Database Schema Design for Scalability: Best Practices, Techniques, and Real-World Examples for High-Performance Systems
Table of Contents Introduction to Database Schema Design for Scalability Normalization Principles and Their Impact on Scalability Indexing Strategies for Scalable Database Performance Partitioning and Sharding Techniques for Database Scalability Data Types and Constraints Selection for Scalable Database Schemas Schema Versioning and Migration Strategies for Scalable Databases Real-World Examples of Scalable Database Schemas Summary of Key Scalability Principles for Database Schema Design Introduction to Database Schema Design for Scalability Database schema design forms the foundation of any application's data architecture. A well-designed schema not only ensures data integrity and reduces redundancy but also plays a crucial role in determining how well your database will scale as your application grows. Scalability in database terms refers to the ability of a database system to handle increasing amounts of data and user load without compromising performance. When designing database schemas with scalability in mind, we need to consider both vertical scaling (adding more resources to a single server) and horizontal scaling (distributing the database across multiple servers). While hardware improvements can temporarily alleviate performance issues, a poorly designed schema will eventually become a bottleneck regardless of the hardware it runs on. The importance of proper schema design cannot be overstated. A schema that works perfectly well for a small application with a few thousand records might completely fall apart when that same application grows to millions of records and thousands of concurrent users. Retrofitting scalability into an existing database is significantly more challenging and risky than building it in from the start. Several key principles guide scalable database design. These include proper normalization, strategic denormalization when necessary, effective indexing, intelligent partitioning and sharding, appropriate data type selection, and forward-thinking schema versioning strategies. Each of these principles involves trade-offs that must be carefully considered in the context of your specific application requirements. It's also worth noting that different database types (relational, document-oriented, graph, time-series, etc.) have different scaling characteristics and design considerations. While this guide will primarily focus on relational database design principles, many concepts apply across database types with appropriate adaptations. Throughout this comprehensive guide, we'll explore each of these principles in depth, providing practical examples and real-world scenarios to illustrate how they can be applied to create database schemas that scale effectively with your application's growth. By the end, you'll have a solid understanding of how to design database schemas that can gracefully handle increasing data volumes and user loads without requiring complete redesigns as your application evolves. Normalization Principles and Their Impact on Scalability Normalization is a fundamental concept in relational database design that involves organizing data to reduce redundancy and improve data integrity. The process follows a series of normal forms, each building upon the previous one to eliminate various types of anomalies. While normalization is essential for data consistency, its relationship with scalability is nuanced and requires careful consideration. The Five Normal Forms First Normal Form (1NF) The first normal form requires that each column contain atomic (indivisible) values, and that each record be unique. This means eliminating repeating groups and ensuring that each cell contains a single value. For example, instead of storing multiple phone numbers in a single field, you would create separate records for each phone number. In terms of scalability, 1NF is a basic requirement. Non-atomic data makes querying and indexing inefficient, which becomes increasingly problematic as data volumes grow. Databases with non-atomic values often require application-level processing, which doesn't scale well with increasing data. Second Normal Form (2NF) The second normal form builds on 1NF by requiring that all non-key attributes be fully functionally dependent on the primary key. This means removing partial dependencies where an attribute depends on only part of a composite key. From a scalability perspective, 2NF helps by reducing update anomalies. When data is duplicated across rows due to partial dependencies, updates require multiple row changes, increasing the likelihood of contention and locks as concurrency increases. This becomes a significant bottleneck in high-throughput systems. Third Normal Form (3NF) The third normal form extends 2NF by eliminating transitive dependencies, where non-key attributes depend on other non-key attributes. This typically involves creating separate tables for
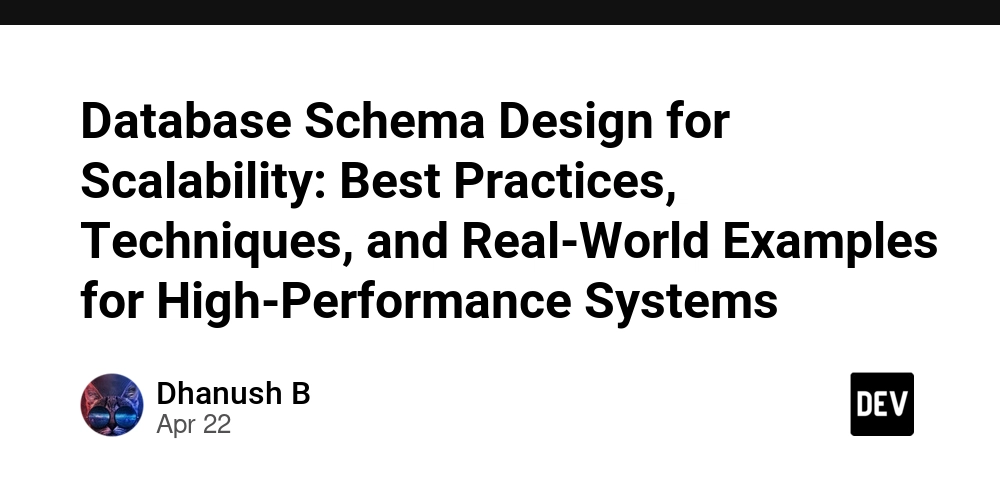
Table of Contents
- Introduction to Database Schema Design for Scalability
- Normalization Principles and Their Impact on Scalability
- Indexing Strategies for Scalable Database Performance
- Partitioning and Sharding Techniques for Database Scalability
- Data Types and Constraints Selection for Scalable Database Schemas
- Schema Versioning and Migration Strategies for Scalable Databases
- Real-World Examples of Scalable Database Schemas
- Summary of Key Scalability Principles for Database Schema Design
Introduction to Database Schema Design for Scalability
Database schema design forms the foundation of any application's data architecture. A well-designed schema not only ensures data integrity and reduces redundancy but also plays a crucial role in determining how well your database will scale as your application grows. Scalability in database terms refers to the ability of a database system to handle increasing amounts of data and user load without compromising performance.
When designing database schemas with scalability in mind, we need to consider both vertical scaling (adding more resources to a single server) and horizontal scaling (distributing the database across multiple servers). While hardware improvements can temporarily alleviate performance issues, a poorly designed schema will eventually become a bottleneck regardless of the hardware it runs on.
The importance of proper schema design cannot be overstated. A schema that works perfectly well for a small application with a few thousand records might completely fall apart when that same application grows to millions of records and thousands of concurrent users. Retrofitting scalability into an existing database is significantly more challenging and risky than building it in from the start.
Several key principles guide scalable database design. These include proper normalization, strategic denormalization when necessary, effective indexing, intelligent partitioning and sharding, appropriate data type selection, and forward-thinking schema versioning strategies. Each of these principles involves trade-offs that must be carefully considered in the context of your specific application requirements.
It's also worth noting that different database types (relational, document-oriented, graph, time-series, etc.) have different scaling characteristics and design considerations. While this guide will primarily focus on relational database design principles, many concepts apply across database types with appropriate adaptations.
Throughout this comprehensive guide, we'll explore each of these principles in depth, providing practical examples and real-world scenarios to illustrate how they can be applied to create database schemas that scale effectively with your application's growth. By the end, you'll have a solid understanding of how to design database schemas that can gracefully handle increasing data volumes and user loads without requiring complete redesigns as your application evolves.
Normalization Principles and Their Impact on Scalability
Normalization is a fundamental concept in relational database design that involves organizing data to reduce redundancy and improve data integrity. The process follows a series of normal forms, each building upon the previous one to eliminate various types of anomalies. While normalization is essential for data consistency, its relationship with scalability is nuanced and requires careful consideration.
The Five Normal Forms
First Normal Form (1NF)
The first normal form requires that each column contain atomic (indivisible) values, and that each record be unique. This means eliminating repeating groups and ensuring that each cell contains a single value. For example, instead of storing multiple phone numbers in a single field, you would create separate records for each phone number.
In terms of scalability, 1NF is a basic requirement. Non-atomic data makes querying and indexing inefficient, which becomes increasingly problematic as data volumes grow. Databases with non-atomic values often require application-level processing, which doesn't scale well with increasing data.
Second Normal Form (2NF)
The second normal form builds on 1NF by requiring that all non-key attributes be fully functionally dependent on the primary key. This means removing partial dependencies where an attribute depends on only part of a composite key.
From a scalability perspective, 2NF helps by reducing update anomalies. When data is duplicated across rows due to partial dependencies, updates require multiple row changes, increasing the likelihood of contention and locks as concurrency increases. This becomes a significant bottleneck in high-throughput systems.
Third Normal Form (3NF)
The third normal form extends 2NF by eliminating transitive dependencies, where non-key attributes depend on other non-key attributes. This typically involves creating separate tables for sets of attributes that are functionally dependent on non-key attributes.
3NF significantly improves scalability by further reducing data redundancy. Less redundancy means smaller tables, which leads to better cache utilization, reduced I/O, and more efficient indexing. These factors become increasingly important as data volumes grow.
Boyce-Codd Normal Form (BCNF)
BCNF is a stricter version of 3NF that addresses certain anomalies not handled by 3NF. It requires that for every non-trivial functional dependency X → Y, X must be a superkey.
BCNF further improves scalability by eliminating more complex update anomalies that can lead to contention in high-concurrency environments.
Fourth Normal Form (4NF) and Fifth Normal Form (5NF)
These higher normal forms deal with multi-valued dependencies (4NF) and join dependencies (5NF). While theoretically important, many practical database designs stop at BCNF, as the additional normalization often provides diminishing returns for scalability while increasing complexity.
Normalization and Scalability Trade-offs
While normalization generally improves data integrity and reduces redundancy, it can sometimes work against scalability due to the following factors:
Join Complexity
Highly normalized databases require more joins to reconstruct complete information. As data volumes grow, these joins become increasingly expensive, especially in distributed database environments where joins across shards are particularly costly.
Query Performance
Retrieving data from multiple normalized tables often requires complex joins, which can impact query performance. This becomes more pronounced as the volume of data increases, potentially leading to scalability issues.
Write vs. Read Optimization
Normalization typically optimizes for write operations by minimizing the amount of data that needs to be updated when changes occur. However, this can come at the expense of read performance, which often requires denormalization for scalability in read-heavy workloads.
Strategic Denormalization for Scalability
In many large-scale systems, strategic denormalization is employed to improve read performance and scalability. This involves deliberately introducing some data redundancy to reduce join complexity and improve query performance. Common denormalization techniques include:
Materialized Views
Precomputing and storing the results of complex joins or aggregations can significantly improve read performance for frequently accessed data patterns.
Embedded Documents
In document databases, embedding related information within a single document (rather than normalizing across collections) can reduce the need for joins and improve read performance.
Redundant Data
Storing the same data in multiple places, particularly when it changes infrequently but is accessed often, can improve read performance at the cost of some write complexity.
Calculated Fields
Storing derived data (such as totals or counts) alongside raw data can eliminate the need for expensive calculations at query time.
Finding the Right Balance
The key to scalable database design is finding the appropriate balance between normalization and denormalization based on your specific workload characteristics:
Analyze access patterns: Understand which data is frequently read together and which data is frequently updated.
Consider read-to-write ratio: Workloads with high read-to-write ratios often benefit from more denormalization, while write-heavy workloads may benefit from more normalization.
Evaluate data volatility: Frequently changing data benefits from normalization to avoid update anomalies, while relatively static data can be denormalized with minimal overhead.
