Day 27-Java collections:
Collections of java: ==> Java Collections are a framework that provides a set of classes and interfaces to store and manipulate data in Java. ==> The Java Collections Framework is a part of the java.util package and is designed to handle groups of objects. There are two main types of collections in Java: ==> Collection Interface ==> Map Interface 1.Collection Interface: List: ==> An ordered collection that can contain duplicate elements. ArrayList: ==> A dynamic array that grows as needed. Elements can be accessed by index. LinkedList: ==> Doubly linked list. It allows for fast insertions/deletions at both ends but is slower for random access. Set: ==> A collection that does not allow duplicates. HashSet: ==> No duplicates and does not maintain any order. LinkedHashSet: ==> No duplicates, but it maintains insertion order. TreeSet: ==> No duplicates, and it orders elements according to their natural ordering or a comparator. Queue: ==> A collection used for holding elements prior to processing. PriorityQueue: ==> A queue where elements are processed in order of their priority. LinkedList: ==> Implements both List and Queue interfaces. 2.Map Interface ==> This is not a true collection but a structure that holds key-value pairs, where each key maps to exactly one value. HashMap: ==> A map that does not maintain any order of keys. LinkedHashMap: ==> A map that maintains the insertion order of keys. TreeMap: ==> A map that maintains keys in sorted order. Example: import java.util.*; public class CollectionExample { public static void main(String[] args) { // List Example (Allow Duplicates) List list = new ArrayList(); list.add("Java"); list.add("Python"); list.add("Java"); System.out.println("List: " + list); // Set Example (No Duplicates) Set set = new HashSet(); set.add("Java"); set.add("C++"); set.add("Java"); System.out.println("Set (No Duplicates): " + set); // Map Example (Key-Value pairs) Map map = new HashMap(); map.put("Java", 8); map.put("Python", 3); map.put("JavaScript", 6); System.out.println("Map: " + map); // Queue Example Queue queue = new LinkedList(); queue.add("First"); queue.add("Second"); queue.add("Third"); System.out.println("Queue: " + queue); } } Output: List: [Java, Python, Java] Set (No Duplicates): [Java, C++] Map: {Java=8, Python=3, JavaScript=6} Queue: [First, Second, Third]
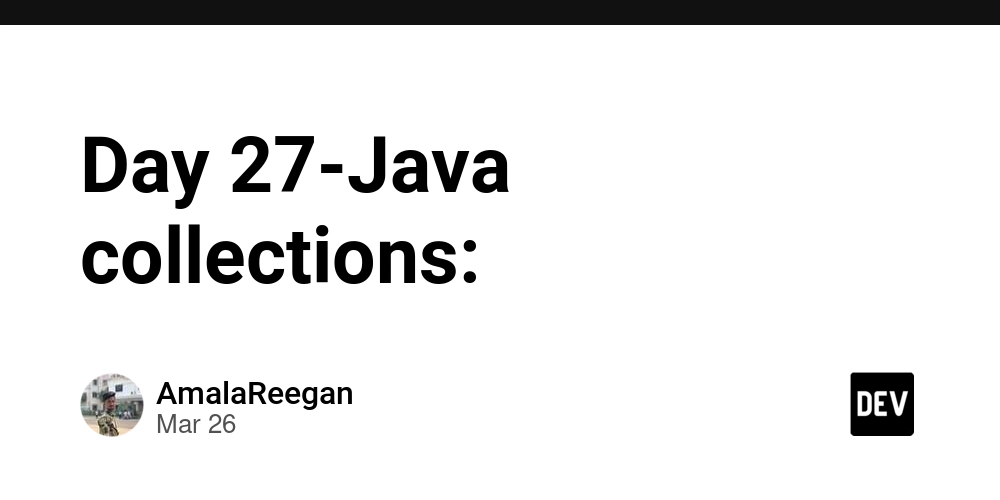
Collections of java:
==> Java Collections are a framework that provides a set of classes and interfaces to store and manipulate data in Java.
==> The Java Collections Framework is a part of the java.util package and is designed to handle groups of objects.
There are two main types of collections in Java:
==> Collection Interface
==> Map Interface
1.Collection Interface:
List:
==> An ordered collection that can contain duplicate elements.
ArrayList:
==> A dynamic array that grows as needed. Elements can be accessed by index.
LinkedList:
==> Doubly linked list. It allows for fast insertions/deletions at both ends but is slower for random access.
Set:
==> A collection that does not allow duplicates.
HashSet:
==> No duplicates and does not maintain any order.
LinkedHashSet:
==> No duplicates, but it maintains insertion order.
TreeSet:
==> No duplicates, and it orders elements according to their natural ordering or a comparator.
Queue:
==> A collection used for holding elements prior to processing.
PriorityQueue:
==> A queue where elements are processed in order of their priority.
LinkedList:
==> Implements both List and Queue interfaces.
2.Map Interface
==> This is not a true collection but a structure that holds key-value pairs, where each key maps to exactly one value.
HashMap:
==> A map that does not maintain any order of keys.
LinkedHashMap:
==> A map that maintains the insertion order of keys.
TreeMap:
==> A map that maintains keys in sorted order.
Example:
import java.util.*;
public class CollectionExample {
public static void main(String[] args) {
// List Example (Allow Duplicates)
List list = new ArrayList<>();
list.add("Java");
list.add("Python");
list.add("Java");
System.out.println("List: " + list);
// Set Example (No Duplicates)
Set set = new HashSet<>();
set.add("Java");
set.add("C++");
set.add("Java");
System.out.println("Set (No Duplicates): " + set);
// Map Example (Key-Value pairs)
Map map = new HashMap<>();
map.put("Java", 8);
map.put("Python", 3);
map.put("JavaScript", 6);
System.out.println("Map: " + map);
// Queue Example
Queue queue = new LinkedList<>();
queue.add("First");
queue.add("Second");
queue.add("Third");
System.out.println("Queue: " + queue);
}
}
Output:
List: [Java, Python, Java]
Set (No Duplicates): [Java, C++]
Map: {Java=8, Python=3, JavaScript=6}
Queue: [First, Second, Third]