Create a Simple API with Python and Flask
https://github.com/dcs-ink/simple-api-python-flask I recently requested my data from Indeed because I was curious about the statistics with my recent job search. I've worked plenty with APIs, but never built one from scratch. I decided to create a simple one using Python and Flask. It's simple really, only consists of two files - the python script and the json file. jobs.json job_api.py Here's a snippet example of the json file I received from Indeed. [ { "title" : "Job Title", "company" : "CoolCompany", "location" : "Cool City, COOL", "date" : "date timestamp" } ] Mine had something like 300 entries, but the point remains the same. Here's the python script import json from flask import Flask, jsonify app = Flask(__name__) jobs_data = [] try: with open('jobs.json', 'r') as f: jobs_data = json.load(f) print(f"Successfully loaded {len(jobs_data)} jobs from jobs.json") except FileNotFoundError: print("Error: jobs.json not found!") except json.JSONDecodeError: print("Error: jobs.json is not valid JSON!") @app.route('/api/jobs', methods=['GET']) def get_all_jobs(): return jsonify(jobs_data) @app.route('/api/jobs/titles', methods=['GET']) def get_job_titles(): titles = [job['title'] for job in jobs_data] return jsonify(titles) @app.route('/api/jobs/companies', methods=['GET']) def get_job_companies(): if not jobs_data: return jsonify({"error": "No job data available"}), 500 companies = list(set(job.get('company', 'N/A') for job in jobs_data)) return jsonify(companies) @app.route('/api/jobs/location', methods=['GET']) def get_job_locations(): if not jobs_data: return jsonify({"error": "No job data available"}), 500 locations = list(set(job.get('location') for job in jobs_data)) return jsonify(locations) if __name__ == '__main__': app.run(debug=True, port=8080) Steps to make it work. After setup and activating a virtual environment... pip install flask pip install jsonify Create python file and paste above python code Create json file and paste above json code Run python script sudo python3 job_api.py Use curl to test curl http://127.0.0.1:8080/api/jobs Response [ { "company": "CoolCompany", "date": "date timestamp", "location": "Cool City, COOL", "title": "Job Title" } ]
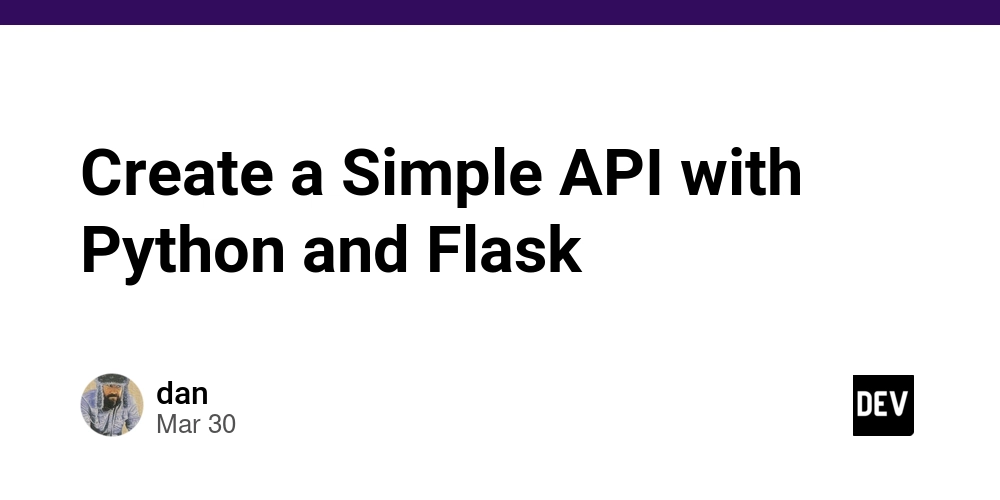
https://github.com/dcs-ink/simple-api-python-flask
I recently requested my data from Indeed because I was curious about the statistics with my recent job search.
I've worked plenty with APIs, but never built one from scratch. I decided to create a simple one using Python and Flask.
It's simple really, only consists of two files - the python script and the json file.
jobs.json
job_api.py
Here's a snippet example of the json file I received from Indeed.
[
{
"title" : "Job Title",
"company" : "CoolCompany",
"location" : "Cool City, COOL",
"date" : "date timestamp"
}
]
Mine had something like 300 entries, but the point remains the same.
Here's the python script
import json
from flask import Flask, jsonify
app = Flask(__name__)
jobs_data = []
try:
with open('jobs.json', 'r') as f:
jobs_data = json.load(f)
print(f"Successfully loaded {len(jobs_data)} jobs from jobs.json")
except FileNotFoundError:
print("Error: jobs.json not found!")
except json.JSONDecodeError:
print("Error: jobs.json is not valid JSON!")
@app.route('/api/jobs', methods=['GET'])
def get_all_jobs():
return jsonify(jobs_data)
@app.route('/api/jobs/titles', methods=['GET'])
def get_job_titles():
titles = [job['title'] for job in jobs_data]
return jsonify(titles)
@app.route('/api/jobs/companies', methods=['GET'])
def get_job_companies():
if not jobs_data:
return jsonify({"error": "No job data available"}), 500
companies = list(set(job.get('company', 'N/A') for job in jobs_data))
return jsonify(companies)
@app.route('/api/jobs/location', methods=['GET'])
def get_job_locations():
if not jobs_data:
return jsonify({"error": "No job data available"}), 500
locations = list(set(job.get('location') for job in jobs_data))
return jsonify(locations)
if __name__ == '__main__':
app.run(debug=True, port=8080)
Steps to make it work.
After setup and activating a virtual environment...
- pip install flask
- pip install jsonify
- Create python file and paste above python code
- Create json file and paste above json code
- Run python script
sudo python3 job_api.py
- Use curl to test
curl http://127.0.0.1:8080/api/jobs
Response
[
{
"company": "CoolCompany",
"date": "date timestamp",
"location": "Cool City, COOL",
"title": "Job Title"
}
]