Assess join complexity: If your queries regularly join across many tables, consider strategic denormalization to reduce join complexity.
Monitor performance: Continuously evaluate the performance impact of your normalization decisions as data volumes grow.
In practice, most scalable database designs use a hybrid approach, with core transactional data maintained in a normalized form to ensure data integrity, while derived or aggregated data is denormalized to optimize read performance. This approach provides the benefits of both normalization (data integrity, reduced redundancy) and denormalization (improved read performance, reduced join complexity) where they matter most.
Remember that normalization is not an all-or-nothing proposition. Different parts of your schema may benefit from different levels of normalization based on their specific access patterns and scalability requirements. The art of scalable database design lies in making these trade-offs deliberately and strategically rather than applying normalization rules blindly.
Indexing Strategies for Scalable Database Performance
Indexing is one of the most powerful tools for improving database performance and scalability. Properly designed indexes can dramatically reduce query execution time, minimize resource consumption, and allow databases to handle larger data volumes and higher concurrency. However, indexes also come with trade-offs that must be carefully considered in a scalable database design.
Fundamentals of Database Indexing
At its core, an index is a data structure that improves the speed of data retrieval operations at the cost of additional writes and storage space. Think of it as the index at the back of a book—instead of scanning every page to find information, you can quickly locate it through the index.
B-Tree Indexes
B-Tree (Balanced Tree) indexes are the most common type of index in relational databases. They organize data in a tree structure that allows the database engine to quickly locate rows based on the indexed columns. B-Tree indexes are particularly effective for:
- Equality searches (WHERE column = value)
- Range queries (WHERE column BETWEEN value1 AND value2)
- Prefix searches (WHERE column LIKE 'prefix%')
- Sorting operations (ORDER BY indexed_column)
B-Tree indexes scale logarithmically with data size, meaning that even as your data grows into millions or billions of rows, index lookups remain relatively efficient. This logarithmic scaling property is crucial for database scalability.
Hash Indexes
Hash indexes use a hash function to map keys to index entries. They excel at equality searches but cannot support range queries or sorting operations. In certain scenarios, particularly for exact-match lookups in memory-optimized tables, hash indexes can provide better performance than B-Tree indexes.
Specialized Index Types
Modern database systems offer various specialized index types that can significantly improve scalability for specific workloads:
- Bitmap Indexes: Efficient for columns with low cardinality (few distinct values), commonly used in data warehousing.
- GiST/GIN Indexes: Support full-text search, spatial data, and other complex data types.
- Covering Indexes: Include all columns needed by a query, eliminating the need to access the table data.
- Partial Indexes: Index only a subset of rows, reducing index size and maintenance overhead.
- Functional Indexes: Index expressions or functions rather than column values directly.
Strategic Indexing for Scalability
Identify High-Impact Queries
The foundation of a good indexing strategy is understanding your application's query patterns. Focus on:
- Frequently executed queries: Queries that run often, even if they're relatively simple.
- Resource-intensive queries: Queries that consume significant CPU, memory, or I/O resources.
- Latency-sensitive queries: Queries where response time is critical to application performance.
Use query performance monitoring tools to identify these high-impact queries rather than guessing. Most database systems provide query analyzers or performance schema that can help identify slow queries and missing indexes.
Indexing for JOIN Operations
JOIN operations are often the most resource-intensive parts of queries, especially as data volumes grow. Proper indexing can dramatically improve JOIN performance:
- Index foreign keys: Always index the foreign key columns used in JOIN conditions.
- Consider composite indexes: If you frequently join on multiple columns, a composite index can be more efficient than separate single-column indexes.
- Index for both sides of the JOIN: Ensure that columns on both sides of the JOIN condition are indexed.
As your database scales, the cost of unindexed JOINs grows quadratically, making proper JOIN indexing increasingly important.
Composite Indexes and Index Order
Composite indexes (indexes on multiple columns) can significantly improve query performance, but their effectiveness depends heavily on the column order:
- Most selective columns first: Generally, place columns with higher cardinality (more unique values) first in the index.
- Match query patterns: Align index column order with how columns are used in WHERE clauses.
- Consider equality vs. range conditions: Columns used in equality conditions should typically precede those used in range conditions.
For example, if you frequently query with WHERE status = 'active' AND created_at > '2023-01-01'
, a composite index on (status, created_at) would be more efficient than one on (created_at, status).
Index Maintenance and Overhead
While indexes improve read performance, they come with maintenance costs that can impact write performance and overall scalability:
- Write overhead: Every INSERT, UPDATE, or DELETE operation must also update all affected indexes.
- Storage overhead: Indexes consume additional storage space, which can be substantial for large tables.
- Fragmentation: Over time, indexes can become fragmented, reducing their efficiency.
To mitigate these issues:
- Avoid over-indexing: Each additional index increases write overhead. Focus on high-impact queries.
- Monitor index usage: Regularly review index usage statistics and remove unused indexes.
- Schedule index maintenance: Implement regular index rebuilding or reorganization to combat fragmentation.
- Consider fill factor: Setting an appropriate fill factor can reduce the frequency of page splits and associated fragmentation.
Indexing for Sorting and Grouping
Operations like ORDER BY and GROUP BY can be resource-intensive, especially with large result sets:
- Index for sort order: If you frequently sort on specific columns, index those columns in the required order.
- Include aggregation columns: For GROUP BY operations, include both the grouping columns and frequently aggregated columns in indexes.
- Consider covering indexes: Include all columns referenced in the query to avoid table lookups.
Indexing in Distributed Databases
In sharded or distributed database environments, indexing strategies become even more critical:
- Local vs. global indexes: Understand the difference between indexes that exist on each shard (local) versus those that span all shards (global).
- Shard key selection: Choose shard keys that align with your indexing strategy to minimize cross-shard operations.
- Replicated indexes: Consider replicating certain indexes across all nodes to improve read performance.
Advanced Indexing Techniques for Extreme Scalability
Partial Indexes
For very large tables, consider partial indexes that only index a subset of rows:
CREATE INDEX idx_recent_orders ON orders (order_date, customer_id)
WHERE status = 'active' AND order_date > '2023-01-01';
This approach can dramatically reduce index size and maintenance overhead while still accelerating queries on the most relevant data.
Covering Indexes
Covering indexes include all columns needed by a query, eliminating the need to access the table data:
CREATE INDEX idx_orders_covering ON orders (order_date, customer_id, status, total_amount);
For frequently executed queries that access a small subset of columns, covering indexes can provide substantial performance improvements, especially as data volumes grow.
Index-Only Scans
Design your indexes to enable index-only scans for your most critical queries. An index-only scan occurs when all the data needed to satisfy a query can be found in the index itself, without accessing the table data. This dramatically reduces I/O and improves query performance.
Functional Indexes
For queries that filter or sort on expressions rather than direct column values, functional indexes can provide significant performance benefits:
CREATE INDEX idx_lower_email ON users (LOWER(email));
This allows efficient case-insensitive searches without requiring function calls on each row during query execution.
Monitoring and Evolving Your Indexing Strategy
As your application and data grow, your indexing strategy must evolve:
- Regular performance reviews: Periodically review query performance and index usage statistics.
- Workload-based tuning: Adjust your indexing strategy based on changing query patterns and data growth.
- A/B testing: Test new indexes in staging environments before deploying to production.
- Automated index suggestions: Many database systems and third-party tools can suggest missing indexes based on query patterns.
Remember that indexing is not a one-time task but an ongoing process of refinement. What works well for a database with millions of rows might be insufficient when it grows to billions.
Balancing Reads and Writes
The ultimate goal of a scalable indexing strategy is to find the right balance between read and write performance based on your specific workload:
- Read-heavy workloads: More aggressive indexing is generally beneficial, even at the cost of some write performance.
- Write-heavy workloads: Be more selective with indexes, focusing only on the most critical queries.
- Mixed workloads: Consider time-based partitioning strategies that allow different indexing approaches for recent (write-heavy) versus historical (read-heavy) data.
By thoughtfully designing your indexing strategy with scalability in mind, you can ensure that your database continues to perform well even as data volumes and query complexity increase. Remember that the best indexing strategy is one that evolves with your application's needs and growth trajectory.
Partitioning and Sharding Techniques for Database Scalability
As databases grow beyond certain thresholds, even the most optimized schema and indexing strategies may not be sufficient to maintain acceptable performance. This is where partitioning and sharding come into play—techniques that divide large tables and databases into smaller, more manageable pieces. These approaches are fundamental to building truly scalable database systems that can handle massive data volumes and high transaction rates.
Table Partitioning
Table partitioning involves dividing a single logical table into multiple physical storage units according to defined rules. The database engine handles this division transparently, so applications can generally interact with a partitioned table as if it were a single entity.
Horizontal Partitioning (Row-Based)
Horizontal partitioning divides a table by rows, with each partition containing a subset of the rows based on a partition key. This is the most common form of partitioning and provides several benefits for scalability:
- Improved query performance: Queries that filter on the partition key can skip irrelevant partitions, a technique known as partition pruning.
- Efficient maintenance: Operations like index rebuilds or statistics updates can be performed on individual partitions rather than the entire table.
- Parallel operations: Queries spanning multiple partitions can be executed in parallel, improving performance.
- Tiered storage: Different partitions can be stored on different types of storage (e.g., recent data on fast SSD, historical data on slower, cheaper storage).
Common horizontal partitioning strategies include:
Range Partitioning
Rows are distributed based on a range of values in the partition key, such as date ranges:
CREATE TABLE orders (
order_id INT,
customer_id INT,
order_date DATE,
total_amount DECIMAL(10,2),
-- other columns
) PARTITION BY RANGE (order_date) (
PARTITION p_2023_q1 VALUES LESS THAN ('2023-04-01'),
PARTITION p_2023_q2 VALUES LESS THAN ('2023-07-01'),
PARTITION p_2023_q3 VALUES LESS THAN ('2023-10-01'),
PARTITION p_2023_q4 VALUES LESS THAN ('2024-01-01'),
PARTITION p_future VALUES LESS THAN (MAXVALUE)
);
Range partitioning is particularly effective for time-series data and historical reporting, as it allows efficient pruning of date-based queries and simplified archiving of old data.
List Partitioning
Rows are distributed based on discrete values in the partition key:
CREATE TABLE customers (
customer_id INT,
name VARCHAR(100),
country_code CHAR(2),
-- other columns
) PARTITION BY LIST (country_code) (
PARTITION p_americas VALUES IN ('US', 'CA', 'MX', 'BR'),
PARTITION p_europe VALUES IN ('UK', 'FR', 'DE', 'IT', 'ES'),
PARTITION p_asia VALUES IN ('CN', 'JP', 'IN', 'SG'),
PARTITION p_other VALUES IN (DEFAULT)
);
List partitioning works well when data naturally falls into discrete categories and queries frequently filter on those categories.
Hash Partitioning
Rows are distributed evenly across partitions using a hash function on the partition key:
CREATE TABLE user_activities (
activity_id INT,
user_id INT,
activity_type VARCHAR(50),
activity_date TIMESTAMP,
-- other columns
) PARTITION BY HASH (user_id) PARTITIONS 16;
Hash partitioning provides even data distribution, which is beneficial for workloads where queries are distributed across all values of the partition key rather than focusing on specific ranges or lists.
