Day-14:Static and non static method
*Static Variables in Java * Static variables in Java are class-level variables that are shared among all objects of a class. They are declared using the "static" keyword and are stored in the class area. Because they are shared, their values can be accessed and modified by any object of the class. They are also known as class variables. Static variables are typically used to represent class-level data, such as the number of instances of a class that have been created, the maximum value allowed for a particular property, or a default value for a property. [TBD] Non-Static Variables in Java Non-static variables in Java are also known as instance variables. They are defined within a class but not marked as static. Unlike static variables, non-static variables are associated with an instance of a class and are stored in heap memory[TBD]. This means that each object of the class will have its own copy of the non-static variables. Non-static variables are used to represent object-level data and are typically used to store data that is unique to each instance of a class. They can be accessed and modified using object references. class BankAccount { private int accountNumber; private String accountHolderName; private double balance; public BankAccount(int accountNumber, String accountHolderName, double balance) { this.accountNumber = accountNumber; this.accountHolderName = accountHolderName; this.balance = balance; } public int getAccountNumber() { return accountNumber; } public String getAccountHolderName() { return accountHolderName; } public double getBalance() { return balance; } } class Main{ public static void main(String args[]){ BankAccount account1 = new BankAccount(12345, "John Doe", 5000.0); BankAccount account2 = new BankAccount(67890, "Jane Doe", 6000.0); System.out.println("Account Number: " + account1.getAccountNumber()); System.out.println("Account Holder Name: " + account1.getAccountHolderName()); System.out.println("Balance: $" + account1.getBalance()); System.out.println("Account Number: " + account2.getAccountNumber()); System.out.println("Account Holder Name: " + account2.getAccountHolderName()); System.out.println("Balance: $" + account2.getBalance()); } } `Output: Account Number: 12345 Account Holder Name: John Doe Balance: $5000.0 Account Number: 67890 Account Holder Name: Jane Doe Balance: $6000.0` Static method. • A static method belongs to the class rather than the object of a class. • A static method can be invoked without the need for creating an instance of a class. • A static method can access static data member and can change the value of it.[TBD] class Test { // static method public static int sum(int a, int b) { return a + b; } class Test { // static method public static int sum(int a, int b) { return a + b; } class Main { public static void main(String[] args) { int n = 3, m = 6; // call the static method int s = Test.sum(n, m); System.out.print("sum is = " + s); } } Output: sum is = 9 Non-Static methods • A non-static method does not have the keyword static before the name of the method. • A non-static method belongs to an object of the class and you have to create an instance of the class to access it. • Non-static methods can access any static method and any static variable without creating an instance of the class.[TBD] class Test{ // static method public int sum(int a, int b) { return a + b; } } class Main { public static void main(String[] args) { int n = 3, m = 6; Test g = new Test(); int s = g.sum(n, m); // call the non-static method System.out.print("sum is = " + s); } } Output: sum is = 9 task A Two's Complement Number is defined as a binary number system where the most significant bit represents the sign, allowing for both positive and negative numbers to be represented 16-bit Unicode or Unicode Transformation Format (UTF-16) is a method of encoding character data, capable of encoding 1,112,064 possible characters in Unicode.[TBD] Reference link (https://pd.daffodilvarsity.edu.bd/course/material/oop-lecture-6-173/pdf_content) (https://www.prepbytes.com/blog/java/difference-between-static-and-non-static-variables-in-java/)
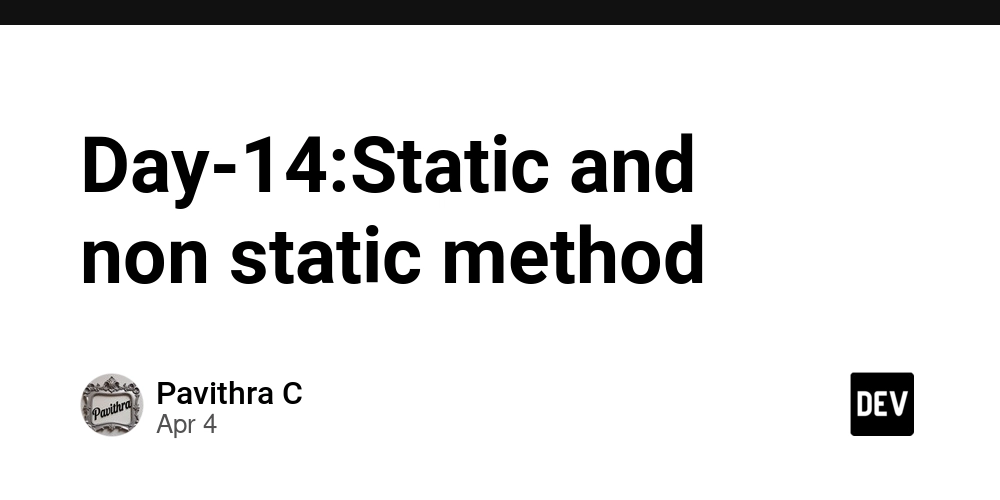
*Static Variables in Java *
Static variables in Java are class-level variables that are shared among all objects of a class. They are declared using the "static" keyword and are stored in the class area. Because they are shared, their values can be accessed and modified by any object of the class. They are also known as class variables.
