Generate Unique, Sortable, and Decodable IDs for Node.js and Browsers. How to use Kinoid
Kinoid is an ultra-lightweight JavaScript library engineered for the generation of unique, URL-friendly, and temporally sortable identifiers. Designed for compatibility across Node.js and browser environments, it leverages a timestamp-based approach to produce compact IDs encoded in base36. Why Choose Kinoid? Kinoid is a robust and efficient solution for unique ID generation. Several key attributes warrant its consideration. Firstly, guaranteed uniqueness is a fundamental characteristic; each generated ID is distinct, even under conditions of high-frequency generation within the same millisecond or across distributed systems. Secondly, the inherent time-sortability of the IDs, based on their creation timestamp, renders them suitable for time-sensitive operations and chronological ordering. Furthermore, decodability is a significant feature, allowing for the extraction of embedded information such as the precise generation timestamp, process identifier, and the singularity factor directly from the ID string. The library exhibits cross-platform compatibility, ensuring seamless operation within both Node.js server-side applications and client-side browser contexts. Finally, Kinoid's design emphasizes efficiency, being lightweight and fast with no external dependencies, thereby facilitating rapid integration and minimizing performance overhead. Irrespective of whether the development context involves backend services, frontend applications, or hybrid architectures, Kinoid offers a concise yet potent mechanism for managing unique dentifiers. How Does Kinoid Work? Kinoid generates unique IDs by combining three key components: Timestamp: Each ID includes the number of milliseconds that have passed since a specific date. This ensures that IDs are naturally sortable by the time they were created. Process ID: To make IDs unique across different processes, Kinoid includes the process ID (PID) of the environment where the ID is generated. This is particularly useful in multi-process or distributed systems. Singularity Factor: Even if multiple IDs are generated within the same millisecond, Kinoid uses a sequential number (the singularity factor) to ensure that each ID remains unique. Once these components are combined, Kinoid encodes the ID in base36 format. This encoding makes the IDs compact, URL-friendly, and easy to read. Why Base36? Base36 encoding uses numbers (0-9) and lowercase letters (a-z), making the IDs shorter and more human-readable compared to other formats like hexadecimal. It’s also ideal for use in URLs, as it avoids special characters that might need encoding. By combining these elements, Kinoid ensures that every ID is: Unique: No two IDs are the same, even if generated in the same millisecond. Sortable: IDs can be sorted chronologically based on their creation time. Decodable: You can extract meaningful information, such as the timestamp, process ID, and singularity factor, directly from the ID. This approach makes Kinoid a reliable and efficient solution for generating unique identifiers in a wide range of applications. Installation # with npm npm install kinoid # with yarn yarn add kinoid Practical Examples 1. Generate a New ID const { newId } = require('kinoid')(); const id = newId(); console.log(id); // cohb4z87mvoyf1zjy 2. Decode an ID const { decodeId } = require('kinoid')(); const decoded = decodeId('cohb4z87mvoyf1zjy'); console.log(decoded); // Output: // { // id: 'cohb4z87mvoyf1zjy', // date: 2024-11-19T16:52:19.962Z, // singularity: 1144, // pid: 5438 // } 3. Validate an ID The decodeId() function returns an object with an error message if it receives an invalid ID as input. It can therefore also be used for ID validation. const { decodeId } = require('kinoid')(); const invalidId = 'c1vz87moyfzjyoHB4'; console.log(decodeId(invalidId)); // Output: // { // id: 'c1vz87moyfzjyoHB4', // error: 'the string c1vz87moyfzjyoHB4 is not a valid ID' // } It is very easy to use decodeId() to build a boolean validator const { decodeId } = require('kinoid')(); function isValidId(id) { Object.hasOwn(decodeId(id), 'error'); } const invalidId = 'c1vz87moyfzjyoHB4'; const validated = isValidId(invalidId); // false Finally, the README file in the GitHub repository or on the NPM page contains detailed examples of how to use Kinoid in all Node.js scenarios or in browser applications. Comparison with Other Libraries Library Uniqueness Sortable Decodable Size (kB) Kinoid ✅ ✅ ✅ ~1.6 uuid ✅ ❌ ❌ ~10.3 nanoid ✅ ❌ ❌ ~0.8 Data collected from bundlephobia and npmjs Some versions of uuid produce a sortable ID. When to Use Kinoid? Kinoid proves particularly beneficial in scenarios requiring the generation of unique identifiers. A primary application lies in the creation of IDs for database records. The time-sor
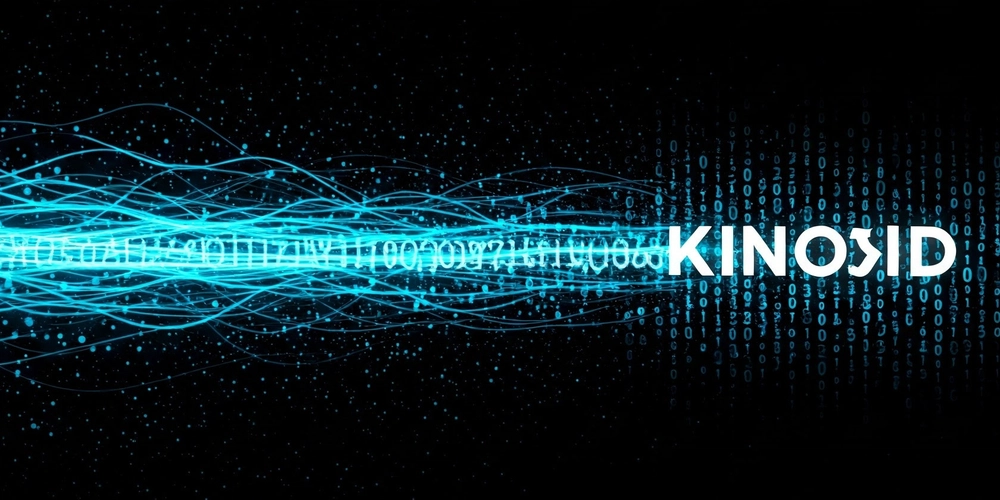
Kinoid is an ultra-lightweight JavaScript library engineered for the generation of unique, URL-friendly, and temporally sortable identifiers. Designed for compatibility across Node.js and browser environments, it leverages a timestamp-based approach to produce compact IDs encoded in base36.
