Angular NgModules vs Standalone Components
Angular provides two powerful ways to organize and encapsulate your application's components, directives, pipes, and services: NgModules and Standalone Components. Understanding the differences, benefits, and proper usage of these systems can greatly enhance your application structure and maintainability. What are NgModules? NgModules group related components, services, directives, and pipes together, providing clear boundaries and helping manage complexity. Basic Structure of an NgModule import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; @NgModule({ declarations: [AppComponent], imports: [BrowserModule], providers: [], bootstrap: [AppComponent] }) export class AppModule { } Benefits of NgModules Clear organization of application features. Improved maintainability through structured encapsulation. Easier lazy loading for performance optimization. What are Standalone Components? Standalone components, introduced in Angular 14, enable you to create components, directives, and pipes independently of NgModules, simplifying the application structure. Creating a Standalone Component import { Component } from '@angular/core'; @Component({ selector: 'app-hello', standalone: true, template: 'Hello, Standalone Component!' }) export class HelloComponent {} Using Standalone Components Standalone components directly import other dependencies without needing NgModules: import { Component } from '@angular/core'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-list', standalone: true, imports: [CommonModule], template: ` {{ item }} ` }) export class ListComponent { items = ['Item 1', 'Item 2', 'Item 3']; } Benefits of Standalone Components Reduced boilerplate code and complexity. Simplified application architecture. Enhanced flexibility in organizing and structuring applications. NgModules vs. Standalone Components Feature NgModules Standalone Components Organization Structured through modules Individual components Dependency Management Centralized via modules Directly imported into components Lazy Loading Supported via module-level routing Granular and direct component loading Complexity Higher due to more boilerplate Reduced boilerplate and complexity Best Practices Use NgModules for large, complex applications that benefit from clear module boundaries and structure. Adopt Standalone Components for new, simpler applications or to gradually refactor existing applications towards a more streamlined architecture. Clearly document the architectural choices in your project to ensure consistent implementation. Conclusion Both NgModules and Standalone Components offer unique strengths. Choosing the right approach depends on your application's needs and complexity. Leveraging these effectively can lead to cleaner, more maintainable, and highly performant Angular applications. Have you started integrating Standalone Components in your Angular projects? Share your insights and experiences below!
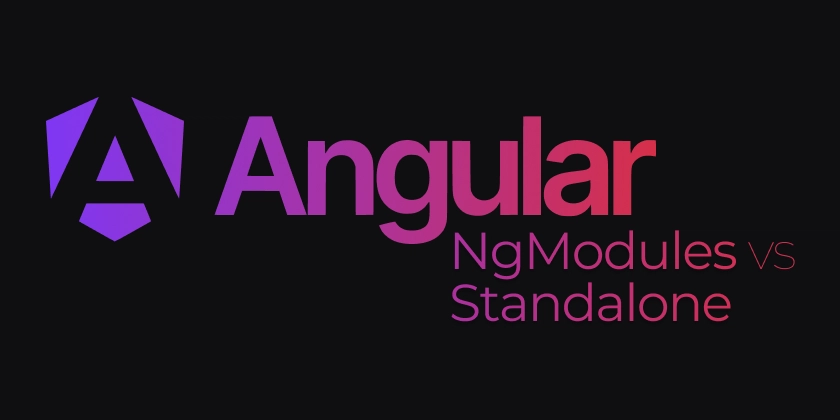
Angular provides two powerful ways to organize and encapsulate your application's components, directives, pipes, and services: NgModules and Standalone Components. Understanding the differences, benefits, and proper usage of these systems can greatly enhance your application structure and maintainability.
What are NgModules?
NgModules group related components, services, directives, and pipes together, providing clear boundaries and helping manage complexity.
Basic Structure of an NgModule
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Benefits of NgModules
- Clear organization of application features.
- Improved maintainability through structured encapsulation.
- Easier lazy loading for performance optimization.
What are Standalone Components?
Standalone components, introduced in Angular 14, enable you to create components, directives, and pipes independently of NgModules, simplifying the application structure.
Creating a Standalone Component
import { Component } from '@angular/core';
@Component({
selector: 'app-hello',
standalone: true,
template: 'Hello, Standalone Component!
'
})
export class HelloComponent {}
Using Standalone Components
Standalone components directly import other dependencies without needing NgModules:
import { Component } from '@angular/core';
import { CommonModule } from '@angular/common';
@Component({
selector: 'app-list',
standalone: true,
imports: [CommonModule],
template: `
- {{ item }}
`
})
export class ListComponent {
items = ['Item 1', 'Item 2', 'Item 3'];
}
Benefits of Standalone Components
- Reduced boilerplate code and complexity.
- Simplified application architecture.
- Enhanced flexibility in organizing and structuring applications.
NgModules vs. Standalone Components
Feature | NgModules | Standalone Components |
---|---|---|
Organization | Structured through modules | Individual components |
Dependency Management | Centralized via modules | Directly imported into components |
Lazy Loading | Supported via module-level routing | Granular and direct component loading |
Complexity | Higher due to more boilerplate | Reduced boilerplate and complexity |
Best Practices
- Use NgModules for large, complex applications that benefit from clear module boundaries and structure.
- Adopt Standalone Components for new, simpler applications or to gradually refactor existing applications towards a more streamlined architecture.
- Clearly document the architectural choices in your project to ensure consistent implementation.
Conclusion
Both NgModules and Standalone Components offer unique strengths. Choosing the right approach depends on your application's needs and complexity. Leveraging these effectively can lead to cleaner, more maintainable, and highly performant Angular applications.
Have you started integrating Standalone Components in your Angular projects? Share your insights and experiences below!