Static variables are typically used to represent class-level data, such as the number of instances of a class that have been created, the maximum value allowed for a particular property, or a default value for a property. [TBD]
Non-Static Variables in Java
Non-static variables in Java are also known as instance variables. They are defined within a class but not marked as static. Unlike static variables, non-static variables are associated with an instance of a class and are stored in heap memory[TBD]. This means that each object of the class will have its own copy of the non-static variables.
Non-static variables are used to represent object-level data and are typically used to store data that is unique to each instance of a class. They can be accessed and modified using object references.
class BankAccount {
private int accountNumber;
private String accountHolderName;
private double balance;
public BankAccount(int accountNumber, String accountHolderName, double balance) {
this.accountNumber = accountNumber;
this.accountHolderName = accountHolderName;
this.balance = balance;
}
public int getAccountNumber() {
return accountNumber;
}
public String getAccountHolderName() {
return accountHolderName;
}
public double getBalance() {
return balance;
}
}
class Main{
public static void main(String args[]){
BankAccount account1 = new BankAccount(12345, "John Doe", 5000.0);
BankAccount account2 = new BankAccount(67890, "Jane Doe", 6000.0);
System.out.println("Account Number: " + account1.getAccountNumber());
System.out.println("Account Holder Name: " + account1.getAccountHolderName());
System.out.println("Balance: $" + account1.getBalance());
System.out.println("Account Number: " + account2.getAccountNumber());
System.out.println("Account Holder Name: " + account2.getAccountHolderName());
System.out.println("Balance: $" + account2.getBalance());
}
}
`Output:
Account Number: 12345
Account Holder Name: John Doe
Balance: $5000.0
Account Number: 67890
Account Holder Name: Jane Doe
Balance: $6000.0`
Static method.
• A static method belongs to the class rather than the object of a class.
• A static method can be invoked without the need for creating an instance of a
class.
• A static method can access static data member and can change the value of it.[TBD]
class Test {
// static method
public static int sum(int a, int b)
{
return a + b;
}
class Test {
// static method
public static int sum(int a, int b)
{
return a + b;
}
class Main {
public static void main(String[] args)
{
int n = 3, m = 6;
// call the static method
int s = Test.sum(n, m);
System.out.print("sum is = " + s);
}
}
Output:
sum is = 9
Non-Static methods
• A non-static method does not have the keyword static before the name of
the method.
• A non-static method belongs to an object of the class and you have to
create an instance of the class to access it.
• Non-static methods can access any static method and any static variable
without creating an instance of the class.[TBD]
class Test{
// static method
public int sum(int a, int b)
{
return a + b;
}
}
class Main {
public static void main(String[] args)
{
int n = 3, m = 6;
Test g = new Test();
int s = g.sum(n, m);
// call the non-static method
System.out.print("sum is = " + s);
}
}
Output:
sum is = 9
task
A Two's Complement Number is defined as a binary number system where the most significant bit represents the sign, allowing for both positive and negative numbers to be represented
16-bit Unicode or Unicode Transformation Format (UTF-16) is a method of encoding character data, capable of encoding 1,112,064 possible characters in Unicode.[TBD]
Reference link
(https://pd.daffodilvarsity.edu.bd/course/material/oop-lecture-6-173/pdf_content)
(https://www.prepbytes.com/blog/java/difference-between-static-and-non-static-variables-in-java/)