Exceptions in DDD
I'm learning DDD and I'm thinking about throwing exceptions in certain situations. I understand that an object can not enter to a bad state so here the exceptions are fine, but in many examples exceptions are throwing also for example if we are trying add new user with exists email in database. public function doIt(UserData $userData) { $user = $this->userRepository->byEmail($userData->email()); if ($user) { throw new UserAlreadyExistsException(); } $this->userRepository->add( new User( $userData->email(), $userData->password() ) ); } So if user with this email exists, then we can in application service catch a exception, but we shouldn't control the operation of the application using the try-catch block. How is best way for this?
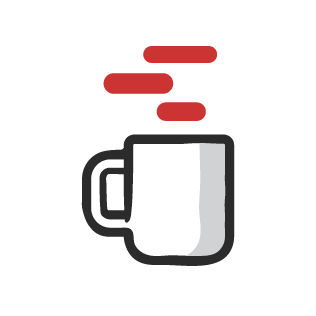
I'm learning DDD and I'm thinking about throwing exceptions in certain situations. I understand that an object can not enter to a bad state so here the exceptions are fine, but in many examples exceptions are throwing also for example if we are trying add new user with exists email in database.
public function doIt(UserData $userData)
{
$user = $this->userRepository->byEmail($userData->email());
if ($user) {
throw new UserAlreadyExistsException();
}
$this->userRepository->add(
new User(
$userData->email(),
$userData->password()
)
);
}
So if user with this email exists, then we can in application service catch a exception, but we shouldn't control the operation of the application using the try-catch block.
How is best way for this?