Concurrency Showdown: Goroutines and Channels vs. C#'s async/await and TPL
In today's software landscape, handling multiple operations simultaneously isn't just a nice-to-have—it's essential for building responsive, efficient applications. As a C# developer, you've likely mastered the async/await pattern and Task Parallel Library (TPL). When diving into Go, you'll encounter a fundamentally different approach to concurrency: goroutines and channels. This article explores how these two concurrency models compare, highlighting their strengths, limitations, and ideal use cases. By understanding both approaches, you'll be better equipped to choose the right tool for your specific needs. The Philosophy: Two Different Worldviews Before diving into implementation details, it's important to understand the philosophical difference between these approaches: C#'s approach: "Communicate by sharing memory" - Objects are shared between threads, with synchronization mechanisms protecting access. Go's approach: "Share memory by communicating" - Data is passed between goroutines through channels, minimizing shared state. This fundamental difference shapes how you design concurrent systems in each language. C#'s Concurrency Model: async/await and TPL C#'s concurrency model centers around the Task class, which represents an asynchronous operation. The async/await pattern, introduced in C# 5.0, makes working with these tasks more intuitive. async/await: Simplified Asynchronous Programming The async/await pattern allows you to write asynchronous code that resembles synchronous code, making it easier to reason about: public async Task FetchDataAsync(string url) { using (HttpClient client = new HttpClient()) { string result = await client.GetStringAsync(url); return result.ToUpper(); // Process after awaiting } } // Usage string processedData = await FetchDataAsync("https://api.example.com/data"); Console.WriteLine(processedData); Under the hood, the compiler transforms this code into a state machine that manages the suspension and resumption of execution. Task Parallel Library: Rich Concurrency Tools For more complex scenarios, C# offers the Task Parallel Library (TPL), which provides tools for parallel processing and advanced task management: // Process items in parallel var results = await Task.WhenAll( urls.Select(url => FetchDataAsync(url)) ); // For CPU-bound work Parallel.ForEach(items, item => { ProcessItem(item); }); // Cancellation support CancellationTokenSource cts = new CancellationTokenSource(); cts.CancelAfter(TimeSpan.FromSeconds(5)); // Timeout after 5 seconds try { var result = await FetchDataAsync(url, cts.Token); } catch (OperationCanceledException) { Console.WriteLine("Operation timed out"); } Error Handling in C# Concurrency C# leverages its exception system for error handling in asynchronous code: public async Task FetchWithErrorHandlingAsync(string url) { try { using (HttpClient client = new HttpClient()) { return await client.GetStringAsync(url); } } catch (HttpRequestException ex) { Console.WriteLine($"Request failed: {ex.Message}"); return string.Empty; } catch (TaskCanceledException) { Console.WriteLine("Request timed out"); return string.Empty; } } This approach integrates well with C#'s existing error-handling mechanisms, allowing exceptions to propagate through async calls. Go's Concurrency Model: Goroutines and Channels Go's concurrency is built around two primary concepts: goroutines (lightweight threads) and channels (typed communication conduits). Goroutines: Lightweight Concurrency Goroutines are functions that run concurrently with other functions. They're extremely lightweight, allowing you to spawn thousands or even millions in a single application: func fetchData(url string) (string, error) { resp, err := http.Get(url) if err != nil { return "", err } defer resp.Body.Close() body, err := io.ReadAll(resp.Body) if err != nil { return "", err } return strings.ToUpper(string(body)), nil } // Launch a goroutine go fetchData("https://api.example.com/data") // Note: This doesn't return a value directly Unlike C# tasks, goroutines don't return values directly. For that, we need channels. Channels: Communication and Synchronization Channels provide a way for goroutines to communicate and synchronize their execution: func fetchData(url string, resultChan chan
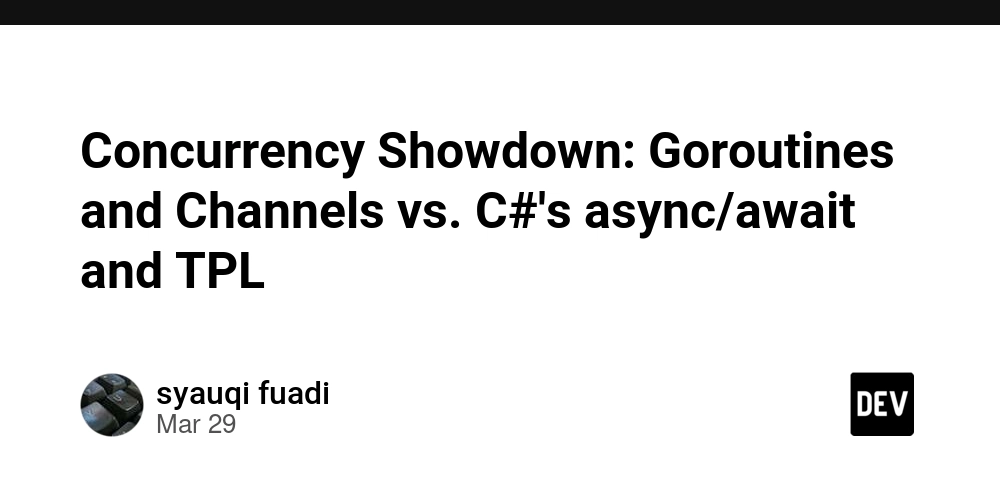
In today's software landscape, handling multiple operations simultaneously isn't just a nice-to-have—it's essential for building responsive, efficient applications. As a C# developer, you've likely mastered the async/await pattern and Task Parallel Library (TPL). When diving into Go, you'll encounter a fundamentally different approach to concurrency: goroutines and channels.