Vertical Partitioning (Column-Based)
Vertical partitioning divides a table by columns rather than rows. This approach is less commonly supported as a native feature in traditional relational databases but can be implemented manually by splitting a logical entity across multiple related tables:
-- Original wide table
CREATE TABLE products (
product_id INT PRIMARY KEY,
name VARCHAR(100),
description TEXT,
price DECIMAL(10,2),
inventory_count INT,
specifications TEXT,
image_data BLOB
);
-- Vertically partitioned into multiple tables
CREATE TABLE product_core (
product_id INT PRIMARY KEY,
name VARCHAR(100),
price DECIMAL(10,2),
inventory_count INT
);
CREATE TABLE product_details (
product_id INT PRIMARY KEY,
description TEXT,
specifications TEXT,
FOREIGN KEY (product_id) REFERENCES product_core(product_id)
);
CREATE TABLE product_images (
product_id INT PRIMARY KEY,
image_data BLOB,
FOREIGN KEY (product_id) REFERENCES product_core(product_id)
);
Vertical partitioning is particularly useful for:
- Tables with very wide rows: Splitting rarely accessed columns into separate tables can improve I/O efficiency for common queries.
- Mixed workload optimization: Frequently accessed columns can be kept together in a highly optimized table, while rarely accessed columns can be stored separately.
- Regulatory compliance: Sensitive data can be isolated in separate tables with different security controls.
Composite Partitioning
Many database systems support composite partitioning, which combines multiple partitioning strategies:
CREATE TABLE sales (
sale_id INT,
store_id INT,
sale_date DATE,
amount DECIMAL(10,2),
-- other columns
) PARTITION BY RANGE (sale_date)
SUBPARTITION BY LIST (store_id) (
PARTITION p_2023_q1 VALUES LESS THAN ('2023-04-01') (
SUBPARTITION p_2023_q1_east VALUES IN (1, 2, 3, 4),
SUBPARTITION p_2023_q1_west VALUES IN (5, 6, 7, 8)
),
PARTITION p_2023_q2 VALUES LESS THAN ('2023-07-01') (
SUBPARTITION p_2023_q2_east VALUES IN (1, 2, 3, 4),
SUBPARTITION p_2023_q2_west VALUES IN (5, 6, 7, 8)
)
-- additional partitions
);
Composite partitioning provides more granular control over data distribution and can be particularly effective for very large tables with complex query patterns.
Database Sharding
While partitioning divides tables within a single database instance, sharding distributes data across multiple database instances or servers. Sharding is a fundamental technique for horizontal scaling, allowing databases to scale beyond the capacity of a single machine.
Sharding Strategies
Key-Based Sharding
Data is distributed across shards based on a value in each record (the shard key):
-
Range-based sharding: Similar to range partitioning, but across separate database instances.
- Example: Customer IDs 1-1,000,000 on Shard 1, 1,000,001-2,000,000 on Shard 2, etc.
- Pros: Simple to implement, efficient range queries within a shard.
- Cons: Potential for uneven distribution, "hot spots" if certain ranges are accessed more frequently.
-
Hash-based sharding: A hash function determines which shard holds each record.
- Example: hash(customer_id) % num_shards determines the shard for each customer.
- Pros: Even distribution of data, minimizes hot spots.
- Cons: Range queries become inefficient as they must query all shards.
-
Directory-based sharding: A lookup service maps keys to shards.
- Example: A separate service maintains mappings of customer_id ranges to specific shards.
- Pros: Flexible, allows for resharding without changing application logic.
- Cons: Additional complexity, lookup service becomes a potential bottleneck.
Entity-Based Sharding
Different entities (tables) are placed on different shards based on their relationships and access patterns:
-
Functional sharding: Dividing by functional area (e.g., user data on one shard, product data on another).
- Pros: Isolates workloads, allows for specialized optimization of each shard.
- Cons: Complicates queries that span functional areas.
-
Tenant-based sharding: In multi-tenant applications, each tenant's data is placed on a specific shard.
- Pros: Natural isolation, tenant-specific scaling, simplified tenant onboarding/offboarding.
- Cons: Potential for uneven distribution if tenants vary significantly in size.
Sharding Considerations for Schema Design
Effective sharding requires careful schema design considerations:
Shard Key Selection
The shard key determines how data is distributed across shards and has profound implications for performance and scalability:
- High cardinality: The shard key should have enough unique values to allow even distribution.
- Low frequency of updates: Changing a shard key value typically requires moving data between shards, which is expensive.
- Query patterns: Ideally, most queries should be satisfiable within a single shard by including the shard key in the query.
Common shard key choices include:
- Customer or tenant ID: In multi-tenant systems or applications where data is naturally customer-centric.
- Geographic location: For applications with strong geographic locality in access patterns.
- Time-based identifiers: For time-series data where recent data is accessed most frequently.
Cross-Shard Operations
Queries that span multiple shards (cross-shard queries) are typically more expensive and complex:
- Minimize cross-shard joins: Design your schema to keep related data on the same shard whenever possible.
- Denormalize across shards: Strategic denormalization can reduce the need for cross-shard operations.
- Global tables: Consider replicating small, relatively static lookup tables across all shards.
- Aggregation services: For operations that must span all shards, consider implementing application-level aggregation services.
Schema Consistency Across Shards
Maintaining consistent schemas across all shards is essential for operational simplicity:
- Automated schema changes: Implement tools to apply schema changes consistently across all shards.
- Version control: Track schema versions for each shard to ensure consistency.
- Canary deployments: Test schema changes on a subset of shards before full deployment.
Partitioning and Sharding in Different Database Systems
Different database systems implement partitioning and sharding in various ways:
Relational Databases
- PostgreSQL: Supports declarative range, list, and hash partitioning with inheritance-based implementation.
- MySQL: Offers range, list, hash, and key partitioning with up to 8,192 partitions per table.
- Oracle: Provides comprehensive partitioning options including interval, reference, and virtual column partitioning.
- SQL Server: Supports range, list, round-robin, and hash partitioning through partition functions and schemes.
NoSQL Databases
- MongoDB: Built with native sharding capabilities using range, hash, or tag-aware sharding.
- Cassandra: Distributes data across nodes using consistent hashing on the partition key.
- DynamoDB: Automatically manages partitioning based on the partition key, with optional sort keys.
- Couchbase: Uses a distributed hash table approach with vBuckets for data distribution.
NewSQL and Distributed SQL
- Google Spanner: Combines sharding with synchronous replication and TrueTime for global consistency.
- CockroachDB: Automatically shards data into ranges distributed across nodes using the Raft consensus algorithm.
- Vitess: Provides MySQL sharding through a proxy layer that manages shard routing and schema changes.
Implementing Scalable Partitioning and Sharding
Phased Approach to Sharding
For most applications, a phased approach to implementing sharding is recommended:
- Start with vertical scaling: Maximize the capacity of a single database instance before introducing sharding complexity.
- Implement read replicas: Add read replicas to offload read queries before sharding.
- Introduce functional sharding: Separate different functional areas into different database instances.
- Implement horizontal sharding: Only after exhausting simpler options, implement full horizontal sharding.
Application-Level Sharding vs. Database-Level Sharding
Sharding can be implemented at different levels:
-
Application-level sharding: The application contains the logic to route queries to the appropriate shard.
- Pros: More control, can work with databases that don't natively support sharding.
- Cons: Increases application complexity, potential for logic inconsistencies.
-
Middleware sharding: A separate middleware layer handles shard routing.
- Pros: Separates sharding logic from application code, consistent implementation.
- Cons: Additional infrastructure component, potential performance overhead.
-
Database-level sharding: The database system itself handles sharding.
- Pros: Simplifies application development, leverages database-specific optimizations.
- Cons: Limited to databases with native sharding support, less flexibility.
Resharding Considerations
As data grows, the initial sharding scheme may become suboptimal, necessitating resharding:
- Design for resharding from the start: Assume you'll need to reshard eventually and design accordingly.
- Implement shard splitting: The ability to split a shard into multiple shards as it grows.
- Consider shard rebalancing: Mechanisms to move data between shards to maintain even distribution.
- Zero-downtime resharding: Techniques like double-writing and background data migration to avoid service disruption.
Partitioning and sharding are powerful techniques for scaling databases beyond the capacity of a single server. However, they introduce complexity and require careful planning. By understanding the trade-offs and designing your schema with partitioning and sharding in mind from the beginning, you can create database systems that scale gracefully as your data and user base grow.
Remember that the best partitioning and sharding strategy depends on your specific workload characteristics, query patterns, and scaling requirements. Start with the simplest approach that meets your needs, and evolve your strategy as your application grows and your understanding of its data access patterns matures.
Data Types and Constraints Selection for Scalable Database Schemas
The selection of appropriate data types and constraints is a fundamental aspect of database schema design that directly impacts scalability, performance, and storage efficiency. While often overlooked in favor of more advanced scaling techniques, poor data type choices can undermine even the most sophisticated partitioning or indexing strategies. This section explores how to select data types and constraints with scalability in mind.
Principles of Data Type Selection for Scalability
Storage Efficiency
Every byte matters when designing for scale. Efficient storage not only reduces costs but also improves performance by:
- Reducing I/O operations: Smaller rows mean more rows per page, reducing the number of disk reads required.
- Improving cache utilization: More efficient data representation allows more data to fit in memory caches.
- Reducing network traffic: For distributed databases, smaller data types reduce the amount of data transferred between nodes.
Processing Efficiency
Different data types have different computational characteristics:
- Integer operations are typically faster than floating-point operations.
- Fixed-length data types often outperform variable-length types for comparison operations.
- Native data types (those directly supported by the CPU) generally outperform complex types that require additional processing.
Scalability Impact of Data Types
As your database grows, the impact of data type choices becomes increasingly significant:
- Linear impact on storage: Every byte saved per row multiplies by the number of rows.
- Compound impact on indexes: Inefficient data types in indexed columns affect both table and index storage.
- Exponential impact on joins: Inefficient data types in join columns can dramatically increase the cost of join operations as data volumes grow.
Optimal Data Type Selection by Category
Numeric Data Types
Integers
Choose the smallest integer type that can accommodate your data range:
Type | Storage | Range | Use Case |
---|---|---|---|
TINYINT | 1 byte | -128 to 127 (signed) | Status codes, small counters |
SMALLINT | 2 bytes | -32,768 to 32,767 | Medium-sized counts |
INT | 4 bytes | -2.1B to 2.1B | General purpose |
BIGINT | 8 bytes | -9.2E+18 to 9.2E+18 | Large IDs, timestamps |
For unsigned values (when supported), specify UNSIGNED to effectively double the positive range.
Scalability Tip: For primary keys and foreign keys that will be heavily indexed and joined, INT or BIGINT is typically the best choice despite the larger size, as they align with CPU register sizes for optimal processing.
Decimal and Floating Point
For financial calculations and other scenarios requiring exact decimal representation:
DECIMAL(precision, scale)
Where precision is the total number of digits, and scale is the number of digits after the decimal point.
For scientific or approximate calculations where exact decimal representation isn't required:
FLOAT(precision) -- Single precision (4 bytes)
DOUBLE(precision) -- Double precision (8 bytes)
Scalability Tip: DECIMAL types with large precision can consume significant storage and processing resources. For high-volume tables, consider if a smaller precision is acceptable or if an integer representation (e.g., storing cents instead of dollars and cents) would be more efficient.
String Data Types
Fixed-Length Strings
For data with a consistent length:
CHAR(length) -- Fixed-length, padded with spaces
Scalability Tip: CHAR is more efficient for very short strings (typically under 10 characters) that are always or nearly always the same length, such as country codes or postal codes.
