Creating apps for Raspberry Pi - Part 2
Raspberry Pi runs on ARM Linux, which makes things a bit trickier than an average x86 Linux PC, but yes, it's absolutely possible to run Flutter desktop apps on it. For installing a desktop Linux app, you need a compiled Linux binary along with assets. You may need to package your app as a .deb, .AppImage, or similar format for distribution. We will cover how to create a debian bundle for distribution. Creating a Debian (.deb) bundle Step 1 Now, let us start with the process of creating the builds for distribution. Create a release specification for our Debian build and add it to the root of the Flutter project. The contents of the distribute_options.yaml file is provided below: output: dist/ releases: - name: dev jobs: - name: release-dev-linux-deb package: platform: linux target: deb build_args: enable-experiment: records The name of the release is specified as dev and inside it there can be multiple jobs, like to build for linux, android, ios, etc. We created a single job with name release-dev-linux-deb where we specified our platform as linux and target as deb. Additional command line arguments or compile time constants can also be specified via build_args like in this case we provided records value to the enable-experiment flag to indicate that the experimental Dart records feature is being used. Step 2 Create a new directory named packaging inside the linux folder in the project home. This folder will contain the config files used for our linux builds. As we want to create a debian build, create a new folder named deb inside the packaging folder. Now, create make_config.yaml inside this folder. This file will be used to specify the details to build the debian bundle. The final path of this file is: /path/to/project/linux/packaging/deb/make_config.yaml In make_config.yaml, we can specify all details that are required to create the .deb build like: The display name for the application The name of the package (lowercase, nospace — actual name which can be executed via terminal) Maintainer information App icon path Metadata such as app category & keywords External dependencies Other required information used for creating the build. A sample make_config.yaml spec is provided below: display_name: Planner package_name: planner maintainer: name: abc xyz email: abc.xyz@gmail.com priority: optional section: x11 installed_size: 15700 dependencies: - mpv essential: false icon: linux/assets/planner.png postuninstall_scripts: - echo "Sorry to see you go." keywords: - API - API Client generic_name: API Client categories: - Development - Utility startup_notify: true Step 3 Your Flutter app might be using packages that have external dependencies that must be specified in the make_config.yaml file as shown below: These dependencies need to be installed on the end users system in order to use the application. To figure out these dependencies, check the pub dev page of packages that have been used in the project. Developers usually specify the dependencies in the readme file. Step 4 Run the following flutter_distributor command to generate the build. flutter_distributor release --name=dev --jobs=release-dev-linux-deb Step 5 Once the build is successfully completed, the location of the bundle will be displayed. Install the application using the below command: sudo apt install ./app.deb Optional: Add Pi Hardware Integration If you want to interact with GPIO, camera, etc., you can: Use platform channels to call Python or C code Use FFI (Dart’s foreign function interface) Final Tip: Performance Enable GPU acceleration if you’re using a windowed environment (e.g., on Pi 4 with KMS or DRM) Use the --release build for best speed Raspberry Pi is known to heat up quickly. Active cooling helps!