This article explores how these two concurrency models compare, highlighting their strengths, limitations, and ideal use cases. By understanding both approaches, you'll be better equipped to choose the right tool for your specific needs.
The Philosophy: Two Different Worldviews
Before diving into implementation details, it's important to understand the philosophical difference between these approaches:
- C#'s approach: "Communicate by sharing memory" - Objects are shared between threads, with synchronization mechanisms protecting access.
- Go's approach: "Share memory by communicating" - Data is passed between goroutines through channels, minimizing shared state.
This fundamental difference shapes how you design concurrent systems in each language.
C#'s Concurrency Model: async/await and TPL
C#'s concurrency model centers around the Task
class, which represents an asynchronous operation. The async/await pattern, introduced in C# 5.0, makes working with these tasks more intuitive.
async/await: Simplified Asynchronous Programming
The async/await pattern allows you to write asynchronous code that resembles synchronous code, making it easier to reason about:
public async Task<string> FetchDataAsync(string url)
{
using (HttpClient client = new HttpClient())
{
string result = await client.GetStringAsync(url);
return result.ToUpper(); // Process after awaiting
}
}
// Usage
string processedData = await FetchDataAsync("https://api.example.com/data");
Console.WriteLine(processedData);
Under the hood, the compiler transforms this code into a state machine that manages the suspension and resumption of execution.
Task Parallel Library: Rich Concurrency Tools
For more complex scenarios, C# offers the Task Parallel Library (TPL), which provides tools for parallel processing and advanced task management:
// Process items in parallel
var results = await Task.WhenAll(
urls.Select(url => FetchDataAsync(url))
);
// For CPU-bound work
Parallel.ForEach(items, item => {
ProcessItem(item);
});
// Cancellation support
CancellationTokenSource cts = new CancellationTokenSource();
cts.CancelAfter(TimeSpan.FromSeconds(5)); // Timeout after 5 seconds
try {
var result = await FetchDataAsync(url, cts.Token);
}
catch (OperationCanceledException) {
Console.WriteLine("Operation timed out");
}
Error Handling in C# Concurrency
C# leverages its exception system for error handling in asynchronous code:
public async Task<string> FetchWithErrorHandlingAsync(string url)
{
try
{
using (HttpClient client = new HttpClient())
{
return await client.GetStringAsync(url);
}
}
catch (HttpRequestException ex)
{
Console.WriteLine($"Request failed: {ex.Message}");
return string.Empty;
}
catch (TaskCanceledException)
{
Console.WriteLine("Request timed out");
return string.Empty;
}
}
This approach integrates well with C#'s existing error-handling mechanisms, allowing exceptions to propagate through async calls.
Go's Concurrency Model: Goroutines and Channels
Go's concurrency is built around two primary concepts: goroutines (lightweight threads) and channels (typed communication conduits).
Goroutines: Lightweight Concurrency
Goroutines are functions that run concurrently with other functions. They're extremely lightweight, allowing you to spawn thousands or even millions in a single application:
func fetchData(url string) (string, error) {
resp, err := http.Get(url)
if err != nil {
return "", err
}
defer resp.Body.Close()
body, err := io.ReadAll(resp.Body)
if err != nil {
return "", err
}
return strings.ToUpper(string(body)), nil
}
// Launch a goroutine
go fetchData("https://api.example.com/data") // Note: This doesn't return a value directly
Unlike C# tasks, goroutines don't return values directly. For that, we need channels.