Variable-Length Strings
For most text data:
VARCHAR(max_length) -- Variable-length with length prefix
Scalability Tip: Always set a reasonable maximum length rather than defaulting to the maximum allowed. This improves both storage efficiency and query planning.
Text/BLOB Types
For large text or binary objects:
TEXT, MEDIUMTEXT, LONGTEXT -- For text data
BLOB, MEDIUMBLOB, LONGBLOB -- For binary data
Scalability Tip: Consider storing very large text or binary objects outside the database with only a reference stored in the database, especially if they are infrequently accessed or updated independently from other row data.
Temporal Data Types
Date and Time
Choose the appropriate temporal type based on your precision requirements:
DATE -- Date only (3 bytes)
TIME -- Time only (3-5 bytes)
DATETIME or TIMESTAMP -- Date and time (7-8 bytes)
Scalability Tip: TIMESTAMP often uses less storage than DATETIME and automatically converts to the current time zone, but has a more limited range (typically 1970-2038). For data outside this range, DATETIME is necessary despite the larger storage footprint.
Boolean Data
For true/false values:
BOOLEAN or TINYINT(1)
Scalability Tip: Many databases implement BOOLEAN as TINYINT(1) internally. For very large tables with many boolean flags, consider using bit fields or bit manipulation to pack multiple boolean values into a single byte.
Specialized Data Types
Modern databases offer specialized data types for specific data categories:
JSON -- For semi-structured data
GEOMETRY, GEOGRAPHY -- For spatial data
ARRAY, HSTORE -- For collections (in supported databases)
UUID -- For universally unique identifiers
INET -- For IP addresses
Scalability Tip: While convenient, specialized types often have performance implications. For example, JSON types typically can't be indexed directly (though JSON paths can be). Evaluate whether a decomposed relational representation might be more efficient for high-volume access patterns.
Constraints and Their Impact on Scalability
Constraints ensure data integrity but can impact performance and scalability. Understanding this trade-off is essential for scalable schema design.
Primary Key Constraints
Primary keys enforce uniqueness and provide a default clustering order for the table:
CREATE TABLE users (
user_id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
-- other columns
);
Scalability Considerations:
- Key size: Smaller primary keys (4-8 bytes) are generally more efficient than larger composite keys.
- Monotonically increasing vs. random: Sequential primary keys (like auto-increment IDs) typically result in better insert performance and less index fragmentation than random values (like UUIDs).
- Surrogate vs. natural keys: Surrogate keys (artificial identifiers) are generally more stable and efficient than natural keys (business attributes) for large-scale systems.
Foreign Key Constraints
Foreign keys enforce referential integrity between tables:
CREATE TABLE orders (
order_id INT PRIMARY KEY,
user_id INT NOT NULL,
-- other columns
FOREIGN KEY (user_id) REFERENCES users(user_id)
);
Scalability Considerations:
- Enforcement overhead: Foreign key constraints add overhead to INSERT, UPDATE, and DELETE operations.
- Cascading actions: Cascading updates or deletes can lead to performance issues with large datasets.
- Distributed databases: Foreign key constraints are often difficult or impossible to enforce across shards in distributed databases.
Scalability Tip: For extremely high-volume write workloads or sharded databases, consider enforcing referential integrity at the application level rather than using database constraints.
Unique Constraints
Unique constraints ensure that values in specified columns are unique across the table:
CREATE TABLE users (
user_id INT PRIMARY KEY,
email VARCHAR(100) UNIQUE,
-- other columns
);
Scalability Considerations:
- Index overhead: Each unique constraint creates an additional index, increasing storage and write overhead.
- Distributed uniqueness: Enforcing uniqueness across shards requires special consideration.
Check Constraints
Check constraints enforce domain integrity by limiting the values that can be placed in a column:
CREATE TABLE products (
product_id INT PRIMARY KEY,
price DECIMAL(10,2) CHECK (price > 0),
-- other columns
);
Scalability Considerations:
- Validation overhead: Complex check constraints can add processing overhead to data modifications.
- Maintenance complexity: Business rules encoded in check constraints may require schema changes as rules evolve.
Not Null Constraints
Not Null constraints ensure that a column cannot contain NULL values:
CREATE TABLE users (
user_id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
-- other columns
);
Scalability Considerations:
- Storage efficiency: Some database systems store NULL values more efficiently than empty values, but this varies by system.
- Index efficiency: Columns with many NULL values may benefit from partial indexes that exclude NULL values.
Advanced Data Type Strategies for Scalability
Normalization vs. Denormalization of Data Types
In some cases, decomposing complex data types into normalized structures can improve scalability:
-- Instead of:
CREATE TABLE users (
user_id INT PRIMARY KEY,
address VARCHAR(500)
);
-- Consider:
CREATE TABLE users (
user_id INT PRIMARY KEY,
-- other columns
);
CREATE TABLE addresses (
address_id INT PRIMARY KEY,
user_id INT,
street VARCHAR(100),
city VARCHAR(50),
state CHAR(2),
postal_code VARCHAR(20),
country CHAR(2),
FOREIGN KEY (user_id) REFERENCES users(user_id)
);
This approach allows for more efficient indexing, querying, and storage of address components, especially if queries frequently filter on specific address parts.
Enumerated Types vs. Lookup Tables
For columns with a fixed set of possible values, you can use either enumerated types or lookup tables:
-- Enumerated type approach:
CREATE TABLE tickets (
ticket_id INT PRIMARY KEY,
status ENUM('open', 'in_progress', 'closed', 'cancelled')
);
-- Lookup table approach:
CREATE TABLE ticket_statuses (
status_id TINYINT PRIMARY KEY,
status_name VARCHAR(20) NOT NULL
);
CREATE TABLE tickets (
ticket_id INT PRIMARY KEY,
status_id TINYINT,
FOREIGN KEY (status_id) REFERENCES ticket_statuses(status_id)
);
Scalability Considerations:
- Storage efficiency: Enumerated types are typically more storage-efficient.
- Query performance: Lookup tables may require joins but provide more flexibility.
- Schema evolution: Adding values to an enumerated type often requires an ALTER TABLE operation, while lookup tables can be updated with simple INSERT statements.
Computed Columns and Generated Values
Modern databases support computed or generated columns that derive their values from other columns:
CREATE TABLE products (
product_id INT PRIMARY KEY,
price DECIMAL(10,2) NOT NULL,
tax_rate DECIMAL(5,2) NOT NULL,
total_price DECIMAL(10,2) GENERATED ALWAYS AS (price * (1 + tax_rate)) STORED
);
Scalability Considerations:
- Storage vs. computation: STORED generated columns consume storage but avoid computation during queries, while VIRTUAL generated columns (when supported) save storage but require computation.
- Indexing: STORED generated columns can be indexed, potentially improving query performance for frequently used calculations.
JSON and Semi-Structured Data
For flexible schema requirements, JSON data types offer a compromise between rigid relational structures and completely schemaless designs:
CREATE TABLE user_preferences (
user_id INT PRIMARY KEY,
preferences JSON,
FOREIGN KEY (user_id) REFERENCES users(user_id)
);
Scalability Considerations:
- Indexing limitations: While many databases now support indexing JSON paths, these indexes are typically less efficient than indexes on regular columns.
- Query optimization: Query optimizers may have limited ability to optimize queries against JSON data.
- Storage overhead: JSON storage typically includes field names with each record, increasing storage requirements compared to columnar storage.
Scalability Tip: Consider a hybrid approach where frequently accessed or queried attributes are stored in regular columns, while more dynamic or rarely queried attributes are stored in JSON.
Data Type Compression and Encoding
Many database systems offer compression features that can significantly improve storage efficiency and, consequently, I/O performance:
Row Compression
Row compression reduces storage by using variable-length encoding for fixed-length types and other techniques:
CREATE TABLE sales (
-- columns
) WITH (DATA_COMPRESSION = ROW); -- SQL Server syntax
Page Compression
Page compression adds additional compression at the page level, identifying and eliminating repeated values:
CREATE TABLE sales (
-- columns
) WITH (DATA_COMPRESSION = PAGE); -- SQL Server syntax
Column Compression
Column-oriented databases or tables can apply type-specific compression algorithms to each column:
CREATE TABLE sales (
-- columns
) USING COLUMNSTORE; -- Example syntax
Scalability Tip: Compression typically trades CPU cycles for reduced I/O. For I/O-bound workloads, this is usually a beneficial trade-off, but for CPU-bound workloads, excessive compression might reduce overall performance.
Database-Specific Considerations
Different database systems have unique characteristics that affect data type selection:
PostgreSQL
- Offers rich set of data types including arrays, JSON, HSTORE, and custom types
- JSONB type provides binary storage and indexing for JSON data
- Efficient handling of text data with TOAST (The Oversized-Attribute Storage Technique)
MySQL/MariaDB
- Different storage engines have different data type efficiencies
- InnoDB has specific optimizations for primary keys
- Careful consideration needed for character sets and collations
SQL Server
- Sparse columns for tables with many NULL values
- Efficient handling of variable-length data with row overflow
- Specific optimizations for clustered columnstore indexes
Oracle
- Efficient handling of large objects with SecureFiles
- Advanced partitioning based on data type characteristics
- Automatic data optimization for temperature-based storage tiering
Practical Guidelines for Scalable Data Type Selection
Start with the smallest sufficient data type: Always choose the smallest data type that can accommodate your data, including future growth.
Consider the entire lifecycle: Data that starts small may grow over time. For example, user IDs might start as INT but eventually require BIGINT.
Balance normalization with query patterns: Normalize data types when components are frequently queried independently; denormalize when they're typically accessed together.
Benchmark critical paths: For high-volume tables, benchmark different data type strategies to find the optimal balance between storage efficiency and query performance.
Document your decisions: Explicitly document why specific data types were chosen, especially when the choice isn't obvious, to prevent future changes that might undermine scalability.
Review periodically: As your application evolves, periodically review data type choices against actual usage patterns and growth rates.
By carefully selecting appropriate data types and constraints with scalability in mind, you can create database schemas that not only ensure data integrity but also perform well and scale efficiently as your data grows. Remember that these foundational choices have compounding effects throughout your database's lifecycle and are often much harder to change later than other aspects of your schema design.
Schema Versioning and Migration Strategies for Scalable Databases
Database schema evolution is inevitable in any growing application. As business requirements change, new features are added, and performance optimizations become necessary, your database schema must adapt accordingly. However, in large-scale systems, schema changes can be risky, disruptive, and potentially catastrophic if not managed properly. This section explores strategies for versioning and migrating database schemas in a way that maintains scalability and minimizes disruption.
The Challenges of Schema Evolution at Scale
Schema changes in large-scale database systems face several unique challenges:
Performance Impact
Schema modifications can lock tables, block writes, or consume significant system resources:
- Table locks: Operations like adding columns or constraints may lock tables, preventing access during the operation.
- Resource consumption: Rebuilding indexes or restructuring data can consume substantial CPU, memory, and I/O resources.
- Replication lag: In replicated environments, schema changes must propagate to all replicas, potentially causing temporary inconsistencies.
Coordination Complexity
In distributed systems, coordinating schema changes across multiple nodes adds complexity:
- Version inconsistency: Different nodes may temporarily operate with different schema versions.
- Distributed transactions: Some schema changes require distributed transactions, which are difficult to implement reliably.
- Rolling deployments: Application and schema changes must be coordinated to maintain compatibility during transitions.
