like me, I'd definitely recommend implementing slugs in your projects - it's a small touch that makes a big difference!
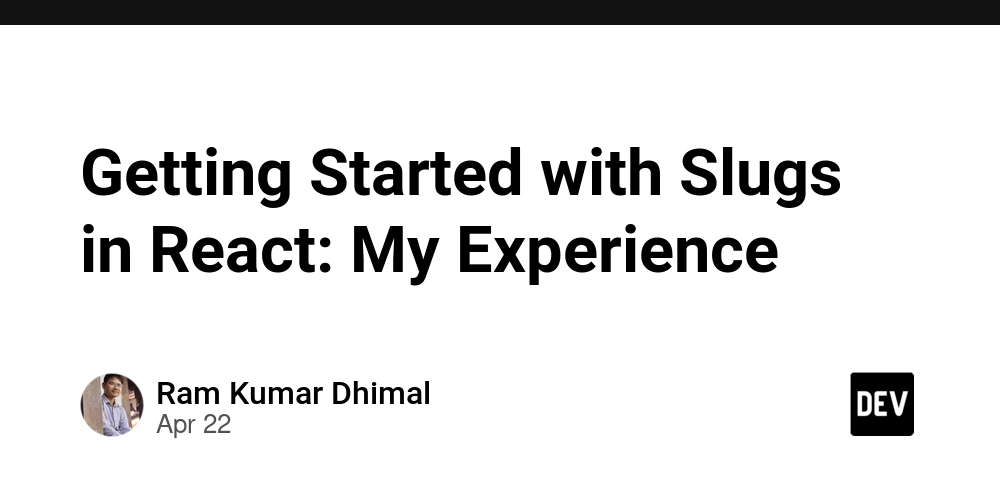
Hey everyone! I recently started working with React and wanted to share how I implemented slugs in my project. It was easier than I expected and made a big difference in my app's URLs.
What is a slug?
A slug is just the part of a URL that identifies a page in human-readable keywords. For example, in mysite.com/blog/learning-react
, the slug is learning-react
.
Why I needed slugs
I was building a small portfolio site with a blog section. At first, my URLs looked like this:
mysite.com/posts/1
mysite.com/posts/2
These numbers didn't tell users or search engines anything about the content. I wanted something more descriptive.
How I implemented slugs
1. Installing slugify
I used the slugify
package:
npm install slugify
2. Creating the slugs
Here's the simple code I used:
import slugify from 'slugify';
// When creating a new post
function createPost(title, content) {
const slug = slugify(title, { lower: true });
// Save post with the slug
savePost({
title,
content,
slug
});
return slug;
}
3. Setting up routes
I used React Router to handle the slug-based URLs:
import { Routes, Route } from 'react-router-dom';
function App() {
return (
<Routes>
<Route path="/" element={<Home />} />
<Route path="/posts/:slug" element={<BlogPost />} />
</Routes>
);
}
4. Displaying posts
In my BlogPost component:
import { useParams } from 'react-router-dom';
function BlogPost() {
const { slug } = useParams();
const [post, setPost] = useState(null);
useEffect(() => {
// Find post by slug
const foundPost = posts.find(p => p.slug === slug);
setPost(foundPost);
}, [slug]);
if (!post) return <p>Post not found</p>;
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
}
Problems I ran into
The only issue I had was duplicate slugs. If two posts had the same title, they'd get the same slug. I fixed this by adding a simple counter:
function createUniqueSlug(title, existingSlugs) {
let slug = slugify(title, { lower: true });
let counter = 1;
while(existingSlugs.includes(slug)) {
slug = `${slugify(title, { lower: true })}-${counter}`;
counter++;
}
return slug;
}
What I learned
Using slugs was a simple change that made my React app more professional. The URLs are now readable and SEO-friendly. The slugify
package handled all the complicated stuff like removing special characters and spaces.
If you're new to React like me, I'd definitely recommend implementing slugs in your projects - it's a small touch that makes a big difference!