Channels: Communication and Synchronization
Channels provide a way for goroutines to communicate and synchronize their execution:
func fetchData(url string, resultChan chan<- string, errorChan chan<- error) {
resp, err := http.Get(url)
if err != nil {
errorChan <- err
return
}
defer resp.Body.Close()
body, err := io.ReadAll(resp.Body)
if err != nil {
errorChan <- err
return
}
resultChan <- strings.ToUpper(string(body))
}
// Usage
resultChan := make(chan string)
errorChan := make(chan error)
go fetchData("https://api.example.com/data", resultChan, errorChan)
// Wait for result or error
select {
case result := <-resultChan:
fmt.Println("Success:", result)
case err := <-errorChan:
fmt.Println("Error:", err)
case <-time.After(5 * time.Second):
fmt.Println("Timeout")
}
The select
statement above demonstrates one of Go's powerful concurrency features: the ability to wait on multiple channel operations simultaneously, including a timeout.
Error Handling in Go Concurrency
Go handles errors in concurrent code by explicitly passing error values through channels or returning them from functions:
func worker(jobs <-chan int, results chan<- int, errors chan<- error) {
for job := range jobs {
// Simulate work that might fail
if job%2 == 0 {
errors <- fmt.Errorf("job %d failed", job)
continue
}
results <- job * 2
}
}
This explicit error handling promotes resilience and makes error flows more visible in concurrent code.
Performance and Resource Comparison
When comparing the performance characteristics of these models, several key differences emerge:
Characteristic | C# (async/await, TPL) | Go (goroutines) |
---|---|---|
Memory per concurrent operation | ~1MB (thread pool thread) | ~2KB (goroutine) |
Max practical concurrency | Thousands | Millions |
Startup overhead | Higher | Lower |
Context switching cost | OS-dependent | Runtime-managed |
Scheduling | Thread pool | Go runtime scheduler |
These differences translate to practical advantages in different scenarios:
- High-concurrency scenarios: Go's lightweight goroutines excel when you need to handle tens of thousands of concurrent operations, such as in web servers or real-time systems.
- CPU-bound operations: C#'s mature JIT compiler and optimized libraries can sometimes provide better performance for compute-intensive tasks.
- Memory-constrained environments: Go's smaller memory footprint per concurrent operation makes it more efficient in environments with limited resources.
Advanced Patterns Comparison
Let's examine how each language handles common concurrency patterns:
Fan-Out/Fan-In Pattern
This pattern involves distributing work to multiple workers and then collecting their results.
C# Implementation:
public async Task<List<Result>> ProcessItemsAsync(List<Item> items)
{
// Fan out: start all tasks
var tasks = items.Select(item => ProcessItemAsync(item)).ToList();
// Fan in: wait for all results
var results = await Task.WhenAll(tasks);
return results.ToList();
}
Go Implementation:
func processItems(items []Item) []Result {
numWorkers := min(len(items), 10) // Limit number of workers
// Create channels
jobs := make(chan Item, len(items))
results := make(chan Result, len(items))
// Fan out: start workers
for w := 0; w < numWorkers; w++ {
go worker(jobs, results)
}
// Send jobs
for _, item := range items {
jobs <- item
}
close(jobs)
// Fan in: collect results
var processedResults []Result
for i := 0; i < len(items); i++ {
processedResults = append(processedResults, <-results)
}
return processedResults
}
func worker(jobs <-chan Item, results chan<- Result) {
for item := range jobs {
results <- processItem(item)
}
}
Go's approach makes the concurrency pattern more explicit, with channels clearly showing the flow of data between goroutines.
Timeout Handling
Both languages support timeouts, but with different approaches:
C# Implementation:
public async Task<string> FetchWithTimeoutAsync(string url)
{
using var cts = new CancellationTokenSource(TimeSpan.FromSeconds(5));
try {
using var client = new HttpClient();
return await client.GetStringAsync(url, cts.Token);
}
catch (TaskCanceledException) {
return "Request timed out";
}
}
Go Implementation:
func fetchWithTimeout(url string) string {
resultChan := make(chan string, 1)
go func() {
result, err := fetchData(url)
if err != nil {
resultChan <- "Error: " + err.Error()
return
}
resultChan <- result
}()
select {
case result := <-resultChan:
return result
case <-time.After(5 * time.Second):
return "Request timed out"
}
}
Go's select
statement provides a more direct way to handle timeouts without requiring exception handling.