Risk Exposure
The impact of schema change failures increases with scale:
- Rollback difficulty: Some schema changes cannot be easily rolled back once started.
- Data integrity risks: Failed migrations can leave data in an inconsistent state.
- Downtime implications: In large systems, even brief downtime can have significant business impact.
Schema Versioning Fundamentals
A robust schema versioning system is the foundation for successful schema evolution:
Version Tracking
Every schema change should be explicitly versioned:
-- Example of a schema version tracking table
CREATE TABLE schema_versions (
version_id INT PRIMARY KEY,
applied_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP,
description VARCHAR(200) NOT NULL,
script_name VARCHAR(100) NOT NULL,
applied_by VARCHAR(50) NOT NULL,
success BOOLEAN NOT NULL
);
This table serves as an audit trail of all schema changes and helps ensure that migrations are applied exactly once in the correct order.
Migration Scripts
Each schema change should be encapsulated in a migration script:
-- V1.0.1__Create_users_table.sql
CREATE TABLE users (
user_id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL UNIQUE,
email VARCHAR(100) NOT NULL UNIQUE,
created_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP
);
-- V1.0.2__Add_user_status.sql
ALTER TABLE users ADD COLUMN status VARCHAR(20) NOT NULL DEFAULT 'active';
Migration scripts should:
- Be immutable: Once a migration has been applied to any environment, it should never be modified.
- Be idempotent: Scripts should be safe to run multiple times without causing errors or duplicate changes.
- Include both up and down migrations: When possible, provide scripts to both apply and revert changes.
Version Control Integration
Schema version control should be integrated with your application code version control:
- Repository structure: Store migration scripts in a dedicated directory within your application repository.
- Branch management: Coordinate schema changes with application changes in feature branches.
- Review process: Include database experts in code reviews for schema changes.
Schema Migration Strategies for Scalability
Different types of schema changes require different approaches to maintain scalability:
Additive Changes
Additive changes (adding tables, columns, or indexes) are generally the safest:
-- Adding a new column (safe)
ALTER TABLE users ADD COLUMN last_login_at TIMESTAMP NULL;
-- Adding a new table (safe)
CREATE TABLE user_preferences (
user_id INT PRIMARY KEY,
theme VARCHAR(20) NOT NULL DEFAULT 'default',
notifications_enabled BOOLEAN NOT NULL DEFAULT TRUE,
FOREIGN KEY (user_id) REFERENCES users(user_id)
);
Scalability Tip: When adding columns to very large tables, consider adding them with NULL or DEFAULT constraints to avoid table rewrites. In some databases, adding a NOT NULL column without a DEFAULT value requires rewriting the entire table, which can be extremely expensive for large tables.
Expansive Changes
Expansive changes modify existing structures in a backward-compatible way:
-- Expanding a column's size (generally safe)
ALTER TABLE products ALTER COLUMN description TYPE VARCHAR(500);
-- Relaxing constraints (generally safe)
ALTER TABLE orders ALTER COLUMN notes DROP NOT NULL;
Scalability Tip: Even seemingly safe expansive changes can cause table rewrites in some database systems. Test these operations on a representative dataset before applying them to production.
Destructive Changes
Destructive changes modify or remove existing structures and are the most challenging to implement safely:
-- Renaming a column (potentially disruptive)
ALTER TABLE users RENAME COLUMN username TO user_name;
-- Dropping a column (potentially disruptive)
ALTER TABLE products DROP COLUMN old_price;
-- Changing column type (potentially disruptive)
ALTER TABLE orders ALTER COLUMN status TYPE VARCHAR(30);
For destructive changes in large-scale systems, a multi-phase approach is typically required.
Multi-Phase Migration Patterns
For complex or potentially disruptive schema changes, multi-phase migration patterns help maintain system availability and data integrity:
Expand and Contract Pattern
Also known as the "parallel change" or "grow and shrink" pattern:
- Expand phase: Add new structures without removing old ones.
- Transition phase: Update application code to write to both old and new structures, but read from new structures.
- Contract phase: Once all systems are using the new structures, remove the old ones.
Example: Renaming a column in a large table
-- Phase 1: Add new column
ALTER TABLE users ADD COLUMN user_name VARCHAR(50);
-- Phase 2: Copy data (may need to be done in batches for very large tables)
UPDATE users SET user_name = username;
-- Phase 3: Application changes
-- Update application to write to both username and user_name
-- Update application to read from user_name
-- Phase 4: Verify and remove old column (after sufficient time)
ALTER TABLE users DROP COLUMN username;
This pattern minimizes risk by ensuring that the system remains functional throughout the migration process.
Feature Flags for Schema Changes
Combine schema migrations with application feature flags:
- Deploy schema changes: Apply backward-compatible schema changes.
- Deploy application with feature flags: Release new application version with feature flags disabled.
- Gradual rollout: Enable feature flags for a small percentage of users or traffic.
- Monitor and expand: Gradually increase the percentage as confidence grows.
This approach allows you to quickly disable features if schema changes cause unexpected issues.
Blue-Green Database Deployments
For major schema overhauls, consider a blue-green deployment approach:
- Set up parallel database: Create a new database instance with the updated schema.
- Synchronize data: Use replication or batch processes to keep the new database in sync.
- Test thoroughly: Validate the new database with realistic workloads.
- Switch traffic: Redirect application traffic from the old database to the new one.
- Maintain fallback: Keep the old database available for quick rollback if needed.
While resource-intensive, this approach minimizes risk for major schema changes.
Schema Migration Tools and Frameworks
Several tools can help manage schema migrations in scalable systems:
Database-Agnostic Migration Tools
- Flyway: Version-based migration tool with support for SQL and Java-based migrations.
- Liquibase: XML, YAML, JSON, or SQL-based migration tool with rollback support.
- Alembic: Python-based migration tool integrated with SQLAlchemy.
Database-Specific Tools
- PostgreSQL: pg_migrate, sqitch
- MySQL: pt-online-schema-change, gh-ost
- MongoDB: mongomirror, mongodb-schema-simulator
Custom Migration Frameworks
For very large-scale systems, custom migration frameworks may be necessary:
- Throttled migrations: Control the pace of migrations to limit performance impact.
- Scheduled windows: Perform high-impact migrations during low-traffic periods.
- Automated verification: Automatically verify data integrity after migrations.
- Canary testing: Test migrations on a subset of data before full deployment.
Online Schema Change Techniques
For high-availability systems, online schema change techniques minimize disruption:
Shadow Tables
The shadow table approach creates a new table with the desired structure, synchronizes data, and then swaps tables:
- Create shadow table: Create a new table with the desired schema.
- Create triggers: Set up triggers to propagate changes from the original table to the shadow table.
- Backfill data: Copy existing data from the original table to the shadow table.
- Verify consistency: Ensure that both tables contain identical data.
- Atomic rename: Rename tables to swap the shadow table into place.
This technique is implemented by tools like pt-online-schema-change and gh-ost.
In-Place Rebuilds with Concurrent Access
Some databases support in-place table rebuilds while maintaining concurrent access:
-- PostgreSQL example
ALTER TABLE large_table ADD COLUMN new_column INT;
In PostgreSQL, this operation creates a new version of the table in the background while allowing continued access to the original table. Once the new version is ready, a brief lock is acquired to swap the tables.
Temporary Column Approach
For column modifications, a temporary column can be used:
- Add temporary column: Add a new column with the desired structure.
- Migrate data: Copy and transform data from the old column to the new one.
- Update application: Modify the application to use the new column.
- Swap columns: Rename columns to put the new column in place of the old one.
This approach works well for column type changes or other modifications that can't be done in-place.
Schema Versioning in Microservices and Distributed Systems
Distributed systems introduce additional complexity for schema versioning:
Database-Per-Service Pattern
In microservice architectures, each service often owns its database:
- Encapsulated schemas: Each service independently manages its schema.
- API contracts: Services interact through well-defined APIs rather than shared databases.
- Versioned APIs: API versioning provides backward compatibility during schema evolution.
This pattern isolates schema changes to individual services, reducing the scope and risk of each change.
Event Sourcing and Schema Evolution
Event sourcing systems store sequences of events rather than current state:
- Immutable events: Once stored, events are never modified.
- Schema versioning: Event schemas evolve over time with explicit versioning.
- Upcasting: Older event formats are transformed to newer formats when read.
This approach provides a natural audit trail and simplifies certain types of schema evolution.
Polyglot Persistence
Different services may use different database types based on their specific requirements:
- Specialized databases: Choose database types that best match each service's data model and access patterns.
- Independent evolution: Each database evolves according to its own capabilities and constraints.
- Integration through APIs: Services integrate through APIs rather than shared data structures.
This approach allows each service to optimize its data storage independently.
Schema Versioning Best Practices for Scalability
Planning and Communication
- Impact assessment: Before any schema change, assess its impact on performance, availability, and dependent systems.
- Communication plan: Inform all stakeholders about upcoming schema changes, especially those with potential user impact.
- Maintenance windows: Schedule high-impact changes during designated maintenance windows when possible.
Testing and Validation
- Representative test environments: Test migrations on datasets that represent production in size and characteristics.
- Performance testing: Measure the performance impact of schema changes before applying them to production.
- Rollback testing: Verify that rollback procedures work correctly for each migration.
Execution and Monitoring
- Batching: Break large migrations into smaller batches to limit resource consumption and lock duration.
- Monitoring: Closely monitor system performance and error rates during and after migrations.
- Circuit breakers: Implement automatic rollback triggers if migrations cause significant performance degradation.
Documentation and Knowledge Sharing
- Schema documentation: Maintain up-to-date documentation of your database schema and its evolution.
- Migration history: Document the reasoning behind schema changes for future reference.
- Knowledge sharing: Share lessons learned from complex migrations with the entire development team.
Case Studies: Schema Evolution at Scale
Example 1: Adding a Column to a Billion-Row Table
Challenge: Adding a new column to a table with billions of rows without downtime.
Solution:
- Add the column with a NULL default value to avoid table rewrite.
- Deploy application changes to populate the column for new records.
- Create a background process to backfill the column for existing records in small batches.
- Once backfill is complete, add any necessary constraints or indexes.
Example 2: Splitting a Table for Improved Scalability
Challenge: Splitting a monolithic table into multiple tables to improve sharding capabilities.
Solution:
- Create new target tables with the desired structure.
- Set up dual-write logic in the application to write to both old and new tables.
- Create a background process to migrate historical data to the new structure.
- Verify data consistency between old and new structures.
- Gradually transition read operations to the new tables.
- Once all operations use the new tables, remove the old table.
Example 3: Changing Primary Key Structure
Challenge: Changing from auto-increment integer IDs to UUIDs for better sharding.
Solution:
- Add a new UUID column to the existing table.
- Generate UUIDs for all existing records.
- Create new foreign key columns in related tables.
- Update related records to include the new UUID foreign keys.
- Deploy application changes to use UUIDs for new records.
- Gradually transition queries to use UUID relationships.
- Eventually remove the original integer ID columns when no longer needed.
Schema versioning and migration are critical aspects of maintaining scalable database systems over time. By implementing robust versioning practices, choosing appropriate migration strategies, and carefully planning and executing schema changes, you can evolve your database schema to meet changing requirements while minimizing disruption and risk.
Remember that in large-scale systems, even seemingly simple schema changes can have significant performance implications. Always test migrations thoroughly, implement changes incrementally, and maintain backward compatibility whenever possible. With the right approach, your database schema can evolve gracefully alongside your application, supporting continued growth and innovation without becoming a bottleneck or source of instability.