Why Choose Kinoid?
Kinoid is a robust and efficient solution for unique ID generation. Several key attributes warrant its consideration.
Firstly, guaranteed uniqueness is a fundamental characteristic; each generated ID is distinct, even under conditions of high-frequency generation within the same millisecond or across distributed systems.
Secondly, the inherent time-sortability of the IDs, based on their creation timestamp, renders them suitable for time-sensitive operations and chronological ordering.
Furthermore, decodability is a significant feature, allowing for the extraction of embedded information such as the precise generation timestamp, process identifier, and the singularity factor directly from the ID string.
The library exhibits cross-platform compatibility, ensuring seamless operation within both Node.js server-side applications and client-side browser contexts.
Finally, Kinoid's design emphasizes efficiency, being lightweight and fast with no external dependencies, thereby facilitating rapid integration and minimizing performance overhead.
Irrespective of whether the development context involves backend services, frontend applications, or hybrid architectures, Kinoid offers a concise yet potent mechanism for managing unique dentifiers.
How Does Kinoid Work?
Kinoid generates unique IDs by combining three key components:
Timestamp: Each ID includes the number of milliseconds that have passed since a specific date. This ensures that IDs are naturally sortable by the time they were created.
Process ID: To make IDs unique across different processes, Kinoid includes the process ID (PID) of the environment where the ID is generated. This is particularly useful in multi-process or distributed systems.
Singularity Factor: Even if multiple IDs are generated within the same millisecond, Kinoid uses a sequential number (the singularity factor) to ensure that each ID remains unique.
Once these components are combined, Kinoid encodes the ID in base36 format. This encoding makes the IDs compact, URL-friendly, and easy to read.
Why Base36?
Base36 encoding uses numbers (0-9) and lowercase letters (a-z), making the IDs shorter and more human-readable compared to other formats like hexadecimal. It’s also ideal for use in URLs, as it avoids special characters that might need encoding.
By combining these elements, Kinoid ensures that every ID is:
- Unique: No two IDs are the same, even if generated in the same millisecond.
- Sortable: IDs can be sorted chronologically based on their creation time.
- Decodable: You can extract meaningful information, such as the timestamp, process ID, and singularity factor, directly from the ID.
This approach makes Kinoid a reliable and efficient solution for generating unique identifiers in a wide range of applications.
Installation
# with npm
npm install kinoid
# with yarn
yarn add kinoid
Practical Examples
1. Generate a New ID
const { newId } = require('kinoid')();
const id = newId();
console.log(id); // cohb4z87mvoyf1zjy
2. Decode an ID
const { decodeId } = require('kinoid')();
const decoded = decodeId('cohb4z87mvoyf1zjy');
console.log(decoded);
// Output:
// {
// id: 'cohb4z87mvoyf1zjy',
// date: 2024-11-19T16:52:19.962Z,
// singularity: 1144,
// pid: 5438
// }
3. Validate an ID
The decodeId()
function returns an object with an error message if it receives an invalid ID as input. It can therefore also be used for ID validation.
const { decodeId } = require('kinoid')();
const invalidId = 'c1vz87moyfzjyoHB4';
console.log(decodeId(invalidId));
// Output:
// {
// id: 'c1vz87moyfzjyoHB4',
// error: 'the string c1vz87moyfzjyoHB4 is not a valid ID'
// }
It is very easy to use decodeId()
to build a boolean validator
const { decodeId } = require('kinoid')();
function isValidId(id) {
Object.hasOwn(decodeId(id), 'error');
}
const invalidId = 'c1vz87moyfzjyoHB4';
const validated = isValidId(invalidId);
// false
Finally, the README
file in the GitHub repository or on the NPM
page contains detailed examples of how to use Kinoid in all Node.js scenarios or in browser applications.
Comparison with Other Libraries
Library | Uniqueness | Sortable | Decodable | Size (kB) |
---|---|---|---|---|
Kinoid | ✅ | ✅ | ✅ | ~1.6 |
uuid | ✅ | ❌ | ❌ | ~10.3 |
nanoid | ✅ | ❌ | ❌ | ~0.8 |
- Data collected from bundlephobia and npmjs
- Some versions of
uuid
produce a sortable ID.
When to Use Kinoid?
Kinoid proves particularly beneficial in scenarios requiring the generation of unique identifiers.
A primary application lies in the creation of IDs for database records. The time-sortable nature of Kinoid-generated IDs can offer advantages in indexing and querying time-based data.
Furthermore, Kinoid is well-suited for generating unique and URL-friendly identifiers for resources within web applications. Its base36 encoding ensures compact and easily shareable URLs.
Another compelling use case involves the creation of unique identifiers for sessions or users, where the embedded timestamp can be valuable for tracking session activity or user creation times.
Conclusion
Kinoid is a simple and powerful solution for generating unique and sortable IDs. Try it today and see how easy it is to integrate into your projects!