Day 2: Looping
What is looping in java?#$ Looping in Java means executing a set of statements multiple times based on a condition. It helps to avoid writing the same code again and again. Java supports three main types of loops: for loop while loop do-while loop Each loop runs repeatedly until the given condition becomes false. We use looping in Java to.#$ 1.Reduce code repetition– avoid writing the same code many times. Perform tasks multiple times – like printing, calculations, or reading data. Make programs efficient and shorter – by handling repetitive tasks automatically. $ when to use each type of loop in Java: for loop – When you know the number of times to repeat. Example: Printing numbers from 1 to 10. while loop – When you don’t know how many times, but repeat while a condition is true. Example: Reading user input until they enter 'exit'. do-while loop – When you want to run at least once, and then check the condition. Example: Showing a menu at least one time. while loop in Java The while loop repeats a block of code as long as the condition is true. Syntax: while (condition) { // code to execute } Example: int i = 1; while (i
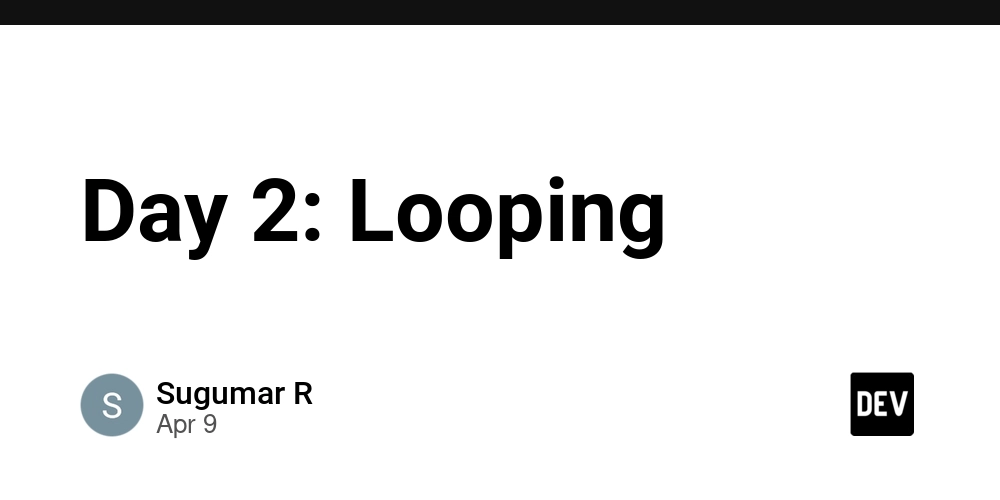
What is looping in java?#$
Looping in Java means executing a set of statements multiple times based on a condition. It helps to avoid writing the same code again and again. Java supports three main types of loops:
for loop
while loop
do-while loop
Each loop runs repeatedly until the given condition becomes false.
We use looping in Java to.#$
1.Reduce code repetition– avoid writing the same code many times.
Perform tasks multiple times – like printing, calculations, or reading data.
Make programs efficient and shorter – by handling repetitive tasks automatically.
$ when to use each type of loop in Java:
for loop – When you know the number of times to repeat.
Example: Printing numbers from 1 to 10.while loop – When you don’t know how many times, but repeat while a condition is true.
Example: Reading user input until they enter 'exit'.do-while loop – When you want to run at least once, and then check the condition.
Example: Showing a menu at least one time.
while loop in Java
The while loop repeats a block of code as long as the condition is true.
Syntax:
while (condition) {
// code to execute
}
Example:
int i = 1;
while (i <= 5) {
System.out.println(i);
i++;
}
Output:
1
2
3
4
5
Use while loop when you don't know how many times the loop should run, and it depends on a condition.