Real-World Examples of Scalable Database Schemas
Understanding theoretical principles of database design is important, but seeing how these principles are applied in real-world scenarios can provide invaluable insights. This section presents several examples of database schemas designed for scalability across different domains. Each example illustrates specific scalability challenges and how they were addressed through thoughtful schema design.
E-Commerce Platform Schema
E-commerce platforms must handle high transaction volumes, seasonal traffic spikes, and ever-growing product catalogs while maintaining fast response times.
Core Schema Design
┌─────────────────┐ ┌─────────────────┐ ┌─────────────────┐
│ Users │ │ Orders │ │ Order_Items │
├─────────────────┤ ├─────────────────┤ ├─────────────────┤
│ user_id (PK) │ │ order_id (PK) │ │ item_id (PK) │
│ email │◄──────┤ user_id (FK) │ │ order_id (FK) │
│ password_hash │ │ order_date │◄──────┤ product_id (FK) │
│ first_name │ │ status │ │ quantity │
│ last_name │ │ shipping_address│ │ price │
│ created_at │ │ billing_address │ │ discount │
│ last_login │ │ payment_method │ └─────────────────┘
└─────────────────┘ │ total_amount │ │
│ └─────────────────┘ │
│ │
│ ▼
┌─────────────────┐ ┌─────────────────┐
│ User_Addresses │ │ Products │
├─────────────────┤ ├─────────────────┤
│ address_id (PK) │ │ product_id (PK) │
│ user_id (FK) │ │ name │
│ address_type │ │ description │
│ street │ │ base_price │
│ city │ │ category_id (FK)│
│ state │ │ inventory_count │
│ postal_code │ │ created_at │
│ country │ │ updated_at │
│ is_default │ └─────────────────┘
└─────────────────┘ │
│
▼
┌─────────────────┐
│ Categories │
├─────────────────┤
│ category_id (PK)│
│ name │
│ parent_id (FK) │
│ level │
│ path │
└─────────────────┘
Scalability Features
- Vertical Partitioning: Product details are separated from core product information:
CREATE TABLE products (
product_id BIGINT PRIMARY KEY,
name VARCHAR(200) NOT NULL,
base_price DECIMAL(10,2) NOT NULL,
category_id INT NOT NULL,
inventory_count INT NOT NULL,
created_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP,
updated_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
FOREIGN KEY (category_id) REFERENCES categories(category_id)
);
CREATE TABLE product_details (
product_id BIGINT PRIMARY KEY,
description TEXT,
specifications JSON,
dimensions VARCHAR(100),
weight DECIMAL(8,2),
FOREIGN KEY (product_id) REFERENCES products(product_id)
);
This separation allows the frequently accessed core product information to be optimized independently from the larger, less frequently accessed product details.
- Horizontal Partitioning for Orders: Orders are partitioned by date range:
CREATE TABLE orders_current_month (
order_id BIGINT PRIMARY KEY,
user_id BIGINT NOT NULL,
order_date TIMESTAMP NOT NULL,
-- other columns
CHECK (order_date >= '2025-04-01' AND order_date < '2025-05-01')
);
CREATE TABLE orders_archive_2025_q1 (
order_id BIGINT PRIMARY KEY,
user_id BIGINT NOT NULL,
order_date TIMESTAMP NOT NULL,
-- other columns
CHECK (order_date >= '2025-01-01' AND order_date < '2025-04-01')
);
This partitioning strategy allows for efficient querying of recent orders (which are accessed most frequently) while maintaining historical data for reporting and analysis.
- Denormalization for Product Search: A denormalized table for product search:
CREATE TABLE product_search (
product_id BIGINT PRIMARY KEY,
name VARCHAR(200) NOT NULL,
category_name VARCHAR(100) NOT NULL,
category_path VARCHAR(255) NOT NULL,
price DECIMAL(10,2) NOT NULL,
average_rating DECIMAL(3,2),
search_vector TSVECTOR,
FOREIGN KEY (product_id) REFERENCES products(product_id)
);
CREATE INDEX idx_product_search_vector ON product_search USING GIN(search_vector);
This denormalized table eliminates joins for common product search operations, improving search performance significantly.
- Sharding Strategy: User data is sharded by user_id ranges:
Shard 1: user_id 1-1,000,000
Shard 2: user_id 1,000,001-2,000,000
...and so on
Each shard contains all tables related to users in that range, including their orders, addresses, and reviews. This approach keeps related data together, minimizing cross-shard operations.
- Read Replicas: Multiple read replicas are deployed for product catalog data, which is read-heavy but updated infrequently.
Performance Considerations
-
Indexing Strategy:
- Composite indexes on (user_id, order_date) for order history queries
- Covering indexes for common product listing queries
- Partial indexes for active products only
-
Caching Layer:
- Product details cached at the application level
- Category hierarchy cached to avoid recursive queries
- Shopping cart data stored in a separate fast-access store (Redis)
-
Data Type Optimization:
- CHAR(2) for country and state codes
- Appropriate VARCHAR lengths based on actual data requirements
- JSON for flexible product specifications
Social Media Platform Schema
Social media platforms must handle complex relationships between users, high write volumes for content creation, and efficient content delivery.
Core Schema Design
┌─────────────────┐ ┌─────────────────┐ ┌─────────────────┐
│ Users │ │ Posts │ │ Comments │
├─────────────────┤ ├─────────────────┤ ├─────────────────┤
│ user_id (PK) │ │ post_id (PK) │ │ comment_id (PK) │
│ username │◄──────┤ user_id (FK) │◄──────┤ post_id (FK) │
│ email │ │ content │ │ user_id (FK) │
│ password_hash │ │ created_at │ │ content │
│ profile_picture │ │ updated_at │ │ created_at │
│ bio │ │ privacy_level │ │ parent_id (FK) │
│ created_at │ └─────────────────┘ └─────────────────┘
└─────────────────┘ │ ▲
│ │ │
│ ▼ │
│ ┌─────────────────┐ │
│ │ Post_Media │ │
│ ├─────────────────┤ │
│ │ media_id (PK) │ │
│ │ post_id (FK) │ │
│ │ media_type │ │
│ │ media_url │ │
│ │ thumbnail_url │ │
│ └─────────────────┘ │
│ │
▼ │
┌─────────────────┐ ┌─────────────────┐ │
│ Relationships │ │ Likes │ │
├─────────────────┤ ├─────────────────┤ │
│ relationship_id │ │ like_id (PK) │ │
│ follower_id (FK)│ │ user_id (FK) │ │
│ followed_id (FK)│ │ content_type │───────────────┘
│ created_at │ │ content_id │
│ status │ │ created_at │
└─────────────────┘ └─────────────────┘
Scalability Features
- Content Sharding: Posts are sharded by user_id:
-- Shard determination function (pseudocode)
function determine_shard(user_id) {
return "posts_shard_" + (user_id % 100);
}
-- Example of a post table on a specific shard
CREATE TABLE posts_shard_42 (
post_id BIGINT PRIMARY KEY,
user_id BIGINT NOT NULL,
content TEXT,
created_at TIMESTAMP NOT NULL,
updated_at TIMESTAMP,
privacy_level TINYINT NOT NULL DEFAULT 0,
CHECK (user_id % 100 = 42)
);
This approach keeps all posts from a single user on the same shard, optimizing for the common case of viewing a user's timeline.
- Polymorphic Associations: A single likes table for multiple content types:
CREATE TABLE likes (
like_id BIGINT PRIMARY KEY,
user_id BIGINT NOT NULL,
content_type TINYINT NOT NULL, -- 1=post, 2=comment, 3=photo
content_id BIGINT NOT NULL,
created_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP,
UNIQUE KEY (user_id, content_type, content_id)
);
CREATE INDEX idx_likes_content ON likes(content_type, content_id);
This design avoids creating separate like tables for each content type while still allowing efficient queries.
- Denormalized Counters: Frequently accessed counts are stored directly:
CREATE TABLE post_counters (
post_id BIGINT PRIMARY KEY,
like_count INT NOT NULL DEFAULT 0,
comment_count INT NOT NULL DEFAULT 0,
share_count INT NOT NULL DEFAULT 0,
view_count INT NOT NULL DEFAULT 0,
last_updated TIMESTAMP NOT NULL,
FOREIGN KEY (post_id) REFERENCES posts(post_id)
);
These counters are updated asynchronously to avoid locking during high-volume operations like liking a popular post.
- Feed Materialization: Pre-computed feeds for active users:
CREATE TABLE user_feeds (
feed_id BIGINT PRIMARY KEY,
user_id BIGINT NOT NULL,
post_id BIGINT NOT NULL,
author_id BIGINT NOT NULL,
score FLOAT NOT NULL, -- relevance score
created_at TIMESTAMP NOT NULL,
UNIQUE KEY (user_id, post_id)
);
CREATE INDEX idx_user_feeds_timeline ON user_feeds(user_id, score DESC);
This table stores pre-computed feed items, allowing for efficient timeline retrieval without complex joins or sorting at read time.
- Graph Database Integration: For complex relationship queries:
// Neo4j Cypher query example for friend-of-friend recommendations
MATCH (user:User {user_id: 12345})-[:FOLLOWS]->(friend)-[:FOLLOWS]->(fof)
WHERE NOT (user)-[:FOLLOWS]->(fof)
RETURN fof.user_id, count(friend) AS common_connections
ORDER BY common_connections DESC
LIMIT 10
While core user data remains in the relational database, a specialized graph database handles complex social queries.
Performance Considerations
-
Indexing Strategy:
- Composite indexes on (user_id, created_at) for timeline queries
- Covering indexes for notification queries
- Partial indexes for active users only
-
Caching Strategy:
- User profiles cached at the application level
- Post content cached in a distributed cache
- Feed items cached for active users
-
Write Optimization:
- Asynchronous counter updates
- Batched feed generation
- Eventual consistency for non-critical operations
IoT Data Platform Schema
IoT platforms must handle massive volumes of time-series data from millions of devices while supporting both real-time monitoring and historical analysis.
Core Schema Design
┌─────────────────┐ ┌─────────────────┐ ┌─────────────────┐
│ Organizations │ │ Devices │ │ Sensor_Data │
├─────────────────┤ ├─────────────────┤ ├─────────────────┤
│ org_id (PK) │ │ device_id (PK) │ │ reading_id (PK) │
│ name │◄──────┤ org_id (FK) │◄──────┤ device_id (FK) │
│ plan_level │ │ name │ │ sensor_type │
│ max_devices │ │ type │ │ timestamp │
│ created_at │ │ location │ │ value │
└─────────────────┘ │ status │ │ quality │
│ last_connected │ └─────────────────┘
└─────────────────┘
│
│
▼
┌─────────────────┐ ┌─────────────────┐
│ Device_Config │ │ Aggregated_Data│
├─────────────────┤ ├─────────────────┤
│ config_id (PK) │ │ agg_id (PK) │
│ device_id (FK) │ │ device_id (FK) │
│ config_key │ │ sensor_type │
│ config_value │ │ period_type │
│ updated_at │ │ period_start │
└─────────────────┘ │ min_value │
│ max_value │
│ avg_value │
│ sample_count │
└─────────────────┘
Scalability Features
- Hypertable Partitioning: Sensor data is stored in time-partitioned hypertables (using TimescaleDB or similar):
-- Create the base table
CREATE TABLE sensor_data (
reading_id BIGSERIAL PRIMARY KEY,
device_id UUID NOT NULL,
sensor_type SMALLINT NOT NULL,
timestamp TIMESTAMPTZ NOT NULL,
value DOUBLE PRECISION NOT NULL,
quality SMALLINT NOT NULL
);
-- Convert to hypertable with time partitioning
SELECT create_hypertable('sensor_data', 'timestamp',
chunk_time_interval => INTERVAL '1 day');
-- Add space partitioning by device_id
SELECT add_dimension('sensor_data', 'device_id', number_partitions => 16);
This approach creates automatic time-based partitions (chunks) and distributes them across multiple nodes based on device_id, optimizing both time-series queries and device-specific queries.
