Installing The XRPL Library This tutorial uses the xrpl library installed via npm npm install xrpl Baby Steps. In order to make meaningful interactions with the xrp ledger , such as currency transactions, wallet creation, etc. We must first connect to the server. We do so, by: importing the Client Object from the xrpl library Instantiating the Client object with the xrpl websocket server address. making a connection using connect() Disconnecting when the interaction is done. //ES6 Imports import {Client} from 'xrpl' async function connectToLedger(){ //Instantiate the object with xrpl address as an argument and store its reference. const client = new Client('wss://s.altnet.rippletest.net:51233/') //Connect Via the connect function await client.connect() console.log(await client.connection) //insert other code down here //Bla Bla Bla client.disconnect(); } connectToLedger() A brief breakdown xrp test network : wss://s.altnet.rippletest.net:51233/1 Faucets In the realm of xrp, there exist a set of developer tools called Faucets aka test nets. Faucets, Replicate the functionality of the mainet allowing you to test ledger interactions on a testing environment, and it also provides a websocket for fulfilling these interactions. These interactions include but are not limited to wallet creation, and token transactions. Learn more: https://xrpl.org/resources/dev-tools/xrp-faucets Why Dov We Need Faucets? Some mainet interactions requires a small amount of xrp for ex; transactions, fund transfers, and tokenization, The benefit of a faucet really starts to shine when it comes to testing transactions and other important blockchain functionality without risking real xrp. Wallet Creation Now lets create a wallet and provide some funds. import {Client} from 'xrpl' async function connectToLedger(){ //Instantiate the object with xrpl address as an argument and store its reference. const client = new Client('wss://s.altnet.rippletest.net:51233/') //Connect Via the connect function await client.connect() //Instantiating A wallet with fundWallet and storing its reference. let testFund = await client.fundWallet(); //Waiting for promise and storing referennce of the wallet property let wallet = (await testFund).wallet //Waiting for promise and storing the wallet balance property let balance = (await testFund).balance console.log(`Wallet Public Key : ${wallet.publicKey} \nWallet Balance : ${balance}` ) client.disconnect(); } connectToLedger() Code Breakdown fundWallet() on line 10 we call the asynchronous fundWallet method from our previous client object. this function returns an object containing a Wallet object, and within the Wallet object there are details such as its publicKey andprivateKey and seed phrase. Alongside the Wallet object we have its balance. In essence the function: generates a new wallet. Initiates a faucet transaction that sends 100 test XRP to the wallet’s public address via a WebSocket connection. This triggers a sequence of interactions between operation and issuer accounts on the test network. Why FundWallet? As we mentioned earlier, this is a testing environment, so we are able to utilize functions such as fundWallet to easily retrieve a dispensable wallet with dispensable funds, however you are not allowed to use fundWallet() on the mainet, you need to generate a wallet and send it funds via an external wallet with xrp or a Digital Exchange. See [[#Generating A wallet on the main-net.]] for more details. Ex { wallet: Wallet { publicKey: 'kgphikthpotpruktf325235rpkopkwpokgwopenr12359322f', privateKey: 'idjfi0wufj14faafaaffff110124812401gsafawfwafa4', classicAddress: 'idjfi0wufj14afafawawfafaafawfawfaw95329595f', seed: 'afiafjawifjawoifaw' }, balance: 100 } Querying data from a ledger. client.request() This method, is used for querying various types of ledger data, Afterall a ledger is a data structure and thus can be used as database. This method accepts a object map for query configuration. The current configuration we are using is: let onChainData= await client.request({ command:"account_info", //The type of data we want to querry account:wallet.address, // The specific account or adress we want to querry ledger_index:"validated", // Most recently validated ledger index }); console.log(`Balance In Drops : ${onChainData.result.account_data.Balance}`) Config Key Description command The query type account The account we desire to query in this context were using the address of the recently generated wallet ledger_index A specific ledger selector - in this context were selecting the most recently v
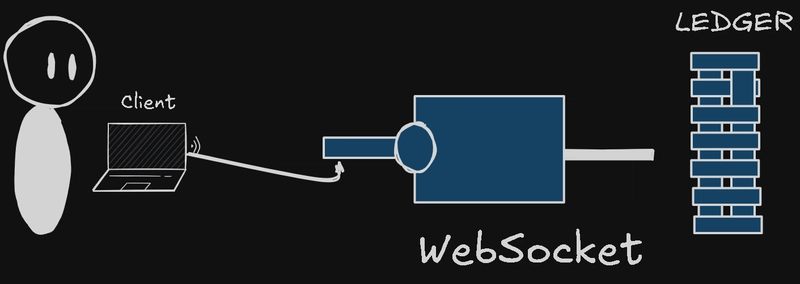
Installing The XRPL Library
This tutorial uses the xrpl library installed via npm
npm install xrpl
Baby Steps.
In order to make meaningful interactions with the xrp ledger , such as currency transactions, wallet creation, etc. We must first connect to the server.
We do so, by:
- importing the
Client
Object from thexrpl
library - Instantiating the
Client
object with the xrplwebsocket
server address. - making a connection
using connect()
- Disconnecting when the interaction is done.
//ES6 Imports
import {Client} from 'xrpl'
async function connectToLedger(){
//Instantiate the object with xrpl address as an argument and store its reference.
const client = new Client('wss://s.altnet.rippletest.net:51233/')
//Connect Via the connect function
await client.connect()
console.log(await client.connection)
//insert other code down here
//Bla Bla Bla
client.disconnect();
}
connectToLedger()
A brief breakdown xrp test network : wss://s.altnet.rippletest.net:51233/1
Faucets
In the realm of xrp, there exist a set of developer tools called Faucets aka test nets.