Real-World Example: API Aggregator
Let's compare implementations of a service that aggregates data from multiple APIs:
C# Implementation:
public async Task<AggregateResult> GetAggregateDataAsync()
{
var result = new AggregateResult();
// Start all requests concurrently
var userTask = _userService.GetUserDataAsync();
var productTask = _productService.GetProductsAsync();
var analyticsTask = _analyticsService.GetStatsAsync();
// Create a timeout for the entire operation
using var cts = new CancellationTokenSource(TimeSpan.FromSeconds(5));
try {
// Wait for all tasks to complete or timeout
await Task.WhenAll(
userTask.AsTask().WaitAsync(cts.Token),
productTask.AsTask().WaitAsync(cts.Token),
analyticsTask.AsTask().WaitAsync(cts.Token)
);
// Assign results
result.UserData = await userTask;
result.Products = await productTask;
result.Stats = await analyticsTask;
}
catch (OperationCanceledException) {
_logger.LogWarning("Aggregate data request timed out");
// Partial results may still be available
}
catch (Exception ex) {
_logger.LogError(ex, "Failed to aggregate data");
// Handle specific service failures
}
return result;
}
Go Implementation:
func GetAggregateData() AggregateResult {
result := AggregateResult{}
// Create channels for results
userChan := make(chan UserData, 1)
productChan := make(chan []Product, 1)
statsChan := make(chan Analytics, 1)
errorChan := make(chan error, 3) // Collect errors
// Launch goroutines for each service
go func() {
userData, err := userService.GetUserData()
if err != nil {
errorChan <- fmt.Errorf("user service: %w", err)
return
}
userChan <- userData
}()
go func() {
products, err := productService.GetProducts()
if err != nil {
errorChan <- fmt.Errorf("product service: %w", err)
return
}
productChan <- products
}()
go func() {
stats, err := analyticsService.GetStats()
if err != nil {
errorChan <- fmt.Errorf("analytics service: %w", err)
return
}
statsChan <- stats
}()
// Use select with timeout to collect results
timeout := time.After(5 * time.Second)
servicesCompleted := 0
for servicesCompleted < 3 {
select {
case result.UserData = <-userChan:
servicesCompleted++
case result.Products = <-productChan:
servicesCompleted++
case result.Stats = <-statsChan:
servicesCompleted++
case err := <-errorChan:
log.Printf("Service error: %v", err)
servicesCompleted++
case <-timeout:
log.Println("Aggregate data request timed out")
return result // Return partial results
}
}
return result
}
The Go version demonstrates several advantages:
- Built-in timeout handling via
select
- Clear error propagation through channels
- Natural composition of concurrent operations
- Explicit control over partial results
However, the C# version benefits from:
- Integration with existing exception handling
- More concise syntax for launching tasks
- Stronger type safety through the task return types
When to Choose Which Model
Based on the comparison, here are guidelines for when to choose each approach:
Choose C# async/await and TPL when:
- Working within the .NET ecosystem
- Building UI applications (WPF, Blazor, etc.)
- Leveraging complex LINQ operations
- Working with strongly-typed hierarchies
- Integrating with existing C# codebases
- Building complex business logic with rich domain models
Choose Go goroutines and channels when:
- Building high-concurrency services or APIs
- Working with limited memory resources
- Creating microservices from scratch
- Implementing network protocols or proxies
- Developing CLI tools that need concurrency
- Building systems where performance predictability is crucial
Adapting Your Mental Model
For C# developers transitioning to Go, the biggest challenge isn't syntax—it's shifting your mental model:
- Think communication, not synchronization: Instead of protecting shared state with locks, pass messages between goroutines.
- Embrace simplicity: Go's concurrency primitives are fewer but more composable.
- Consider resource usage: Goroutines enable concurrency patterns that would be prohibitively expensive in C#.
- Explicit error handling: Errors are values to be checked, not exceptions to be caught.
Conclusion: Different Tools for Different Jobs
Neither concurrency model is universally superior. C#'s approach excels in object-oriented environments with complex business logic, while Go's lightweight goroutines shine in high-throughput scenarios.
As a C# developer exploring Go, you'll find that understanding both models makes you more effective at designing concurrent systems. You might even find yourself bringing Go's philosophy back to your C# code, creating more explicit communication patterns and simpler concurrency models.
By mastering both approaches, you gain flexibility in designing systems that require concurrency at scale, whether you're building microservices, data processors, or network applications.
Next up: Exploring Go's dependency management compared to NuGet, and why Go modules might change how you think about package versioning.