- Continuous Aggregation: Automatic rollup of time-series data:
-- Create a continuous aggregate view for hourly data
CREATE MATERIALIZED VIEW sensor_data_hourly
WITH (timescaledb.continuous) AS
SELECT
device_id,
sensor_type,
time_bucket('1 hour', timestamp) AS hour,
MIN(value) AS min_value,
MAX(value) AS max_value,
AVG(value) AS avg_value,
COUNT(*) AS sample_count
FROM sensor_data
GROUP BY device_id, sensor_type, hour;
-- Set refresh policy
SELECT add_continuous_aggregate_policy('sensor_data_hourly',
start_offset => INTERVAL '3 days',
end_offset => INTERVAL '1 hour',
schedule_interval => INTERVAL '1 hour');
This creates automatically updated aggregates that dramatically improve query performance for historical analysis while reducing storage requirements.
- Data Retention Policies: Automated data lifecycle management:
-- Keep raw data for 30 days
SELECT add_retention_policy('sensor_data', INTERVAL '30 days');
-- Keep hourly aggregates for 1 year
SELECT add_retention_policy('sensor_data_hourly', INTERVAL '1 year');
-- Keep daily aggregates for 5 years
SELECT add_retention_policy('sensor_data_daily', INTERVAL '5 years');
These policies automatically remove old data according to business requirements, keeping the database size manageable.
- Organization-Based Sharding: Multi-tenant isolation through sharding:
-- Each organization gets its own schema
CREATE SCHEMA org_12345;
-- Create organization-specific tables
CREATE TABLE org_12345.devices (
device_id UUID PRIMARY KEY,
name VARCHAR(100) NOT NULL,
type SMALLINT NOT NULL,
location GEOGRAPHY(POINT),
status SMALLINT NOT NULL,
last_connected TIMESTAMPTZ
);
This approach provides strong isolation between organizations while allowing for organization-specific optimizations.
- Hybrid Storage Model: Different storage engines for different data types:
- Time-series data: Specialized time-series database (TimescaleDB)
- Device metadata: Traditional relational tables
- Device location history: PostGIS spatial extensions
- Configuration settings: Key-value store (Redis)
Performance Considerations
-
Indexing Strategy:
- Time-based indexes for recent data queries
- Device-based indexes for device-specific queries
- Composite indexes for combined filtering
-
Query Optimization:
- Query routing to appropriate aggregation level based on time range
- Parallel query execution for large historical queries
- Approximate queries for dashboard visualizations
-
Write Optimization:
- Batch inserts for sensor data
- Asynchronous processing of non-critical updates
- Write-optimized storage for raw data ingestion
SaaS Application Platform Schema
SaaS platforms must support multi-tenancy while providing isolation, customization, and scalability for each tenant.
Core Schema Design
┌─────────────────┐ ┌─────────────────┐ ┌─────────────────┐
│ Tenants │ │ Tenant_Users │ │ Projects │
├─────────────────┤ ├─────────────────┤ ├─────────────────┤
│ tenant_id (PK) │ │ user_id (PK) │ │ project_id (PK) │
│ name │◄──────┤ tenant_id (FK) │◄──────┤ tenant_id (FK) │
│ subdomain │ │ email │ │ name │
│ plan_type │ │ name │ │ description │
│ max_users │ │ role │ │ created_by (FK) │
│ created_at │ │ last_login │ │ created_at │
└─────────────────┘ └─────────────────┘ └─────────────────┘
│ │
│ │
▼ ▼
┌─────────────────┐ ┌─────────────────┐
│ Tenant_Settings │ │ Tasks │
├─────────────────┤ ├─────────────────┤
│ setting_id (PK) │ │ task_id (PK) │
│ tenant_id (FK) │ │ project_id (FK) │
│ setting_key │ │ title │
│ setting_value │ │ description │
│ updated_at │ │ status │
└─────────────────┘ │ assigned_to (FK)│
│ due_date │
│ created_at │
└─────────────────┘
Scalability Features
- Schema-Based Multi-Tenancy: Each tenant gets its own schema:
-- Create tenant schema
CREATE SCHEMA tenant_12345;
-- Create tenant-specific tables
CREATE TABLE tenant_12345.projects (
project_id SERIAL PRIMARY KEY,
name VARCHAR(100) NOT NULL,
description TEXT,
created_by INT NOT NULL,
created_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP
);
This approach provides strong isolation between tenants while allowing for tenant-specific customizations.
- Shared Table Multi-Tenancy: For smaller tenants, a shared table approach:
CREATE TABLE shared.projects (
project_id SERIAL PRIMARY KEY,
tenant_id INT NOT NULL,
name VARCHAR(100) NOT NULL,
description TEXT,
created_by INT NOT NULL,
created_at TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (tenant_id) REFERENCES tenants(tenant_id)
);
-- Always filter by tenant_id
CREATE INDEX idx_projects_tenant ON shared.projects(tenant_id);
This design allows for efficient resource utilization while maintaining logical separation between tenants.
- Hybrid Approach: Combining schema-based and shared approaches:
-- Function to determine tenant storage strategy
CREATE OR REPLACE FUNCTION get_tenant_table(tenant_id INT, table_name TEXT)
RETURNS TEXT AS $$
DECLARE
tenant_plan TEXT;
BEGIN
SELECT plan_type INTO tenant_plan FROM tenants WHERE tenant_id = tenant_id;
IF tenant_plan IN ('enterprise', 'premium') THEN
RETURN 'tenant_' || tenant_id || '.' || table_name;
ELSE
RETURN 'shared.' || table_name;
END IF;
END;
$$ LANGUAGE plpgsql;
This function dynamically routes queries to either dedicated schemas for premium tenants or shared tables for basic tenants.
- Tenant-Specific Customizations: Extensible schema for custom fields:
CREATE TABLE custom_fields (
field_id SERIAL PRIMARY KEY,
tenant_id INT NOT NULL,
entity_type VARCHAR(50) NOT NULL, -- 'project', 'task', etc.
field_name VARCHAR(100) NOT NULL,
field_type VARCHAR(50) NOT NULL, -- 'text', 'number', 'date', etc.
is_required BOOLEAN NOT NULL DEFAULT FALSE,
default_value TEXT,
UNIQUE (tenant_id, entity_type, field_name)
);
CREATE TABLE custom_field_values (
value_id SERIAL PRIMARY KEY,
field_id INT NOT NULL,
entity_id INT NOT NULL,
value TEXT,
FOREIGN KEY (field_id) REFERENCES custom_fields(field_id)
);
This design allows tenants to define custom fields without requiring schema changes.
- Tenant-Based Sharding: Distributing tenants across database instances:
Shard 1: Tenants A-F
Shard 2: Tenants G-M
Shard 3: Tenants N-S
Shard 4: Tenants T-Z
Large enterprise tenants may get their own dedicated database instances for maximum isolation and performance.
Performance Considerations
-
Indexing Strategy:
- Tenant-specific indexes for large tenants
- Tenant-filtered indexes for shared tables
- Partial indexes for active projects only
-
Query Optimization:
- Row-level security policies for shared tables
- Query rewriting to include tenant filters
- Prepared statements with tenant context
-
Resource Isolation:
- Connection pooling with tenant-specific pools
- Resource quotas for large tenants
- Background job scheduling with tenant prioritization
Financial Transaction Processing Schema
Financial systems must handle high transaction volumes with absolute data integrity, comprehensive audit trails, and complex reporting requirements.
Core Schema Design
┌─────────────────┐ ┌─────────────────┐ ┌─────────────────┐
│ Accounts │ │ Transactions │ │ Transaction_Items│
├─────────────────┤ ├─────────────────┤ ├─────────────────┤
│ account_id (PK) │ │ txn_id (PK) │ │ item_id (PK) │
│ account_number │◄──────┤ from_account(FK)│◄──────┤ txn_id (FK) │
│ account_type │ │ to_account (FK) │ │ account_id (FK) │
│ currency │ │ amount │ │ amount │
│ balance │ │ currency │ │ type │
│ status │ │ txn_date │ │ balance_after │
│ owner_id │ │ status │ │ description │
└─────────────────┘ │ reference │ └─────────────────┘
└─────────────────┘
│
│
▼
┌─────────────────┐ ┌─────────────────┐
│ Audit_Trail │ │ Ledger_Entries │
├─────────────────┤ ├─────────────────┤
│ audit_id (PK) │ │ entry_id (PK) │
│ entity_type │ │ txn_id (FK) │
│ entity_id │ │ account_id (FK) │
│ action │ │ debit_amount │
│ old_values │ │ credit_amount │
│ new_values │ │ entry_date │
│ user_id │ │ journal_id │
│ timestamp │ └─────────────────┘
└─────────────────┘
Scalability Features
- Partitioning by Date: Transactions are partitioned by date:
CREATE TABLE transactions (
txn_id BIGINT PRIMARY KEY,
from_account BIGINT,
to_account BIGINT,
amount DECIMAL(18,2) NOT NULL,
currency CHAR(3) NOT NULL,
txn_date TIMESTAMP NOT NULL,
status VARCHAR(20) NOT NULL,
reference VARCHAR(100),
FOREIGN KEY (from_account) REFERENCES accounts(account_id),
FOREIGN KEY (to_account) REFERENCES accounts(account_id)
) PARTITION BY RANGE (txn_date);
CREATE TABLE transactions_2025_04 PARTITION OF transactions
FOR VALUES FROM ('2025-04-01') TO ('2025-05-01');
CREATE TABLE transactions_2025_03 PARTITION OF transactions
FOR VALUES FROM ('2025-03-01') TO ('2025-04-01');
This approach improves performance for date-range queries and simplifies archiving of historical data.
- Double-Entry Accounting: Every transaction creates balanced ledger entries:
CREATE TABLE ledger_entries (
entry_id BIGSERIAL PRIMARY KEY,
txn_id BIGINT NOT NULL,
account_id BIGINT NOT NULL,
debit_amount DECIMAL(18,2) NOT NULL DEFAULT 0,
credit_amount DECIMAL(18,2) NOT NULL DEFAULT 0,
entry_date TIMESTAMP NOT NULL,
journal_id BIGINT NOT NULL,
FOREIGN KEY (txn_id) REFERENCES transactions(txn_id),
FOREIGN KEY (account_id) REFERENCES accounts(account_id),
CHECK (debit_amount >= 0 AND credit_amount >= 0),
CHECK (debit_amount = 0 OR credit_amount = 0)
);
CREATE INDEX idx_ledger_account_date ON ledger_entries(account_id, entry_date);
This design ensures data integrity through balanced debits and credits while supporting efficient account statement generation.