Faucets, Replicate the functionality of the mainet
allowing you to test ledger interactions on a testing environment, and it also provides a websocket
for fulfilling these interactions. These interactions include but are not limited to wallet creation, and token transactions.
Learn more: https://xrpl.org/resources/dev-tools/xrp-faucets
Why Dov We Need Faucets?
Some mainet
interactions requires a small amount of xrp for ex; transactions, fund transfers, and tokenization, The benefit of a faucet really starts to shine when it comes to testing transactions and other important blockchain functionality without risking real xrp.
Wallet Creation
Now lets create a wallet and provide some funds.
import {Client} from 'xrpl'
async function connectToLedger(){
//Instantiate the object with xrpl address as an argument and store its reference.
const client = new Client('wss://s.altnet.rippletest.net:51233/')
//Connect Via the connect function
await client.connect()
//Instantiating A wallet with fundWallet and storing its reference.
let testFund = await client.fundWallet();
//Waiting for promise and storing referennce of the wallet property
let wallet = (await testFund).wallet
//Waiting for promise and storing the wallet balance property
let balance = (await testFund).balance
console.log(`Wallet Public Key : ${wallet.publicKey} \nWallet Balance : ${balance}` )
client.disconnect();
}
connectToLedger()
Code Breakdown
fundWallet()
on line 10
we call the asynchronous fundWallet
method from our previous client object.
this function returns an object containing a Wallet
object, and within the Wallet
object there are details such as its publicKey
andprivateKey
and seed phrase.
Alongside the Wallet
object we have its balance.
In essence the function:
generates a new wallet.
-
Initiates a faucet transaction that sends 100 test XRP to the wallet’s public address via a WebSocket connection. This triggers a sequence of interactions between operation and issuer accounts on the test network.
Why
FundWallet
?As we mentioned earlier, this is a testing environment, so we are able to utilize functions such as
fundWallet
to easily retrieve a dispensable wallet with dispensable funds, however you are not allowed to usefundWallet()
on themainet
, you need to generate a wallet and send it funds via an external wallet with xrp or aDigital Exchange
. See [[#Generating A wallet on the main-net.]] for more details.
Ex
{
wallet: Wallet {
publicKey: 'kgphikthpotpruktf325235rpkopkwpokgwopenr12359322f',
privateKey: 'idjfi0wufj14faafaaffff110124812401gsafawfwafa4',
classicAddress: 'idjfi0wufj14afafawawfafaafawfawfaw95329595f',
seed: 'afiafjawifjawoifaw'
},
balance: 100
}
Querying data from a ledger.
client.request()
This method, is used for querying various types of ledger data, Afterall a ledger is a data structure and thus can be used as database. This method accepts a object map for query configuration. The current configuration we are using is:
let onChainData= await client.request({
command:"account_info", //The type of data we want to querry
account:wallet.address, // The specific account or adress we want to querry
ledger_index:"validated", // Most recently validated ledger index
});
console.log(`Balance In Drops : ${onChainData.result.account_data.Balance}`)
Config Key | Description |
---|---|
command |
The query type |
account |
The account we desire to query in this context were using the address of the recently generated wallet |
ledger_index |
A specific ledger selector - in this context were selecting the most recently validated ledger index containing information surrounding this address. |
Once the request is fulfilled it should return an object similar to this one:
{
api_version: 2,
id: 6,
result: {
account_data: {
Account: 'fafawfawwfajifjawifawjfawf',
Balance: '100000000',
//.... Ledger Data
},
account_flags: {
//.... Ledger Data
//.... Ledger Data
},
ledger_hash: 'faiwhfa9w0fhaw0f9ahf90awhf90awfhaw90fhawf0aw9ha9fhaw',
ledger_index: 67999825,
validated: true
},
type: 'response'
}
Final Source Code
import {Client} from 'xrpl'
async function connectToLedger(){
//Instantiate the object with xrpl address as an argument and store its reference.
const client = new Client('wss://s.altnet.rippletest.net:51233/')
//Connect Via the connect function
await client.connect()
//Instantiating A wallet with fundWallet and storing its reference.
let testFund = await client.fundWallet();
//Waiting for promise and storing the wallet property
let wallet = (await testFund).wallet
//Waiting for promise and storing the wallet balance property
let balance = (await testFund).balance
console.log(`Wallet Public Key : ${wallet.publicKey} \nWallet Balance : ${balance}` )
//Configuring querry via object
let onChainData= await client.request({
command:"account_info", //The type of data we want to querry
account:wallet.address, // The specific account or adress we want to querry
ledger_index:"validated", // Most recently validated ledger index
});
console.log(`Balance In Drops : ${onChainData.result.account_data.Balance}`)
client.disconnect(); // A gracefull disconnect
}
connectToLedger()
Generating a wallet and account on the main-net.
You can generate and receive its private and public key but it or any related data wont be on the ledger until a transaction its made to the wallet address.
Generating A Wallet
import {Wallet} from xrpl
let wallet = Wallet.generate();
Generate a wallet from seed
If you want to generate a wallet address based on a seed phrase:
import {Wallet} from xrpl
let wallet = Wallet.fromSeed("AFNAK9310913JFSAANFJFn")