Debug Like a Pro with 'git bisect'
Most programmers spent hours checking git commits to identify which commit introduced the bug. It’s frustrating. But instead of manually checking commit after commit, Git has a built-in tool that saves your time using Binary Search — git bisect! Let me walk you through it. It’s easier than you think. Step 1: Start Bisecting First, tell Git you want to start looking for the bad commit: git bisect start That’s it! Now Git knows you’re about to go on a debugging hunt. Step 2: Mark a "Good" and "Bad" Commit Now, we need to give Git some context. Find a past commit where everything worked fine and mark it as good: git bisect good Then, mark the commit where the bug appeared as bad: git bisect bad Don’t know the exact good commit? No worries—just pick an older one where you think things were stable. Step 3: Let Git Do the Heavy Lifting Now comes the magic! Git will check out a commit in the middle of the range. You test it and tell Git whether the bug is there or not. If the bug isn’t there, run: git bisect good If the bug exists, run: git bisect bad Git keeps narrowing it down until it finds the exact commit that introduced the issue. Step 4: End Bisect & Fix the Bug Once Git pinpoints the problematic commit, reset everything back to normal: git bisect reset Then, take a look at the commit, figure out what went wrong, and fix the bug at its source! BTW if you have a test script that can detect the bug automatically, you don’t even have to manually test each commit! Just run: git bisect run ./test-script.sh Git will keep running the script until it finds the bad commit — completely hands-free debugging! If you haven’t tried git bisect yet, give it a shot! It might just become your new favorite debugging tool. Don't forget to share this. Might help someone in your network.
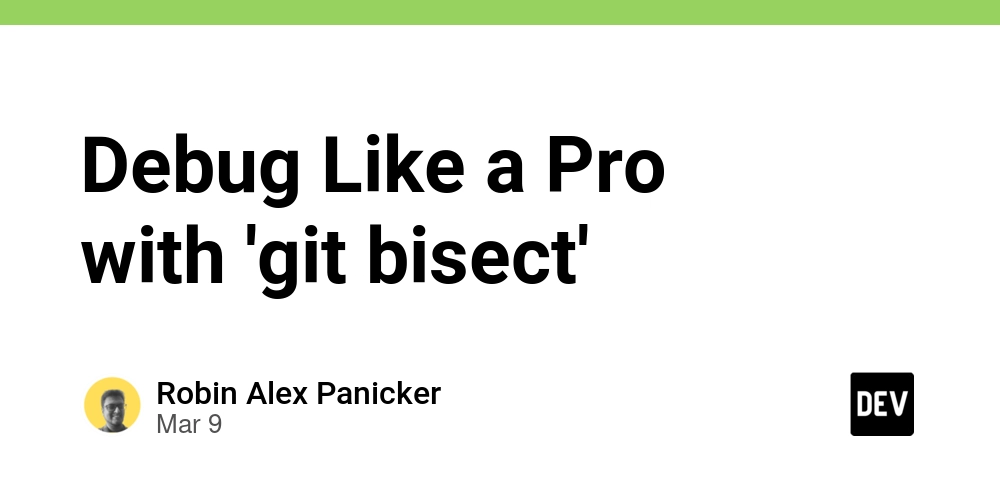
Most programmers spent hours checking git commits to identify which commit introduced the bug. It’s frustrating. But instead of manually checking commit after commit, Git has a built-in tool that saves your time using Binary Search — git bisect!
Let me walk you through it. It’s easier than you think.
Step 1: Start Bisecting
First, tell Git you want to start looking for the bad commit:
git bisect start
That’s it! Now Git knows you’re about to go on a debugging hunt.
Step 2: Mark a "Good" and "Bad" Commit
Now, we need to give Git some context. Find a past commit where everything worked fine and mark it as good:
git bisect good
Then, mark the commit where the bug appeared as bad:
git bisect bad
Don’t know the exact good commit? No worries—just pick an older one where you think things were stable.
Step 3: Let Git Do the Heavy Lifting
Now comes the magic! Git will check out a commit in the middle of the range. You test it and tell Git whether the bug is there or not.
If the bug isn’t there, run:
git bisect good
If the bug exists, run:
git bisect bad
Git keeps narrowing it down until it finds the exact commit that introduced the issue.
Step 4: End Bisect & Fix the Bug
Once Git pinpoints the problematic commit, reset everything back to normal:
git bisect reset
Then, take a look at the commit, figure out what went wrong, and fix the bug at its source!
BTW if you have a test script that can detect the bug automatically, you don’t even have to manually test each commit! Just run:
git bisect run ./test-script.sh
Git will keep running the script until it finds the bad commit — completely hands-free debugging!
If you haven’t tried git bisect yet, give it a shot! It might just become your new favorite debugging tool.
Don't forget to share this. Might help someone in your network.