How to Build an AI Voice Assistant Using Node.js and OpenAI API
Voice isn’t the future — it’s already here. And with the rise of conversational AI, building your own voice assistant has never been more accessible. With over a decade in tech content creation, I've seen trends come and go. But voice interfaces and AI-powered assistants? They're here to stay. From Siri to Alexa, we’re now living in a world where talking to machines feels natural — even expected. So, what if you could build your own AI voice assistant using just Node.js and the OpenAI API? Let me walk you through the why, the how, and the code — step by step. What You’ll Need to build an AI Voice Assistant? Here’s what you need to get started: Node.js installed A free or paid OpenAI API key A microphone and speakers (for testing) Optional: Speech-to-text (like Whisper API) and text-to-speech (like Google TTS) Step-by-Step: Building Your AI Assistant 1. Set Up the Project mkdir voice-assistant cd voice-assistant npm init -y npm install openai axios dotenv Create a .env file: OPENAI_API_KEY=your_openai_api_key_here 2. Capture User Voice Input (STT) Use any speech-to-text tool like Whisper API or browser-based audio input to capture user speech and convert it to text. const userInput = "What's the weather like today?"; 3. Send to OpenAI GPT-4 const { Configuration, OpenAIApi } = require('openai'); require('dotenv').config(); const configuration = new Configuration({ apiKey: process.env.OPENAI_API_KEY, }); const openai = new OpenAIApi(configuration); async function getAIResponse(prompt) { const response = await openai.createChatCompletion({ model: 'gpt-4', messages: [{ role: 'user', content: prompt }], }); return response.data.choices[0].message.content; } 4. Convert Text Response to Voice (TTS) Use a text-to-speech engine like Google Cloud TTS, Amazon Polly, or even ElevenLabs. // Pseudo-code const speak = (text) => { // Send to TTS engine and play audio }; Final Thoughts Building a voice assistant in 2025 isn’t just a weekend project — it’s a gateway into the next generation of human-computer interaction. Whether you’re a developer, hobbyist, or product builder, now’s the perfect time to experiment with Node.js + OpenAI and create something truly interactive. The future is conversational. Are you building it?
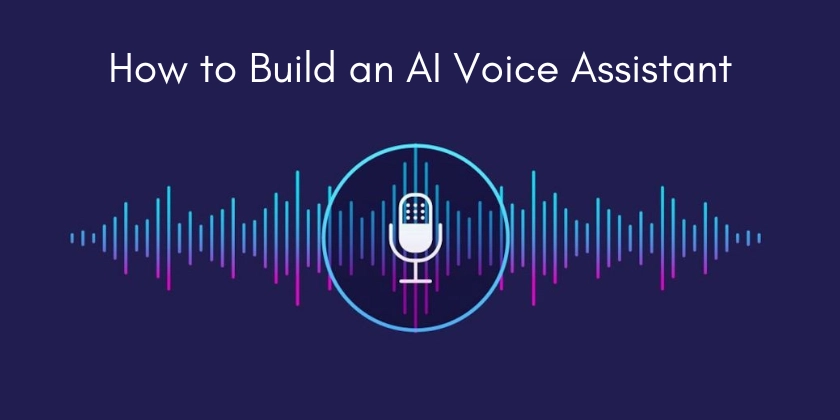
Voice isn’t the future — it’s already here. And with the rise of conversational AI, building your own voice assistant has never been more accessible.
With over a decade in tech content creation, I've seen trends come and go. But voice interfaces and AI-powered assistants? They're here to stay. From Siri to Alexa, we’re now living in a world where talking to machines feels natural — even expected.
So, what if you could build your own AI voice assistant using just Node.js and the OpenAI API?
Let me walk you through the why, the how, and the code — step by step.
What You’ll Need to build an AI Voice Assistant?
Here’s what you need to get started:
- Node.js installed
- A free or paid OpenAI API key
- A microphone and speakers (for testing)
- Optional: Speech-to-text (like Whisper API) and text-to-speech (like Google TTS)
Step-by-Step: Building Your AI Assistant
1. Set Up the Project
mkdir voice-assistant
cd voice-assistant
npm init -y
npm install openai axios dotenv
Create a .env file:
OPENAI_API_KEY=your_openai_api_key_here
2. Capture User Voice Input (STT)
Use any speech-to-text tool like Whisper API or browser-based audio input to capture user speech and convert it to text.
const userInput = "What's the weather like today?";
3. Send to OpenAI GPT-4
const { Configuration, OpenAIApi } = require('openai');
require('dotenv').config();
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
async function getAIResponse(prompt) {
const response = await openai.createChatCompletion({
model: 'gpt-4',
messages: [{ role: 'user', content: prompt }],
});
return response.data.choices[0].message.content;
}
4. Convert Text Response to Voice (TTS)
Use a text-to-speech engine like Google Cloud TTS, Amazon Polly, or even ElevenLabs.
// Pseudo-code
const speak = (text) => {
// Send to TTS engine and play audio
};
Final Thoughts
Building a voice assistant in 2025 isn’t just a weekend project — it’s a gateway into the next generation of human-computer interaction.
Whether you’re a developer, hobbyist, or product builder, now’s the perfect time to experiment with Node.js + OpenAI and create something truly interactive.
The future is conversational. Are you building it?