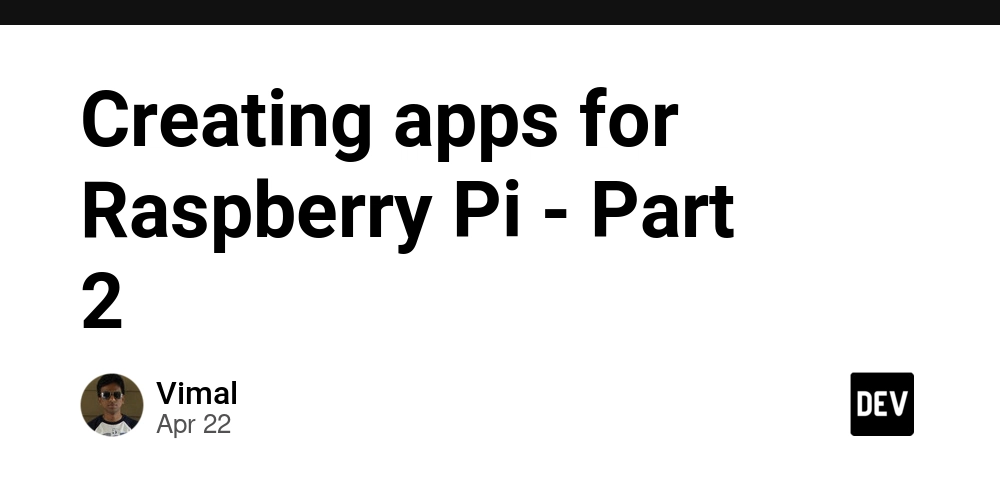
Raspberry Pi runs on ARM Linux, which makes things a bit trickier than an average x86 Linux PC, but yes, it's absolutely possible to run Flutter desktop apps on it.
For installing a desktop Linux app, you need a compiled Linux binary along with assets. You may need to package your app as a .deb, .AppImage, or similar format for distribution. We will cover how to create a debian bundle for distribution.
Creating a Debian (.deb) bundle
Step 1
Now, let us start with the process of creating the builds for distribution.
Create a release specification for our Debian build and add it to the root of the Flutter project. The contents of the distribute_options.yaml
file is provided below:
output: dist/
releases:
- name: dev
jobs:
- name: release-dev-linux-deb
package:
platform: linux
target: deb
build_args:
enable-experiment: records
The name of the release is specified as dev and inside it there can be multiple jobs, like to build for linux, android, ios, etc.
We created a single job with name release-dev-linux-deb where we specified our platform as linux and target as deb. Additional command line arguments or compile time constants can also be specified via build_args like in this case we provided records value to the enable-experiment flag to indicate that the experimental Dart records feature is being used.
Step 2
Create a new directory named packaging
inside the linux folder in the project home. This folder will contain the config files used for our linux builds.
As we want to create a debian build, create a new folder named deb
inside the packaging folder.
Now, create make_config.yaml
inside this folder. This file will be used to specify the details to build the debian bundle.
The final path of this file is:
/path/to/project/linux/packaging/deb/make_config.yaml
In make_config.yaml
, we can specify all details that are required to create the .deb build like:
- The display name for the application
- The name of the package (lowercase, nospace — actual name which can be executed via terminal)
- Maintainer information
- App icon path
- Metadata such as app category & keywords
- External dependencies
- Other required information used for creating the build.
A sample make_config.yaml spec is provided below:
display_name: Planner
package_name: planner
maintainer:
name: abc xyz
email: abc.xyz@gmail.com
priority: optional
section: x11
installed_size: 15700
dependencies:
- mpv
essential: false
icon: linux/assets/planner.png
postuninstall_scripts:
- echo "Sorry to see you go."
keywords:
- API
- API Client
generic_name: API Client
categories:
- Development
- Utility
startup_notify: true
Step 3
Your Flutter app might be using packages that have external dependencies that must be specified in the make_config.yaml
file as shown below:
These dependencies need to be installed on the end users system in order to use the application. To figure out these dependencies, check the pub dev page of packages that have been used in the project. Developers usually specify the dependencies in the readme file.
Step 4
Run the following flutter_distributor command to generate the build.
flutter_distributor release --name=dev --jobs=release-dev-linux-deb
Step 5
Once the build is successfully completed, the location of the bundle will be displayed. Install the application using the below command:
sudo apt install ./app.deb
Optional: Add Pi Hardware Integration
If you want to interact with GPIO, camera, etc., you can:
- Use platform channels to call Python or C code
- Use FFI (Dart’s foreign function interface)
Final Tip: Performance
Enable GPU acceleration if you’re using a windowed environment (e.g., on Pi 4 with KMS or DRM)
Use the --release
build for best speed
Raspberry Pi is known to heat up quickly. Active cooling helps!