- Materialized Account Balances: Pre-calculated account balances:
CREATE TABLE account_balances (
balance_id BIGSERIAL PRIMARY KEY,
account_id BIGINT NOT NULL,
as_of_date DATE NOT NULL,
opening_balance DECIMAL(18,2) NOT NULL,
closing_balance DECIMAL(18,2) NOT NULL,
total_debits DECIMAL(18,2) NOT NULL,
total_credits DECIMAL(18,2) NOT NULL,
UNIQUE (account_id, as_of_date),
FOREIGN KEY (account_id) REFERENCES accounts(account_id)
);
These pre-calculated balances dramatically improve performance for balance inquiries and statement generation.
- Comprehensive Audit Trail: Immutable audit records for all changes:
CREATE TABLE audit_trail (
audit_id BIGSERIAL PRIMARY KEY,
entity_type VARCHAR(50) NOT NULL,
entity_id BIGINT NOT NULL,
action VARCHAR(20) NOT NULL,
old_values JSONB,
new_values JSONB,
user_id BIGINT,
ip_address INET,
timestamp TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP
);
CREATE INDEX idx_audit_entity ON audit_trail(entity_type, entity_id);
CREATE INDEX idx_audit_timestamp ON audit_trail(timestamp);
This design captures all changes to the system in an immutable log, supporting compliance requirements and forensic analysis.
- Sharding by Account Range: Accounts and their transactions are sharded by account number ranges:
Shard 1: Account numbers 1000000-1999999
Shard 2: Account numbers 2000000-2999999
...and so on
This approach keeps related accounts and transactions together, minimizing cross-shard operations for common transaction patterns.
Performance Considerations
-
Indexing Strategy:
- Composite indexes for account statements (account_id, txn_date)
- Covering indexes for balance lookups
- Partial indexes for active accounts only
-
Transaction Processing:
- Two-phase commit for cross-shard transactions
- Optimistic locking for concurrent updates
- Read-committed isolation level for most operations
-
Reporting Optimization:
- Materialized views for common reports
- Summary tables updated in batch processes
- Separate reporting database with denormalized structures
Lessons from Real-World Examples
These examples illustrate several common patterns for scalable database design:
Domain-Specific Partitioning: Each domain has natural partitioning boundaries (time for financial transactions, users for social media, tenants for SaaS).
Strategic Denormalization: All examples include some form of denormalization (counters, materialized views, pre-computed feeds) to optimize for common access patterns.
Hybrid Storage Models: Many large-scale systems combine multiple database types to leverage their specific strengths (relational for transactions, time-series for IoT data, graph for social relationships).
Tiered Data Management: All examples implement some form of data lifecycle management, with different storage and access patterns for hot, warm, and cold data.
Scalability vs. Complexity Trade-offs: More complex schemas (with partitioning, sharding, and denormalization) enable greater scalability but require more sophisticated application logic and maintenance.
By studying these real-world examples and understanding the principles behind their design decisions, you can apply similar patterns to your own database schemas, adapting them to your specific domain and scalability requirements.
Summary of Key Scalability Principles for Database Schema Design
Throughout this guide, we've explored various aspects of database schema design with a focus on scalability. As systems grow in terms of data volume, user load, and complexity, the initial design decisions you make become increasingly important. This final section synthesizes the key principles that underpin scalable database design across all the topics we've covered.
Foundational Principles
1. Design for Growth from the Beginning
Scalability should never be an afterthought. While it's important not to over-engineer solutions for problems you don't yet have, certain fundamental decisions are difficult to change later:
Choose appropriate primary key strategies: Select primary key types and generation methods that will scale with your data. Auto-incrementing integers work well for single-node databases but can become bottlenecks in distributed systems. Consider UUIDs or other distributed ID generation approaches for systems that will eventually be sharded.
Plan for data volume expansion: Estimate how your data will grow over time and design schemas that can accommodate that growth. This includes selecting appropriate data types, planning for partitioning, and considering how indexes will scale.
Consider future access patterns: While you can't predict every way your application will evolve, thinking about likely future access patterns can help you make design decisions that won't need to be completely reversed later.
2. Balance Normalization and Denormalization
The tension between normalization (for data integrity and storage efficiency) and denormalization (for query performance) is at the heart of scalable schema design:
Start with proper normalization: Begin with a well-normalized schema to ensure data integrity and minimize redundancy. This provides a solid foundation for future evolution.
Strategically denormalize for performance: Identify specific performance bottlenecks and selectively denormalize to address them. This might include adding redundant data, creating summary tables, or materializing computed values.
Consider read-to-write ratios: Workloads with high read-to-write ratios often benefit from more aggressive denormalization, while write-heavy workloads may perform better with more normalized schemas.
Use hybrid approaches: Many scalable systems maintain core transactional data in normalized form while creating denormalized views or projections for specific access patterns.
3. Design for Distribution
Modern scalable systems almost inevitably involve some form of data distribution across multiple nodes:
Identify natural sharding keys: Look for attributes in your data model that provide natural boundaries for distribution, such as customer ID, geographic region, or time periods.
Minimize cross-shard operations: Design your schema to keep related data together on the same shard whenever possible to reduce the need for distributed transactions or joins.
Plan for eventual consistency: In distributed systems, immediate consistency across all nodes is often impractical. Design your schema and application logic to handle eventual consistency where appropriate.
Consider replication strategies: Determine which data needs to be replicated across all nodes (reference data, configuration) versus data that should be partitioned (transactional data, user-specific data).
Technical Implementation Principles
4. Optimize Indexing Strategies
Indexes are powerful tools for improving query performance, but they come with trade-offs:
Index for high-impact queries: Focus on queries that run frequently or consume significant resources rather than trying to optimize every possible query path.
Consider index overhead: Each index adds write overhead and storage requirements. Be selective about which columns to index.
Use composite indexes strategically: Order columns in composite indexes based on query patterns, typically with equality predicates before range predicates.
Leverage specialized index types: Many databases offer specialized indexes (partial, functional, spatial, full-text) that can dramatically improve performance for specific query patterns.
Monitor and maintain indexes: Regularly review index usage and performance, removing unused indexes and rebuilding fragmented ones.
5. Implement Effective Partitioning
Partitioning divides large tables into smaller, more manageable pieces:
Choose appropriate partitioning keys: Select partitioning keys based on common query patterns, typically time-based for historical data or entity-based for multi-tenant systems.
Balance partition sizes: Aim for relatively even distribution of data across partitions to avoid hotspots.
Align partitioning with data lifecycle: Design partitioning schemes that facilitate archiving, purging, or moving data to different storage tiers as it ages.
Consider query patterns: Ensure that common queries can benefit from partition pruning, where the database engine can skip irrelevant partitions.
6. Select Appropriate Data Types
Data type selection affects storage requirements, processing efficiency, and ultimately scalability:
Use the smallest sufficient data type: Choose data types that can accommodate your data (including future growth) without excessive overhead.
Consider processing efficiency: Some data types are more efficiently processed than others. For high-volume operations, this can make a significant difference.
Be consistent across related columns: Use the same data types for columns that will be joined or compared to avoid implicit conversions.
Leverage specialized data types: Modern databases offer specialized data types for common data categories (JSON, spatial, network addresses) that can improve both developer productivity and performance.
7. Plan for Schema Evolution
Schemas inevitably change over time. Planning for this evolution is crucial for maintaining scalability:
Implement robust versioning: Track all schema changes with explicit versioning to ensure consistent deployment across environments.
Use non-destructive migration patterns: Prefer additive changes and multi-phase migration patterns that maintain backward compatibility during transitions.
Test migrations thoroughly: Validate migrations on representative datasets to understand their performance impact before applying them to production.
Automate schema changes: Develop automated processes for applying schema changes consistently across all environments, including sharded or distributed databases.
Operational Principles
8. Monitor and Measure
Scalability is not a one-time achievement but an ongoing process:
Establish performance baselines: Measure and document the performance of key queries under normal conditions to help identify degradation over time.
Monitor query performance: Regularly review slow query logs and performance metrics to identify emerging bottlenecks.
Track growth rates: Monitor how quickly your data is growing and how this affects performance to anticipate scaling needs before they become critical.
Test at scale: Periodically test your system with data volumes and traffic patterns that exceed your current needs to identify potential scalability issues early.
9. Evolve Incrementally
Scalability improvements are most effective when implemented incrementally:
Address the current bottleneck: Focus on the most immediate constraint rather than trying to solve all potential scalability issues at once.
Measure the impact: Quantify the improvement from each change to ensure it's providing the expected benefit.
Maintain simplicity: Prefer simpler solutions that address specific problems over complex architectures designed to solve hypothetical future issues.
Learn and adapt: Use each scaling challenge as an opportunity to refine your understanding of your system's behavior and improve your approach to future challenges.
10. Consider the Full Technology Stack
Database schema design is just one aspect of overall system scalability:
Balance database and application logic: Determine which operations should be handled by the database versus the application based on their respective strengths.
Leverage caching strategically: Implement caching at appropriate levels (database, application, API) to reduce database load for frequently accessed data.
Consider alternative data stores: Some workloads may be better served by specialized databases (document, graph, time-series) rather than trying to force everything into a relational model.
Optimize the entire data path: Look beyond the database to network configuration, client-side processing, and API design for end-to-end performance optimization.
Domain-Specific Principles
11. E-Commerce Systems
Separate transactional and analytical data: Maintain clean separation between order processing and reporting/analytics to prevent analytical queries from impacting customer experience.
Plan for seasonal spikes: Design schemas that can handle order volumes many times higher than average during peak periods.
Optimize for product discovery: Create specialized structures (search indexes, recommendation tables) to support efficient product discovery.
12. Social and Content Platforms
Design for content virality: Ensure that suddenly popular content doesn't create database hotspots that degrade performance.
Optimize for feed generation: Pre-compute or cache personalized feeds rather than generating them on demand for each user request.
Balance consistency requirements: Determine which operations require strong consistency (financial transactions) versus those that can tolerate eventual consistency (like counts, comment threads).
13. IoT and Time-Series Data
Prioritize write performance: Design for high-volume data ingestion with minimal overhead.
Implement data lifecycle policies: Automatically aggregate and archive older data to maintain performance as data volumes grow.
Optimize for time-based queries: Ensure that time-range queries remain efficient even as historical data accumulates.
14. Multi-Tenant SaaS Applications
Isolate tenant data: Design schemas that prevent one tenant's activities from impacting others, either through separate schemas or robust filtering.
Support tenant-specific customization: Create flexible schemas that allow for tenant-specific fields and workflows without requiring schema changes.
Plan for tenant diversity: Accommodate tenants of vastly different sizes and activity levels within the same overall architecture.
Conclusion: The Art of Scalable Schema Design
Designing database schemas for scalability is as much an art as it is a science. It requires balancing competing concerns, making trade-offs based on specific requirements, and continuously adapting as systems evolve. The principles outlined in this guide provide a foundation for making informed decisions, but every system has unique characteristics that will influence the optimal approach.
Remember that scalability is not an absolute quality but a relative one—a system is scalable if it can grow to meet the specific demands placed upon it. By understanding the fundamental principles of scalable schema design and applying them thoughtfully to your specific context, you can create database systems that grow gracefully with your application, supporting your business needs without becoming a limiting factor.
The most successful database architects combine deep technical knowledge with pragmatism, focusing on solving real problems rather than achieving theoretical perfection. They understand that scalability is a journey rather than a destination—a continuous process of monitoring, learning, and adapting as requirements evolve.
By applying the principles discussed throughout this guide, you'll be well-equipped to design database schemas that not only meet your current needs but can scale effectively as your data, user base, and application complexity grow over time.